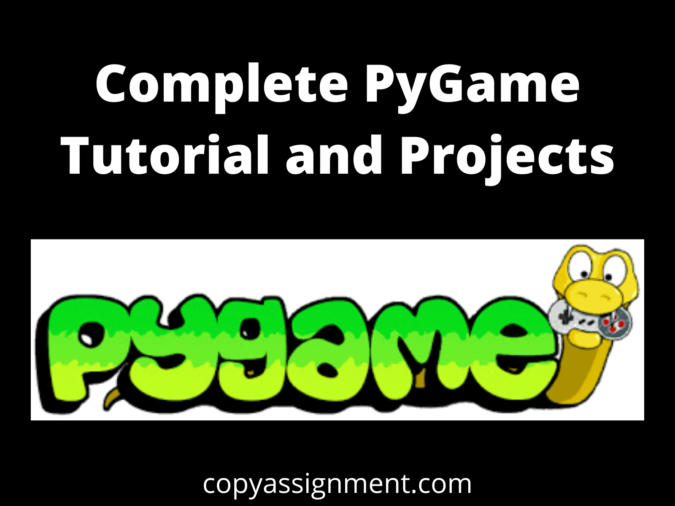
Without a doubt, we can say that Python is a multi-purpose language. Ranging from basic programming to its application in Data Science, from Machine Learning to Artificial Intelligence, and also in Developing Games. In simple words, it’s the language that is incomparable.
With knowing the advantages of Python, today, in this article of Complete PyGame Tutorial and Projects, we are going to check out Python’s library which is PyGame. We will learn about its basic functions, methods, etc, and then as usual develop projects, to get hands-on experience and apply what we have learned.
Before starting with the projects, first, we will learn about built-in functions, methods, etc in the
Contents of the article:
There will be two main parts
- Basic Overview
- Projects with Explanation
Basic Overview:
What is PyGame?
Pygame is a collection of Python modules that were created to contribute to the development of video games and other multimedia applications. The Pygame framework comprises a number of modules that provide functions for drawing images, playing audio, handling mouse input, and other tasks that you’ll encounter while programming games in Python. It has extra features that make it simple to construct fully functional games. Learning Pygame is a great place to start if you want to be a game developer. It also assists you in exploring various programming options.
PyGame Installation, Check, and Upgradation:
Install PyGame:
pip install pygame
To check whether your machine has the PyGame library or not then use the below command:
pip show pygame
If present already and want to upgrade then this command is used:
pip install pygame --upgrade

Importing and Initializing the library:
To import the library use the below command:
import pygame
This library imports all of the pygame modules that are accessible into the pygame package. If you don’t see any errors and get the following message:
“Hello from the pygame community. https://www.pygame.org/contribute.html“
then this means that the pygame library was properly imported, and you’re ready to go.
To initialize the library
pygame.init()
This is used to initialize all the important modules that might be needed during the development.
Adding a screen:
Whatever we code has an output and to check that output a screen is required in the case of the pygame library. It is the first task we complete in order to be able to display our output on something. Here’s how to get a blank screen.
pygame.display.set_mode((window_width,window_height))
Explanation:
pygame provides a separate module for display settings i.e display and here we have used a function named set_mode that takes two parameters width and the height of the window.
Changing screen background color:
colour = (5,46,255)
pygame.surface.fill(colour)
Output:

Explanation:
To fill the color of the screen we make use of the surface.fill()
Naming the window:
This means that pygame allows us to name the window that we just generated. To change the name we use the display module.
pygame.display.set_caption('add_title_here')
Explanation:
set_caption function is used. The parameter for this function is nothing else but the name that the user wants for the window.
Adding an icon:
add_img = pygame.image.load('add_image_name')
pygame.display.set_icon(add_img )
Explanation:
In order to add an icon, in the first line, we used the image module and passed the name of the image as an argument to the function load, and stored it in a variable named add_img
In the second line, we used a function from the display module and passed the variable add_img as the argument.
Accepting keyboard input:
Moving ahead in Complete PyGame Tutorial and Projects article, the next topic that we are going to learn is accepting input from users. In a game, taking the player’s input is very important. This can be done using the keyboard. But ever wondered how will the computer know?
To do so, we must be able to see all the activities that are taking place. With the events.get() function, we can observe how Pygame maintains track of what happens. In this part of the article, we’ll look at how to get and use different keyboard inputs in Pygame. pygame.event() queues the pygame.KEYDOWN and pygame.KEYUP events whenever a key is pressed or released.
Here’s a table of important keys in pygame and its corresponding description:
KEYS | DESCRIPTION |
K_0 to K_9 | 0 to 9 digits |
K_SPACE | Sets a space |
K_a to K_z | a to z alphabets |
K_UP | Upward arrow |
K_DOWN | Downward arrow |
K_RIGHT | Right side arrow |
K_LEFT | Left side arrow |
K_RSHIFT | Right side shift |
K_LSHIFT | Left side shift |
K_NUMLOCK | Number lock |
K_CAPSLOCK | Caps lock |
Adding images to the window:
Adding an image to our pygame screen is way easy. Images enhance the overall gaming experience of the player and make the visualization more soothing to the eyes.
Syntax:
pygame.image.load("image_name_or_path")
Determining the width and height of an image:
We learned how to add an image in the pygame window. Now let’s move ahead and learn how to get the width and height of any image. For this purpose, there are two methods available. They are as follows:
- Using get_size()
- And using get_height() and get_width()
With get_size():
Syntax:
img_size = py.image.load('image_path')
print("size : (width,height): ", img_size.get_size())
Explanation:
Here, we first loaded the image and stored it in an img_size object. And then we printed out the size that the get_size() function returns using the print function.
Using get_height and get_width():
Syntax:
img_size = py.image.load('image_path')
print("Width : " + str(img_size .get_width()))
print("Height : " + str(img_size .get_height()))
Explanation:
Here, we first loaded the image and stored it in an img_size object. And now we used the the built-in functions get_width() and get_height() to get width and height respectively.
Adding text:
In Complete PyGame Tutorial and Projects, let’s learn how to add text which is an important thing while developing the game. Whether it is for displaying the rules of the games or informing users about the controls, the text is used. So let’s learn how to add text in our pygame window.
Syntax:
pygame.font.init()
f = pygame.font.SysFont('font_file',font_size)
t = f.render('enter_text_here', true_or_false, (text_colour))
Example:
pygame.font.init()
f = pygame.font.SysFont('didot.ttc',40)
t = f.render('Copy Assignments', True, GREEN)
Explanation:
When working with text, we will use the font module. The first line is used to initialize the font module and in the second line, we used the SysFont function of the font module and passed the name of the font and the size we want.
The third line contains the render function that takes in the actual text that we want to print and the conditional rendering status (True or False) and the color of the text. We can also pass the color in the RGB form but inside brackets.
Adding sound effects:
So far, we’ve concentrated on the game’s gameplay and visuals. Now let’s look at how we can add some audio to our game. All sound-related actions are handled by the mixer in pygame. The classes and methods in this module will be used to create background music and sound effects for various actions.
The word mixer refers to the module’s ability to blend a variety of sounds into a unified entity. We can stream individual sound files in a variety of formats, including MP3, Ogg, and Mod, using the music sub-module. Sound can also be used to store and play a single sound effect in Ogg or uncompressed WAV files. Because all playback occurs in the background, when you play as sound, the method returns as soon as the sound has finished playing.
Syntax:
pygame.mixer.init()
pygame.init()
pygame.mixer.music.load("file_name_with_extension")
pygame.mixer.music.play(loops=0)
Explanation:
In Line 1: pygame.mixer.init() allows a number of options, the defaults are usually sufficient.
2: This is the initialization of the pygame.
3: This will load the music file (please mention the file along with the extension)
4: This line will actually play the music. loops is an optional integer input that specifies how many times the music should be repeated. It is set to 0 by default. If this parameter is set to -1, the music will continue to play eternally.
Controller module:
In the complete tutorial of Complete PyGame Tutorial and Projects, “Controller module” is necessary to be explained as this module allows you to control popular controllers such as the Dualshock 4 and Xbox 360 controllers: They have two analog sticks, two triggers, two shoulder buttons, a d-pad, four side buttons, and two (or three) center buttons. Pygame follows the naming conventions of Xbox controllers (a, b, x, y for buttons), however, they all relate to the same buttons.
Some of the functions and their corresponding descriptions:
KEYS | DESCRIPTION |
pygame._sdl2.controller.init | Used to initialize the controller module |
pygame._sdl2.controller.set_eventstate | Used to set the state of the events |
pygame._sdl2.controller.get_eventstate | Used to get the state of the events |
pygame._sdl2.controller.get_count | Used to get the number of connected joysticks |
pygame._sdl2.controller.name_forindex | Used to get the name of the controller |
pygame._sdl2.controller.Controller | Used to create a new object |
pygame._sdl2.controller.get_init | Used to check if the module is initialized or not (True: If initialized) |
Drawing various shapes:
A game’s integral part is the shapes. Let’s learn how to make some of them.
Drawing a rectangle:
A rectangle can be drawn using the rect() function of the draw module.
Syntax:
pygame.draw.rect(surface, colour, rect, line_thickness)
Example:
screen= pygame.display.set_mode((600, 600))
screen.fill((255, 255, 255))
pygame.draw.rect(screen, (235, 119, 52),[100, 100, 400, 100], 4)
Output:

Explanation:
Here we have 4 parameters in the rect() function.
surface: It is the name of our screen on which our output will be displayed.
color: This will set the color of our shape.
rect: This will allow the user to enter the desired dimensions for the rectangle.
line_thickness: This helps the user to set the width of the border(line) of the shape that is being drawn.
Drawing a circle:
A circle can be drawn using the circle() function of the draw module.
Syntax:
pygame.draw.circle(surface, colour, center, radius, line_thickness)
Example:
screen= pygame.display.set_mode((600, 600))
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (235, 119, 52),[300, 300], 170, 3)
Output:
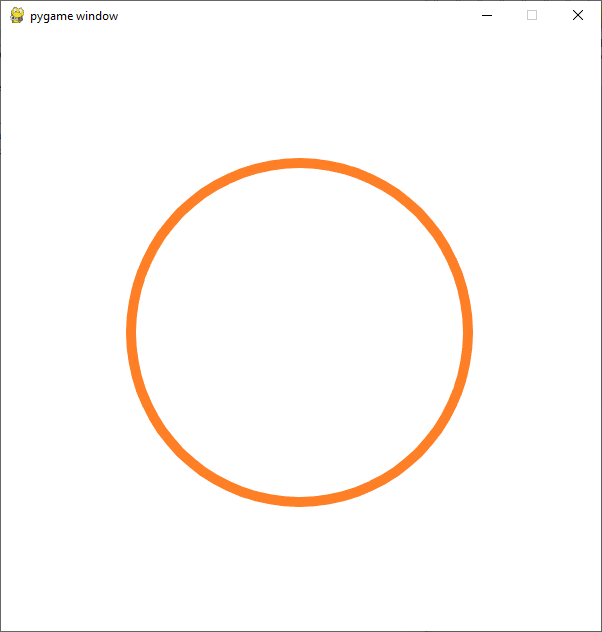
Explanation:
Here we have 5 parameters in the circle() function.
surface: It is the name of our screen on which our output will be displayed.
color: This will set the color of our shape.
center: Here a set of x and y coordinates are to be passed and that will set the position of the circle according to the screen.
radius: Here we have to specify the radius of the circle.
line_thickness: This helps the user to set the width of the border(line) of the shape that is being drawn.
Drawing a triangle:
A triangle can be drawn using the polygon() function of the draw module.
Syntax:
pygame.draw.polygon(surface, colour, points, line_thickness)
Example:
screen= pygame.display.set_mode((600, 600))
screen.fill((255, 255, 255))
pygame.draw.polygon(screen, (235, 119, 52),
[[300, 300], [100, 400],[100, 300]], 5)
Output:

Explanation:
Here we have 4 parameters in the polygon() function.
surface: It is the name of our screen on which our output will be displayed.
color: This will set the color of our shape.
points: This helps in setting the position.
line_thickness: This helps the user to set the width of the border(line) of the shape that is being drawn.
Drawing an ellipse:
An ellipse can be drawn using the ellipse() function of the draw module.
Syntax:
pygame.draw.ellipse(surface,colour,int_tuple)
Example:
screen= pygame.display.set_mode((600, 600))
screen.fill((255, 255, 255))
pygame.draw.ellipse(screen,(235, 119, 52),(165,125,100,50))
Output:
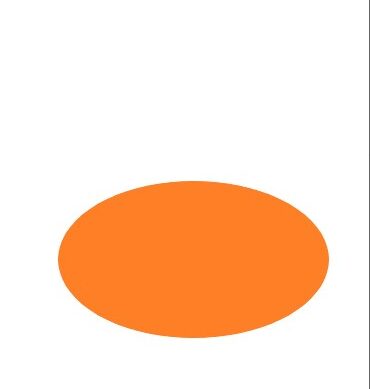
Explanation:
In the ellipse() function of the draw module, it has 3 parameters.
surface: It is the name of our screen on which our output will be displayed.
color: This will set the color of our shape.
int_tuple: This argument should be in the form of the tuple that will set a rectangle inside which ur ellipse will be formed.
In the above topics that we covered in Complete PyGame Tutorial and Projects articles, we learned the basics and important things that you need to know in order to build projects. We encourage you to self explore the documentation and learn many new functions, modules, etc and use them in your projects to make them more interactive.
Here’s the link to the documentation of the PyGame library: PyGame Documentation
Projects:
Finally starting with the projects. We have already learned the basics of pygame, important modules, and their functions. From drawing shapes to adding color to it and from adding images to working with texts, we have learned these all. Now, in this article, Complete PyGame Tutorial and Projects, let’s get started with projects and level up our experience.
Bouncing Ball:
This is the most basic implementation of the pygame with which one can start off. Here we aim to make a bouncing ball animation using pygame. We will use various functions that we learned in the above tutorial. Let’s start with the implementation of this mini-project.
Code:
import sys, pygame
pygame.init()
size = width, height = 320, 240
speed = [2, 2]
black = 0, 0, 0
screen = pygame.display.set_mode(size)
ball = pygame.image.load("bouncing_ball.gif")
b_rect = ball.get_rect()
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT: sys.exit()
b_rect = b_rect .move(speed)
if b_rect.left < 0 or b_rect.right > width:
speed[0] = -speed[0]
if b_rect.top < 0 or b_rect.bottom > height:
speed[1] = -speed[1]
screen.fill(black)
screen.blit(ball, b_rect)
pygame.display.flip()
Explanation:
In Line 1: Here in line one we imported two of python’s most famous libraries i.e sys and obviously pygame. import pygame aims at importing all the required packages along with all the modules.
2: This line will initialize all those modules that we imported in line 1.
3 to 5: We have declared some variables and assigned values to them for use in the program.
6: This line is used to get a graphical display window. Here we have used set_mode and passed a size variable that we declared inline no. 3.
The display.set mode() function creates a new Surface object that represents the graphics that are actually displayed. Any drawing that we will make on this surface will appear on our monitor.
Line 7: Now, we have defined a variable named ball and used the load function of the image module to load our image.
Line 8: We established a variable called ballrect after loading the ball picture. Rect is a useful utility object type included with Pygame that represents a rectangular region.
Line 9: This is the infinite while loop, having always true condition.
Line 10 to 11: In this line, we are checking if the quit event has happened or not. If yes, then the program flow will end.
12: Moves the ball with the speed that we entered in the variable named speed.
13 to 16: These 4 lines help to bring back the ball to the position just in case the ball is out of sight which means not on the screen.
17: This line fills the screen with black color. To do this we have made use of the fill function from the screen module.
Line 18: On this line, we draw the image of the ball onto the screen. The surface. blit() method is responsible for drawing pictures. The term “blit” refers to the process of transferring pixel colors from one image to another. A source surface to copy from and a location to transfer the source onto the destination are passed to the blit function.
Line 19: This allows us to see whatever we’ve drawn on the screen surface. This buffering ensures that we only see fully rendered frames on the screen. Without it, the user would see the screen’s half-completed sections as they were being built.
Avoid other Colors:
Now, in this project, we are going to make a bit advanced level game and make use of advanced pygame modules. We’re going to make a simple game with the following guidelines:-
(1) Only vertical movement is possible for the player.
(2) There will be two extra blocks in addition to the player block.
(3) One will be an enemy block, while the other will be a scoring block.
If the player collides with an enemy block, the game ends; if the player collides with a scoring block, the score is increased, and collecting all score blocks is required. We’ll use and implement a variety of strategies, including functions, random variables, and several pygame routines, among others.
import pygame
import sys
import random
Explanation:
In the above three lines, we have imported all the required libraries. pygame for game development, sys for manipulating the environment, and random module to add a bit of mathematics to the game and some functionalities.
pygame.init()
res =(720, 720)
Explanation:
Here we have initialized pygame and in another line, we have defined a variable that stores the screen resolution size.
c1 = random.randint(125, 255)
c2 = random.randint(0, 255)
c3 = random.randint(0, 255)
Explanation:
Here, we have declared 3 variables. We have used these variables in order to store the values that we will get from the randint function of the random library. In the randint function, we have passed two parameters i.e the starting and the ending range.
screen = pygame.display.set_mode(res)
clock = pygame.time.Clock()
Explanation:
To set the size of the display we have used set_mode and passed the argument res (the variable that we declared earlier)
clock stores the object that helps to keep the track of time. We have used the Clock() function of the time module.
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
color_list = [red, green, blue]
colox_c1 = 0
colox_c2 = 0
colox_c3 = 254
colox_c4 = 254
Explanation:
Basic variables declaration for use in the code.
player_c = random.choice(color_list)
Explanation:
This line will pick up the random element from the color_list that contains the list of colors.
startd = (100, 100, 100)
white = (255, 255, 255)
start = (255, 255, 255)
width = screen.get_width()
height = screen.get_height()
Explanation:
Here we have declared the variables that store the RGB color codes. get_width() is used to get the width of the screen and the value will be stored in the width variable the same goes for get_height() which returns the height of the screen.
You read till here which means you are enjoying and learning from our “Complete PyGame Tutorial and Projects” article, that’s great.
lead_x = 40
lead_y = height / 2
x = 300
y = 290
width1 = 100
height1 = 40
enemy_size = 50
Explanation:
These different variable declarations are used to set the initial position of the block. The position is set in the form of X and Y coordinates.
smallfont = pygame.font.SysFont('Corbel', 35)
Explanation:
Font declaration is done using the SysFont of font module. It takes two parameters as mentioned in the above tutorials i.e the name of the font and the other parameter is the size of the font.
text = smallfont.render('Start', True, white)
text1 = smallfont.render('Options', True, white)
exit1 = smallfont.render('Exit', True, white)
Explanation:
The font that we want to display on our screen is done using the render() function. It takes three parameters. The first one is the desired text, the second is the text conditionals (True or False) and the last one is the color of the text that we are rendering on the screen.
colox = smallfont.render('Colox', True, (c3, c2, c1))
x1 = random.randint(width / 2, width)
y1 = random.randint(100, height / 2)
x2 = 40
y2 = 40
speed = 15
Explanation:
Some variable declaration and setting the title of the game using render.
count = 0
rgb = random.choice(color_list)
Explanation:
This count variable is initially set to zero and will keep the score count of the player.
e_p = [width, random.randint(50, height - 50)]
e1_p = [random.randint(width, width + 100), random.randint(50, height
- 100)]
Explanation:
To set the position of the enemy upcoming blocks e_p and e1_p are declared. These two variables simply contain the values in the form of a list. The values in the list are set using the randint()
def game_over():
while True:
for ev in pygame.event.get():
if ev.type == pygame.QUIT:
pygame.quit()
if ev.type == pygame.MOUSEBUTTONDOWN:
if 100 < mouse1[0] < 140 and height - 100 < mouse1[1] \
< height - 80:
pygame.quit()
if ev.type == pygame.MOUSEBUTTONDOWN:
if width - 180 < mouse1[0] < width - 100 and height \
- 100 < mouse1[1] < height - 80:
game(lead_x, lead_y, speed, count)
screen.fill((65, 25, 64))
smallfont = pygame.font.SysFont('Corbel', 60)
smallfont1 = pygame.font.SysFont('Corbel', 25)
game_over = smallfont.render('GAME OVER', True, white)
game_exit = smallfont1.render('exit', True, white)
restart = smallfont1.render('restart', True, white)
mouse1 = pygame.mouse.get_pos()
if 100 < mouse1[0] < 140 and height - 100 < mouse1[1] < height - 80:
pygame.draw.rect(screen, startl, [100, height - 100, 40,20])
else:
pygame.draw.rect(screen, startd, [100, height - 100, 40,20])
if width - 180 < mouse1[0] < width - 100 and height - 100 < mouse1[1] < height - 80:
pygame.draw.rect(screen, startl, [width - 180, height- 100, 80, 20])
else:
pygame.draw.rect(screen, startd, [width - 180, height- 100, 80, 20])
screen.blit(game_exit, (100, height - 100))
screen.blit(restart, (width - 180, height - 100))
screen.blit(game_over, (width / 2 - 150, 295))
pygame.display.update()
pygame.draw.rect(screen, startd, [100, height - 100, 40, 20])
pygame.draw.rect(screen, startd, [width - 180, height - 100, 40, 50])
Complete Code:
This is the function for game over functionality.
First, we used a while loop and inside that, we used if-else conditionals that check for the events.
Then the code for screen-filling comes into execution.
Exit functionality is added inside this function and its execution is based on if-else conditionals and the same goes for restart functionality.
screen_blit() function takes in two arguments and is responsible for the merging of the blocks.
To update, the frames of the game pygame.display.update() are used. This can be done using the update() function of the display module.
def game(
lead_y,
lead_X,
speed,
count,
):
while True:
for ev in pygame.event.get():
if ev.type == pygame.QUIT:
pygame.quit()
keys = pygame.key.get_pressed()
if keys[pygame.K_UP]:
lead_y -= 10
if keys[pygame.K_DOWN]:
lead_y += 10
screen.fill((65, 25, 64))
clock.tick(speed)
rect = pygame.draw.rect(screen, player_c, [lead_x, lead_y, 40,40])
pygame.draw.rect(screen, (c1, c2, c3), [0, 0, width, 40])
pygame.draw.rect(screen, (c3, c2, c1), [0, 680, width, 40])
pygame.draw.rect(screen, startd, [width - 100, 0, 100, 40])
smallfont = pygame.font.SysFont('Corbel', 35)
exit2 = smallfont.render('Exit', True, white)
mouse = pygame.mouse.get_pos()
if width - 100 < mouse[0] < width and 0 < mouse[1] < 40:
pygame.draw.rect(screen, startl, [width - 100, 0, 100, 40])
else:
pygame.draw.rect(screen, startd, [width - 100, 0, 100, 40])
if width - 100 < mouse[0] < width and 0 < mouse[1] < 40:
if ev.type == pygame.MOUSEBUTTONDOWN:
pygame.quit()
if e_p[0] > 0 and e_p[0] <= width:
e_p[0] -= 10
else:
if e_p[1] <= 40 or e_p[1] >= height - 40:
e_p[1] = height / 2
if e1_p[1] <= 40 or e1_p[1] >= height - 40:
e1_p[1] = random.randint(40, height - 40)
e_p[1] = random.randint(enemy_size, height - enemy_size)
e_p[0] = width
if lead_x <= e_p[0] <= lead_x + 40 and lead_y >= e_p[1] >= lead_y - 40:
game_over()
if lead_y <= e_p[1] + enemy_size <= lead_y + 40 and lead_x <= e_p[0] <= lead_x + 40:
game_over()
pygame.draw.rect(screen, red, [e_p[0], e_p[1], enemy_size,enemy_size])
if e1_p[0] > 0 and e1_p[0] <= width + 100:
e1_p[0] -= 10
else:
if e1_p[1] <= 40 or e1_p[1] >= height - 40:
e1_p[1] = height / 2
e1_p[1] = random.randint(enemy_size, height - 40)
e1_p[0] = width + 100
if lead_x <= e1_p[0] <= lead_x + 40 and lead_y >= e1_p[1] >= lead_y - 40:
e1_p[0] = width + 100
e1_p[1] = random.randint(40, height - 40)
count += 1
speed += 1
if lead_y <= e1_p[1] + enemy_size <= lead_y + 40 and lead_x <= e1_p[0] <= lead_x + 40:
e1_p[0] = width + 100
e1_p[1] = random.randint(40, height - 40)
count += 1
speed += 1
if count >= 45:
speed = 60
if lead_y <= 38 or lead_y >= height - 38:
game_over()
if e1_p[0] <= 0:
game_over()
pygame.draw.rect(screen, blue, [e1_p[0], e1_p[1], enemy_size,
enemy_size])
score1 = smallfont.render('Score:', True, white)
screen.blit(score1, (width - 120, height - 40))
screen.blit(exit2, (width - 80, 0))
pygame.display.update()
Explanation:
This function is for the main body of the game.
At the start of this function, we have used a while loop and inside it, the conditionals are used to keep the track of the keys that the players press.
The conditionals are set in such a way that if the UP arrow key is pressed then player Y position is decremented by 10 units.
And if the player presses the DOWN arrow key then the player Y position is incremented by 10 units.
The enemy position is determined using the if-else block. If the enemy block’s X coordinate is between 0 and the width of the screen the X value gets decremented by 10.
Collision detection and checking if the player’s block has collided with the enemy block is done with the help of the game_over function.
count variable increases if the blue box is hit.
In order to add the functionality of increasing the speed as the score increases are tracked using the speed variable.
def intro(
colox_c1,
colox_c2,
colox,
exit1,
text1,
text,
):
intro = True
while intro:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
screen.fill((65, 25, 64))
mouse = pygame.mouse.get_pos()
if x < mouse[0] < x + width1 and y < mouse[1] < y + height1:
pygame.draw.rect(screen, startl, [x, y, width1, height1])
else:
if x < mouse[0] < x + width1 + 40 and y + 70 < mouse[1] < y \
+ 70 + height1:
pygame.draw.rect(screen, startl, [x, y + 70, width1+40,height1])
else:
if x < mouse[0] < width1 + x and y + 140 < mouse[1] < y + 140 + height1:
pygame.draw.rect(screen, startl, [x, y + 140,width1,height1])
else:
pygame.draw.rect(screen, startd, [x, y, width1,height1])
pygame.draw.rect(screen, startd, [x, y + 70, width1
+ 40, height1])
pygame.draw.rect(screen, startd, [x, y + 140,width1, height1])
if event.type == pygame.MOUSEBUTTONDOWN:
if x < mouse[0] < x + width1 and y < mouse[1] < y + height1:
game(lead_y, lead_x, speed, count)
if event.type == pygame.MOUSEBUTTONDOWN:
if x < mouse[0] < width1 + x and y + 140 < mouse[1] < y + 140 + height1:
pygame.quit()
if 0 <= colox_c1 <= 254 or 0 <= colox_c2 <= 254:
colox_c1 += 1
colox_c2 += 1
if colox_c1 >= 254 or colox_c2 >= 254:
colox_c1 = c3
colox_c2 = c3
pygame.draw.rect(screen, (c2, colox_c1, colox_c2), [0, 0, 40,
height])
pygame.draw.rect(screen, (c2, colox_c1, colox_c2), [width - 40,
0, 40, height])
smallfont = pygame.font.SysFont('Corbel', 35)
text = smallfont.render('Start', True, white)
text1 = smallfont.render('Options', True, white)
exit1 = smallfont.render('Exit', True, white)
colox = smallfont.render('Colox', True, (c1, colox_c1,
colox_c2))
screen.blit(colox, (312, 50))
screen.blit(text, (312, 295))
screen.blit(text1, (312, 365))
screen.blit(exit1, (312, 435))
screen.blit(sig, (320, height - 50))
clock.tick(60)
pygame.display.update()
Explanation:
The intro() function is used to actually initialize the game.
The basic button hovering effects are implemented in this function.
The start button function is added using the “MOUSEBUTTONDOWN” event. This is checked using the if-else block and if the event took place then the game starts.
intro(
colox_c1,
colox_c2,
colox,
exit1,
text1,
text,
)
Explanation:
We have call the intro() function by passing multiple parameters like:
colox_c1, colox_c1, colox_c2, colox, exit1, text1, text.
EndNote:
We hope that this article on Complete PyGame Tutorial and Projects was useful to you and you gained enough knowledge on PyGame and its application. At violet-cat-415996.hostingersite.com, we firmly believe that coding is not without application and so any article that we present is our effort to bring out the importance of doing projects in a programmer’s mind.