
This blog will discuss event listeners in JavaScript. An event is a small interaction of the user with the website on any HTML element. We can program to run a JavaScript code when the user conducts a specific kind of event on the website. We use the onclick attribute conventionally.
In JavaScript, we use the method addEventListener() to attach an event handler to the desired HTML Element.
What are HTML Events?
- Mouse Clicks
- Input Field Changes
- Form Submission
- Web Page / Media Load
Example:
<html>
<body>
<p onclick="this.innerHTML = 'You clicked Me'">Click Me</p>
</body>
</html>
When you run this HTML code and click on the element, the HTML content of the element will be changed as programmed above.
Some Special Events in JavaScript and HTML
- onchange
- onmouseover
- onmouseout
- onmousedown
- onmouseup
- onclick
Event Listeners in JavaScript – addEventListener():
In JavaScript, we use the method addEventListener() to attach an event handler to the desired HTML Element. You can add more than one event handler to the element. You can also add more than one event handler to the same element. For example, we can more than one click event to the same DOM element.
It is a method that lets the programmer attach as many event handlers to an element without losing current event handlers. You can also add event listeners to the windows object hence it is not limited only to HTML elements.
Syntax:
element.addEventListener(htmlEvent, javascriptFunction);
Adding an Event Handler in JavaScript to an Element:
Example: One Event Handler to One Element
h1.addEventListener("click", function(){ alert("You clicked Me"); });
Or, you could reference the function outside.
h1.addEventListener("click", callMeFunction() });
function callMeFunction() {
alert("Clicked on Me");
}
Example: Many Event Handlers to Same Element
h1.addEventListener("click", callMeFunction);
h1.addEventListener("click", callMeTwiceFunction);
Add Event Handler to DOM Elements (Not only HTML Elements):
As we have discussed, we can add event listeners to not only HTML Elements but all of the DOM elements and even the window object. You can even add an event listener to the XMLHttpRequest object.
Example:
window.addEventListener("resize", function(){
document.getElementById("paragraph").innerHTML = "This is the replaced text";
});
Event Bubbling:
Suppose an event happens on an element. Now, the handlers will work on that specific element after that it will work on the preceding element and then the preceding of that element.
For example, let’s take a nested set of elements of div > p > h1 with an event handler on all of them.
<div onclick="alert('divs')">
<p onclick="alert('paragraph')">
<h1 onclick="console.log('headAlert')">This is the heading</h1>
</p>
</div>
Event Capturing:
Capturing is a feature of JavaScript that is fun to play around with but rarely has really less real-world applications. In Event Capturing, the outermost element of a nested elements group is handled first opposite to that of event bubbling. In the above given example, the order for handling the elements will be div > p > h1.
Stay tuned for a detailed article about DOM Nodes in the future.
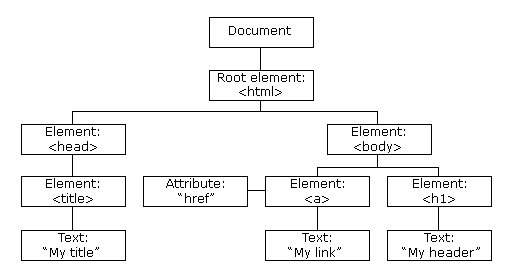
We will bed discussing more topics on JavaScript in the coming days. There are existing articles too. Please check the out: https://copyassignment.com/?s=javascript and MDN Docs Link
KEEP Learning, and KEEP Coding.