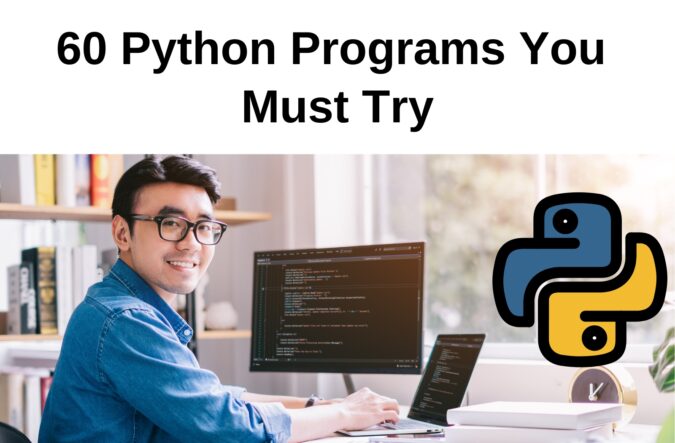
Python is a popular, widely-used programming language and easy to learn for beginners because of its short syntax and readability, and learning to code in python creates a lot of opportunities in today’s time. Practicing Programs in Python can help you become more familiar with the language and its syntax, which will make it easier for you to write and understand code in the future.
60 fundamental Programs in Python
In this article, we will see 60 fundamental Programs in Python i.e. Python programming examples that you must learn and solve as they will help you improve your problem-solving and critical thinking skills, and give you an idea of how you must break down complex problems into smaller, more manageable pieces and then find a solution for each piece.
1. Python program to find the sum of elements
A list in python is like an array in any other programming language. We will write a Python program to find the sum of all elements of a list.
def arraySum(arr):
sum = 0
for i in arr:
sum += i
return sum
print(arraySum([1, 2, 3, 4, 5]))
Output:
15
2. Python program to check whether a number is even or odd
Even numbers are divisible by 2 and odd numbers are not divisible by 2. We will use a modulo operator to check if a number is completely divisible by 2 or not.
def is_even(n):
if n%2==0:
return True
else:
return False
print(is_even(3))
Output:
False, True
3. Python program to find the average of all elements
Take a list and print the average of all the elements in it. The average of numbers is calculated by adding all the numbers and then dividing by the count of those numbers.
def average(arr):
sum = 0
for i in arr:
sum += i
return sum / len(arr)
print(average([1, 2, 3, 4, 5]))
Output:
3.0
4. Python program to count the number of Vowels in a String
Vowels are “a, e, i, o, u”. We will write a python program to check the number of vowels present in a string.
def countVowels(str):
vowels = ['a', 'e', 'i', 'o', 'u']
count = 0
for i in str:
if i in vowels:
count += 1
return count
print('number of vowels in hello is '+str(countVowels('hello')))
Output:
number of vowels in hello is 2
5. Python Program to check if a string is a palindrome
A palindrome is a word that reads the same forward and backward. For example – “Level”, “Nun”
my_str = 'malayalam'
print(my_str==my_str[::-1])
Output:
True
6. Python Program to reverse a list
We will write a program to reverse the order of a list in python using the two-pointer approach.
def reverse_list(arr):
left = 0
right = len(arr)-1
while (left < right):
temp = arr[left]
arr[left] = arr[right]
arr[right] = temp
left += 1
right -= 1
return arr
arr = [1, 2, 3, 4, 5, 6, 7]
print(reverse_list(arr))
# another and short approach
print(arr[::-1])
Output:
[7, 6, 5, 4, 3, 2, 1]
7. Python program to find the factorial of a number
The factorial of a number is the product of all the positive integers from 1 up to that number. For example, the factorial of 5 is 5! = 5*4*3*2*1 = 120
def factorial(num):
if num == 1:
return 1
else:
return num * factorial(num - 1)
print(factorial(5))
Output:
120
8. Python program to concatenate all the words in a list
arr = ['hello', 'world']
st = ''
for i in arr:
st += i
print(st)
Output:
helloworld
9. Python program to remove all the vowels in a string
s = 'hello'
vowels = ['a', 'e', 'i', 'o', 'u']
newStr = ''
for i in s:
if i not in vowels:
newStr += i
print(newStr)
Output:
hll
10. Armstrong Number Program In Python
An Armstrong number is a number that is equal to the sum of its own digits raised to the power of the number of digits. For example, 371 is an Armstrong number because 3^3 + 7^3 + 1^3 = 371.
n = 371
num_digits = len(str(n))
sum = 0
for digit in str(n):
sum += int(digit)**num_digits
print(sum == n)
Output:
True
11. Python Program to remove duplicates from a list
A set is a data structure that only contains unique elements. We will use a set in python to remove all the duplicate items.
my_list = [1, 2, 3, 2, 1]
new_list = list(set(my_list))
print(new_list)
Output:
[1, 2, 3]
12. Fibonacci Series program
A Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding numbers. For example, the first few numbers in the Fibonacci sequence are 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, etc.
n = 10
a, b = 0, 1
print(a)
print(b)
for i in range(2, n):
c = a + b
print(c)
a, b = b, c
Output:
0 1 1 2 3 5 8 13 21 34
13. Remove all odd elements in a list
arr = [1, 2, 3, 4, 5]
newArr = []
for i in arr:
if i % 2 == 0:
newArr.append(i)
print(newArr)
Output:
[2, 4]
14. Leap Year Program in Python
A leap year is a year that has an extra day in it (February 29th). Leap year has 366 days.
year = 2000
if year % 400 == 0:
print('leap year')
elif year % 4 == 0:
print('leap year')
elif year % 100 == 0:
print('not a leap year')
else:
print('not a leap year')
Output:
leap year
15. Prime Number Program in Python
A prime number is a natural number greater than 1 that is not a product of two smaller natural numbers. The first few prime numbers are 2, 3, 5, 7, and 11.
n = 7
is_prime = True
if n < 2:
is_prime = False
else:
for i in range(2, n):
if n % i == 0:
is_prime = False
break
if is_prime:
print("Prime")
else:
print("Not prime")
Output:
Prime
16. LCM program in python
The least common multiple (LCM) of two or more numbers is the smallest number which is a multiple of all of the numbers.
a = 6
b = 9
lcm = a
while lcm % b != 0:
lcm += a
print(f"LCM of {a} and {b} is {lcm}.")
Output:
LCM of 6 and 9 is 18.
17. HCF program in python
The highest common factor (HCF) of two or more numbers is the largest number that divides all of the numbers exactly. For example, the HCF of 15 and 20 is 5, because 5 is the largest number that divides both 15 and 20 exactly.
a = 15
b = 20
if a > b:
smaller = b
else:
smaller = a
for i in range(1, smaller+1):
if (a % i == 0) and (b % i == 0):
hcf = i
print(f"HCF of {a} and {b} is {hcf}")
Output:
HCF of 15 and 20 is 5
18. Python program to swap two numbers
first = 27
second = 4
temp = first
first = second
second = temp
print(f"After swapping, the first number is {first} and the second number is {second}")
Output:
After swapping, the first number is 4 and the second number is 27.
19. Python program to print all prime numbers up to n
n = 10
for i in range(2, n+1):
is_prime = True
for j in range(2, i):
if i % j == 0:
is_prime = False
break
if is_prime:
print(i)
Output:
2 3 5 7
20. Python program to find the greatest of three numbers
num1 = 10
num2 = 14
num3 = 12
if (num1 >= num2) and (num1 >= num3):
largest = num1
elif (num2 >= num1) and (num2 >= num3):
largest = num2
else:
largest = num3
print(largest)
Output:
14
21. Multiplication table program
num = 19
for i in range(1, 11):
print(num*i)
Output:
19 38 57 76 95 114 133 152 171 190
22. Python program to sort an array(list)
numbers = [2, 3, 4, 1]
numbers.sort()
print(numbers)
Output:
[1, 2, 3, 4]
23. Python program to convert all characters to uppercase
s = 'follow us'
newStr = ''
for i in s:
newStr += i.upper()
print(newStr)
Output:
FOLLOW US
24. Python program to capitalize the first letter of each word
s = 'copy assignment'
newStr = ''
for i in range(len(s)):
if i == 0 or s[i - 1] == ' ':
newStr += s[i].upper()
else:
newStr += s[i]
print(newStr)
Output:
Copy Assignment
25. Python program to convert all characters to lowercase
s = 'COPYASSIGNMENT'
newStr = ''
for i in s:
newStr += i.lower()
print(newStr)
Output:
copyassignment
26. Python program to reverse a string
s = 'tnemngissAypoC'
newStr = ""
for i in s:
newStr = i + newStr
print(newStr)
Output:
CopyAssignment
27. Calculator Program in Python
We will write a basic calculator program that can add, subtract, multiply and divide.
def add(a,b):
return a+b
def sub(a,b):
return a-b
def multi(a,b):
return a * b
def div(a,b):
return a / b
print(add(10,15))
print(sub(13,10))
Output:
25 3
28. Python program for Pyramid pattern
n=5 for i in range(0, n): for j in range(0, i+1): print("* ",end="") print("\r")
Output:

29. Reverse Pyramid pattern
n=5 k = 2*n - 2 for i in range(0, n): for j in range(0, k): print(end=" ") k = k - 2 for j in range(0, i+1): print("* ", end="") print("\r")
Output:
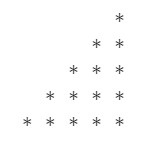
30. Continuous Character pattern program in Python
n = 5 num = 65 for i in range(0, n): for j in range(0, i+1): ch = chr(num) print(ch, end=" ") num = num + 1 print("\r")
Output:

31. Python program to print Triangle
n=5 k = n - 1 for i in range(0, n): for j in range(0, k): print(end=" ") k = k - 1 for j in range(0, i+1): print("* ", end="") print("\r")
Output:
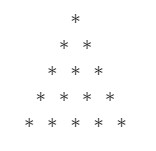
32. Reverse Triangle program
rows = 4 k = 2 * rows - 2 for i in range(rows, -1, -1): for j in range(k, 0, -1): print(end=" ") k = k + 1 for j in range(0, i + 1): print("*", end=" ") print("")
Output:

33. Python program to print a Diamond shape
To print a diamond shape, first, we will print the upper half of triangle and then the lower half triangle.
n = 4 k = 2 * n - 2 for i in range(0, n): for j in range(0, k): print(end=" ") k = k - 1 for j in range(0, i + 1): print("* ", end="") print("") k = n - 2 for i in range(n, -1, -1): for j in range(k, 0, -1): print(end=" ") k = k + 1 for j in range(0, i + 1): print("* ", end="") print("")
Output:

34. Print Hourglass Pattern program
To print the hourglass shape, first, we will print the downward triangle and then the upward triangle.
row = 5 for i in range(row, 0, -1): for j in range(row-i): print(" ", end="") for j in range(1, 2*i): print("*", end="") print() for i in range(2, row+1): for j in range(row-i): print(" ", end="") for j in range(1, 2*i): print("*", end="") print()
Output:

35. Print Butterfly Pattern program
To print the Butterfly pattern, we will follow the same approach of printing the upper half first and then the lower half.
row = 8 n = row//2 for i in range(1,n+1): for j in range(1, 2*n+1): if j>i and j< 2*n+1-i: print(" ", end="") else: print("* ", end="") print() for i in range(n,0,-1): for j in range(2*n,0,-1): if j>i and j< 2*n+1-i: print(" ", end="") else: print("* ", end="") print()
Output:
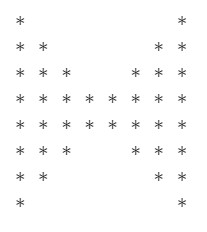
36. Print Christmas Tree Pattern using python program
To print a Christmas tree pattern, we can divide it into three parts. First is the upper triangle, second is the mid triangle and third is a vertical rectangle.
n = 5 #upper triangle for i in range(n): for j in range(n-i): print(' ', end=' ') for k in range(2*i+1): print('*',end=' ') print() #mid triangle for i in range(n): for j in range(n-i): print(' ', end=' ') for k in range(2*i+1): print('*',end=' ') print() #pole shape for i in range(n): for j in range(n-1): print(' ', end=' ') print('* * *')
Output:
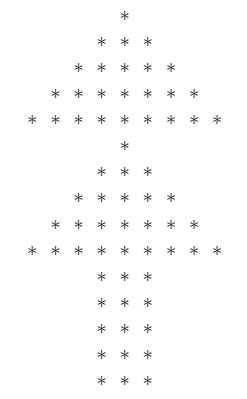
37. Python program to Reverse words in a String
string = "more for copyassignment visit" s = string.split()[::-1] l = [] for i in s: l.append(i) print(" ".join(l))
Output:
visit copyassignment for more
38. Python program to Check if Two Strings are Anagram
An anagram is a word formed by rearranging the letters of one word. For example “listen” and “silent” are anagrams since they contain the same letters but are arranged differently.
s1='listen' s2='silent' if(sorted(s1)== sorted(s2)): print("The strings are anagrams") else: print("The strings aren't anagrams")
Output:
The strings are anagrams
39. Python program to print number divisible by 3 or 5 in a given range
n = 30
for i in range(1, n):
if i % 3 == 0 or i % 5 == 0:
print(i)
Output:
3 5 6 9 10 12 15 18 20 21 24 25 27
40. Python program to sort all words alphabetically
arr = ['hello', 'world', 'its', 'copyassignment', 'website'] for i in range(len(arr)): for j in range(i + 1, len(arr)): if arr[i] > arr[j]: arr[i], arr[j] = arr[j], arr[i] print(arr)
Output:
['copyassignment', 'hello', 'its', 'website', 'world']
41. Python program for two sum problem
Given a list of numbers, we have to find two numbers whose sum is equal to the given target
def twoSum(num_arr, pair_sum): for i in range(len(num_arr) - 1): for j in range(i + 1, len(num_arr)): if num_arr[i] + num_arr[j] == pair_sum: print("Pair with sum", pair_sum,"is: ", num_arr[i],",",num_arr[j]) num_arr = [3, 5, 2, 4, 8, 1] pair_sum = 4 twoSum(num_arr, pair_sum)
Output:
Pair with sum 4 is: 3 , 1
42. Python program for Perfect number
A perfect number is a positive integer that is equal to the sum of its proper positive divisors excluding the number itself. For example, the proper positive divisors of 6 are 1, 2, and 3, which add up to 6. So 6 is a perfect number.
n = 6 sum1 = 0 for i in range(1, n): if(n % i == 0): sum1 = sum1 + i if (sum1 == n): print("Perfect number") else: print("Not a Perfect number")
Output:
Perfect number
43. Python program for Strong Number
A strong number is a number in which the sum of the factorials of its digits is equal to the number itself. For example, 145 is a strong number because 1! + 4! + 5! = 1 + 24 + 120 = 145.
sum=0 num=145 temp=num while(num): i=1 fact=1 r=num%10 while(i<=r): fact=fact*i i=i+1 sum=sum+fact num=num//10 if(sum==temp): print("Strong number") else: print("Not a Strong number")
Output:
Strong number
44. Python program to find Prime Factors of a number
The prime factors of a number are the prime numbers that divide the number completely. For example, the prime factors of 15 are 3 and 5, because 3 and 5 are prime numbers, and 3 x 5 = 15.
n=15 i=1 print("Factors:") while(i<=n): k=0 if(n%i==0): j=1 while(j<=i): if(i%j==0): k=k+1 j=j+1 if(k==2): print(i) i=i+1
Output:
Factors: 3 5
45. Program to reverse a number in Python
To reverse a number we follow these steps.
- Obtain the last digit of the number by using the modulus(%) operator.
- Store this last digit in a new variable at the one’s place.
- Remove the last digit from the current number by diving(/) it with 10.
- Repeat the above steps till the current number becomes empty.
n= 123456 reversed=0 while(n>0): dig=n%10 reversed=reversed*10+dig n=n//10 print("Reversed number is:",reversed)
Output:
Reversed number is: 654321
46. Python program to find the Sum of all digits in a number
To sum all the digits of a number we follow the same steps as reversing a number with a minor change. Instead of storing the resultant number, we keep on adding these numbers to the sum variable.
n=12345 total=0 while(n>0): lastDigit=n%10 total=total+lastDigit n=n//10 print("Total sum of digits in number:",total)
Output:
Total sum of digits in number: 15
47. Python program to check Amicable numbers or not
Two numbers are amicable numbers if the sum of the divisors of the first number is equal to the second number, and the sum of the divisors of the second number is equal to the first number. For example, 220 and 284 are Amicable numbers.
a=220 b=284 sum1=0 sum2=0 for i in range(1,a): if a%i==0: sum1+=i for j in range(1,b): if b%j==0: sum2+=j if(sum1==b and sum2==a): print('Amicable') else: print('Not Amicable')
Output:
Amicable
48. Python program to print Perfect Squares in a range
start = int(input("Enter the Lower bound")) end = int(input("Enter the upper bound")) for n in range(start, end+1): sum = 0 for i in range(1, n): if n%i == 0: sum += i if n == sum: print(n)
Output:
Enter the Lower bound 1 Enter the upper bound 50 6 28
49. Python program to print Identity Matrix
An identity matrix is a square matrix with 1s on the diagonal (from the top left to the bottom right) and 0s everywhere else.
rows=3 for i in range(0,rows): for j in range(0,rows): if(i==j): print("1",sep=" ",end=" ") else: print("0",sep=" ",end=" ") print()
Output:

50. Python Program to Swap Two Numbers without using Third Variable
This is a famous interview question that you must learn once. To swap two numbers without any third variable we follow these steps
- Add both numbers and assign it to the first number
- Subtract both numbers and assign them to the second number
- Again, subtract both numbers and assign them to the first number
We can also use division and multiplication to swap two numbers just like this.
a=4
b=10
a=a+b
b=a-b
a=a-b
print("a is",a,"and b is",b)
Output:
a is 10 and b is 4
51. Print Numbers in a Range Without using Loops
We will use a simple recursive program, to print numbers in the range of 1 to 10 without loops
def printno(upper):
if(upper>0):
printno(upper-1)
print(upper)
printno(10)
Output:
1 2 3 4 5 6 7 8 9 10
52. Python program to find Simple Interest

principle=float(input("Principle amount:")) time=int(input("Time(years):")) rate=float(input("Rate(%):")) simple_interest=(principle*time*rate)/100 print("Simple interest is:",simple_interest)
Output:

53. Python program to find Compound Interest
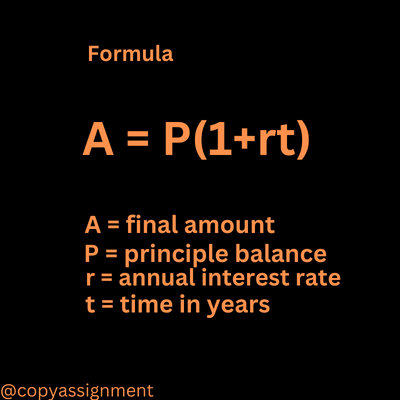
principal = float(input("Principle amount:")) time=int(input("Time(years):")) rate=float(input("Rate(%):")) Amount = principal * (pow((1 + rate / 100), time)) CI = Amount - principal print("Compound interest is", CI)
Output:

54. Python program to Convert Celsius to Fahrenheit
celsius=32
f=(celsius*1.8)+32
print("Temperature in farenheit is:",f)
Output:
Temperature in farenheit is: 89.6
55. Python program to Calculate the Grade of a Student
eng=int(input("Enter marks of English: ")) hindi=int(input("Enter marks of Hindi: ")) math=int(input("Enter marks of Math: ")) sci=int(input("Enter marks of Science: ")) sanskrit=int(input("Enter marks of588 Sanskrit: ")) avg=(eng+hindi+math+sci+sanskrit)/5 if(avg>=90): print("Grade: A") elif(avg>=80 and avg<90): print("Grade: B") elif(avg>=70 and avg<80): print("Grade: C") elif(avg>=60 and avg<70): print("Grade: D") else: print("Grade: F")
Output:
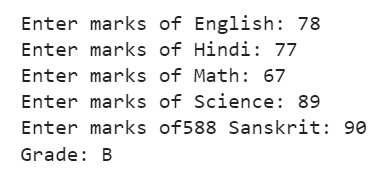
56. Find the largest element in an array(list)
list = [2, 4, 1, 16, 25, 3]
max = list[0]
for x in list:
if x > max:
max = x
print(max)
Output:
25
57. Python program to find the intersection of two lists in python
The intersection of two objects means to include only those elements that are common in both objects.
list1 = [4, 9, 1, 17, 11, 26, 28, 54, 69]
list2 = [9, 9, 74, 21, 45, 11, 63, 28, 26]
list3 = [value for value in list1 if value in list2]
print(list3)
Output:
[9, 11, 26, 28]
58. Python program to Print Pascal Triangle
n=5 a=[] for i in range(n): a.append([]) a[i].append(1) for j in range(1,i): a[i].append(a[i-1][j-1]+a[i-1][j]) if(n!=0): a[i].append(1) for i in range(n): print(" "*(n-i),end=" ",sep=" ") for j in range(0,i+1): print('{0:6}'.format(a[i][j]),end=" ",sep=" ") print()
Output:

59. Python program to print Quotient and Remainder
a=325
b=15
print("Quotient:",a//b)
print("Remainder:",a%b)
Output:
Quotient: 21 Remainder: 10
60. Python program to print the sum of first n Numbers
n = int(input("Enter a number: "))
sum = 0
while(n > 0):
sum += n
n -= 1
print("Total sum is",sum)
Output:
Enter a number: 10 Total sum is 55
Conclusion
In this article, we have covered 60 Python Programming Examples and various fundamental Programs in Python on various python topics which will help you to learn and apply these concepts to many different problems. We recommend you start solving all the questions in this article to get a good hold of problem-solving in python.
We hope you enjoyed our list of Python programs. Thank you for visiting our website.