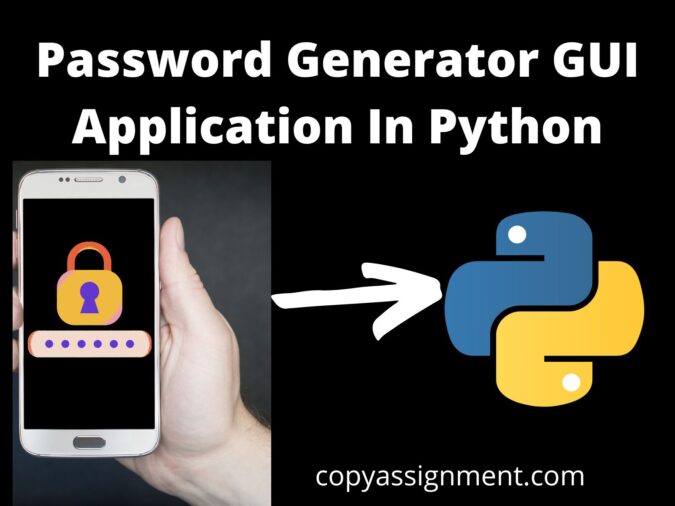
Google gives you suggestions when you create your new passwords Right? But how about creating your own password generator in python, a GUI application? Sounds amazing, isn’t it? So let’s go ahead and make another great GUI application or you can say a mini project in python. As we all know whenever we sign up for a new website we need to set our username and password. After typing our username when we take our cursor to the password section Google gives us a suggestion for a new password.
In the same way, python makes it too easy for you to create your own password generator rather than using other tools like google. Let’s have some more privacy in the case of passwords and create our own password generator in python.
Complete Code for Password Generator In Python
We are providing you the full source code of the password generator GUI application in python with an explanation at every line using comments because we are sure this will help you a lot. You are free to use this code but don’t forget to add your own styling to the code. You can add this project to your GitHub also.
In this password generator GUI application, we are going to use two 3 modules. Firstly, the Tkinter module for creating an application window. Secondly, pyperclip module for copying the generated password. Now, remember these two modules are not inbuilt modules. So you have to install them using the pip install command. The third and final module we are going to use is a random module to generate the random password finally. The quick logic for this GUI application is going to be like this: Firstly, We will import the necessary modules. Then we will create the application window. After that, we will generate a random password and copy it. Afterward, In the end, we will create some buttons to make our password generator application more interactive for the user. Because after all, it’s a GUI.
# importing the tkinter module from tkinter import * # importing the pyperclip module to use it to copy our generated # password to clipboard import pyperclip # random module will be used in generating the random password import random # initializing the tkinter root = Tk() # setting the width and height of the gui root.geometry("400x400") # x is small case here # declaring a variable of string type and this variable will be # used to store the password generated passstr = StringVar() # declaring a variable of integer type which will be used to # store the length of the password entered by the user passlen = IntVar() # setting the length of the password to zero initially passlen.set(0) # function to generate the password def generate(): # storing the keys in a list which will be used to generate # the password pass1 = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', ' ', '!', '@', '#', '$', '%', '^', '&', '*', '(', ')'] # declaring the empty string password = "" # loop to generate the random password of the length entered # by the user for x in range(passlen.get()): password = password + random.choice(pass1) # setting the password to the entry widget passstr.set(password) # function to copy the password to the clipboard def copytoclipboard(): random_password = passstr.get() pyperclip.copy(random_password) # Creating a text label widget Label(root, text="Password Generator Application", font="calibri 20 bold").pack() # Creating a text label widget Label(root, text="Enter password length").pack(pady=3) # Creating a entry widget to take password length entered by the # user Entry(root, textvariable=passlen).pack(pady=3) # button to call the generate function Button(root, text="Generate Password", command=generate).pack(pady=7) # entry widget to show the generated password Entry(root, textvariable=passstr).pack(pady=3) # button to call the copytoclipboard function Button(root, text="Copy to clipboard", command=copytoclipboard).pack() # mainloop() is an infinite loop used to run the application when # it's in ready state root.mainloop()
Output:

Understanding the Code of GUI Application
Firstly, Let’s understand the coding now with some technical terms. Here, we are going to explain the code stepwise. So that if you have some knowledge of python you will be able to make this password generator application on your own by just reading this section.
Step1: Firstly, Import necessary modules ( Tkinter, pyperclip and random)
Step2: Then, Initialize Tkinter using Tk() method
Step3: After that, Create an application window for the GUI application using the geometry method of Tkinter
Step4: Declare 2 variables. One for storing the generated password and another one for taking password length input from the user.
Step5: Then, Define the generate password method.
Step6: Define the copy-to-clipboard method.
Step7: Then, Create Button widgets and set commands as per the above-defined methods.
Step8: Finally, Run an infinite main loop to run the application.
Thanks for reading
If you found this article useful please support us by commenting “nice article” and don’t forget to share it with your friends and enemies! If you have any queries feel free to ask in the comment section.
Happy Coding!
Also read:
- See connected wifi passwords using Python
- Simple Text-To-Speech In Python
- GUI Calculator Using Python tkinter
- Creating User-defined Entry Widgets
- GUI Stone Paper Scissor Game: Python
- Displaying Images in Tkinter
- GUI Age Calculator
- Changing Screen Size: Tkinter
- Simple Music Player Using Python
- Adding three matrices
- Auto-Login with Python