Problem Statement:
You are on the top floor of a mall and you want to go out of the building. You’re car is in the parking area on the ground floor. But there’s some issue with the lift hence, it’s not working. Now you are left with stairs.
You are a programmer and you have to code the quickest route possible.
You have a floor map consisting of the 2D matrix.
Your position over the topmost floor is represented by the number 3.
– On each floor, there is at least a staircase represented by the number 1.
– All the walkable space is represented by the number 0.
– Ground floor place will have all 0’s.
Find the quickest path from your position on the top floor to the rightmost place on the ground floor.
Example:
Input1:
Enter Dimensions R and C: 4 4
Enter contents:
0 0 3 0
1 0 0 1
0 1 1 0
0 0 0 0
Output1:
The quickest path is: R1 C3->R2 C4->R3 C3->R4 C4
Input2:
Enter Dimensions R and C: 3 3
Enter contents:
3 0 0
1 1 1
0 0 0
Output2:
The quickest path is: R1 C1->R2 C1->R3 C3
Code Reaching Ground Floor in Python:
'''calculate nearest stair''' def nearest_distance(row,pos): #compare distance to left stair and distance to right stair left = -1 right = -1 for i in range(pos,-1,-1): if(row[i]==1): left=i break for i in range(pos,len(row)): if(row[i]==1): right=i break if(right==-1): return left elif(left==-1): return right elif((pos-left)<(right-pos)): return left else: return right '''find the fastest route''' def route(matrix,rows,cols): path = list() rows = len(matrix) pos = matrix[0].index(3) path.append(f'R1 C{pos+1}') num=2 nex=pos for i in range(1,rows-1): nex = nearest_distance(matrix[i],nex) path.append(f'R{num} C{nex+1}') num +=1 path.append(f'R{rows} C{cols}') return "->".join(path) '''input''' r,c = map(int,input("Enter Dimensions R and C: ").split(" ")) matrix = list() print("Enter contents: ") for i in range(r): row = list(map(int,input().split())) matrix.append(row) '''output''' print("The quickest path is: ", route(matrix,r,c))
Input and Output 1:

Input and Output 2:
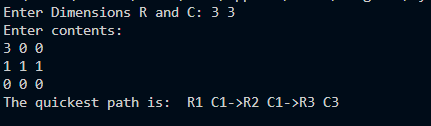
Also Read:
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Shift Numbers in Python | Assignment Expert
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python