Problem Statement:
There’s a big festival sale running on an e-commerce website. There are certain conditions for shipping charges and under the sale and there are a lot of discounts as well. Design a program that calculates the final cart values i.e. prices after the discount and shipping charges from the given prices of the cart.
Discounts are based on the following criteria:
i. For the cart rate less than 299, no discount will be applicable.
ii. For the cart rate between 299 and 699 (both inclusive), a 12% discount will be applicable.
iii. For the cart rate between 700 and 1599 (both inclusive), a 28% discount will be applicable.
iv. For the cart rate between 1600 and 2499 (both inclusive), a 32% discount will be applicable.
v. For the cart rate equal to and above 2500, a 40% discount will be applicable.
Shipping charges of Rs. 99 will be applicable if the final cart value is under 500. Above and equal to 500 will be eligible for free shipping.
Given the product prices of customers, return the final cart values including discount and shipping.
Also specify, if the customer is applicable for free shipping or not.
Example:
Input:
5
99 389 700 1700 5000
Output:
Order values of Customer 1: 198
Order values of Customer 2: 442
Order values of Customer 3: 504 (Free Shipping)
Order values of Customer 4: 1156 (Free Shipping)
Order values of Customer 5: 3000 (Free Shipping)
Code:
# function to find final cart values def isFree(cart_values,n): results = list() for i in range(n): if(cart_values[i]>=299 and cart_values[i]<=699): results.append(cart_values[i] - (12*cart_values[i])//100) elif(cart_values[i]>699 and cart_values[i]<=1599): results.append(cart_values[i] - (28*cart_values[i])//100) elif(cart_values[i]>1599 and cart_values[i]<=2499): results.append(cart_values[i] - (32*cart_values[i])//100) elif(cart_values[i]>2499): results.append(cart_values[i] - (40*cart_values[i])//100) else: results.append(cart_values[i]) return results customers = int(input("Enter the no. of customers: ")) cart_values = list(map(int,input("Enter the cart values: ").split())) results = isFree(cart_values, customers) #print the result i=1 for each in results: if(each<=499): each = each + 99 print(f"Order values of Customer {i}: {each}") else: print(f"Order values of Customer {i}: {each} (Free Shipping)") i+=1
Input:

Output:
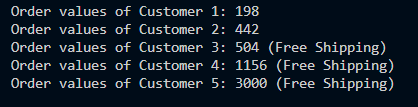
Also Read:
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Shift Numbers in Python | Assignment Expert
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python