Problem statement:
In String Rotation in Python, we are given two strings, we need to find the number of rotations required to match 1 string to another string. If there is no match, print No rotations found.
Code for String Rotation in Python:
# importing deque from collections module
from collections import deque
# declaring 2 string
mystr1 = 'copyassignment'
mystr2 = 'assignmentcopy'
# declaring an empty list
allrotations = []
# finding all rotations of mystr1 and storing them to allrotations
for i in mystr1:
items = deque(mystr1)
items.rotate(1)
mystr1 = list(items)
mystr1 = ''.join(mystr1)
allrotations.append(mystr1)
found = False
# checking the mystr2 in allrotations and printing number of rotations required
for i in range(len(allrotations)):
if mystr2==allrotations[i]:
found = True
print(i+1)
else:
if not found:
print("No rotations found")
Output:
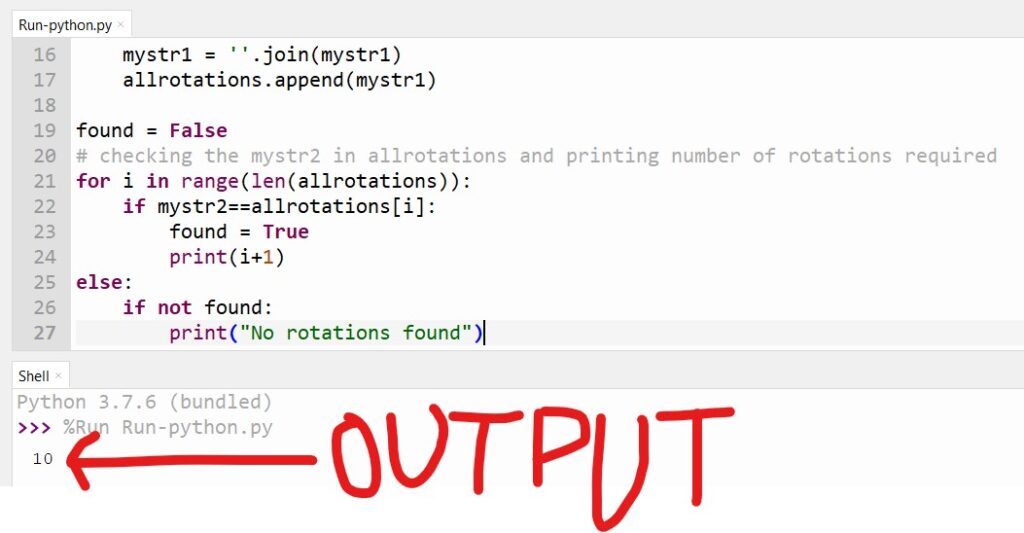
Explanation:
We will understand the logic behind the code, not the code. So, we have used deque from the collections library to find rotations of a string. Then, we have generated all rotations inside a for loop and stored them in allrotations list. Then, have compared all the rotations with the second string and found the index number of the rotated string which matches and prints its index number. The index number is equal to the number of rotations required to rotate the first string to make it equal to the second string.
Also Read:
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Shift Numbers in Python | Assignment Expert
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python