This blog will discuss the principle of slicing in Python. Python has become one of the most popular languages in 2022 with the most job opportunities because of its versatility. There are many python core principles in order to be ready for a python development job.
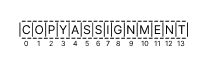
We can use the index of strings in Python to access them. The index will always start a 0. For example, to access the “A” in the following string, we will use the code:
string = "COPYASSIGNMENT"
print(string[5]) #displays "S"
We can also do slicing in Python. While slicing a string, Python will take the string as an array of characters. Note that we should add 1 to the stop index to get the intended result. The syntax is:
array = [1, 2, 3]
string[start:stop]
start — the starting index of the slice, which defaults to 0, or the initial index, and includes the element at that position unless it is the same as stop. Negative values indicate starting n items from the end.
stop — The length of the sequence being sliced, that is, up to and including the end, defaults to the ending index of the slice, which does not include the element at this index.
In the above example, to access the string from “C” to “A”, we can use the following code.
string = "COPYASSIGNMENT"
print(string[0:5]) #displays "COPYA"
#we used 5 because it will only display upto index 4.
We can also access the indexes by skipping the middle ones. For example, if we want to access the elements of odd indices, we can use the third parameter in the slicing.
The syntax will be:
print(string[start:stop:step])
step — the index’s default increment factor is 1, which is 1. If the result is negative, you slice through the iterable backward.
For example,
string = "COPYASSIGNMENT"
print(string[::2]) #displays "CPASGMN"
Examples of String Slicing in Python
1. Starting from the initial
array = [1, 2, 3, 4, 5]
print(array[0:3]) #displays [1, 2, 3]
string = "ANKUR"
print(string[:4]) #displays "ANKU"
2. Starting from the middle and ending in the middle
my_arr = [2, 4, 6, 8, 10]
print(my_arr[1:3]) #displays [4, 6]
my_str = "COPYASSIGNMENT"
print(my_str[3:7]) #displays "YASS"
3. Starting from the middle until the end
mero = [1, 4, 7, 10, 13]
print(mero[2:]) #dislays [7, 10, 13]
sample_str = "PYTHON"
print(sample_str[1:]) #displays "YTHON"
4. Step in the middle
mero = [1, 4, 7, 10, 13]
print(mero[::3]) #dislays [1, 10]
sample_str = "PYTHON"
print(sample_str[1::2]) #displays "YHN"
5. Remove the elements in the middle
arr = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
arr[3:8] = [] #[8, 10, 12, 14, 16] ==> []
print(arr) #displays [2, 4, 6, 18, 20]
Official documentation of Slicing: Click here.
Learn other core principles of Python: https://copyassignment.com/python/
KEEP LEARNING, KEEP CODING