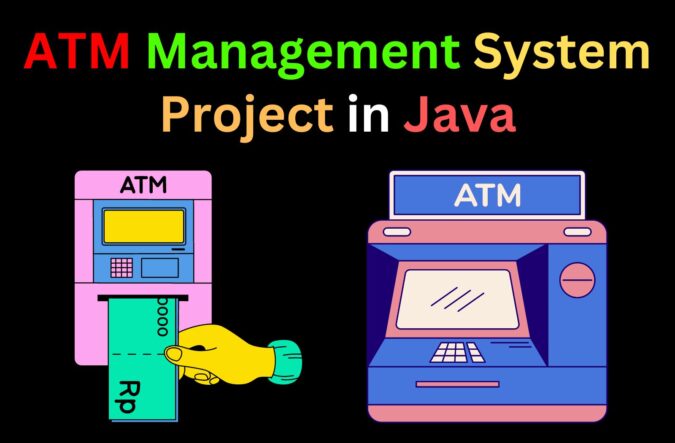
We will discuss the project on ATM Management System Project in Java and MySql. The article is best for projects on databases and java. There are two users: Admin and Users. Admin can add the users and users can do operations like deposit, withdrawal, pin change, and statement checking.
ATM Management System Project In Java: Project Overview
Project Name: | ATM Management System Project in Java |
Abstract: | It’s a GUI-based project used with the Swing module to manage ATM transactions of a bank. |
Language/s Used: | Java |
IDE: | Eclipse IDE for Java Developers |
Java version (Recommended): | Java SE 18.0. 2.1 |
Database: | MySQL |
Type: | Desktop Application |
Recommended for: | Final Year Students |
Setting Up the environment
Make sure JDK is present in your system. It can be installed using the IDE you are using.
The first step is to create a project in the name you wish. Create a package named “atm“. We are creating java classes in this package.
To connect the project to the database, follow the steps:
- Make sure MySQL is installed on your system.
- Download the MySQL connector from here.
- Open the properties of the project from the dropdown list which appears on right-click on the project. Navigate to the libraries tab and click on the classpath. Select “Add External JARs…”. Select the file you downloaded. This step will differ on IDE you are using.
MySQL Setup for Attendance Management System Project In Java
1. Creating the database
--CREATING THE DATABASE
CREATE DATABASE atm;
2. Selecting the database
--SELECTING THE DATABASE
USE atm;
3. Create a users table
--CREATE USERS TABLE
CREATE TABLE users(id int primary key auto_increment, card varchar(16), pin varchar(4), uname varchar(25), bal int);
4. Create a transaction table
--CREATE TRANSACTION TABLE
CREATE TABLE transactions(transid int primary key auto_increment, id int, amount int, stat varchar(3), bal int);
5. Create an admin user
--CREATING AN ADMIN USER
INSERT INTO users VALUES(1, "admin", "1234", "admin", 0);
Coding ATM Management System Project In Java
1. Main Module
File Name: “Main.java“
This module contains the main function. ie, the execution of the program begins here. This calls the login Module.
package atm; import java.sql.SQLException; public class Main { public static void main(String[] args) throws InterruptedException, SQLException { Login login = new Login(); login.loginView(); } }
2. Login Module
File Name: “Login.java“
This module creates a page where we have to enter the ATM card numbers manually. We can also enter to admin page from this page.
package atm; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextField; public class Login extends Commons{ public void loginView() { Commons common = new Commons(); JFrame frame = (JFrame)common.Frame(); Font txt = new Font("", Font.BOLD, 15); Pin pin = new Pin(); //---------------CARDNUMBER---------------- JLabel card = new JLabel("ENTER YOUR CARD NUMBER"); card.setBounds(50, 270, 250, 20); card.setFont(txt); JTextField cardNumber = new JTextField(); cardNumber.setBounds(50, 300, 500, 35); cardNumber.setFont(txt); frame.add(cardNumber); frame.add(card); //----------------------------------------- //----------------ADMIN-------------------- JLabel admin = new JLabel("ADMIN LOGIN >"); admin.setBounds(0, 500, 570, 30); admin.setHorizontalAlignment(JLabel.RIGHT); admin.setFont(txt); frame.add(admin); admin.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { pin.pinView("admin"); frame.dispose(); } }); //------------------------------------------ //-----------------BUTTON----------------- JButton cont = new JButton("COUNTINUE"); cont.setBounds(200, 400, 200, 50); cont.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(cont); cont.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(cardNumber.getText().length() == 16) { pin.pinView(cardNumber.getText()); frame.dispose(); } else { Fail fail = new Fail(); fail.failView("WRONG CARD NUMBER!!!"); frame.dispose(); } } }); //---------------------------------------- frame.setVisible(true); } }

Enter the card number in the text field and continue to enter the pin. Admin login in the bottom right helps you to log in as admin.
3. Pin Checking Module
File Name: “Pin.java“
This module checks the pin of the user is correct or not. Shows us the transaction fail page in case of the wrong password.
package atm; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.ResultSet; import java.sql.SQLException; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPasswordField; public class Pin { public void pinView(String cardNum) { Commons common = new Commons(); JFrame frame = (JFrame)common.Frame(); Font txt = new Font("", Font.BOLD, 15); Home home = new Home(); Admin admin = new Admin(); //---------------PASSWORD---------------- JLabel pswd = new JLabel("ENTER YOUR PIN"); pswd.setBounds(50, 270, 250, 20); pswd.setFont(txt); JPasswordField pswdField = new JPasswordField(); pswdField.setBounds(50, 300, 500, 35); pswdField.setFont(txt); frame.add(pswdField); frame.add(pswd); //----------------------------------------- //-----------------BUTTON----------------- JButton cont = new JButton("COUNTINUE"); cont.setBounds(200, 400, 200, 50); cont.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(cont); cont.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { try { SQLManage man = new SQLManage(); ResultSet rst = man.check(cardNum, pswdField.getText()); if(rst.next()) { if(rst.getString("card").equals("admin")) { admin.adminView(); frame.dispose(); } else { home.homeView(rst.getInt("id")); frame.dispose(); } } else { Fail fail = new Fail(); fail.failView("WRONG PIN!!!"); frame.dispose(); } } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------- frame.setVisible(true); } }

Type the pin to your card, for admin enter “1234” as the pin. It will take you to your respective pages.
4. Admin home page
File Name: “Admin.java“
This is the module for the admin home page. Here we have two buttons. One to add users and the other to quit.
package atm; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.SQLException; import javax.swing.JButton; import javax.swing.JFrame; public class Admin { public void adminView() { Commons commons = new Commons(); JFrame frame = (JFrame) commons.Frame(); //-------------ADDUSERS--------------------- JButton add = new JButton("ADD USERS"); add.setBounds(150, 250, 300, 100); add.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(add); add.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { AddUser user = new AddUser(); try { user.addView(); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); //------------------------------------------ //--------------EXIT--------------------------- JButton exit = new JButton("EXIT"); exit.setBounds(150, 400, 300, 100); exit.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(exit); exit.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.exit(0); } }); //--------------------------------------------- frame.setVisible(true); } }
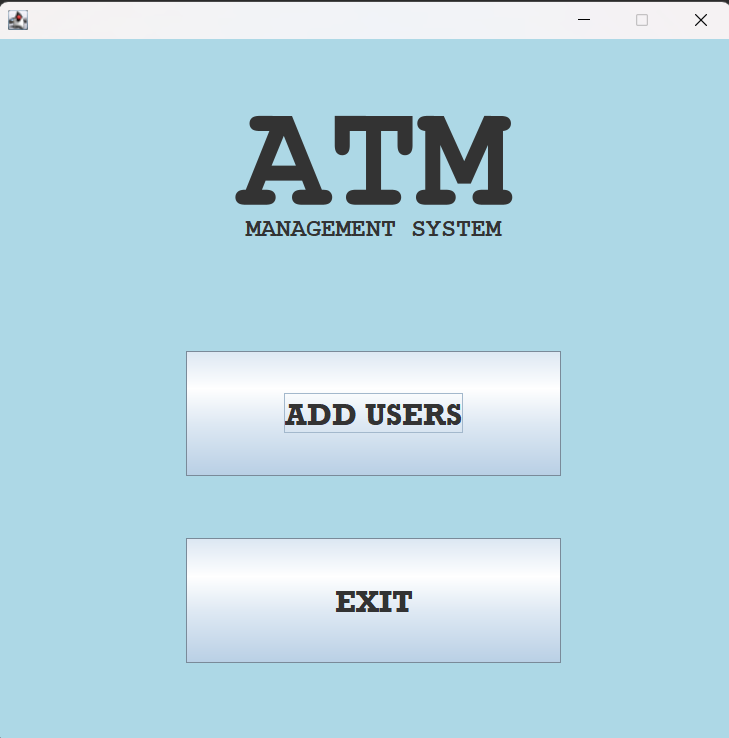
Add user button directs you to a page where you can add users with their current balance.
The exit button closes the application.
5. Module to add users
File Name: “AddUser.java“
This module helps the admin to add users to the database. The card number and password are generated automatically.
package atm; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.SQLException; import java.util.Random; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextField; public class AddUser { JTextField pinField, atmField; Random random = new Random(); public void addView() throws SQLException { Commons commons = new Commons(); JFrame frame = (JFrame) commons.Frame(); Font txt = new Font("", Font.BOLD, 20); SQLManage manage = new SQLManage(); Success success = new Success(); //--------------NAME-------------------- JLabel name = new JLabel("Name : "); name.setBounds(50, 200, 100, 25); name.setFont(txt); JTextField nmField = new JTextField(); nmField.setBounds(50, 230, 500, 30); frame.add(nmField); frame.add(name); //-------------------------------------- //-------------ATMNUMBER------------------ JLabel atmno = new JLabel("ATM Card Number : "); atmno.setBounds(50, 300, 500, 25); atmno.setFont(txt); atmField = new JTextField(); atmField.setBounds(50, 330, 500, 30); atmField.setEditable(false); frame.add(atmField); frame.add(atmno); //---------------------------------------- //-------------ATMPIN------------------ JLabel atmpin = new JLabel("ATM Card PIN : "); atmpin.setBounds(50, 400, 500, 25); atmpin.setFont(txt); pinField = new JTextField(); pinField.setBounds(50, 430, 200, 30); pinField.setEditable(false); frame.add(pinField); frame.add(atmpin); //---------------------------------------- //-------------BALANCE------------------ JLabel bal = new JLabel("BALANCE : "); bal.setBounds(350, 400, 500, 25); bal.setFont(txt); JTextField balField = new JTextField(); balField.setBounds(350, 430, 200, 30); frame.add(balField); frame.add(bal); //---------------------------------------- //--------------AUTOGENERATION---------------- auto(); //--------------------------------------------- //---------------SUBMIT------------------- JButton sbmt = new JButton("SUBMIT"); sbmt.setBounds(200, 500, 200, 50); frame.add(sbmt); sbmt.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(!nmField.getText().equals("")) { if(balField.getText().equals("")) balField.setText("0"); try { manage.adding(atmField.getText(), pinField.getText(), nmField.getText(), balField.getText()); } catch (SQLException e1) { e1.printStackTrace(); } success.detailView(atmField.getText(), pinField.getText()); balField.setText(""); nmField.setText(""); auto(); } } }); //------------------------------------------ frame.setVisible(true); } public void auto() { String str = ""; for(int i=0; i<16; i++) { str += random.nextInt(9 - 0 + 1) + 0; } atmField.setText(str); str = ""; for(int i=0; i<4; i++) { str += random.nextInt(9 - 0 + 1) + 0; } pinField.setText(str); } }

Enter name and balance to add the user into the system. The part of admin ended here.
6. Database Management Module
File Name: “SQLManage.java“
This java class does all the operations with the database. Other classes just call the method in this for all database operations to be done.
package atm; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class SQLManage { Connection con; SQLManage() throws SQLException { String usr = "root"; String pass = "password"; String url = "jdbc:mysql://localhost:3306/atm"; con = DriverManager.getConnection(url, usr, pass); } public ResultSet check(String usr, String pass) throws SQLException { String str = "SELECT * FROM users WHERE card = '"+ usr +"' AND pin = '"+ pass+ "'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); return rst; } public void deposit(int amt, int id) throws SQLException { String str = "UPDATE users SET bal = bal + "+amt+" WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(str); int bal = balCheck(id); str = "INSERT INTO transactions (id, amount, stat, bal) VALUES("+id+", "+amt+", 'dep', "+bal+")"; Statement stm2 = con.createStatement(); stm2.executeUpdate(str); } public int withdraw(int amt, int id) throws SQLException { int bal = balCheck(id); if(bal >= amt) { String str = "UPDATE users SET bal = bal - "+amt+" WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(str); bal -= amt; str = "INSERT INTO transactions (id, amount, stat, bal) VALUES("+id+", "+amt+", 'wit', "+bal+")"; Statement stm2 = con.createStatement(); stm2.executeUpdate(str); return 1; } return 0; } public void pinchange(String pin, int id) throws SQLException { String str = "UPDATE users SET pin = '"+pin+"' WHERE id = " + id; Statement stm = con.createStatement(); stm.executeUpdate(str); } public int balCheck(int id) throws SQLException { String str = "SELECT bal FROM users WHERE id = " + id; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); rst.next(); return rst.getInt("bal"); } public ResultSet stmt(int id) throws SQLException { String str = "SELECT * FROM transactions WHERE id = " + id + " order by transid desc"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); return rst; } public void adding(String card, String pin, String name, String bal) throws SQLException { String str = "INSERT INTO users (card, pin, uname, bal) values ('" +card+ "', '"+pin+"', '"+name+"', "+bal+")"; Statement stm = con.createStatement(); stm.executeUpdate(str); } }
7. Common Frame and Logo
File Name: “Commons.java“
This class here allows us to avoid code duplication. Calling the function in this class gets us the frame and logo on the screen.
package atm; import java.awt.Color; import java.awt.Component; import java.awt.Font; import javax.swing.JFrame; import javax.swing.JLabel; public class Commons { public Component Frame() { JFrame frame = new JFrame(); frame.setSize(600, 600); frame.setLocationRelativeTo(null); frame.setLayout(null); frame.setResizable(false); frame.getContentPane().setBackground(Color.decode("#ADD8E6")); //------------------LOGO---------------------------------- JLabel atm = new JLabel("ATM"); atm.setBounds(0, 30, 600, 120); atm.setHorizontalAlignment(JLabel.CENTER); atm.setFont(new Font("Monospaced", Font.BOLD, 120)); JLabel man = new JLabel("MANAGEMENT SYSTEM"); man.setBounds(0, 140, 600, 20); man.setHorizontalAlignment(JLabel.CENTER); man.setFont(new Font("Monospaced", Font.BOLD, 20)); frame.add(man); frame.add(atm); //----------------------------------------------- return frame; } }
8. Success Page
File Name: “Success.java“
This module here shows us the success page when a transaction is successfully executed. Invokes a new page when called.
package atm; import java.awt.Font; import java.sql.SQLException; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextField; public class Success { public void successView(int id) throws SQLException { Home home = new Home(); Commons commons = new Commons(); JFrame frame =(JFrame) commons.Frame(); //-----------------SUCCESS------------------ JLabel sucss = new JLabel("TRANSACTION SUCCESS."); sucss.setBounds(0, 280, 600, 50); sucss.setHorizontalAlignment(JLabel.CENTER); sucss.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(sucss); //----------------------------------------- home.homeView(id); frame.setVisible(true); } public void detailView(String num, String pin) { Commons commons = new Commons(); JFrame frame =(JFrame) commons.Frame(); //-----------------DETAILS------------------ JLabel sucss = new JLabel("REMEMBER THE DETAILS!!!"); sucss.setBounds(0, 200, 600, 50); sucss.setHorizontalAlignment(JLabel.CENTER); sucss.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(sucss); JTextField number = new JTextField("CARD NUMBER : " + num); number.setBounds(0, 300, 600, 50); number.setEditable(false); number.setHorizontalAlignment(JLabel.CENTER); number.setFont(new Font("Rockwell", Font.BOLD, 20)); frame.add(number); JTextField pinno = new JTextField("DEFAULT PIN : " + pin); pinno.setBounds(0, 400, 600, 50); pinno.setHorizontalAlignment(JLabel.CENTER); pinno.setEditable(false); pinno.setFont(new Font("Rockwell", Font.BOLD, 20)); frame.add(pinno); //----------------------------------------- frame.setVisible(true); } }

Here we have a page labeled “TRANSACTION SUCCESSFUL“. There is also another method executed in this class if adding users is done.

9. Failed Transaction Page
File Name: “Fail.java“
This Page here invokes whenever a transaction is failed.
package atm; import java.awt.Font; import javax.swing.JFrame; import javax.swing.JLabel; public class Fail { public void failView(String str) { Commons commons = new Commons(); JFrame frame =(JFrame) commons.Frame(); //-----------------FAIL------------------ JLabel fail = new JLabel("YOUR TRANSACTIONS FAILED!!!"); fail.setBounds(0, 280, 600, 50); fail.setHorizontalAlignment(JLabel.CENTER); fail.setFont(new Font("Rockwell", Font.BOLD, 25)); JLabel st = new JLabel(str); st.setBounds(0, 320, 600, 50); st.setHorizontalAlignment(JLabel.CENTER); st.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(st); frame.add(fail); //----------------------------------------- frame.setVisible(true); } }

Here the page is labeled with the string “TRANSACTION FAILED” and the reason for it.
10. Home Page for Users
File Name: “Home.java“
This shows us the home page for the user. It contains the options that are available for the users to use. All options are given as labels and are forwarded as per the instruction.
package atm; import java.awt.Font; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.SQLException; import javax.swing.JFrame; import javax.swing.JLabel; public class Home { public void homeView(int id) throws SQLException { Operations operations = new Operations(); Font txt = new Font("", Font.BOLD, 25); Commons commons = new Commons(); JFrame frame = (JFrame)commons.Frame(); JLabel quick = new JLabel("< Quick Cash"); quick.setBounds(30, 250, 200, 30); quick.setFont(txt); JLabel withdraw = new JLabel("Withdraw >"); withdraw.setBounds(350, 250, 200, 30); withdraw.setHorizontalAlignment(JLabel.RIGHT); withdraw.setFont(txt); JLabel deposit = new JLabel("< Deposit"); deposit.setBounds(30, 350, 200, 30); deposit.setFont(txt); JLabel sts = new JLabel("Mini Statement >"); sts.setBounds(350, 350, 200, 30); sts.setHorizontalAlignment(JLabel.RIGHT); sts.setFont(txt); JLabel bal = new JLabel("< Balance Enquiry"); bal.setBounds(30, 450, 250, 30); bal.setFont(txt); JLabel pinchange = new JLabel("Change Pin >"); pinchange.setBounds(350, 450, 200, 30); pinchange.setHorizontalAlignment(JLabel.RIGHT); pinchange.setFont(txt); frame.add(quick); frame.add(withdraw); frame.add(deposit); frame.add(sts); frame.add(bal); frame.add(pinchange); frame.setVisible(true); quick.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { Quick qk = new Quick(); try { qk.quickView(id); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); withdraw.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { try { operations.opView("Withdraw Amount", id); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); deposit.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { try { operations.opView("Deposit Amount", id); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); sts.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { Statements state = new Statements(); try { state.stateView(id); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); bal.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { try { operations.opView("Balance", id); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); pinchange.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { try { operations.opView("New PIN", id); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); } }
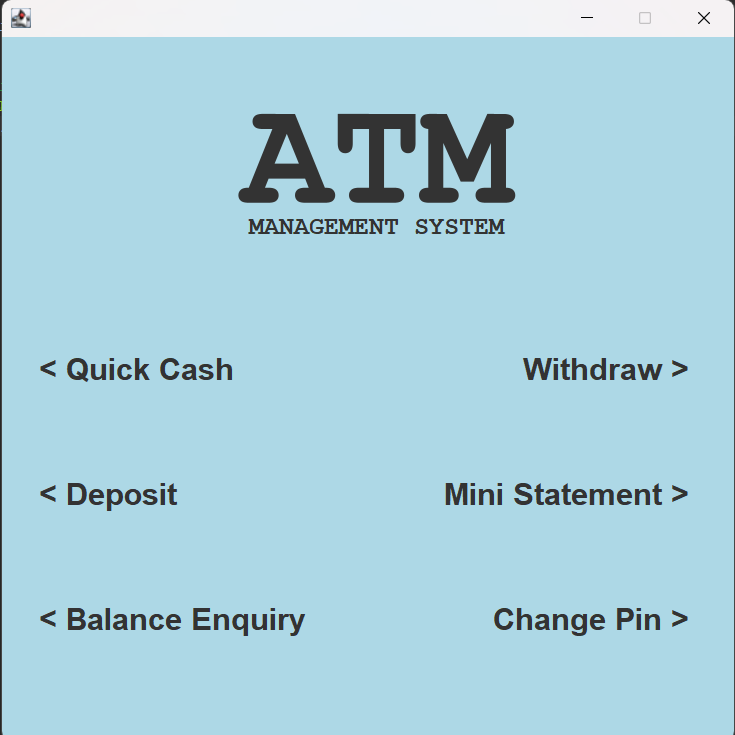
As we can see, there are 6 options available for the users. We can choose any to go forward with the same.
11. Quick Cash Window
File Name: “Quick.java“
This is the module to View the quick cash withdrawal window. It is to withdraw money faster.
package atm; import java.awt.Font; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.SQLException; import javax.swing.JFrame; import javax.swing.JLabel; public class Quick { public void quickView(int id) throws SQLException { Operations oper = new Operations(); Font txt = new Font("", Font.BOLD, 25); Commons commons = new Commons(); JFrame frame = (JFrame)commons.Frame(); JLabel two = new JLabel("< 200"); two.setBounds(30, 250, 200, 30); two.setFont(txt); JLabel five = new JLabel("500 >"); five.setBounds(350, 250, 200, 30); five.setHorizontalAlignment(JLabel.RIGHT); five.setFont(txt); JLabel ten = new JLabel("< 1000"); ten.setBounds(30, 350, 200, 30); ten.setFont(txt); JLabel twenty = new JLabel("2000 >"); twenty.setBounds(350, 350, 200, 30); twenty.setHorizontalAlignment(JLabel.RIGHT); twenty.setFont(txt); JLabel fifty = new JLabel("< 5000"); fifty.setBounds(30, 450, 250, 30); fifty.setFont(txt); JLabel hundred = new JLabel("10000 >"); hundred.setBounds(350, 450, 200, 30); hundred.setHorizontalAlignment(JLabel.RIGHT); hundred.setFont(txt); frame.add(two); frame.add(five); frame.add(ten); frame.add(twenty); frame.add(fifty); frame.add(hundred); frame.setVisible(true); two.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { oper.withdrawal(200, id); frame.dispose(); } }); five.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { oper.withdrawal(500, id); frame.dispose(); } }); ten.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { oper.withdrawal(1000, id); frame.dispose(); } }); twenty.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { oper.withdrawal(2000, id); frame.dispose(); } }); fifty.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { oper.withdrawal(5000, id); frame.dispose(); } }); hundred.addMouseListener(new MouseAdapter() { public void mousePressed(MouseEvent e) { oper.withdrawal(10000, id); frame.dispose(); } }); } }

Here many amounts are fed by default. By Selecting any one of them, that amount will be remitted from the account balance.
12. Other Operations
File Name: “Operations.java“
This module shows a window on the options you selected and does the operation that is needed.
package atm; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.SQLException; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextField; public class Operations { SQLManage manage; Fail fail; Success success; Operations() throws SQLException { manage = new SQLManage(); fail = new Fail(); success = new Success(); } public void opView(String str, int id) throws SQLException { Commons commons = new Commons(); JFrame frame = (JFrame)commons.Frame(); Font txt = new Font("", Font.BOLD, 15); //-----------------AMOUNT/PIN------------------ JLabel label = new JLabel("Enter the " + str); label.setBounds(50, 270, 250, 20); label.setFont(txt); JTextField amt = new JTextField(); amt.setBounds(50, 300, 500, 35); amt.setFont(txt); frame.add(label); frame.add(amt); //---------------------------------------------- //------------------SUBMIT------------------------ JButton sbt = new JButton("SUBMIT"); sbt.setBounds(200, 400, 200, 50); sbt.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(sbt); sbt.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(str.equals("Withdraw Amount")) { withdrawal(Integer.parseInt(amt.getText()), id); frame.dispose(); } else if(str.equals("Deposit Amount")) { try { manage.deposit(Integer.parseInt(amt.getText()), id); success.successView(id); frame.dispose(); } catch (SQLException e1) { e1.printStackTrace(); } } else if(str.equals("New PIN")){ try { manage.pinchange(amt.getText(), id); success.successView(id); frame.dispose(); } catch (SQLException e1) { e1.printStackTrace(); } } } }); //------------------------------------------------ if (str.equals("Balance")){ amt.setVisible(false); sbt.setVisible(false); label.setText("Your Balance is : "); JLabel bal; try { bal = new JLabel(manage.balCheck(id)+""); bal.setBounds(0, 325, 600, 20); bal.setHorizontalAlignment(JLabel.CENTER); bal.setFont(new Font("", Font.BOLD, 25)); frame.add(bal); } catch (SQLException e1) { e1.printStackTrace(); } } frame.setVisible(true); } public void withdrawal(int amount, int id) { try { int check = manage.withdraw(amount, id); if(check==1) { success.successView(id); } else { fail.failView("INSUFFICIENT BALANCE!!!"); } } catch (SQLException e1) { e1.printStackTrace(); } } }

All data for the deposit, withdrawal, changing pin, etc… are fed here. Enter the data and press SUBMIT to continue the transaction.
13. Bank statements
File Name: “Statements.java“
This module helps us in retrieving the data of transactions that we have done. This data is shown in a table.
package atm; import java.awt.Font; import java.sql.ResultSet; import java.sql.SQLException; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.table.DefaultTableModel; public class Statements { public void stateView(int id) throws SQLException { DefaultTableModel model = new DefaultTableModel(); Commons commons = new Commons(); JFrame frame = (JFrame)commons.Frame(); SQLManage manage = new SQLManage(); //----------------LABEL----------------------- JLabel label = new JLabel("MINI STATEMENTS"); label.setBounds(0, 200, 575, 30); label.setHorizontalAlignment(JLabel.CENTER); label.setFont(new Font("Rockwell", Font.BOLD, 25)); frame.add(label); //-------------------------------------------- //---------------TABLE-------------------- JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ return false; } }; model = (DefaultTableModel)table.getModel(); model.addColumn("ID"); model.addColumn("DEPOSIT"); model.addColumn("WITHDRAW"); model.addColumn("BALANCE"); table.getColumnModel().getColumn(0).setPreferredWidth(50); table.getColumnModel().getColumn(1).setPreferredWidth(150); table.getColumnModel().getColumn(2).setPreferredWidth(150); table.getColumnModel().getColumn(2).setPreferredWidth(150); JScrollPane sc = new JScrollPane(table); sc.setBounds(50, 250, 500, 200); frame.add(sc); //----------------------------------------------- //--------------------TABLEDATA------------------------ ResultSet rst = manage.stmt(id); int i=0; while(rst.next()) { model.addRow(new Object[0]); model.setValueAt(rst.getInt("transid"), i, 0); if(rst.getString("stat").equals("dep")) { model.setValueAt(rst.getString("amount"), i, 1); model.setValueAt("-", i, 2); } else { model.setValueAt("-", i, 1); model.setValueAt(rst.getString("amount"), i, 2); } model.setValueAt(rst.getInt("bal"), i, 3); i++; } //----------------------------------------------------- frame.setVisible(true); } }

As we can see above, The deposit and withdrawal amounts are stated with the current balance. The latest transactions are represented first.
Output for ATM Management System Project in Java
The user Module:
The admin Module:
Conclusion
So in this project, we have learned to design a simple ATM Management System Project in Java with a database using MySQL. We can deposit, withdraw, change pin, and view statements and balances in this system. Adding user access is given to the admin.
Hope this GUI-based program helps you guys a lot!
Thanking you for visiting our website.
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023
- Snake Game in Java