Introduction
In this article, we will build Hospital Management System Project in Java and MySQL with source code. This project is great for those at an intermediate level in Java who want to advance their coding skills. In this project, the users will be able to perform the following functionalities Admin Login, Patient details, Doctor details, Room details, and Services. Let’s get started!
Setting the development environment
You must have java JDK installed on your system and we are using Eclipse IDE to build this project, you can use either this or Netbeans IDE.
The first step will be to create a new project. Name it as you wish. In the src folder create a hospital package. In that package, we will be creating some files for different modules.
To connect the system with the database you will need to follow specific steps.
- Have Java JDK already installed and an IDE like Eclipse
- Install MySQL on the system.
- Download the MySQL connector from here.
- In Eclipse, under your project, expand external libraries, right-click, and select Open Library Settings. Select the libraries tab and click on the + button. Browse your jar file downloaded from the above step and click on it. This will add a dependency to your project. The steps will differ if you are using a different IDE.
MySql Setup for Hospital Management System Project in Java
1. Create a database
-- Creating database
create database hospital;
2. Select the database
-- Selecting the database
use hospital;
3. Create an admin table
-- Create admin table
create table admin (
login_id varchar(25),
password varchar(25)
);
4. Create a Patients table
-- Creating Patients table
create table patients (
patient_id int primary key,
patient_name varchar(25),
fathers_name varchar(15),
address varchar(250),
contact_no varchar(10),
age int
);
5. Create a doctor’s table
-- Creating doctors table
create table doctors (
doctor_id int primary key,
doctor_name varchar(25),
father_name varchar(25),
contact_no varchar(10),
address varchar(250)
date_joining date
);
6. Create a room table
-- Create room table
create table room (
room_no int,
room_charges int
);
6. Insert some values in the admin table
-- Inserting values
insert into admin values
("admin","1234")
Implementation of the Hospital Management System Project in Java
1. Login module
This module helps to add new users who can enter the user name and password to the hospital management system in java. Name the file login.java.
import java.awt.HeadlessException; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import javax.swing.JOptionPane; import java.sql.*; public class login extends javax.swing.JFrame { public login() { initComponents(); } @SuppressWarnings("unchecked") private void initComponents() { jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); txtUsername = new javax.swing.JTextField(); jButton1 = new javax.swing.JButton(); jPassword = new javax.swing.JPasswordField(); jLabel1.setFont(new java.awt.Font("Arial", 0, 18)); jLabel1.setText("Username"); jLabel2.setFont(new java.awt.Font("Arial", 0, 18)); jLabel2.setText("Password"); jButton1.setText("OK"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(27, 27, 27) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel1) .addComponent(jLabel2)) .addGap(35, 35, 35) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addComponent(txtUsername) .addComponent(jPassword, javax.swing.GroupLayout.DEFAULT_SIZE, 122, Short.MAX_VALUE)) .addContainerGap(61, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(jButton1, javax.swing.GroupLayout.PREFERRED_SIZE, 95, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(24, 24, 24)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(62, 62, 62) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel1) .addComponent(txtUsername, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(29, 29, 29) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel2) .addComponent(jPassword, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(44, 44, 44) .addComponent(jButton1) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) ); pack(); } private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) { Connection con=null; ResultSet rs=null; PreparedStatement pst=null; if (txtUsername.getText().equals("")) { JOptionPane.showMessageDialog( this, "Please enter user name"); return; } if (jPassword.getText().equals("")) { JOptionPane.showMessageDialog( this, "Please enter password"); return; } con=Connect.ConnectDB(); String sq1= "select * from users where user_name= '" + txtUsername.getText() + "' and password ='" + jPassword.getText() + "'"; try{ pst=con.prepareStatement(sq1); rs= pst.executeQuery(); if (rs.next()){ this.hide(); Main frm=new Main(); frm.setVisible(true); } else{ JOptionPane.showMessageDialog(null, "Login Failed..Try again !"); } }catch(SQLException | HeadlessException e){ JOptionPane.showMessageDialog(null, e); // TODO add your handling code here: } } public static void main(String args[]) { try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Metal".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(login.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new login().setVisible(true); } }); } private javax.swing.JButton jButton1; private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JPasswordField jPassword; private javax.swing.JTextField txtUsername; }
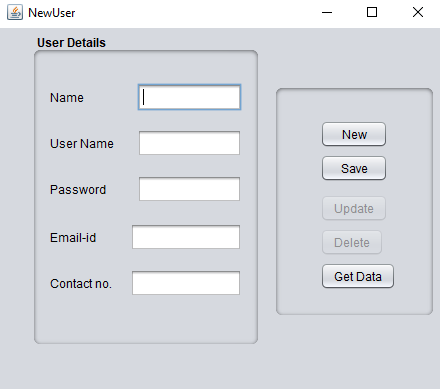
2. Home page Module
Once we enter the correct username and password on the login page, it takes us to the home page of our hospital management application. Name the file main.java
public class Main extends javax.swing.JFrame { public Main() { initComponents(); } @SuppressWarnings("unchecked") private void initComponents() jMenuItem11 = new javax.swing.JMenuItem(); jPanel1 = new javax.swing.JPanel(); jLabel2 = new javax.swing.JLabel(); jMenuBar1 = new javax.swing.JMenuBar(); jMenu1 = new javax.swing.JMenu(); jMenuItem1 = new javax.swing.JMenuItem(); jMenuItem2 = new javax.swing.JMenuItem(); jMenuItem3 = new javax.swing.JMenuItem(); jMenu2 = new javax.swing.JMenu(); jMenuItem6 = new javax.swing.JMenuItem(); jMenuItem7 = new javax.swing.JMenuItem(); jMenu3 = new javax.swing.JMenu(); jMenuItem4 = new javax.swing.JMenuItem(); jMenu5 = new javax.swing.JMenu(); jMenu7 = new javax.swing.JMenu(); jMenuItem8 = new javax.swing.JMenuItem(); jMenu8 = new javax.swing.JMenu(); jMenuItem9 = new javax.swing.JMenuItem(); jMenu9 = new javax.swing.JMenu(); jMenuItem12 = new javax.swing.JMenuItem(); jMenu6 = new javax.swing.JMenu(); jMenuItem10 = new javax.swing.JMenuItem(); jMenu4 = new javax.swing.JMenu(); jMenuItem5 = new javax.swing.JMenuItem(); jMenuItem11.setText("jMenuItem11"); setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); jPanel1.setBackground(new java.awt.Color(52,122,235)); jLabel2.setFont(new java.awt.Font("Impact", 1, 36)); jLabel2.setForeground(new java.awt.Color(255,255,255)); jLabel2.setText("Hospital Management System"); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup() .addContainerGap(174, Short.MAX_VALUE) .addComponent(jLabel2) .addGap(150, 150, 150)) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(157, 157, 157) .addComponent(jLabel2) .addContainerGap(190, Short.MAX_VALUE)) ); jMenu1.setText("Admin"); jMenu1.setFont(new java.awt.Font("Arial", 0, 18)); jMenu1.setPreferredSize(new java.awt.Dimension(60, 25)); jMenuItem1.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_N, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem1.setText("New User"); jMenuItem1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem1ActionPerformed(evt); } }); jMenu1.add(jMenuItem1); jMenuItem2.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_C, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem2.setText("Change Password"); jMenuItem2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem2ActionPerformed(evt); } }); jMenu1.add(jMenuItem2); jMenuItem3.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_L, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem3.setText("Login details"); jMenuItem3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem3ActionPerformed(evt); } }); jMenu1.add(jMenuItem3); jMenuBar1.add(jMenu1); jMenu2.setText("Patients"); jMenu2.setFont(new java.awt.Font("Arial", 0, 18)); jMenu2.setPreferredSize(new java.awt.Dimension(70, 25)); jMenuItem6.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_R, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem6.setText("Registration"); jMenuItem6.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem6ActionPerformed(evt); } }); jMenu2.add(jMenuItem6); jMenuItem7.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_S, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem7.setText("Services"); jMenuItem7.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem7ActionPerformed(evt); } }); jMenu2.add(jMenuItem7); jMenuBar1.add(jMenu2); jMenu3.setText("Doctors"); jMenu3.setFont(new java.awt.Font("Arial", 0, 18)); jMenu3.setPreferredSize(new java.awt.Dimension(71, 25)); jMenuItem4.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_E, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem4.setText("Entry"); jMenuItem4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem4ActionPerformed(evt); } }); jMenu3.add(jMenuItem4); jMenuBar1.add(jMenu3); jMenu5.setText("Rooms"); jMenu5.setFont(new java.awt.Font("Arial", 0, 18)); jMenu7.setText("Add rooms"); jMenuItem8.setText("Room"); jMenuItem8.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem8ActionPerformed(evt); } }); jMenu7.add(jMenuItem8); jMenu5.add(jMenu7); jMenu8.setText("Admit"); jMenuItem9.setText("Room"); jMenuItem9.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem9ActionPerformed(evt); } }); jMenu8.add(jMenuItem9); jMenu5.add(jMenu8); jMenu9.setText("Discharge"); jMenuItem12.setText("Room"); jMenuItem12.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem12ActionPerformed(evt); } }); jMenu9.add(jMenuItem12); jMenu5.add(jMenu9); jMenu6.setText("Billing"); jMenuItem10.setText("Room"); jMenuItem10.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem10ActionPerformed(evt); } }); jMenu6.add(jMenuItem10); jMenu5.add(jMenu6); jMenuBar1.add(jMenu5); jMenu4.setText("Services"); jMenu4.setFont(new java.awt.Font("Arial", 0, 18)); jMenu4.setPreferredSize(new java.awt.Dimension(75, 25)); jMenuItem5.setAccelerator(javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_I, java.awt.event.InputEvent.CTRL_MASK)); jMenuItem5.setText("About"); jMenuItem5.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem5ActionPerformed(evt); } }); jMenu4.add(jMenuItem5); jMenuBar1.add(jMenu4); setJMenuBar(jMenuBar1); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) ); pack(); } private void jMenuItem2ActionPerformed(java.awt.event.ActionEvent evt) { //GEN-FIRST:event_jMenuItem2ActionPerformed ChangePassword ob1=new ChangePassword(); ob1.setVisible(true); } private void jMenuItem1ActionPerformed(java.awt.event.ActionEvent evt) { //GEN-FIRST:event_jMenuItem1ActionPerformed NewUser ob1=new NewUser(); ob1.setVisible(true); } private void jMenuItem3ActionPerformed(java.awt.event.ActionEvent evt) { //GEN-FIRST:event_jMenuItem3ActionPerformed LoginDetails ob1=new LoginDetails(); ob1.setVisible(true); } private void jMenuItem4ActionPerformed(java.awt.event.ActionEvent evt) { //GEN-FIRST:event_jMenuItem4ActionPerformed Entry ob1=new Entry(); ob1.setVisible(true); }//GEN-LAST:event_jMenuItem4ActionPerformed private void jMenuItem5ActionPerformed(java.awt.event.ActionEvent evt) { //GEN-FIRST:event_jMenuItem5ActionPerformed About ob1=new About(); ob1.setVisible(true); }//GEN-LAST:event_jMenuItem5ActionPerformed private void jMenuItem6ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem6ActionPerformed Registration ob1=new Registration(); ob1.setVisible(true); }//GEN-LAST:event_jMenuItem6ActionPerformed private void jMenuItem7ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem7ActionPerformed Services ob1=new Services(); ob1.setVisible(true); }//GEN-LAST:event_jMenuItem7ActionPerformed private void jMenuItem8ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem8ActionPerformed Room obj1=new Room(); obj1.setVisible(true); }//GEN-LAST:event_jMenuItem8ActionPerformed private void jMenuItem9ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem9ActionPerformed Patient_Admit_Room obj1=new Patient_Admit_Room(); obj1.setVisible(true); }//GEN-LAST:event_jMenuItem9ActionPerformed private void jMenuItem10ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem10ActionPerformed Bill_Room obj1=new Bill_Room(); obj1.setVisible(true); }//GEN-LAST:event_jMenuItem10ActionPerformed private void jMenuItem12ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem12ActionPerformed Patient_Discharge_room obj1=new Patient_Discharge_room(); obj1.setVisible(true); } public static void main(String args[]){ try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(Main.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new Main().setVisible(true); } }); } private javax.swing.JLabel jLabel2; private javax.swing.JMenu jMenu1; private javax.swing.JMenu jMenu2; private javax.swing.JMenu jMenu3; private javax.swing.JMenu jMenu4; private javax.swing.JMenu jMenu5; private javax.swing.JMenu jMenu6; private javax.swing.JMenu jMenu7; private javax.swing.JMenu jMenu8; private javax.swing.JMenu jMenu9; private javax.swing.JMenuBar jMenuBar1; private javax.swing.JMenuItem jMenuItem1; private javax.swing.JMenuItem jMenuItem10; private javax.swing.JMenuItem jMenuItem11; private javax.swing.JMenuItem jMenuItem12; private javax.swing.JMenuItem jMenuItem2; private javax.swing.JMenuItem jMenuItem3; private javax.swing.JMenuItem jMenuItem4; private javax.swing.JMenuItem jMenuItem5; private javax.swing.JMenuItem jMenuItem6; private javax.swing.JMenuItem jMenuItem7; private javax.swing.JMenuItem jMenuItem8; private javax.swing.JMenuItem jMenuItem9; private javax.swing.JPanel jPanel1; }

3. Patient Module
This module helps to add new patient details in the hospital management system in java. Also, we can edit or delete the existing patient records. Name the file Patient.java
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import javax.swing.JOptionPane; import net.proteanit.sql.DbUtils; public class PatientRec extends javax.swing.JFrame { Connection con=null; ResultSet rs=null; PreparedStatement pst=null; public PatientRec() { initComponents(); con= Connect.ConnectDB(); Get_Data(); } private void Get_Data(){ String sql="select PatientID as 'Patient ID', PatientName as 'Patient Name',FatherName as 'Father Name',Address,ContactNo as 'Contact No',Email as 'Email ID',Age,Gen as 'Gender',BG as 'Blood Group',Remarks from Patientregistration"; try{ pst=con.prepareStatement(sql); rs= pst.executeQuery(); jTable1.setModel(DbUtils.resultSetToTableModel(rs)); }catch(Exception e){ JOptionPane.showMessageDialog(null, e); } } @SuppressWarnings("unchecked") private void initComponents() { jScrollPane1 = new javax.swing.JScrollPane(); jTable1 = new javax.swing.JTable(); setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE); addWindowListener(new java.awt.event.WindowAdapter() { public void windowClosing(java.awt.event.WindowEvent evt) { formWindowClosing(evt); } }); jTable1.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { jTable1MouseClicked(evt); } }); jScrollPane1.setViewportView(jTable1); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jScrollPane1, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, 612, Short.MAX_VALUE) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 388, Short.MAX_VALUE) ); pack(); } private void jTable1MouseClicked(java.awt.event.MouseEvent evt) { //GEN-FIRST:event_jTable1MouseClicked try{ con=Connect.ConnectDB(); int row= jTable1.getSelectedRow(); String table_click= jTable1.getModel().getValueAt(row, 0).toString(); String sql= "select * from PatientRegistration where PatientID = '" + table_click + "'"; pst=con.prepareStatement(sql); rs= pst.executeQuery(); if(rs.next()){ this.hide(); Registration frm = new Registration(); frm.setVisible(true); String add1=rs.getString("PatientID"); frm.txtId.setText(add1); String add2=rs.getString("Patientname"); frm.txtName.setText(add2); String add3=rs.getString("Fathername"); frm.txtFname.setText(add3); String add5=rs.getString("Email"); frm.txtEmail.setText(add5); int add6 = rs.getInt("Age"); String add= Integer.toString(add6); frm.txtAge.setText(add); String add7=rs.getString("Remarks"); frm.txtInfo.setText(add7); String add9=rs.getString("BG"); frm.cmbBG.setSelectedItem(add9); String add11=rs.getString("Gen"); frm.cmbGender.setSelectedItem(add11); String add15=rs.getString("Address"); frm.txtAdd.setText(add15); String add16=rs.getString("ContactNo"); frm.txtContact.setText(add16); frm.btnUpdate.setEnabled(true); frm.btnDelete.setEnabled(true); frm.btnSave.setEnabled(false); } }catch(Exception ex){ JOptionPane.showMessageDialog(this,ex); } }//GEN-LAST:event_jTable1MouseClicked private void formWindowClosing(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_formWindowClosing } public static void main(String args[]) { try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(PatientRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(PatientRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(PatientRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(PatientRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new PatientRec().setVisible(true); } }); } }
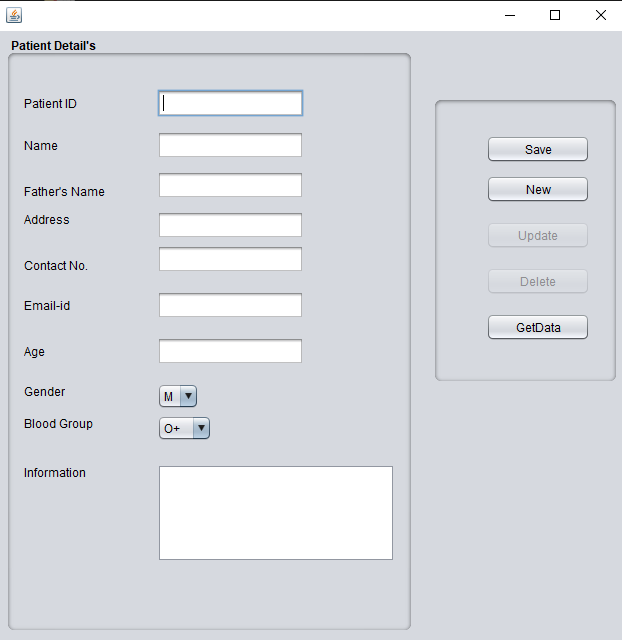
4. Doctor Module
This module helps to add doctor details in the hospital management system in java. Also, we can edit or delete the doctor’s details. Name the file Doctor.java
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import javax.swing.JOptionPane; import net.proteanit.sql.DbUtils; public class DocRec extends javax.swing.JFrame { Connection con=null; ResultSet rs=null; PreparedStatement pst=null; public DocRec() { initComponents(); con= Connect.ConnectDB(); Get_Data(); } private void Get_Data(){ String sql="select DoctorID as 'Doctor ID', DoctorName as 'Doctor Name',FatherName as 'Father Name',Address,ContacNo as 'Contact No',Email as 'Email ID',Qualifications,Gender,BloodGroup as 'Blood Group',DateOfJoining as 'Joining Date' from Doctor order by DoctorName"; try{ pst=con.prepareStatement(sql); rs= pst.executeQuery(); jTable1.setModel(DbUtils.resultSetToTableModel(rs)); }catch(Exception e){ JOptionPane.showMessageDialog(null, e); } } @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents private void initComponents() { jScrollPane1 = new javax.swing.JScrollPane(); jTable1 = new javax.swing.JTable(); setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE); addWindowListener(new java.awt.event.WindowAdapter() { public void windowClosing(java.awt.event.WindowEvent evt) { formWindowClosing(evt); } }); jTable1.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { jTable1MouseClicked(evt); } }); jScrollPane1.setViewportView(jTable1); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 612, Short.MAX_VALUE) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 345, Short.MAX_VALUE) ); pack(); } private void jTable1MouseClicked(java.awt.event.MouseEvent evt) { //GEN-FIRST:event_jTable1MouseClicked try{ con=Connect.ConnectDB(); int row= jTable1.getSelectedRow(); String table_click= jTable1.getModel().getValueAt(row, 0).toString(); String sql= "select * from Doctor where DoctorID = '" + table_click + "'"; pst=con.prepareStatement(sql); rs= pst.executeQuery(); if(rs.next()){ this.hide(); Entry frm = new Entry(); frm.setVisible(true); String add1=rs.getString("DoctorID"); frm.txtId.setText(add1); String add2=rs.getString("Doctorname"); frm.txtName.setText(add2); String add3=rs.getString("Fathername"); frm.txtFname.setText(add3); String add5=rs.getString("Email"); frm.txtE.setText(add5); String add6=rs.getString("Qualifications"); frm.txtQ.setText(add6); String add9=rs.getString("BloodGroup"); frm.cmbB.setSelectedItem(add9); String add11=rs.getString("Gender"); frm.cmbG.setSelectedItem(add11); String add14=rs.getString("DateOfJoining"); frm.txtD.setText(add14); String add15=rs.getString("Address"); frm.txtAd.setText(add15); String add16=rs.getString("ContacNo"); frm.txtC.setText(add16); frm.btnUpdate.setEnabled(true); frm.btnDelete.setEnabled(true); frm.btnSave.setEnabled(false); } }catch(Exception ex){ JOptionPane.showMessageDialog(this,ex); } }//GEN-LAST:event_jTable1MouseClicked private void formWindowClosing(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_formWindowClosing } public static void main(String args[]) { try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(DocRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(DocRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(DocRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(DocRec.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } java.awt.EventQueue.invokeLater(new Runnable() { @Override public void run() { new DocRec().setVisible(true); } }); } }

5. Rooms Module
This module helps to add new room details in the hospital management system in java. Also, we can edit or delete the existing room details. Name the file Room.java
import java.awt.HeadlessException; import java.awt.event.KeyEvent; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JOptionPane; import net.proteanit.sql.DbUtils; public class Room extends javax.swing.JFrame { Connection con=null; ResultSet rs=null; PreparedStatement pst=null; public Room() { initComponents(); con= Connect.ConnectDB(); Get_Data(); setLocationRelativeTo(null); } private void Reset() { txtRoomNo.setText(""); txtRoomCharges.setText(""); cmbRoomType.setSelectedIndex(-1); btnSave.setEnabled(true); btnDelete.setEnabled(false); btnUpdate.setEnabled(false); txtRoomNo.requestDefaultFocus(); Get_Data(); } private void Get_Data(){ String sql="select RoomNo as 'Room No.',RoomType as 'Room Type', RoomCharges as 'Room Charges',RoomStatus as 'Room Status' from Room"; try{ pst=con.prepareStatement(sql); rs= pst.executeQuery(); Room_table.setModel(DbUtils.resultSetToTableModel(rs)); }catch(Exception e){ JOptionPane.showMessageDialog(null, e); }} @SuppressWarnings("unchecked") private void initComponents() { jPanel1 = new javax.swing.JPanel(); jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); txtRoomNo = new javax.swing.JTextField(); cmbRoomType = new javax.swing.JComboBox(); txtRoomCharges = new javax.swing.JTextField(); jLabel4 = new javax.swing.JLabel(); jPanel3 = new javax.swing.JPanel(); btnNew = new javax.swing.JButton(); btnSave = new javax.swing.JButton(); btnUpdate = new javax.swing.JButton(); btnDelete = new javax.swing.JButton(); btnGetData = new javax.swing.JButton(); jScrollPane1 = new javax.swing.JScrollPane(); Room_table = new javax.swing.JTable(); jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Room Info")); jLabel1.setText("Room No."); jLabel2.setText("Room Type"); jLabel3.setText("Room Charges"); cmbRoomType.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "General", "Deluxe" })); cmbRoomType.setSelectedIndex(-1); txtRoomCharges.addKeyListener(new java.awt.event.KeyAdapter() { public void keyTyped(java.awt.event.KeyEvent evt) { txtRoomChargesKeyTyped(evt); } }); private void txtRoomChargesKeyTyped(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtRoomChargesKeyTyped } private void btnNewActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnNewActionPerformed Reset(); } private void btnSaveActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnSaveActionPerformed try{ con=Connect.ConnectDB(); if (txtRoomNo.getText().equals("")) { JOptionPane.showMessageDialog( this, "Please enter room no.","Error", JOptionPane.ERROR_MESSAGE); return; } if (cmbRoomType.getSelectedItem().equals("")) { JOptionPane.showMessageDialog( this, "Please select room type","Error", JOptionPane.ERROR_MESSAGE); return; } if (txtRoomCharges.getText().equals("")) { JOptionPane.showMessageDialog( this, "Please enter room Charges","Error", JOptionPane.ERROR_MESSAGE); return; } Statement stmt; stmt= con.createStatement(); String sql1="Select RoomNo from Room where RoomNo= '" + txtRoomNo.getText() + "'"; rs=stmt.executeQuery(sql1); if(rs.next()){ JOptionPane.showMessageDialog( this, "Room No. already exists","Error", JOptionPane.ERROR_MESSAGE); txtRoomNo.setText(""); txtRoomNo.requestDefaultFocus(); return; } String sql= "insert into Room(RoomNo,RoomType,RoomCharges,RoomStatus)values('"+ txtRoomNo.getText() + "','"+ cmbRoomType.getSelectedItem() + "'," + txtRoomCharges.getText() + ",'Vacant')"; pst=con.prepareStatement(sql); pst.execute(); JOptionPane.showMessageDialog(this,"Successfully saved","Room Record",JOptionPane.INFORMATION_MESSAGE); btnSave.setEnabled(false); Get_Data(); }catch(HeadlessException | SQLException ex){ JOptionPane.showMessageDialog(this,ex); } } private void btnUpdateActionPerformed(java.awt.event.ActionEvent evt) { //GEN-FIRST:event_btnUpdateActionPerformed try{ con=Connect.ConnectDB(); String sql= "update Room set Roomtype='"+ cmbRoomType.getSelectedItem() + "',RoomCharges=" + txtRoomCharges.getText() + " where RoomNo='" + txtRoomNo.getText() + "'"; pst=con.prepareStatement(sql); pst.execute(); JOptionPane.showMessageDialog(this,"Successfully updated","Room Record",JOptionPane.INFORMATION_MESSAGE); btnUpdate.setEnabled(false); Get_Data(); }catch(HeadlessException | SQLException ex){ JOptionPane.showMessageDialog(this,ex); } } private void btnDeleteActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnDeleteActionPerformed try { int P = JOptionPane.showConfirmDialog(null," Are you sure want to delete ?","Confirmation",JOptionPane.YES_NO_OPTION); if (P==0) { con=Connect.ConnectDB(); String sql= "delete from Room where RoomNo = '" + txtRoomNo.getText() + "'"; pst=con.prepareStatement(sql); pst.execute(); JOptionPane.showMessageDialog(this,"Successfully deleted","Record",JOptionPane.INFORMATION_MESSAGE); Reset(); } }catch(HeadlessException | SQLException ex){ JOptionPane.showMessageDialog(this,ex); } }

6. Services Module
This module helps to add the service details in the hospital management system in java. Name the file Services.java
import java.awt.HeadlessException; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.text.DateFormat; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Locale; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.JOptionPane; import net.proteanit.sql.DbUtils; public class Services extends javax.swing.JFrame { Connection con=null; ResultSet rs=null; PreparedStatement pst=null; public Services() { initComponents(); setLocationRelativeTo(null); txtServiceID.setVisible(false); Get_Data1(); } private void Get_Data1(){ try{ con=Connect.ConnectDB(); String sql="select PatientID as 'Patient ID', PatientName as 'Patient Name' from Patientregistration order by PatientName"; pst=con.prepareStatement(sql); rs= pst.executeQuery(); tblPatient.setModel(DbUtils.resultSetToTableModel(rs)); }catch(Exception e){ JOptionPane.showMessageDialog(null, e); } } private void Reset() { txtPatientID.setText(""); txtServiceCharges.setText(""); txtPatientName.setText(""); txtServiceDate.setText(""); txtServiceName.setText(""); txtSave.setEnabled(true); txtUpdate.setEnabled(false); txtDelete.setEnabled(false); } @SuppressWarnings("unchecked") private void initComponents() { jPanel1 = new javax.swing.JPanel(); jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jLabel4 = new javax.swing.JLabel(); jLabel5 = new javax.swing.JLabel(); txtServiceName = new javax.swing.JTextField(); jLabel36 = new javax.swing.JLabel(); txtPatientID = new javax.swing.JTextField(); txtPatientName = new javax.swing.JTextField(); txtServiceCharges = new javax.swing.JTextField(); txtServiceDate = new javax.swing.JTextField(); jPanel2 = new javax.swing.JPanel(); txtNew = new javax.swing.JButton(); txtSave = new javax.swing.JButton(); txtDelete = new javax.swing.JButton(); txtUpdate = new javax.swing.JButton(); txtGetData = new javax.swing.JButton(); jScrollPane2 = new javax.swing.JScrollPane(); tblPatient = new javax.swing.JTable(); txtServiceID = new javax.swing.JTextField(); jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Services Info")); jLabel1.setText("Service Name"); jLabel2.setText("Service Date"); jLabel3.setText("Patient ID"); jLabel4.setText("Patient Name"); jLabel5.setText("Service Charges"); txtServiceName.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtServiceNameActionPerformed(evt); } }); jLabel36.setText("(YYYY-MM-DD)"); txtPatientID.setEditable(false); txtPatientName.setEditable(false); txtServiceCharges.addKeyListener(new java.awt.event.KeyAdapter() { public void keyTyped(java.awt.event.KeyEvent evt) { txtServiceChargesKeyTyped(evt); } }); txtServiceDate.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtServiceDateActionPerformed(evt); } }); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(36, 36, 36) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel1) .addComponent(jLabel2) .addComponent(jLabel3) .addComponent(jLabel4) .addComponent(jLabel5)) .addGap(24, 24, 24) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addComponent(txtServiceName) .addGroup(jPanel1Layout.createSequentialGroup() .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addComponent(txtPatientID, javax.swing.GroupLayout.DEFAULT_SIZE, 120, Short.MAX_VALUE) .addComponent(txtServiceDate)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(jLabel36)) .addComponent(txtPatientName)) .addComponent(txtServiceCharges, javax.swing.GroupLayout.PREFERRED_SIZE, 119, javax.swing.GroupLayout.PREFERRED_SIZE)) .addContainerGap(26, Short.MAX_VALUE)) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(20, 20, 20) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel1) .addComponent(txtServiceName, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel2) .addComponent(jLabel36) .addComponent(txtServiceDate, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel3) .addComponent(txtPatientID, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel4) .addComponent(txtPatientName, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel5) .addComponent(txtServiceCharges, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) ); jPanel2.setBorder(javax.swing.BorderFactory.createTitledBorder("")); txtNew.setText("New"); txtNew.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtNewActionPerformed(evt); } }); txtSave.setText("Save"); txtSave.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtSaveActionPerformed(evt); } }); txtDelete.setText("Delete"); txtDelete.setEnabled(false); txtDelete.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtDeleteActionPerformed(evt); } }); txtUpdate.setText("Update"); txtUpdate.setEnabled(false); txtUpdate.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtUpdateActionPerformed(evt); } }); txtGetData.setText("Get Data"); txtGetData.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtGetDataActionPerformed(evt); } }); }

Conclusion
In this project, we developed a GUI-based project, a Hospital Management System Project in Java and MySQL. The users are able to perform the operations such as Admin Login, Patient details, Doctor details, Room details, and Services. I hope you enjoyed doing this project!
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023
- Snake Game in Java