
In this article, we will build Electricity Bill Management System Project in Java and MySQL with source code. This project primarily focuses on calculating the number of units utilized by the customer and generating new Meter Information associated with a new customer.
Electricity Bill Management System Project in Java
Project Name: | Electricity Bill Management System Project in Java |
Abstract: | It’s a GUI-based project used with the Swing module to manage Electricity Management System in Java. |
Language: | Java |
IDE: | NetBeans IDE |
Java version (Recommended): | Java SE 18.0. 2.1 |
Database: | MySQL |
Type: | Desktop Application |
Recommended for: | Final Year Students |
Time Needed: | 3-4 hours |
Features
There are two aspects of this application – Admin and Customer.
- The admin can create a new customer with new meter information assigned to the customer, view all customer details, and calculate the bills of all the customers.
- The user can create his own account after the admin has created his meter information and once the account is created user can view his account information.
Setup the development environment
Open NetBeans IDE and create a new Java project with the name ElectricityManagementSystem. Now create two new packages with the name electricitymanagment and icon. Inside the electricitymanagment package, we will create all the Java files and inside the icon, we will keep all the images for the project.
Make sure you have MySQL server and Workbench installed on your system. If not then follow this.
Download these two jar files and images.
Now we need to add the dependencies of the project. Expand the project and right-click on libraries, click on add jar/folder and browse the two jar file you have downloaded from above and add them. You also need to keep all the images in the icon folder.
MySQL setup for Electricity Bill Management System in Java
Open the MySQL workbench and run the following command. These commands will create a new database and two tables with the name login and customer.
CREATE DATABASE ebs; USE ebs; create table login ( meter_no varchar(20), username varchar(30), name varchar(30), password varchar(20), user varchar(200) ); create table customer ( name varchar(20), meter_no varchar(20), address varchar(50), city varchar(30), state varchar(30), email varchar(40), phone varchar(20) );
Complete Code for Electricity Bill Management System Project in Java
1. Database Connection module
In this module, we create the conn.java class which is used to connect to MySQL Database and in all other java classes, we use the object of this class to perform all the operations on the database. In this file, you have to keep the credentials of your MySQL server account.
import java.sql.*; public class Conn { Connection c; Statement s; Conn() { try { c = DriverManager.getConnection("jdbc:mysql:///ebs", "root", "root"); // replace the password according to your account s = c.createStatement(); } catch (Exception e) { } } }
2. Main Screen Module
The Electricity Bill Management System starts from here. There is a progress bar on this screen and when the time is over the login module is called from here. Name it MainScreen.java.
import javax.swing.*; import java.awt.*; public class MainScreen { JFrame frame; ImageIcon i1 = new ImageIcon(ClassLoader.getSystemResource("icon/electricity.png")); Image i2 = i1.getImage(); ImageIcon i3 = new ImageIcon(i2); JLabel image=new JLabel(i3); JLabel text=new JLabel("Electricity Management System"); JProgressBar progressBar=new JProgressBar(); JLabel message=new JLabel(); MainScreen() { createGUI(); addImage(); addText(); addProgressBar(); runningPBar(); } public void createGUI(){ frame=new JFrame(); frame.getContentPane().setLayout(null); frame.setUndecorated(true); frame.setSize(600,600); frame.setLocationRelativeTo(null); frame.getContentPane().setBackground(new Color(0X78DEC7)); frame.setVisible(true); } public void addImage(){ image.setBounds(90, 70, 400, 200); frame.add(image); } public void addText() { text.setFont(new Font("MV Boli",Font.BOLD,22)); text.setBounds(120,300,400,50); text.setForeground(Color.black); frame.add(text); } public void addProgressBar(){ progressBar.setBounds(100,380,400,30); progressBar.setBorderPainted(true); progressBar.setStringPainted(true); progressBar.setBackground(Color.black); progressBar.setForeground(new Color(0XFF6464)); progressBar.setValue(0); frame.add(progressBar); } public void runningPBar(){ int i=0; while( i<=100) { try{ Thread.sleep(40); progressBar.setValue(i); i++; if(i==100) frame.dispose(); }catch(Exception e){ e.printStackTrace(); } } } public static void main(String[] args) { new MainScreen(); new Login(); } }

3. Login Module
This class of Electricity Bill Management System will help to create a login/register page so the user can enter the details and log in or go to the signup page to register. Name it Login.java.
import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.sql.*; public class Login extends JFrame implements ActionListener{ JButton login, cancel, signup; JTextField username, password; Choice logginin; Login() { super("Login Page"); getContentPane().setBackground(new Color(0X78DEC7)); setLayout(null); JLabel lblusername = new JLabel("Username"); lblusername.setBounds(350, 120, 100, 20); lblusername.setFont(new Font("MV BOli", Font.BOLD, 14)); add(lblusername); username = new JTextField(); username.setBounds(450, 120, 150, 20); add(username); JLabel lblpassword = new JLabel("Password"); lblpassword.setFont(new Font("MV BOli", Font.BOLD, 14)); lblpassword.setBounds(350, 160, 100, 20); add(lblpassword); password = new JTextField(); password.setBounds(450, 160, 150, 20); add(password); JLabel loggininas = new JLabel("Loggin in as"); loggininas.setFont(new Font("MV BOli", Font.BOLD, 14)); loggininas.setBounds(350, 200, 100, 20); add(loggininas); logginin = new Choice(); logginin.add("Admin"); logginin.add("Customer"); logginin.setBounds(450, 200, 150, 20); add(logginin); login = new JButton("Login"); login.setBounds(350, 260, 100, 40); login.setBackground(Color.orange); login.addActionListener(this); add(login); cancel = new JButton("Cancel"); cancel.setBounds(450, 350, 100, 40); cancel.setBackground(Color.orange); cancel.addActionListener(this); add(cancel); signup = new JButton("Signup"); signup.setBackground(Color.orange); signup.setBounds(550, 260, 100, 40); signup.addActionListener(this); add(signup); ImageIcon i7 = new ImageIcon(ClassLoader.getSystemResource("icon/secondd.png")); Image i8 = i7.getImage().getScaledInstance(150, 150, Image.SCALE_DEFAULT); ImageIcon i9 = new ImageIcon(i8); JLabel image = new JLabel(i9); image.setBounds(40, 70, 250, 250); add(image); setSize(840, 500); setLocation(200, 100); setVisible(true); } @Override public void actionPerformed(ActionEvent ae) { if (ae.getSource() == login) { String susername = username.getText(); String spassword = password.getText(); String user = logginin.getSelectedItem(); try { Conn c = new Conn(); String query = "select * from login where username = '"+susername+"' and password = '"+spassword+"' and user = '"+user+"'"; ResultSet rs = c.s.executeQuery(query); if (rs.next()) { String meter = rs.getString("meter_no"); setVisible(false); new Home(user, meter); } else { JOptionPane.showMessageDialog(null, "Invalid Login"); username.setText(""); password.setText(""); } } catch (Exception e) { } } else if (ae.getSource() == cancel) { setVisible(false); } else if (ae.getSource() == signup) { setVisible(false); new Signup(); } } public static void main(String[] args) { new Login(); } }

4. Signup Module
On this page of Electricity Bill Management System, the user can enter the details and create an account as a customer or an admin. Name it Signup.java.
import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.sql.*; public class Signup extends JFrame implements ActionListener{ JButton create; Choice accountType; JTextField meter, username, name, password; Signup(){ setBounds(450, 150, 700, 400); getContentPane().setBackground(new Color(0X78DEC7)); setLayout(null); JPanel panel = new JPanel(); panel.setBounds(30, 30, 650, 300); panel.setBackground(new Color(0X78DEC7)); panel.setLayout(null); add(panel); JLabel heading = new JLabel("Create Account As"); heading.setBounds(100, 50, 140, 20); heading.setForeground(Color.black); heading.setFont(new Font("MV Boli", Font.BOLD, 14)); panel.add(heading); accountType = new Choice(); accountType.add("Admin"); accountType.add("Customer"); accountType.setBounds(260, 50, 150, 20); panel.add(accountType); JLabel lblmeter = new JLabel("Meter Number"); lblmeter.setBounds(100, 90, 140, 20); lblmeter.setForeground(Color.black); lblmeter.setFont(new Font("MV BOli", Font.BOLD, 14)); lblmeter.setVisible(false); panel.add(lblmeter); meter = new JTextField(); meter.setBounds(260, 90, 150, 20); meter.setVisible(false); panel.add(meter); JLabel lblusername = new JLabel("Username"); lblusername.setBounds(100, 130, 140, 20); lblusername.setForeground(Color.black); lblusername.setFont(new Font("MV Boli", Font.BOLD, 14)); panel.add(lblusername); username = new JTextField(); username.setBounds(260, 130, 150, 20); panel.add(username); JLabel lblname = new JLabel("Name"); lblname.setBounds(100, 170, 140, 20); lblname.setForeground(Color.black); lblname.setFont(new Font("MV Boli", Font.BOLD, 14)); panel.add(lblname); name = new JTextField(); name.setBounds(260, 170, 150, 20); panel.add(name); meter.addFocusListener(new FocusListener() { public void focusGained(FocusEvent fe) {} public void focusLost(FocusEvent fe) { try { Conn c = new Conn(); ResultSet rs = c.s.executeQuery("select * from login where meter_no = '"+meter.getText()+"'"); while(rs.next()) { name.setText(rs.getString("name")); } } catch (Exception e) { e.printStackTrace(); } } }); JLabel lblpassword = new JLabel("Password"); lblpassword.setBounds(100, 210, 140, 20); lblpassword.setForeground(Color.black); lblpassword.setFont(new Font("MV Boli", Font.BOLD, 14)); panel.add(lblpassword); password = new JTextField(); password.setBounds(260, 210, 150, 20); panel.add(password); accountType.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent ae) { String user = accountType.getSelectedItem(); if (user.equals("Customer")) { lblmeter.setVisible(true); meter.setVisible(true); name.setEditable(false); } else { lblmeter.setVisible(false); meter.setVisible(false); name.setEditable(true); } } }); create = new JButton("Create"); create.setBackground(Color.black); create.setForeground(Color.white); create.setBounds(260, 260, 120, 25); create.addActionListener(this); panel.add(create); setVisible(true); } public void actionPerformed(ActionEvent ae) { if (ae.getSource() == create) { String atype = accountType.getSelectedItem(); String susername = username.getText(); String sname = name.getText(); String spassword = password.getText(); String smeter = meter.getText(); try { Conn c = new Conn(); String query = null; if (atype.equals("Admin")) { query = "insert into login values('"+smeter+"', '"+susername+"', '"+sname+"', '"+spassword+"', '"+atype+"')"; } else { query = "update login set username = '"+susername+"', password = '"+spassword+"', user = '"+atype+"' where meter_no = '"+smeter+"'"; } c.s.executeUpdate(query); JOptionPane.showMessageDialog(null, "Successfull"); setVisible(false); new Login(); } catch (Exception e) { } } } public static void main(String[] args) { new Signup(); } }
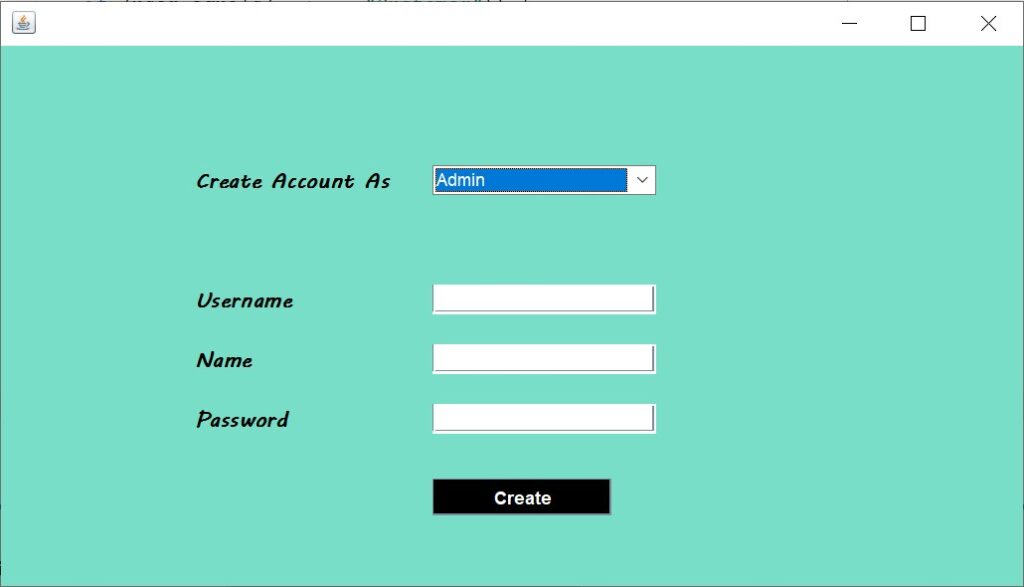
5. Home Module
After the user is logged in, he will be redirected to this screen where a bunch of options is available according to the user type i.e admin or customer. Name it Home.java.
import javax.swing.*; import java.awt.*; import java.awt.event.*; public class Home extends JFrame implements ActionListener{ String atype, meter; Home(String atype, String meter) { this.atype = atype; this.meter = meter; setLayout(null); setBounds(300, 100, 700, 600); setResizable(false); ImageIcon i1 = new ImageIcon(ClassLoader.getSystemResource("icon/elec1.png")); Image i2 = i1.getImage().getScaledInstance(700, 600, Image.SCALE_DEFAULT); ImageIcon i3 = new ImageIcon(i2); JLabel image = new JLabel(i3); add(image); JMenuBar mb = new JMenuBar(); mb.setBackground(new Color(0XFF5757)); setJMenuBar(mb); JMenu master = new JMenu("Administrator"); master.setFont(new Font("MV Boli", Font.BOLD, 25)); master.setForeground(Color.black); JMenuItem newcustomer = new JMenuItem("New Customer"); newcustomer.setFont(new Font("MV Boli", Font.BOLD, 15)); newcustomer.setBackground(Color.WHITE); newcustomer.setBorder(BorderFactory.createLineBorder(Color.BLACK, 2)); newcustomer.setPreferredSize(new Dimension(170, 50)); newcustomer.addActionListener(this); master.add(newcustomer); JMenuItem customerdetails = new JMenuItem("Customer Details"); customerdetails.setFont(new Font("MV Boli", Font.BOLD, 15)); customerdetails.setBackground(Color.WHITE); customerdetails.setBorder(BorderFactory.createLineBorder(Color.BLACK, 2)); customerdetails.setPreferredSize(new Dimension(170, 50)); customerdetails.addActionListener(this); master.add(customerdetails); JMenuItem calculatebill = new JMenuItem("Calculate Bill"); calculatebill.setFont(new Font("MV Boli", Font.BOLD, 15)); calculatebill.setBackground(Color.WHITE); calculatebill.setBorder(BorderFactory.createLineBorder(Color.BLACK, 2)); calculatebill.setPreferredSize(new Dimension(170, 50)); calculatebill.addActionListener(this); master.add(calculatebill); JMenu info = new JMenu("Information"); info.setFont(new Font("MV Boli", Font.BOLD, 25)); info.setForeground(Color.black); JMenuItem viewinformation = new JMenuItem("View Information"); viewinformation.setFont(new Font("MV Boli", Font.BOLD, 15)); viewinformation.setBackground(Color.WHITE); viewinformation.setBorder(BorderFactory.createLineBorder(Color.BLACK, 2)); viewinformation.setPreferredSize(new Dimension(170, 50)); viewinformation.addActionListener(this); info.add(viewinformation); if (atype.equals("Admin")) { mb.add(master); } else { mb.add(info); } setLayout(new FlowLayout()); setVisible(true); } public void actionPerformed(ActionEvent ae) { String msg = ae.getActionCommand(); if (msg.equals("New Customer")) { new NewCustomer(); } else if (msg.equals("Customer Details")) { new CustomerDetails(); } else if (msg.equals("Calculate Bill")) { new CalculateBill(); } else if (msg.equals("View Information")) { new ViewInformation(meter); } } public static void main(String[] args) { new Home("", ""); } }

6. New Customer Module
This java class of Electricity Bill Management System Project in Java will be responsible for creating a new customer in the database from the admin account. Name it NewCustomer.java.
import javax.swing.*; import java.awt.*; import java.util.*; import java.awt.event.*; public class NewCustomer extends JFrame implements ActionListener{ JTextField tfname, tfaddress, tfstate, tfcity, tfemail, tfphone; JButton next; JLabel lblmeter; NewCustomer() { setSize(700, 500); setLocation(400, 100); JPanel p = new JPanel(); p.setLayout(null); p.setBackground(new Color(0X78DEC7)); add(p); JLabel heading = new JLabel("New Customer"); heading.setBounds(180, 10, 200, 25); heading.setFont(new Font("Tahoma", Font.PLAIN, 24)); p.add(heading); JLabel lblname = new JLabel("Customer Name"); lblname.setBounds(100, 80, 100, 20); p.add(lblname); tfname = new JTextField(); tfname.setBounds(240, 80, 200, 20); p.add(tfname); JLabel lblmeterno = new JLabel("Meter Number"); lblmeterno.setBounds(100, 120, 100, 20); p.add(lblmeterno); lblmeter = new JLabel(""); lblmeter.setBounds(240, 120, 100, 20); p.add(lblmeter); Random ran = new Random(); long number = ran.nextLong() % 1000000; lblmeter.setText("" + Math.abs(number)); JLabel lbladdress = new JLabel("Address"); lbladdress.setBounds(100, 160, 100, 20); p.add(lbladdress); tfaddress = new JTextField(); tfaddress.setBounds(240, 160, 200, 20); p.add(tfaddress); JLabel lblcity = new JLabel("City"); lblcity.setBounds(100, 200, 100, 20); p.add(lblcity); tfcity = new JTextField(); tfcity.setBounds(240, 200, 200, 20); p.add(tfcity); JLabel lblstate = new JLabel("State"); lblstate.setBounds(100, 240, 100, 20); p.add(lblstate); tfstate = new JTextField(); tfstate.setBounds(240, 240, 200, 20); p.add(tfstate); JLabel lblemail = new JLabel("Email"); lblemail.setBounds(100, 280, 100, 20); p.add(lblemail); tfemail = new JTextField(); tfemail.setBounds(240, 280, 200, 20); p.add(tfemail); JLabel lblphone = new JLabel("Phone Number"); lblphone.setBounds(100, 320, 100, 20); p.add(lblphone); tfphone = new JTextField(); tfphone.setBounds(240, 320, 200, 20); p.add(tfphone); next = new JButton("Submit"); next.setBounds(250, 390, 100,25); next.setBackground(Color.BLACK); next.setForeground(Color.WHITE); next.addActionListener(this); p.add(next); setLayout(new BorderLayout()); add(p, "Center"); ImageIcon i1 = new ImageIcon(ClassLoader.getSystemResource("icon/new-cust.png")); Image i2 = i1.getImage().getScaledInstance(150, 150, Image.SCALE_DEFAULT); ImageIcon i3 = new ImageIcon(i2); JLabel image = new JLabel(i3); add(image, "East"); getContentPane().setBackground(new Color(0X78DEC7)); setVisible(true); } public void actionPerformed(ActionEvent ae) { if (ae.getSource() == next) { String name = tfname.getText(); String meter = lblmeter.getText(); String address = tfaddress.getText(); String city = tfcity.getText(); String state = tfstate.getText(); String email = tfemail.getText(); String phone = tfphone.getText(); String query1 = "insert into customer values('"+name+"', '"+meter+"', '"+address+"', '"+city+"', '"+state+"', '"+email+"', '"+phone+"')"; String query2 = "insert into login values('"+meter+"', '', '"+name+"', '', '')"; try { Conn c = new Conn(); c.s.executeUpdate(query1); c.s.executeUpdate(query2); JOptionPane.showMessageDialog(null, "Successful"); setVisible(false); } catch (Exception e) { } } } public static void main(String[] args) { new NewCustomer(); } }

7. Customer Details Module
This class is responsible for showing the details of all the customers in the admin account. Name it CustomerDetails.java.
import java.awt.*; import javax.swing.*; import java.sql.*; import net.proteanit.sql.DbUtils; import java.awt.event.*; import java.awt.print.PrinterException; public class CustomerDetails extends JFrame implements ActionListener{ JTable table; JButton print; CustomerDetails(){ super("Customer Details"); setSize(700, 500); setLocation(200, 150); table = new JTable(); table.setBackground(new Color(0X78DEC7)); try { Conn c = new Conn(); ResultSet rs = c.s.executeQuery("select * from customer"); table.setModel(DbUtils.resultSetToTableModel(rs)); } catch (Exception e) { } JScrollPane sp = new JScrollPane(table); add(sp); print = new JButton("Print"); print.addActionListener(this); print.setBackground(new Color(0X78DEC7)); add(print, "South"); setVisible(true); } public void actionPerformed(ActionEvent ae) { try { table.print(); } catch (PrinterException e) { } } public static void main(String[] args) { new CustomerDetails(); } }

8. Calculate Bill Module
This java class of Electricity Bill Management System Project in Java will be responsible for showing the calculate bill option to the admin. The admin can update the bill of all the users from this page. Name it CalculateBill.java.
import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.sql.*; public class CalculateBill extends JFrame implements ActionListener{ JTextField tfunits; JButton next; JLabel lblname, labeladdress; Choice meternumber, cmonth; CalculateBill() { setSize(600, 500); setLocation(400, 150); JPanel p = new JPanel(); p.setLayout(null); p.setBackground(new Color(0X78DEC7)); add(p); JLabel heading = new JLabel("Calculate Electricity Bill"); heading.setBounds(150, 10, 400, 25); heading.setFont(new Font("MV Boli", Font.BOLD, 25)); p.add(heading); JLabel lblmeternumber = new JLabel("Meter Number"); lblmeternumber.setBounds(100, 80, 100, 20); p.add(lblmeternumber); meternumber = new Choice(); try { Conn c = new Conn(); ResultSet rs = c.s.executeQuery("select * from customer"); while(rs.next()) { meternumber.add(rs.getString("meter_no")); } } catch (Exception e) { } meternumber.setBounds(240, 80, 200, 20); p.add(meternumber); JLabel lblmeterno = new JLabel("Name"); lblmeterno.setBounds(100, 120, 100, 20); p.add(lblmeterno); lblname = new JLabel(""); lblname.setBounds(240, 120, 100, 20); p.add(lblname); JLabel lbladdress = new JLabel("Address"); lbladdress.setBounds(100, 160, 100, 20); p.add(lbladdress); labeladdress = new JLabel(); labeladdress.setBounds(240, 160, 200, 20); p.add(labeladdress); try { Conn c = new Conn(); ResultSet rs = c.s.executeQuery("select * from customer where meter_no = '"+meternumber.getSelectedItem()+"'"); while(rs.next()) { lblname.setText(rs.getString("name")); labeladdress.setText(rs.getString("address")); } } catch (Exception e) { } meternumber.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent ie) { try { Conn c = new Conn(); ResultSet rs = c.s.executeQuery("select * from customer where meter_no = '"+meternumber.getSelectedItem()+"'"); while(rs.next()) { lblname.setText(rs.getString("name")); labeladdress.setText(rs.getString("address")); } } catch (Exception e) { } } }); JLabel lblcity = new JLabel("Units Consumed"); lblcity.setBounds(100, 200, 100, 20); p.add(lblcity); tfunits = new JTextField(); tfunits.setBounds(240, 200, 200, 20); p.add(tfunits); JLabel lblstate = new JLabel("Month"); lblstate.setBounds(100, 240, 100, 20); p.add(lblstate); cmonth = new Choice(); cmonth.setBounds(240, 240, 200, 20); cmonth.add("January"); cmonth.add("February"); cmonth.add("March"); p.add(cmonth); next = new JButton("Submit"); next.setBounds(250, 350, 100,25); next.setBackground(Color.BLACK); next.setForeground(Color.WHITE); next.addActionListener(this); p.add(next); setLayout(new BorderLayout()); add(p, "Center"); getContentPane().setBackground(new Color(0X78DEC7)); setVisible(true); } public void actionPerformed(ActionEvent ae) { JOptionPane.showMessageDialog(null, "Customer Bill Updated Successfully"); } public static void main(String[] args) { new CalculateBill(); } }

9. View Information Module
import javax.swing.*; import java.awt.*; import java.sql.*; public class ViewInformation extends JFrame{ ViewInformation(String meter) { setBounds(350, 150, 500, 300); getContentPane().setBackground(new Color(0X78DEC7)); setLayout(null); JLabel heading = new JLabel("CUSTOMER INFORMATION"); heading.setBounds(150, 20, 500, 40); heading.setFont(new Font("MV Boli", Font.BOLD, 15)); add(heading); JLabel lblname = new JLabel("Name:"); lblname.setFont(new Font("MV Boli", Font.BOLD, 15)); lblname.setBounds(100, 60, 100, 40); add(lblname); JLabel name = new JLabel(""); name.setFont(new Font("MV Boli", Font.BOLD, 15)); name.setBounds(250, 60, 100, 40); add(name); JLabel lblmeternumber = new JLabel("Meter No:"); lblmeternumber.setFont(new Font("MV Boli", Font.BOLD, 15)); lblmeternumber.setBounds(70, 130, 100, 40); add(lblmeternumber); JLabel meternumber = new JLabel(""); meternumber.setFont(new Font("MV Boli", Font.BOLD, 20)); meternumber.setBounds(250, 130, 100, 40); add(meternumber); try { Conn c = new Conn(); ResultSet rs = c.s.executeQuery("select * from customer where meter_no = '"+meter+"'"); while(rs.next()) { name.setText(rs.getString("name")); meternumber.setText(rs.getString("meter_no")); } } catch (Exception e) { } setVisible(true); } public static void main(String[] args) { new ViewInformation(""); } }

Output for Electricity Bill Management System Project in Java:
Video Output for Admin Side
Video Output for Customer Side
Conclusion
We have built a GUI-based project for Electricity Bill Management System Project in Java with MySQL. I hope I included most of the required functions and that this management app can be used in the real world as well but if not then I recommend you must add the functionalities you may want/require.
Thank you for visiting our website.
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023
- Snake Game in Java