
Introduction
In this article, we will build Restaurant Management System Project in Java and MySQL with source code. This project is great for those at an intermediate level in Java who want to advance their coding skills. In this project, the users will be able to perform the following functionalities Login, Add food items, update food, delete food, and generate bills. Let’s get started!
Restaurant Management System Project in Java: Project Overview
Project Name: | Restaurant Management System Project in Java |
Abstract | It’s a GUI-based project with the Swing module to organize all the elements under restaurant management. |
Language/s Used: | Java |
IDE | IntelliJ Idea Professional and Eclipse(Recommended) |
Java version (Recommended): | Java SE 18.0. 2.1 |
Database: | MySQL |
Type: | Desktop Application |
Recommended for | Final Year Students |
Setting the development environment
You must have java JDK installed on your system and we are using Eclipse IDE to build this project, you can use either this or Netbeans IDE.
The first step will be to create a new project. Name it as you wish. In the src folder create a restaurantmanagement package. In that package, we will be creating some files for different modules.
To connect the system with the database you will need to follow specific steps.
- Have Java JDK already installed and an IDE like Eclipse
- Install MySQL on the system.
- Download the MySQL connector from here.
- In Eclipse, under your project expand external libraries and right-click, and select Open restaurantmanagement Settings. Select the libraries tab and click on the + button. Browse your jar file downloaded from the above step and click on it. This will add a dependency to your project. The steps will differ if you are using a different IDE.
MySql Setup for Restaurant Management System Project in Java
1. Create a database
-- Creating database
create database restaurant;
2. Select the database
-- Selecting the database
use restaurant;
3. Create a food table
-- Creating food table
create table food (
id int primary key,
food_name varchar(25) not null,
price int not null,
quantity int
);
4. Create an admin table
-- Create admin table
create table admin (
login_id varchar(25),
password varchar(25)
);
5. Insert some values in the admin table
-- Inserting value in admin table
insert into admin values
("admin","admin")
Implementation of the Restaurant Management System Project in Java
1. Login module
This module helps to make a login page so the user can enter the user name and password to the restaurant management system in java. Name the file login.java
import java.awt.*; import java.awt.FlowLayout; import java.awt.GridLayout; import javax.swing.*; import javax.swing.colorchooser.AbstractColorChooserPanel; public class Frame1 extends JFrame{ JLabel idLabel; JLabel passLabel; JLabel background; JLabel headerLabel; JLabel devInfo; JTextField id; JPasswordField pass; JButton submit; public Frame1(){ setTitle("Restaurant Management System"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true) this.background = new JLabel(new ImageIcon("restaurant.jpg")); this.init(); add(background); background.setVisible(true); this.pack(); this.setSize(700,400); this.setLocationRelativeTo(null); } public void init(){ headerLabel = new JLabel(); this.headerLabel.setText("Restaurant Management System"); this.headerLabel.setBounds(270,1,200,100); this.headerLabel.setFont(new Font("Geomanist", Font.BOLD, 25)); headerLabel.setForeground(Color.white); add(headerLabel); idLabel = new JLabel(); this.idLabel.setText("Username"); this.idLabel.setBounds(190,110,100,50); this.idLabel.setFont(new Font(null, Font.BOLD, 20)); idLabel.setForeground(Color.white); add(idLabel); passLabel=new JLabel("Password"); this.passLabel.setBounds(190,165,100,50); this.passLabel.setFont(new Font(null, Font.BOLD, 20)); passLabel.setForeground(Color.white); add(passLabel); devInfo = new JLabel(); this.devInfo.setBounds(130,300,1000,30); this.devInfo.setFont(new Font("Geomanist", Font.PLAIN, 15)); devInfo.setForeground(Color.white); add(devInfo); id=new JTextField(); this.id.setBounds(300,125,200,30); add(id); pass=new JPasswordField(); this.add(pass); this.pass.setBounds(300,175,200,30); this.id.setVisible(true); this.submit=new JButton("Login"); this.submit.setBounds(400,230,100,25); add(submit); submit.addActionListener(this::submitActionPerformed); } public void submitActionPerformed(java.awt.event.ActionEvent evt){ if(id.getText().equals("admin") && pass.getText().equals("admin")){ this.hide(); Frame2new fn=new Frame2new(); fn.showButtonDemo(); } else{ JOptionPane.showMessageDialog(null, "Invalid password!"); } } } class MyGui{ public static void main(String[] a){ Frame1 f = new Frame1(); f.setVisible(true); } }
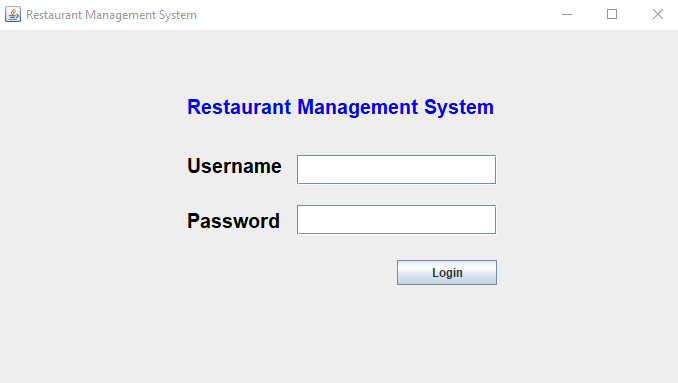
2. Home Module
Once we enter the correct username and password on the login page, it takes us to the home page of our restaurant management application. Name the file home.java
import java.awt.*; import java.awt.event.*; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.*; import java.awt.Color; public class Home { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; public Home(){ prepareGUI(); } public static void main(String[] args){ Frame2new swingControlDemo = new Frame2new(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Restaurant Management System"); mainFrame.setBounds(100,100,700,400); mainFrame.setLayout(new GridLayout(3,1)); mainFrame.getContentPane().setBackground(Color.pink); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new JLabel("", JLabel.CENTER); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,300); controlPanel = new JPanel(); controlPanel.setLayout(new GridLayout(1,5)); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Restaurant Management System"); this.headerLabel.setFont(new Font(null, Font.BOLD, 27)); headerLabel.setForeground(Color.white); JButton fkButton = new JButton("Food Info"); JButton billButton = new JButton("Billing Info"); JButton afButton = new JButton("Insert Item"); JButton ufButton = new JButton("Update Item"); //DELETE BUTTON JButton dlButton = new JButton("Delete Item"); fkButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { ItemInfo ii=new ItemInfo(); try { ii.showButtonDemo(); } catch (SQLException ex) { Logger.getLogger(Frame2new.class.getName()).log(Level.SEVERE, null, ex); } } }); billButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { GenerateBill gb=new GenerateBill();} }); afButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { EnterFood ef=new EnterFood(); ef.showButtonDemo(); } }); ufButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { UpdateFood uf=new UpdateFood(); uf.showButtonDemo(); } }); //delete button actionlistener dlButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { DeleteFood dl=new DeleteFood(); dl.showButtonDemo(); } }); controlPanel.add(ufButton); controlPanel.add(afButton); controlPanel.add(billButton); controlPanel.add(fkButton); controlPanel.add(dlButton); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } }

3. Add food module
This module helps to add a new food item in the restaurant management system in java. Name the file addFood.java
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class EnterFood { private JFrame mainFrame; private JLabel headerLabel; private JPanel controlPanel; private JLabel id,name,price,quantity; private static int count = 0; GridLayout experimentLayout = new GridLayout(0,2); ResultSet rs; EnterFood(){ prepareGUI(); } public static void main(String[] args){ EnterFood swingControlDemo = new EnterFood(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Insert a new food item!"); mainFrame.setSize(700,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.getContentPane().setBackground(Color.pink); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ mainFrame.setVisible(false); } }); headerLabel = new JLabel("", JLabel.CENTER); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Restaurant Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 27)); name = new JLabel("Enter Name"); JTextField tf2=new JTextField(); tf2.setSize(100,40); price = new JLabel("Enter Price"); JTextField tf3=new JTextField(); tf3.setSize(100,40); quantity = new JLabel("Enter Quantity"); JTextField tf4=new JTextField(); tf4.setSize(100,40); JButton okButton = new JButton("OK"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("insert into canteenmanagement.food(f_name,f_prize,f_quantity) values (?,?,?)"); pst.setString(1, tf2.getText()); pst.setDouble(2, Double.parseDouble(tf3.getText())); pst.setInt(3, Integer.parseInt(tf4.getText())); pst.execute(); JOptionPane.showMessageDialog(null, "Done Inserting " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("EEEE"); JOptionPane.showMessageDialog(null, "Inserting Error : " + tf2.getText()); }finally{ } } }); JPanel jp = new JPanel(null); jp.add(name); jp.add(tf2); jp.add(price); jp.add(tf3); jp.add(quantity); jp.add(tf4); jp.setSize(500,500); jp.setLayout(experimentLayout); controlPanel.add(jp); jp.add(okButton); mainFrame.setLocationRelativeTo(null); mainFrame.setVisible(true); } }
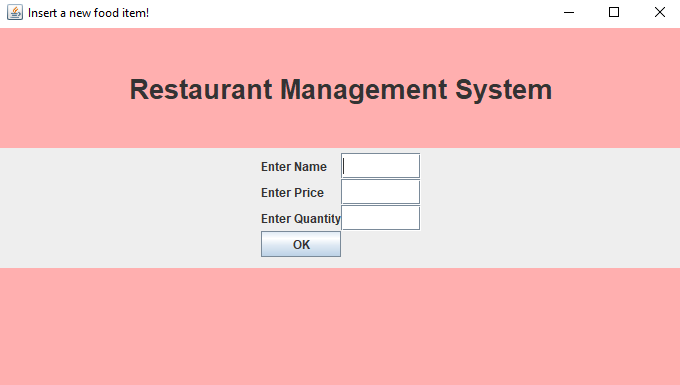
4. Food info Module
This module helps to view the food item in the restaurant management system in java. Name the file FoodInfo.java
import java.awt.*; import java.awt.event.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import javax.swing.*; public class ItemInfo { private JFrame mainFrame; private JLabel headerLabel; //private JLabel statusLabel; private JPanel controlPanel; ItemInfo (){ prepareGUI(); } public static void main(String[] args) throws SQLException{ ItemInfo swingControlDemo = new ItemInfo(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Showing all items"); mainFrame.setSize(700,400); mainFrame.getContentPane().setBackground(Color.pink); // mainFrame.setLayout(new GridLayout(4, 1)); mainFrame.setLayout(new FlowLayout()); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ //System.exit(0); } }); headerLabel = new JLabel("", JLabel.CENTER); // statusLabel = new JLabel("",JLabel.CENTER); // statusLabel.setSize(450,200); controlPanel = new JPanel(); controlPanel.setSize(700,400); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); //mainFrame.add(statusLabel); mainFrame.setVisible(true); } public void showButtonDemo() throws SQLException{ headerLabel.setText("Restaurant Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 25)); String[] columnNames = {"ID","Food Name", "Price", "Quantity" }; Object[][] data = new Object[100][4]; PreparedStatement pst; ResultSet rs; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("select * from canteenmanagement.food"); rs = pst.executeQuery(); int i=0; while(rs.next()){ data[i][0] = rs.getInt("f_id"); data[i][1] = rs.getString("f_name"); data[i][2] = rs.getDouble("f_prize"); data[i][3] = rs.getInt("f_quantity"); i++; } mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("Error"); JOptionPane.showMessageDialog(null, "Error !"); } JTable table = new JTable(data, columnNames); table.setSize(400, 400); table.setVisible(true); // table.setEnabled(false); controlPanel.add(new JScrollPane(table, JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER)); //controlPanel.add(table); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } }

5. Update the food Module
This module helps to update the food info in the restaurant management system in java. Name the file updateFood.java
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class UpdateFood { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; private JLabel id,name,price,quantity; private static int count = 0; GridLayout experimentLayout = new GridLayout(0,2); ResultSet rs; UpdateFood(){ prepareGUI(); } public static void main(String[] args){ UpdateFood swingControlDemo = new UpdateFood(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Update!"); mainFrame.setSize(700,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.getContentPane().setBackground(Color.pink); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ mainFrame.setVisible(false); } }); headerLabel = new JLabel("", JLabel.CENTER); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,400); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Restaurant Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 27)); name = new JLabel("Enter Name"); JTextField tf2=new JTextField(); tf2.setSize(100,30); price = new JLabel("Enter Price"); JTextField tf3=new JTextField(); tf3.setSize(100,30); quantity = new JLabel("Enter Quantity"); JTextField tf4=new JTextField(); tf4.setSize(100,30); JButton okButton = new JButton("UPDATE"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("UPDATE canteenmanagement.food SET f_quantity= ?, f_prize=? where f_name = ?"); pst.setString(3, tf2.getText()); pst.setDouble(2, Double.parseDouble(tf3.getText())); pst.setInt(1, Integer.parseInt(tf4.getText())); pst.execute(); JOptionPane.showMessageDialog(null, "Done Updating " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("Error"); JOptionPane.showMessageDialog(null, "Inserting Error : " + tf2.getText()); }finally{ } } }); JPanel jp = new JPanel(); jp.add(name); jp.add(tf2); jp.add(price); jp.add(tf3); jp.add(quantity); jp.add(tf4); jp.setSize(200,200); jp.setLayout(experimentLayout); controlPanel.add(jp); jp.add(okButton); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } }

6. Delete the food Module
This module helps to delete the food item in the restaurant management system in java. Name the file deleteFood.java
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class DeleteFood { private JFrame mainFrame; private JLabel headerLabel; private JPanel controlPanel; private JLabel id,name,price,quantity; private static int count = 0; GridLayout experimentLayout = new GridLayout(1,1); ResultSet rs; DeleteFood(){ prepareGUI(); } public static void main(String[] args){ UpdateFood swingControlDemo = new UpdateFood(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Delete!"); mainFrame.setSize(700,400); mainFrame.getContentPane().setBackground(Color.pink); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ mainFrame.setVisible(false); } }); headerLabel = new JLabel("", JLabel.CENTER); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Restaurant Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 27)); headerLabel.setForeground(Color.white); name = new JLabel("Enter Name"); JTextField tf2=new JTextField(); tf2.setSize(100,30); JButton okButton = new JButton("DELETE"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("DELETE FROM canteenmanagement.food where f_name = ?"); pst.setString(1,tf2.getText()); pst.execute(); JOptionPane.showMessageDialog(null, "Item Deleted " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("EEEE"); JOptionPane.showMessageDialog(null, "Item not found : " + tf2.getText()); }finally{ } } }); JPanel jp = new JPanel(); jp.add(name); jp.add(tf2); jp.setSize(700,400); jp.setLayout(experimentLayout); controlPanel.add(jp); jp.add(okButton); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } }

7. Generate bill Module
This module helps to generate bills in the restaurant management system in java. Name the file login.java
import static com.sun.java.accessibility.util.AWTEventMonitor.addActionListener; import java.awt.*; import java.awt.event.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.ArrayList; import javax.swing.*; import javax.swing.table.DefaultTableModel; public class GenerateBill extends JFrame{ JTextField food,quantity; String[] columnNames = {"Food Name", "Quantity", "Price" }; JTable cart; // JLabel totalP = new JLabel("TOTAL PRICE : 0.0tk"); JLabel totalP = new JLabel(); Object data[][] = new Object[100][3]; int i = 0; double totalprice = 0; ArrayList<foodCart> foodList = new ArrayList<>(); GenerateBill(){ JPanel jp1 = new JPanel(); setBackground(Color.red); //jp1.getContentPane().setBackground(Color.orange); this.setLayout(new GridLayout(2,2)); JLabel a = new JLabel("Food Name : "); jp1.add(a); food = new JTextField(50); jp1.add(food); JLabel b = new JLabel("Quantity : "); jp1.add(b); quantity = new JTextField(50); jp1.add(quantity); JButton ok = new JButton("OK"); JPanel jp2 = new JPanel(); jp2.setSize(700, 400); jp1.add(ok); ok.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); ResultSet rs; try{ pst = con.mkDataBase().prepareStatement("select f_prize from canteenmanagement.food where f_name = ?"); pst.setString(1, food.getText()); rs = pst.executeQuery(); while (rs.next()){ foodCart f = new foodCart(); f.name = food.getText(); f.quantity = Integer.parseInt(quantity.getText()); f.totalPer = f.quantity*rs.getDouble("f_prize"); totalprice += f.quantity*rs.getDouble("f_prize"); foodList.add(f); data[i][0] = f.name; data[i][1] = Integer.parseInt(quantity.getText()); data[i][2] = f.quantity*rs.getDouble("f_prize"); i++; food.setText(""); quantity.setText(""); DefaultTableModel model = (DefaultTableModel) cart.getModel(); model.setRowCount(0); cart = new JTable(data, columnNames); System.out.println(totalprice); removeAll(); //Total price not refreshing // totalP.setText("TOTAL Price : " + Double.toString(totalprice) + "tk"); totalP.revalidate(); totalP.repaint(); revalidate(); repaint(); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } catch(Exception ex){ System.out.println(ex); } } }); cart = new JTable(data, columnNames); cart.setSize(300, 450); //cart.setEnabled(false); // jp2.setLayout(new GridLayout(1,1)); jp2.setLayout(new FlowLayout()); jp2.add(totalP); jp2.add(new JScrollPane(cart, JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER)); JButton checkOut = new JButton("CheckOut"); checkOut.setSize(40, 50); jp2.add(checkOut); checkOut.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { int count = 1; for(foodCart fc : foodList){ System.out.println(count + ": Food Name : " + fc.name + " Quantity : "+ fc.quantity + " Price : " + fc.totalPer + "tk"); } double vat = 15; System.out.println("Total Cost : " + (totalprice+totalprice*vat) + "tk"); JOptionPane.showMessageDialog(null, "Total Cost : " + (totalprice+totalprice*vat/100) + "tk with vat " + vat+"%"); hide(); } }); this.add(jp1); this.add(jp2); this.setSize(600,550); this.setLocationRelativeTo(null); this.setVisible(true); } class foodCart{ String name; Double totalPer; int quantity; } }
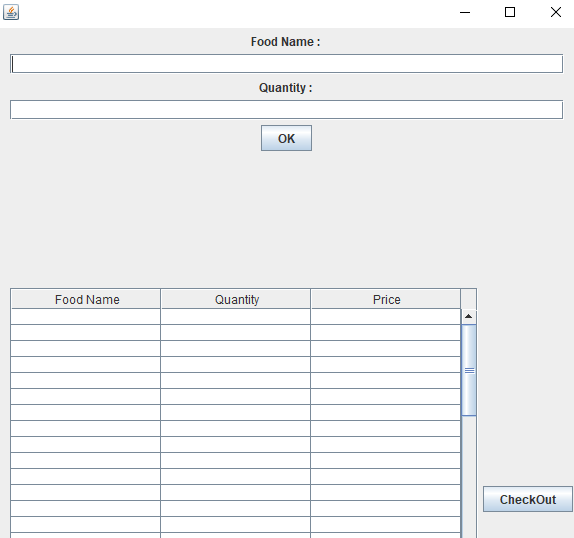
Conclusion
In this project, we developed a GUI-based project, a Restaurant Management System Project in Java and MySQL. The users are able to perform the operations such as Login, Adding food items, updating food, deleting food, and generating bills. I hope you enjoyed doing this project!
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023
- Snake Game in Java