
In this article, we will learn to create a project on survey management system in Java. There are two actors: The user who can add questions and surveys and view the survey results and the guest user who can answer those surveys.
Survey Management System In Java: Project Overview
Project Name: | Survey Management System in Java |
Abstract: | It’s a GUI-based project with the Swing module to create, manage and answer surveys. |
Language/s Used: | Java |
IDE: | Eclipse IDE for Java Developers |
Java version (Recommended): | Java SE 18.0. 2.1 |
Database: | MySQL |
Type: | Desktop Application |
Recommended for: | Final Year Students |
Setting Up the environment
Make sure JDK is present in your system. It can be installed using the IDE you are using.
The first step is to create a project in the name you wish. Create a package named “survey“. We are creating java classes in this package.
To connect the project to the database, follow the steps:
- Make sure MySQL is installed on your system.
- Download the MySQL connector from here.
- Open the properties of the project from the dropdown list which appears on right-click on the project. Navigate to the libraries tab and click on the classpath. Select “Add External JARs…”. Select the jar file from the file you downloaded after extracting it. This step will differ on IDE you are using.
MySQL Setup for Survey Management System Project In Java
1. Create the database
CREATE DATABASE survey;
2. Use the database
USE survey;
3. Create necessary tables
--Actor table
CREATE TABLE actors(id int primary key auto_increment, fname varchar(50), uname varchar(50), pass varchar(50));
--User Question Table
CREATE TABLE userQuestions(id int, surveycode varchar(5), total int);
--Questions table
CREATE TABLE questions(surveycode varchar(5), question varchar(255), option1 varchar(255), option2 varchar(255), option3 varchar(255), option4 varchar(255));
--Survey Answer table
CREATE TABLE surveyquestions(surveycode varchar(5), qno int, opno int);
Coding Survey Management System in Java
1. Main Module
File Name: “Main.java“
This class contains the main function where the program starts to execute.
package survey; import java.sql.SQLException; public class Main { public static void main(String args[]) throws SQLException { Login login = new Login(); login.loginView(); } }
2. Login Module
File Name: “Login.java“
This class contains the frame for the login page. Option for a signup and guest login is also present.
package survey; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.SQLException; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPasswordField; import javax.swing.JTextField; public class Login { int id; public void loginView() throws SQLException { SQLManage manage = new SQLManage(); JFrame frame = new JFrame(); frame.setSize(450, 450); frame.setLayout(null); frame.setLocationRelativeTo(null); JLabel heading = new JLabel("SURVEY SYSTEM"); heading.setBounds(0, 50, 450, 50); heading.setHorizontalAlignment(JLabel.CENTER); heading.setFont(new Font("Times New Roman", Font.BOLD, 40)); frame.add(heading); JLabel uname = new JLabel("Username : "); uname.setBounds(50, 130, 150, 50); frame.add(uname); JTextField name = new JTextField(); name.setBounds(50, 170, 350, 30); frame.add(name); JLabel upass = new JLabel("Password : "); upass.setBounds(50, 200, 150, 50); frame.add(upass); JPasswordField pass = new JPasswordField(); pass.setBounds(50, 240, 350, 30); frame.add(pass); JButton login = new JButton("LOGIN"); login.setBounds(100, 300, 100, 40); frame.add(login); login.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String username = name.getText(); String password = pass.getText(); if(username.isEmpty() || password.isEmpty()) { JOptionPane.showMessageDialog(frame, "Please Enter All Details!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } else { try { SQLManage manage= new SQLManage(); id = manage.authUser(username, password); } catch (SQLException e1) { e1.printStackTrace(); } if (id == -1) { JOptionPane.showMessageDialog(frame, "No User Found!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } else if(id == 0) { JOptionPane.showMessageDialog(frame, "Wrong Password!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } else { MainPage mainPage = new MainPage(); try { mainPage.mainPageView(id); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } } } }); JButton signUp = new JButton("SIGNUP"); signUp.setBounds(250, 300, 100, 40); frame.add(signUp); signUp.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { SignUp signup = new SignUp(); signup.signUpView(); } }); JButton attend = new JButton("ATTEND A SURVEY (GUEST)"); attend.setBounds(100, 350, 250, 40); frame.add(attend); attend.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String surveyCode = JOptionPane.showInputDialog("Enter the Survey Code : "); try { if(!surveyCode.isEmpty() && surveyCode.length() == 5) { if(manage.check(surveyCode)) { Guest guest = new Guest(); guest.guestView(surveyCode); } else { JOptionPane.showMessageDialog(frame, "No Survey Available!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } } } catch(Exception e2) { } } }); frame.setVisible(true); } }

3. SignUp Module
File Name: “SignUp.java“
This module helps to create new users.
package survey; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.SQLException; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPasswordField; import javax.swing.JTextField; public class SignUp { public void signUpView() { JFrame frame = new JFrame(); frame.setSize(450, 450); frame.setLayout(null); frame.setLocationRelativeTo(null); JLabel heading = new JLabel("SURVEY SYSTEM"); heading.setBounds(0, 50, 450, 50); heading.setHorizontalAlignment(JLabel.CENTER); heading.setFont(new Font("Times New Roman", Font.BOLD, 40)); frame.add(heading); JLabel fName = new JLabel("Name : "); fName.setBounds(50, 120, 150, 50); frame.add(fName); JTextField fNameField = new JTextField(); fNameField.setBounds(50, 160, 350, 30); frame.add(fNameField); JLabel uName = new JLabel("Username : "); uName.setBounds(50, 190, 150, 50); frame.add(uName); JTextField uNameField = new JTextField(); uNameField.setBounds(50, 230, 350, 30); frame.add(uNameField); JLabel uPass = new JLabel("Password : "); uPass.setBounds(50, 260, 150, 50); frame.add(uPass); JPasswordField uPassField = new JPasswordField(); uPassField.setBounds(50, 300, 150, 30); frame.add(uPassField); JLabel uPass2 = new JLabel("Confirm Password : "); uPass2.setBounds(250, 260, 150, 50); frame.add(uPass2); JPasswordField uPassField2 = new JPasswordField(); uPassField2.setBounds(250, 300, 150, 30); frame.add(uPassField2); JButton submit = new JButton("SUBMIT"); submit.setBounds(175, 350, 100, 40); frame.add(submit); submit.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String fname = fNameField.getText(); String uname = uNameField.getText(); String pass1 = uPassField.getText(); String pass2 = uPassField2.getText(); if(fname.isEmpty() || uname.isEmpty() || pass1.isEmpty() || pass2.isEmpty()) { JOptionPane.showMessageDialog(frame, "Please Enter All Details!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } else { if(pass1.equals(pass2)) { try { SQLManage manage = new SQLManage(); manage.newUser(fname, uname, pass1); fNameField.setText(""); uNameField.setText(""); uPassField.setText(""); uPassField2.setText(""); JOptionPane.showMessageDialog(frame, "User Created Succesfully!!!", "User Created", JOptionPane.PLAIN_MESSAGE); frame.dispose(); } catch (SQLException e1) { JOptionPane.showMessageDialog(frame, "Something went wrong!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } } else { JOptionPane.showMessageDialog(frame, "Password Mismatch", "Warning Message", JOptionPane.WARNING_MESSAGE); } } } }); frame.setVisible(true); } }
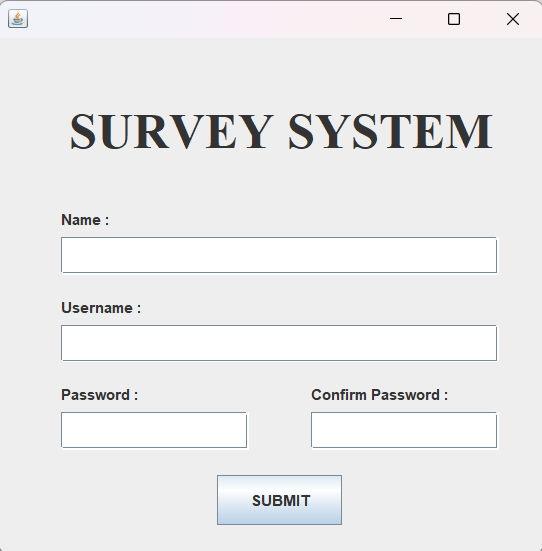
4. Home page Module
File Name: “MainPage.java“
This is the module for the home page of the users. Options for creating new surveys and viewing their result can be done from this page.
package survey; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.nio.charset.Charset; import java.sql.ResultSet; import java.sql.SQLException; import java.util.Random; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextField; import javax.swing.table.DefaultTableModel; public class MainPage { SQLManage manage; JButton submit; String[] questionsArray, option1Array, option2Array, option3Array, option4Array; static DefaultTableModel model; String cd; int i=0, h=0; String[] queStr = new String[50]; String[] op1Str = new String[50]; String[] op2Str = new String[50]; String[] op3Str = new String[50]; String[] op4Str = new String[50]; int id; public void mainPageView(int id) throws SQLException { this.id=id; questionsArray = new String[25]; option1Array = new String[25]; option2Array = new String[25]; option3Array = new String[25]; option4Array = new String[25]; manage = new SQLManage(); JFrame frame = new JFrame(); frame.setSize(800, 600); frame.setLayout(null); frame.setLocationRelativeTo(null); //-------------------------------------ADD PANEL-------------------------------------------------- JPanel addPanel = new JPanel(); addPanel.setBounds(250, 0, 550, 600); addPanel.setLayout(null); JLabel question = new JLabel("Question : "); question.setBounds(50, 100, 100, 20); addPanel.add(question); JTextField questionField = new JTextField(); questionField.setBounds(50, 125, 450, 30); addPanel.add(questionField); JLabel option1 = new JLabel("Option 1 : "); option1.setBounds(50, 165, 100, 20); addPanel.add(option1); JTextField option1Field = new JTextField(); option1Field.setBounds(50, 190, 200, 30); addPanel.add(option1Field); JLabel option2 = new JLabel("Option 2 : "); option2.setBounds(50, 230, 100, 20); addPanel.add(option2); JTextField option2Field = new JTextField(); option2Field.setBounds(50, 255, 200, 30); addPanel.add(option2Field); JLabel option3 = new JLabel("Option 3 : "); option3.setBounds(50, 295, 100, 20); addPanel.add(option3); JTextField option3Field = new JTextField(); option3Field.setBounds(50, 320, 200, 30); addPanel.add(option3Field); JLabel option4 = new JLabel("Option 4 : "); option4.setBounds(50, 360, 100, 20); addPanel.add(option4); JTextField option4Field = new JTextField(); option4Field.setBounds(50, 385, 200, 30); addPanel.add(option4Field); JLabel start = new JLabel("CREATE A SURVEY"); start.setBounds(0, 10, 550, 50); start.setHorizontalAlignment(JLabel.CENTER); start.setFont(new Font("Times New Roman", Font.BOLD, 40)); addPanel.add(start); JButton next = new JButton("ADD QUESTION >"); next.setBounds(50, 440, 450, 35); addPanel.add(next); next.addActionListener(new ActionListener( ) { @Override public void actionPerformed(ActionEvent e) { String qn = questionField.getText(); String op1 = option1Field.getText(); String op2 = option2Field.getText();; String op3 = option3Field.getText();; String op4 = option4Field.getText();; if(qn.equals("") || op1.equals("") || op2.equals("") || op3.equals("") || op4.equals("")) { JOptionPane.showMessageDialog(frame, "Please Enter All Details!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } else { questionField.setText(""); option1Field.setText(""); option2Field.setText(""); option3Field.setText(""); option4Field.setText(""); queStr[i] = qn; op1Str[i] = op1; op2Str[i] = op2; op3Str[i] = op3; op4Str[i] = op4; i++; submit.setEnabled(true); } } }); submit = new JButton("SUBMIT"); submit.setBounds(50, 490, 200, 50); submit.setEnabled(false); addPanel.add(submit); submit.addActionListener(new ActionListener( ) { @Override public void actionPerformed(ActionEvent e) { String code = stringGenerator(); String qn = questionField.getText(); String op1 = option1Field.getText(); String op2 = option2Field.getText();; String op3 = option3Field.getText();; String op4 = option4Field.getText();; if(!(qn.equals("") && op1.equals("") && op2.equals("") && op3.equals("") && op4.equals(""))) { JOptionPane.showMessageDialog(frame, "Last details are not added. If not required, Please clear the fields!!!", "Warning Message", JOptionPane.WARNING_MESSAGE); } else { if(i==0) { JOptionPane.showMessageDialog(frame, "No Questions Added", "Warning Message", JOptionPane.WARNING_MESSAGE); } else { try { manage.userQuestionAdd(id, code); for(int j=0; j<i; j++) { manage.newQuestion(code, queStr[j], op1Str[j], op2Str[j], op3Str[j], op4Str[j]); } JOptionPane.showMessageDialog(frame, "Survey Added. Survey Code : " + code, "Congradulations", JOptionPane.PLAIN_MESSAGE); } catch (SQLException e1) { e1.printStackTrace(); } } } submit.setEnabled(false); } }); JButton cancel = new JButton("CANCEL"); cancel.setBounds(300, 490, 200, 50); addPanel.add(cancel); cancel.addActionListener(new ActionListener( ) { @Override public void actionPerformed(ActionEvent e) { questionField.setText(""); option1Field.setText(""); option2Field.setText(""); option3Field.setText(""); option4Field.setText(""); i=0; } }); frame.add(addPanel); //--------------------------------------------------------------------------------------------------- //----------------------------------------------------VIEW PANEL--------------------------------------------- JPanel viewPanel = new JPanel(); viewPanel.setBounds(250, 0, 550, 600); viewPanel.setLayout(null); JLabel searchLabel = new JLabel("Search : "); searchLabel.setBounds(100, 20, 100, 50); viewPanel.add(searchLabel); JTextField search = new JTextField(); search.setBounds(160, 30, 290, 30); viewPanel.add(search); search.addKeyListener(new KeyListener() { @Override public void keyReleased(KeyEvent e) { tblupdt(search.getText()); } @Override public void keyTyped(KeyEvent e) { //To avoid errors. } @Override public void keyPressed(KeyEvent e) { //To avoid errors. } }); JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ return false; } }; model = (DefaultTableModel)table.getModel(); table.setBackground(Color.decode("#f9d6c4")); model.addColumn("Your Surveys"); tblupdt(""); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(100, 70, 350, 225); viewPanel.add(scPane); JLabel quesView = new JLabel(); quesView.setBounds(50, 340, 150, 50); viewPanel.add(quesView); JLabel op1View = new JLabel(); op1View.setBounds(70, 380, 150, 50); viewPanel.add(op1View); JLabel op2View = new JLabel(); op2View.setBounds(70, 420, 150, 50); viewPanel.add(op2View); JLabel op3View = new JLabel(); op3View.setBounds(70, 460, 150, 50); viewPanel.add(op3View); JLabel op4View = new JLabel(); op4View.setBounds(70, 500, 150, 50); viewPanel.add(op4View); JLabel op1Select = new JLabel(); op1Select.setBounds(100, 400, 150, 50); viewPanel.add(op1Select); JLabel op2Select = new JLabel(); op2Select.setBounds(100, 440, 150, 50); viewPanel.add(op2Select); JLabel op3Select = new JLabel(); op3Select.setBounds(100, 480, 150, 50); viewPanel.add(op3Select); JLabel op4Select = new JLabel(); op4Select.setBounds(100, 520, 150, 50); viewPanel.add(op4Select); JButton delete = new JButton("DELETE"); delete.setBounds(210, 300, 130, 50); delete.setEnabled(false); viewPanel.add(delete); JButton viewPrev = new JButton("PREVIOUS"); viewPrev.setBounds(100, 300, 100, 50); viewPrev.setEnabled(false); viewPanel.add(viewPrev); viewPrev.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(h>=0) { h--; quesView.setText(questionsArray[h]); op1View.setText(option1Array[h]); op2View.setText(option2Array[h]); op3View.setText(option3Array[h]); op4View.setText(option4Array[h]); try { op1Select.setText(String.valueOf(manage.getCount(cd, h, 1))); op2Select.setText(String.valueOf(manage.getCount(cd, h, 2))); op3Select.setText(String.valueOf(manage.getCount(cd, h, 3))); op4Select.setText(String.valueOf(manage.getCount(cd, h, 4))); } catch (SQLException e1) { e1.printStackTrace(); } } } }); delete.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { try { manage.removeSurvey(cd); } catch (SQLException e1) { e1.printStackTrace(); } tblupdt(search.getText()); } }); JButton viewNext = new JButton("NEXT"); viewNext.setBounds(350, 300, 100, 50); viewNext.setEnabled(false); viewPanel.add(viewNext); viewNext.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { h++; quesView.setText(questionsArray[h]); op1View.setText(option1Array[h]); op2View.setText(option2Array[h]); op3View.setText(option3Array[h]); op4View.setText(option4Array[h]); try { op1Select.setText(String.valueOf(manage.getCount(cd, h, 1))); op2Select.setText(String.valueOf(manage.getCount(cd, h, 2))); op3Select.setText(String.valueOf(manage.getCount(cd, h, 3))); op4Select.setText(String.valueOf(manage.getCount(cd, h, 4))); } catch (SQLException e1) { e1.printStackTrace(); } } }); table.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { h=0; delete.setEnabled(true); viewNext.setEnabled(true); viewPrev.setEnabled(true); int row = table.getSelectedRow(); cd = String.valueOf(model.getValueAt(row, 0)); try { ResultSet rst1 = manage.getQuestions(cd); for(int x=0; rst1.next(); x++) { questionsArray[x] = rst1.getString("question"); option1Array[x] = rst1.getString("option1"); option2Array[x] = rst1.getString("option2"); option3Array[x] = rst1.getString("option3"); option4Array[x] = rst1.getString("option4"); } op1Select.setText(String.valueOf(manage.getCount(cd, h, 1))); op2Select.setText(String.valueOf(manage.getCount(cd, h, 2))); op3Select.setText(String.valueOf(manage.getCount(cd, h, 3))); op4Select.setText(String.valueOf(manage.getCount(cd, h, 4))); } catch (SQLException e1) { e1.printStackTrace(); } quesView.setText(questionsArray[h]); op1View.setText(option1Array[h]); op2View.setText(option2Array[h]); op3View.setText(option3Array[h]); op4View.setText(option4Array[h]); } }); frame.add(viewPanel); //-------------------------------------------------------------------------------------------------------------- //-----------------------------------------------SIDE PANEL------------------------------------------------------- JPanel optionPanel = new JPanel(); optionPanel.setBounds(0, 0, 250, 600); optionPanel.setBackground(Color.gray); optionPanel.setLayout(null); frame.add(optionPanel); JButton addSurvey = new JButton("ADD SURVEY"); addSurvey.setBounds(50, 113, 150, 50); optionPanel.add(addSurvey); addSurvey.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { viewPanel.setVisible(false); addPanel.setVisible(true); } }); JButton viewSurvey = new JButton("VIEW SURVEY"); viewSurvey.setBounds(50, 276, 150, 50); optionPanel.add(viewSurvey); viewSurvey.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { tblupdt(search.getText()); viewPanel.setVisible(true); addPanel.setVisible(false); } }); JButton logout = new JButton("LOGOUT"); logout.setBounds(50, 440, 150, 50); optionPanel.add(logout); logout.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { Login login = new Login(); try { login.loginView(); } catch (SQLException e1) { e1.printStackTrace(); } frame.dispose(); } }); //------------------------------------------------------------------------------------------------------- viewPanel.setVisible(false); frame.setVisible(true); } public String stringGenerator() { String AlphaNumericString = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; String sb = ""; for (int i = 0; i < 5; i++) { int index = (int)(AlphaNumericString.length() * Math.random()); sb += (AlphaNumericString.charAt(index)); } return sb; } public void tblupdt(String str) { try { SQLManage man = new SQLManage(); ResultSet res = man.surveys(id, str); int rowCount = model.getRowCount(); int i; for (i = rowCount - 1; i >= 0; i--) model.removeRow(i); for(i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getString("surveycode"), i, 0); } } catch (SQLException e1) { e1.printStackTrace(); } } }

This page helps to create surveys.
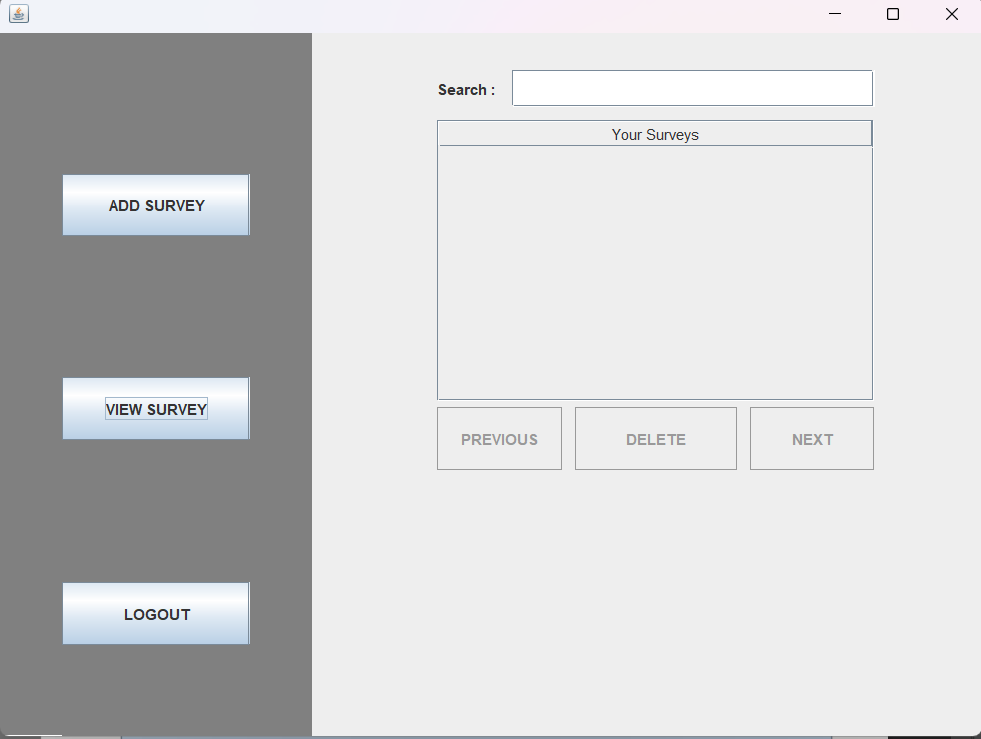
This page helps to view the response from the survey.
5. Guest Module
File Name: “Guest.java“
This module helps the users to attend the survey without logging in.
package survey; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.ResultSet; import java.sql.SQLException; import javax.swing.ButtonGroup; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JRadioButton; public class Guest { SQLManage manage; int[] opt; int k; public void guestView(String surveyCode) throws SQLException { manage = new SQLManage(); ResultSet rst = manage.getQuestions(surveyCode); opt = new int[50]; Font options = new Font("Times New Roman", Font.BOLD, 15); JFrame frame = new JFrame(); frame.setSize(800, 600); frame.setLayout(null); frame.setLocationRelativeTo(null); JLabel start = new JLabel("ATTENDING THE SURVEY"); start.setBounds(0, 50, 800, 50); start.setHorizontalAlignment(JLabel.CENTER); start.setFont(new Font("Times New Roman", Font.BOLD, 40)); frame.add(start); JLabel ques = new JLabel("Question Here!!!"); ques.setBounds(80, 200, 500, 30); ques.setFont(new Font("Times New Roman", Font.BOLD, 18)); frame.add(ques); JRadioButton op1 = new JRadioButton("Option1"); JRadioButton op2 = new JRadioButton("Option2"); JRadioButton op3 = new JRadioButton("Opyion3"); JRadioButton op4 = new JRadioButton("Option4"); ButtonGroup bgroup = new ButtonGroup(); bgroup.add(op1); bgroup.add(op2); bgroup.add(op3); bgroup.add(op4); op1.setBounds(100, 250, 500, 30); op2.setBounds(100, 300, 500, 30); op3.setBounds(100, 350, 500, 30); op4.setBounds(100, 400, 500, 30); op1.setFont(options); op2.setFont(options); op3.setFont(options); op4.setFont(options); if(rst.next()) { ques.setText(rst.getString("question")); op1.setText(rst.getString("option1")); op2.setText(rst.getString("option2")); op3.setText(rst.getString("option3")); op4.setText(rst.getString("option4")); } frame.add(op1); frame.add(op2); frame.add(op3); frame.add(op4); k=0; JButton nextButton = new JButton("NEXT"); nextButton.setBounds(100, 470, 600, 50); frame.add(nextButton); nextButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { int x; if(op1.isSelected()) { x=1; } else if(op2.isSelected()) { x=2; } else if(op3.isSelected()) { x=3; } else if(op4.isSelected()) { x=4; } else x=0; if(x!=0) { opt[k] = x; k++; try { if(rst.next()) { ques.setText(rst.getString("question")); op1.setText(rst.getString("option1")); op2.setText(rst.getString("option2")); op3.setText(rst.getString("option3")); op4.setText(rst.getString("option4")); } else { for(int j=0; j<k; j++) { manage.answerUpdt(surveyCode, j+1, opt[j]); } JOptionPane.showMessageDialog(frame, "Survey Completed. Thank You.", "Congradulations", JOptionPane.PLAIN_MESSAGE); manage.addTotal(); frame.dispose(); } } catch (SQLException e1) { e1.printStackTrace(); } } else { JOptionPane.showMessageDialog(frame, "Select an option!", "Warning", JOptionPane.WARNING_MESSAGE); } bgroup.clearSelection(); } }); frame.setVisible(true); } }

This page helps to answer the surveys. We can use the radio buttons.
6. MySql Manage Module
File Name: “SQLManage.java“
This class manages all the operations with databases. All classes call this class for SQL operations.
package survey; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class SQLManage { Connection con; public SQLManage() throws SQLException { String url = "jdbc:mysql://localhost:3306/survey"; String usr = "root"; String pass = "roshanhussainv1969@"; con = DriverManager.getConnection(url, usr, pass); } public void newUser(String name, String uname, String pass) throws SQLException { String str = "INSERT INTO actors(fname, uname, pass) values ('"+name+"', '"+uname+"', '"+pass+"')"; Statement stm = con.createStatement(); stm.executeUpdate(str); } public int authUser(String uname, String pass) throws SQLException { String str = "SELECT * FROM actors WHERE uname = '"+uname+"'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); if (!rst.next()) return -1; else { if(rst.getString("pass").equals(pass)) return rst.getInt("id"); else return 0; } } public void newQuestion(String code, String question, String op1, String op2, String op3, String op4) throws SQLException { String str = "INSERT INTO questions values ('"+code+"', '"+question+"', '"+op1+"', '"+op2+"', '"+op3+"', '"+op4+"')"; Statement stm = con.createStatement(); stm.executeUpdate(str); } public void userQuestionAdd(int id, String surveycode) throws SQLException { String str = "INSERT INTO userQuestions values ("+id+", '"+surveycode+"', 0)"; Statement stm = con.createStatement(); stm.executeUpdate(str); } public void answerUpdt(String surveycode, int qno, int option) throws SQLException { String str = "INSERT INTO surveyquestions values ('"+surveycode+"', " + qno + ", " + option + ")"; Statement stm = con.createStatement(); stm.executeUpdate(str); } public ResultSet getQuestions(String surveycode) throws SQLException { String str = "SELECT * FROM questions WHERE surveycode = '"+surveycode+"'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); return rst; } public ResultSet surveys(int id, String search) throws SQLException { String str = "SELECT * FROM userQuestions WHERE id = "+id+" and surveycode like '%"+search+"%'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); return rst; } public void addTotal() throws SQLException { String str = "UPDATE userQuestions SET total = total+1"; Statement stm = con.createStatement(); stm.executeUpdate(str); } public boolean check(String search) throws SQLException { String str = "SELECT * FROM userQuestions WHERE surveycode = '"+search+"'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); if(rst.next()) return true; else return false; } public void removeSurvey(String surveycode) throws SQLException { String str = "DELETE FROM questions WHERE surveycode = '"+surveycode+"'"; Statement stm = con.createStatement(); stm.executeUpdate(str); str = "DELETE FROM surveyquestions WHERE surveycode = '"+surveycode+"'"; stm.executeUpdate(str); str = "DELETE FROM userQuestions WHERE surveycode = '"+surveycode+"'"; stm.executeUpdate(str); } public int getCount(String surveycode, int qno, int op) throws SQLException { String str = "SELECT count(opno) FROM surveyquestions WHERE surveycode = '"+surveycode+"' AND qno = "+(qno+1)+" AND opno = "+op; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); if(rst.next()) return rst.getInt("count(opno)"); else return 0; } }
Output for Survey Management System In Java
Output 1: Login and Signup
Output 2: Home Page
Output 3: Attending Survey (Guest)
Thank you for visiting us.
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023
- Snake Game in Java