
In this article, we will build Hospital Management System Project in C++ and MySQL with source code. This project deals with all the essential operations of managing the crucial data of any hospital, it’s a console-based application written in C++ language and for the database, we have used MySQL database with the help of Xampp software.
Project Overview: Hospital Management System Project in C++
Project Name: | Hospital Management System Project in C++ |
Abstract: | We will create a console-based project to help in hospital management. |
Language Used: | C++ |
IDE: | Code::Blocks(16.01) |
Database: | MySQL |
Type: | Console Application |
Recommended for: | Beginners and Intermediates of C++ |
Features
- Admin can log in and log out into the application with protected credentials (username admin, password admin)
- View available lists of Doctors
- Create a new appointment with any available doctor
- View all appointments for a particular day
- Check the number of available beds
- View all staff information
- Add new staff information
Setup the Development Environment
To successfully develop and run the application you will need to install the following software and follow the below step.
- Dev C++
- Code::Blocks (16.01)
- Xampp(For MySQL Database)
Now you need to set up the Code::Blocks IDE to be able to connect your C++ application to the MySQL database. You can follow this tutorial.
MySQL setup for Hospital Management System in C++
We will use Xampp software to easily manage and use MySql on the system.
- Open the Xampp software and run Apache and MySql.
- Go to your browser window and search for localhost/phpmyadmin/.
- Run the following SQL command. These commands will create a database with the name hospitalmanagement and two tables within this database with some information.
CREATE DATABASE hospitalmanagement; use hospitalmanagement; CREATE TABLE users( username Varchar(20), password varchar(20) ); INSERT INTO users (username, password) VALUES ('admin', 'admin'); CREATE TABLE appointment( doctor varchar(20), patientname varchar(20), time varchar(20), age varchar(10), contactno varchar(20), symptoms varchar(30) ); INSERT INTO appointment(doctor, patientname, time, age, contactno, symptoms) VALUES ('Dr. Waqar', 'Raj', '10:30 AM', '28', '9945367281', 'cough'); INSERT INTO appointment(doctor, patientname, time, age, contactno, symptoms) VALUES ('Dr. Shankar', 'Rana', '1:30 PM', '18', '9945367281', 'fever'); INSERT INTO appointment(doctor, patientname, time, age, contactno, symptoms) VALUES ('Dr. Krishna', 'Rahul', '9 AM', '38', '9945367281', 'mild fever'); INSERT INTO appointment(doctor, patientname, time, age, contactno, symptoms) VALUES ('Dr. Roy', 'Ravi', '1 PM', '28', '9945367281', 'cough'); CREATE TABLE staff( name varchar(20), age varchar(10), salary varchar(50), contactno varchar(20), position varchar(40) ); INSERT INTO staff(name, age, salary, contactno, position) VALUES ('Vikram Raj', '33', '20000', '9934627721', 'dispensor');
Code for Hospital Management System Project in C++
Open Code::Blocks IDE and create a new project with the name HospitalManagement. Now paste the below code in the main.cpp file and run it.
#include <iostream> #include<winsock.h> #include<windows.h> #include<sstream> #include<ctime> #include<string> #include<mysql.h> #include<cstring> #include<conio.h> #include<cstring> #include<map> using namespace std; int users =0; int count_attempt=0; int curr_user=0; int user_no=0; map <string,string> issued; string loggedin_user=""; void showtime() { time_t now = time(0); char *dt = ctime(&now); cout<<" \t \t \t \t \t @copyassignment " << dt; cout<<endl<<endl; } void showDoctorsDetails() { system("cls"); showtime(); cout<<"<==========================================================>"<<endl; cout<<" Dr. Waqar "<<endl; cout<<" -----------Timing----------- "<<endl; cout<<" Monday To Friday 9AM - 5PM "<<endl; cout<<" Saturday 10AM - 1PM "<<endl; cout<<" Sunday OFF "<<endl; cout<<"<==========================================================>"<<endl; cout<<"<==========================================================>"<<endl; cout<<" Dr. Shankar "<<endl; cout<<" -----------Timing----------- "<<endl; cout<<" Monday To Friday 1PM - 9PM "<<endl; cout<<" Saturday 1PM - 5PM "<<endl; cout<<" Sunday OFF "<<endl; cout<<"<==========================================================>"<<endl; cout<<"<==========================================================>"<<endl; cout<<" Dr. Krishna "<<endl; cout<<" -----------Timing----------- "<<endl; cout<<" Monday To Friday 8AM - 6PM "<<endl; cout<<" Saturday 10AM - 1PM "<<endl; cout<<" Sunday 3PM - 5PM "<<endl; cout<<"<==========================================================>"<<endl; cout<<"<==========================================================>"<<endl; cout<<" Dr. Roy "<<endl; cout<<" -----------Timing----------- "<<endl; cout<<" Monday To Friday 9AM - 5PM "<<endl; cout<<" Saturday 10AM - 1PM "<<endl; cout<<" Sunday 7PM - 9PM "<<endl; cout<<"<==========================================================>"<<endl; } class user { private: string username; string password; public: // This method is used to take user input and check against the data in the database to login to the system void login() { if(curr_user==1) { cout<<" \t \t \t \t \t Already logged in"; return; } if(count_attempt==3) { cout<<endl<<endl; cout<<" \t \t \t \t \t No more attempts"; exit(0); } cout<<"Enter Username and Password to Login"<<endl; string user_name; string pass_word; cout<<"Username:"; cin>>user_name; cout<<"Password:"; cin>>pass_word; cin.ignore(); system("cls"); MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "hospitalmanagement" ,0,NULL,0); if(conn) { int qstate = mysql_query(conn,"SELECT username,password FROM users"); bool flag=false; if(!qstate) { res = mysql_store_result(conn); while(row = mysql_fetch_row(res)) { if(row[0]==user_name && row[1]==pass_word) { loggedin_user = row[0]; showtime(); cout<<" \t \t \t \t \t \tLogin Successful"<<endl<<endl; cout<<"\t\t\t==============================================================="<<endl; cout<<"\t\t\t Welcome Admin "<<endl; cout<<"\t\t\t==============================================================="<<endl; curr_user=1; flag=true; break; } } if(!flag) { cout<<" \t \t \t \t \t \t Incorrect Username or Password"<<endl; cout<<" \t \t \t \t \t \t Press Enter and Try again"<<endl; cin.ignore(); system("cls"); count_attempt++; login(); } } else { cout<<" \t \t \t \t \t No Accounts Registered"; } } } void logout() { cout<<"Logout Successful"<<endl; curr_user=0; } }; class hospital : public user { public: // This method is used to view all the appointment details from the database void allAppointment() { if(loggedin_user=="") { cout<<" \t \t \t \t \t Please Login"<<endl; return ; } MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "hospitalmanagement" ,0,NULL,0); if(conn) { int qstate = mysql_query(conn,"SELECT doctor, patientname, time, age, contactno, symptoms from appointment"); if(!qstate) { res = mysql_store_result(conn); system("cls"); showtime(); cout<<"Appointments for today are"<<endl; cout<<endl; while(row = mysql_fetch_row(res)) { cout<<"Doctor: "<<row[0]<<endl; cout<<"Patient Name: "<<row[1]<<endl; cout<<"Appointment Time: "<<row[2]<<endl; cout<<"Age: "<<row[3]<<endl; cout<<"Contact No: "<<row[4]<<endl; cout<<"Symptoms: "<<row[5]<<endl; cout<<endl; } } } else cout<<"Failed"<<endl; } // This method is used to add a new appointment in the database void addappointment(){ string doc, name, time, symptoms; long long int age, contactno; if(loggedin_user=="") { cout<<" \t \t \t \t \t Please Login"<<endl; return ; } cout<<"Choose Doctor from the list of availble doctors:"<<endl; cout<<"1. Dr. Waqar"<<endl; cout<<"2. Dr. Shankar"<<endl; cout<<"3. Dr. Krishna"<<endl; cout<<"4. Dr. Roy"<<endl; cout<<"\nEnter Doctor Name:"<<endl; cin.ignore(); getline(cin,doc); cout<<"\nEnter Patient Name:"<<endl; cin>>name; cout<<"\nEnter Appointment Time:"<<endl; cin.ignore(); getline(cin,time); cout<<"\nEnter Patient Age:"<<endl; cin>>age; cout<<"\nEnter Patient Contact no:"<<endl; cin>>contactno; cout<<"\nEnter Patient Symptoms:"<<endl; cin.ignore(); getline(cin,symptoms); string nul=""; MYSQL* conn; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "hospitalmanagement" ,0,NULL,0); stringstream ss; ss<<"INSERT INTO appointment(doctor, patientname, time, age, contactno, symptoms) VALUES('"<<doc<<"','"<<name<<"','"<<time<<"','"<<age<<"', '"<<contactno<<"','"<<symptoms<<"')"; int qstate=0; string query = ss.str(); const char* q= query.c_str(); qstate = mysql_query(conn,q); if(qstate==0) { cout<<" \t \t \t \t \t Appointment added Successfully!"<<endl; return; } else { cout<<" \t \t \t \t \t Failed"<<endl; return; } } // This method is used to add a new staff entry in the database void addstaff(){ string name, position; long long int age, contactno, salary; if(loggedin_user=="") { cout<<" \t \t \t \t \t Please Login"<<endl; return ; } cout<<"\nEnter Staff Name:"<<endl; cin.ignore(); getline(cin,name); cout<<"\nEnter Staff Age:"<<endl; cin>>age; cout<<"\nEnter Staff Salary:"<<endl; cin>> salary; cout<<"\nEnter Staff Contact No:"<<endl; cin>>contactno; cout<<"\nEnter Staff Position:"<<endl; cin>>position; string nul=""; MYSQL* conn; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "hospitalmanagement" ,0,NULL,0); stringstream ss; ss<<"INSERT INTO staff(name, age, salary, contactno, position) VALUES('"<<name<<"','"<<age<<"','"<<salary<<"','"<<contactno<<"','"<<position<<"')"; int qstate=0; string query = ss.str(); const char* q= query.c_str(); qstate = mysql_query(conn,q); if(qstate==0) { cout<<" \t \t \t \t \t Staff Information added Successfully!"<<endl; return; } else { cout<<" \t \t \t \t \t Failed"<<endl; return; } } // This method is used to view all the available staff details in the database void allstaff(){ if(loggedin_user=="") { cout<<" \t \t \t \t \t Please Login"<<endl; return ; } showtime(); MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "hospitalmanagement" ,0,NULL,0); if(conn) { int qstate = mysql_query(conn,"SELECT name, age, salary, contactno, position from staff"); if(!qstate) { res = mysql_store_result(conn); system("cls"); cout<<"--------------All Staffs Details------------"<<endl; cout<<endl; while(row = mysql_fetch_row(res)) { cout<<"Name: "<<row[0]<<endl; cout<<"Age: "<<row[1]<<endl; cout<<"Salary: "<<row[2]<<endl; cout<<"Contact No: "<<row[3]<<endl; cout<<"Position: "<<row[4]<<endl; cout<<endl; } } } else cout<<"Failed"<<endl; } // This method is used to check the number of available beds randomly void checkBeds(){ if(loggedin_user=="") { cout<<" \t \t \t \t \t Please Login"<<endl; return ; } system("cls"); showtime(); cout<<endl; int random_no_of_beds = 0; int N = 200; random_no_of_beds = rand() % 200; //this will keep the random number within the range of 200 cout<<"Number of beds available today are: "<<random_no_of_beds<<endl; } }; int main() { system("Color DE"); showtime(); cout<<"\t\t\t=============================================================================="<<endl; cout<<"\t\t\t Hospital Management System "<<endl; cout<<"\t\t\t=============================================================================="<<endl; user u1; int x; int choice=0; hospital h; do { cout<<endl; cout<<"\t----------choose from below----------"<<endl; cout<<"\t1. Login"<<endl; cout<<"\t2. Available Doctors"<<endl; cout<<"\t3. Check All Appointments"<<endl; cout<<"\t4. Add new Doctor Appointment"<<endl; cout<<"\t5. Check no of beds available"<<endl; cout<<"\t6. Store new Staff Information"<<endl; cout<<"\t7. Check all Staff Information"<<endl; cout<<"\t8. Logout"<<endl; cout<<"\t0 to exit"<<endl; cout<<"\t-------------------------------------"<<endl; cin>>x; switch(x) { case 1 : u1.login(); break; case 2 : showDoctorsDetails(); break; case 3 : h.allAppointment(); break; case 4 : h.addappointment(); break; case 5 : h.checkBeds(); break; case 6 : h.addstaff(); break; case 7 : h.allstaff(); break; case 8 : u1.logout(); break; case 0 : choice=1; } } while(choice==0); }
Output for Hospital Management System Project in C++
Image Output:
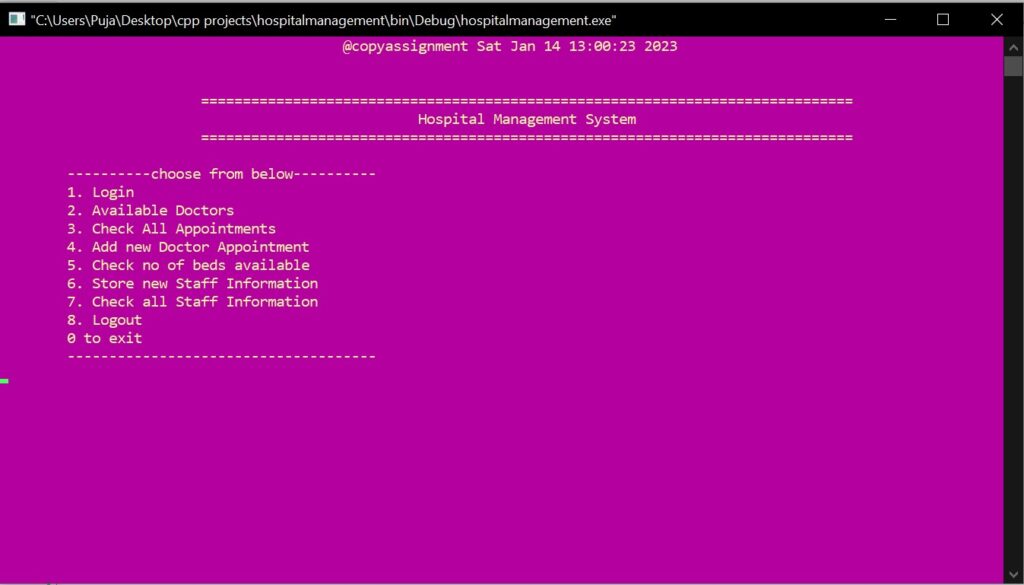
Video Output:
Conclusion
The Hospital Management System Project in C++ can be very helpful for any hospital as this will automate most of the manual tasks. In this project we have added most of the major operations of hospital management like viewing all available doctors, creating a new appointment, checking all appointments, viewing the number of available beds, adding new staff information, and viewing all existing staff information.
Thank you for visiting our website.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector