
In this article, we will build Student Management System Project in C++ and MySQL. Student Management System can help a college or university to maintain and manage all details of students easily. We have used the MySQL database with the help of Xampp software.
Project Overview: Student Management System Project in C++
Project Name: | Student Management System Project in C++ |
Abstract: | We will create a console-based project to help in student management. |
Language Used: | C++ |
IDE: | Code::Blocks(16.01) |
Database: | MySQL |
Type: | Console Application |
Recommended for: | Beginners and Intermediates of C++ |
Features of Student Management System Project in C++
- Admin can log in to the system with a protected username and password (default credentials for admin is username admin password admin)
- Admin can add new student records, view all existing records, update a record and delete a record.
Setup the Development Environment
To successfully develop and run the application you will need to install the following software and follow the below step.
- Dev C++
- Code::Blocks (16.01)
- Xampp(For MySQL Database)
Now you need to set up the Code::Blocks IDE to be able to connect your C++ application to the MySQL database. You can follow this tutorial.
MySQL setup for Student Management System in C++
We will use Xampp software to easily manage and use MySQL on the system.
- Open the Xampp software and run Apache and MySQL.
- Go to your browser window and search for localhost/phpmyadmin/.
- Run the following SQL command. These commands will create a database with the name studentmanagement and two tables within this database with some records.
CREATE DATABASE studentmanagement; use studentmanagement; CREATE TABLE userdetail( username Varchar(20), password varchar(20) ); INSERT INTO userdetail (username, password) VALUES ('admin', 'admin'); CREATE TABLE studentdetails( studentno int, firstname varchar(20), lastname varchar(20), mobileno varchar(20), course varchar(20), stream varchar(20) ); INSERT INTO studentdetails (studentno, firstname, lastname, mobileno, course, stream) VALUES ('1', 'Ravi', 'Sharma', '9936277219', 'BTech', 'CSE'); INSERT INTO studentdetails (studentno, firstname, lastname, mobileno, course, stream) VALUES ('2', 'Kriti', 'Singh', '9924657219', 'BTech', 'IT'); INSERT INTO studentdetails (studentno, firstname, lastname, mobileno, course, stream) VALUES ('3', 'Krishna', 'Kumar', '9213477219', 'BTech', 'ECE'); INSERT INTO studentdetails (studentno, firstname, lastname, mobileno, course, stream) VALUES ('4', 'Rahul', 'Sharma', '9932378219', 'BTech', 'EEE'); INSERT INTO studentdetails (studentno, firstname, lastname, mobileno, course, stream) VALUES ('5', 'Rani', 'Sinha', '9239869521', 'BTech', 'IT');
Code for Student Management System Project in C++
Open Code::Blocks IDE and create a new project with the name StudentManagement. Now paste the below code in the main.cpp file and run it.
#include <iostream> #include<winsock.h> #include<windows.h> #include<sstream> #include<ctime> #include<string> #include<mysql.h> #include<cstring> #include<conio.h> #include<cstring> #include<map> using namespace std; int count_attempt=0; int curr_user=0; int user_no=0; string loggedin_user=""; void showtime() { time_t now = time(0); char *dt = ctime(&now); cout<<" \t \t \t \t \t @copyassignment " << dt; cout<<endl<<endl; } class user { private: string username; string password; public: // This method is used to take user input and check against the data in the database to login to the system void login() { if(curr_user==1) { cout<<" \t \t \t \t \t Already logged in"; return; } if(count_attempt==3) { cout<<endl<<endl; cout<<" \t \t \t \t \t No more attempts"; exit(0); } cout<<"Enter Username and Password to Login"<<endl; string user_name; string pass_word; cout<<"Username:"; cin>>user_name; cout<<"Password:"; cin>>pass_word; cin.ignore(); system("cls"); MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "studentmanagement" ,0,NULL,0); if(conn) { int qstate = mysql_query(conn,"SELECT username,password FROM userdetail"); bool flag=false; if(!qstate) { res = mysql_store_result(conn); while(row = mysql_fetch_row(res)) { if(row[0]==user_name && row[1]==pass_word) { loggedin_user = row[0]; cout<<" \t \t \t \t \t \t Login Successful"<<endl; cout<<" \t \t \t \t \t \t WELCOME "<< loggedin_user<<endl; curr_user=1; flag=true; break; } } if(!flag) { cout<<" \t \t \t \t \t \t Incorrect Username or Password"<<endl; cout<<" \t \t \t \t \t \t Press Enter and Try again"<<endl; cin.ignore(); system("cls"); count_attempt++; login(); } } else { cout<<" \t \t \t \t \t No Accounts Registered"; } } } void logout() { curr_user=0; } }; class student : public user { long long int sno; string firstname, lastname; long long int mobile; string course, strm; public: // This method is protected for admin use only void addStudent() { if(loggedin_user=="") { cout<<" \t \t \t \t \t Please Login"<<endl; return ; } if(loggedin_user!="admin") { cout<<" \t \t \t \t \t Not Authorized. Only admin can add new books"<<endl; return ; } cout<<"Enter student roll number \n"; cin>>sno; cin.ignore(); cout<<"Enter student first name: \n"; cin>>firstname; cin.ignore(); cout<<"Enter last name: \n"; cin>>lastname; cout<<"Enter mobile number \n"; cin>>mobile; cin.ignore(); cout<<"Enter course name: \n"; cin>>course; cin.ignore(); cout<<"Enter stream: \n"; cin>>strm; string nul=""; MYSQL* conn; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "studentmanagement" ,0,NULL,0); stringstream ss; ss<<"INSERT INTO studentdetails(studentno, firstname, lastname, mobileno, course, stream) VALUES('"<<sno<<"','"<<firstname<<"','"<<lastname<<"','"<<mobile<<"','"<<course<<"','"<<strm<<"')"; int qstate=0; string query = ss.str(); const char* q= query.c_str(); qstate = mysql_query(conn,q); if(qstate==0) { cout<<" \t \t \t \t \t Student Record Added Successfully!"<<endl; return; } else { cout<<" \t \t \t \t \t Failed"<<endl; return; } } // This method is used to search for a particular record in the database bool searchStudent(string no) { if(loggedin_user=="") { cout<<" \t \t \t \t \t Please Login"<<endl; return false; } bool flag=false; MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "studentmanagement" ,0,NULL,0); if(conn) { int qstate = mysql_query(conn,"SELECT studentno, firstname, lastname, mobileno, course, stream from studentdetails"); if(!qstate) { res = mysql_store_result(conn); while(row = mysql_fetch_row(res)) { if(row[0]== no) { cout<<"Student Details are"<<endl; cout<<row[0]<<" "<<row[1]<<" "<<row[2]<<" "<<row[3]<<" "<<row[4]<<" "<<row[5]<<endl; flag=true; } } } else cout<<"Failed"<<endl; if(!flag) { cout<<"Student Details Not Found"<<endl; return false; } } } // This method is used to view all the records in the database void allStuddents() { MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "studentmanagement" ,0,NULL,0); if(conn) { int qstate = mysql_query(conn,"SELECT studentno, firstname, lastname, mobileno, course, stream from studentdetails"); if(!qstate) { res = mysql_store_result(conn); while(row = mysql_fetch_row(res)) { cout<<" \t \t \t \t \t "<<row[0] <<" "<<row[1]<<" "<<row[2]<<" "<<row[3]<<" "<<row[4]<<" "<<row[5]<<" "<<endl; } } } else cout<<"Failed"<<endl; } // This method is used to update the record of student in database void updateStudent() { if(loggedin_user=="") { cout<<"Please Login"<<endl; return ; } string sno; cout<<" \t Enter student roll number to update"<<endl; cin>>sno; string fname, lname, crse, sec; long long int mobileno; cout<<" \t Enter new first name"<<endl; cin>>fname; cin.ignore(); cout<<" \t Enter new last name"<<endl; cin>>lname; cin.ignore(); cout<<" \t Enter new mobile name"<<endl; cin>>mobileno; cin.ignore(); cout<<" \t Enter new course name"<<endl; cin>>crse; cin.ignore(); cout<<" \t Enter new section name"<<endl; cin>>sec; MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "studentmanagement" ,0,NULL,0); stringstream ss; int qstate=0; if(conn) { int qstate = mysql_query(conn,"SELECT studentno, firstname, lastname, mobileno, course, stream from studentdetails"); if(!qstate) { res = mysql_store_result(conn); while(row = mysql_fetch_row(res)) { if(sno==row[0]) { ss<<"UPDATE studentdetails SET firstname= '"<<fname<<"', lastname = '"<< lname <<"', mobileno = '"<<mobileno<<"', course = '"<<crse<<"', stream ='"<<sec<<"' WHERE studentno = "<<sno; string query = ss.str(); const char* q= query.c_str(); qstate = mysql_query(conn,q); if(qstate==0){ cout<<" \t \t \t \t \t Details Updated Successfully "<<endl; return; } else cout<<"Failed"<<endl; return; } } } } } // This method is used to delete a record void deleteStudent() { if(loggedin_user=="") { cout<<"Please Login"<<endl; return ; } string sno; cout<<" \t Enter student roll number to be deleted"<<endl; cin>>sno; MYSQL* conn; MYSQL_ROW row; MYSQL_RES* res; conn = mysql_init(0); conn = mysql_real_connect(conn ,"localhost" ,"root", "", "studentmanagement" ,0,NULL,0); stringstream ss; int qstate=0; if(conn) { int qstate = mysql_query(conn,"SELECT studentno, firstname, lastname, mobileno, course, stream from studentdetails"); if(!qstate) { res = mysql_store_result(conn); while(row = mysql_fetch_row(res)) { if(sno==row[0]) { ss<<"DELETE FROM studentdetails WHERE studentno="<<sno; string query = ss.str(); const char* q= query.c_str(); qstate = mysql_query(conn,q); if(qstate==0){ cout<<" \t \t \t \t \t Details Deleted Successfully "<<endl; } else cout<<"Failed"<<endl; return; } } } } } }; int main() { showtime(); system("Color E4"); cout<<"\t\t\t=============================================================================="<<endl; cout<<"\t\t\t Student Management System "<<endl; cout<<"\t\t\t=============================================================================="<<endl; user u1; int x; int choice=0; student b1; do { cout<<endl; cout<<"\tMenu:"<<endl; cout<<" \t 1. Administrator Login"<<endl; cout<<" \t 2. All Students"<<endl; cout<<" \t 3. Add New Student Record"<<endl; cout<<" \t 4. Search Student Record"<<endl; cout<<" \t 5. Delete a Student Record"<<endl; cout<<" \t 6. Update Student Record"<<endl; cout<<" \t 7. Logout"<<endl; cout<<" \t 0 to exit"<<endl; cin>>x; string no2; switch(x) { case 1 : u1.login(); break; case 2 : b1.allStuddents(); break; case 3 : b1.addStudent(); break; case 4 : cout<<"Enter student roll no"; cin>>no2; b1.searchStudent(no2); break; case 5 : b1.deleteStudent(); break; case 6 : b1.updateStudent(); break; case 7: u1.logout(); break; case 0 : choice=1; } } while(choice==0); }
Output for Student Management System Project in C++
Image Output:
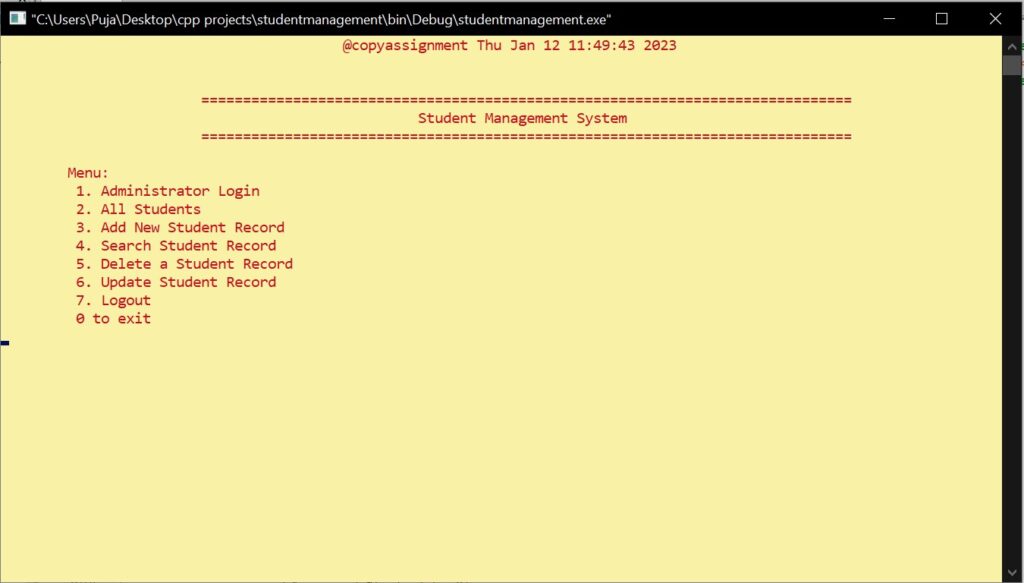
Video Output:
Conclusion
The Student Management System Project in C++ can help colleges or universities in storing all the records of students safely in one place. This will ease the process of managing thousands of records. In this project, we have added the major operations which can be performed on records like adding a new record, updating records, deleting records, and viewing all records.
Thank you for visiting our website.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector