
Introduction
In this article, we will build Blood Bank Management System Project in Java and MySQL with source code. This project is great for those at an intermediate level in Java who want to advance their coding skills. In this project, the users can perform the following functionalities Login, Add donor details, update donor details, view donor details, and delete donor details. Let’s get started!
Setting the development environment
You must have java JDK installed on your system and we are using Eclipse IDE to build this project, you can use either this or Netbeans IDE.
The first step will be to create a new project. Name it as you wish. In the src folder create a bloodbankmanagement package. In that package, we will be creating some files for different modules.
To connect the system with the database you will need to follow specific steps.
- You should have Java JDK installed and an IDE like Eclipse.
- Install MySQL on the system.
- Download the MySQL connector from here.
- In Eclipse, under your project expand external libraries and right-click, and select Open bloodbankmanagement Settings. Select the libraries tab and click on the + button. Browse your jar file downloaded from the above step and click on it. This will add a dependency to your project. The steps will differ if you are using a different IDE.
MySql Setup for Blood Bank Management System
1. Create a database
-- Creating database
create database bloodbank;
2. Select the database
-- Selecting the database
use bloodbank;
3. Create a bloodbank table
-- Creating bloodbank table
create table bloodbank (
id int primary key,
name varchar(25) not null,
bloodgroup varchar(5),
quantity int
);
4. Create an admin table
-- Create admin table
create table admin (
login_id varchar(25),
password varchar(25)
);
5. Insert some values in the admin table
-- Inserting value in admin table
insert into admin values
("admin","admin")
Coding Blood Bank Management System Project in Java
First, we need to create a class DbConnection to connect our database to the program
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class DBConnection { public Connection con; String url ="jdbc:mysql://localhost:3307/bloodbank"; String db = "bloodbank"; String user = "root"; String pass = "root"; public Connection mkDataBase() throws SQLException{ try { Class.forName("com.mysql.jdbc.Driver"); con = DriverManager.getConnection(url, user, pass); } catch (ClassNotFoundException ex) { Logger.getLogger(DBConnection.class.getName()).log(Level.SEVERE, null, ex); } return con; } }
1. Login module
This module helps to make a login page so the user can enter the user name and password to the blood bank management system in java. Name the file login.java.
import java.awt.*; import javax.swing.*; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.colorchooser.AbstractColorChooserPanel; public class Login extends JFrame{ JLabel idLabel; JLabel passLabel; JLabel background; JLabel headerLabel; JLabel devInfo; JTextField id; JPasswordField pass; JButton submit; public Login(){ setTitle("Blood Bank Management System"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true); this.background = new JLabel(new ImageIcon("")); this.init(); add(background); background.setVisible(true); this.pack(); this.setSize(700,400); this.setLocationRelativeTo(null); } public void init(){ headerLabel = new JLabel(); this.headerLabel.setText("Blood Bank Management System"); this.headerLabel.setBounds(190,1,370,150); this.headerLabel.setFont(new Font("Geomanist", Font.BOLD, 20)); headerLabel.setForeground(Color.red); add(headerLabel); idLabel = new JLabel(); this.idLabel.setText("Username"); this.idLabel.setBounds(190,110,100,50); this.idLabel.setFont(new Font(null, Font.BOLD, 20)); idLabel.setForeground(Color.black); add(idLabel); passLabel=new JLabel("Password"); this.passLabel.setBounds(190,165,100,50); this.passLabel.setFont(new Font(null, Font.BOLD, 20)); passLabel.setForeground(Color.black); add(passLabel); devInfo = new JLabel(); this.devInfo.setText(""); this.devInfo.setBounds(130,300,1000,30); this.devInfo.setFont(new Font("Geomanist", Font.PLAIN, 15)); devInfo.setForeground(Color.white); add(devInfo); id=new JTextField(); this.id.setBounds(300,125,200,30); add(id); pass=new JPasswordField(); this.add(pass); this.pass.setBounds(300,175,200,30); this.id.setVisible(true); this.submit=new JButton("Login"); this.submit.setBounds(400,230,100,25); add(submit); submit.addActionListener(this::submitActionPerformed); id=new JTextField(); this.id.setBounds(300,125,200,30); add(id); pass=new JPasswordField(); this.add(pass); this.pass.setBounds(300,175,200,30); this.id.setVisible(true); this.submit=new JButton("Login"); this.submit.setBounds(400,230,100,25); add(submit); submit.addActionListener(this::submitActionPerformed); } public class DBConnection { public Connection con; String url ="jdbc:mysql://localhost:3306/bloodbankmanagement"; String db = "bloodbankmanagement"; String user = "root"; String pass = ""; public Connection mkDataBase() throws SQLException{ try { Class.forName("com.mysql.jdbc.Driver"); con = DriverManager.getConnection(url, user, pass); } catch (ClassNotFoundException ex) { Logger.getLogger(DBConnection.class.getName()).log(Level.SEVERE, null, ex); } return con; } } public void submitActionPerformed(java.awt.event.ActionEvent evt){ if(id.getText().equals("admin") && pass.getText().equals("admin")){ this.hide(); Frame2new fn=new Frame2new(); fn.showButtonDemo(); } else{ JOptionPane.showMessageDialog(null, "Invalid password!"); } } }
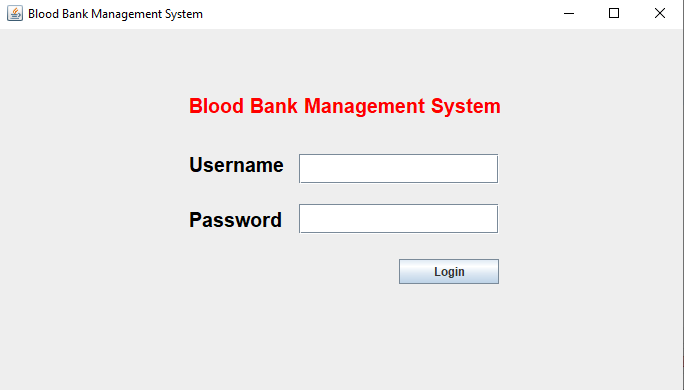
2. Home Module
Once we enter the correct username and password on the login page, it takes us to the home page of our Blood Bank Management System Project in Java. Name the file home.java.
import java.awt.*; import java.awt.event.*; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.*; import java.awt.Color; public class Home { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; public static void main(String[] args){ Frame2new swingControlDemo = new Frame2new(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Blood Bank Management System"); mainFrame.setBounds(100,100,700,400); mainFrame.setLayout(new GridLayout(3,1)); mainFrame.getContentPane().setBackground(Color.red); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new JLabel("", JLabel.CENTER); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,300); controlPanel = new JPanel(); controlPanel.setLayout(new GridLayout(1,5)); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Blood Bank Management System"); this.headerLabel.setFont(new Font(null, Font.BOLD, 27)); headerLabel.setForeground(Color.white); JButton fkButton = new JButton("Donors"); JButton billButton = new JButton("Blood Donations"); JButton afButton = new JButton("Add Donor"); JButton ufButton = new JButton("Update Donor"); JButton dlButton = new JButton("Delete Donor"); fkButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { ItemInfo ii=new ItemInfo(); try { ii.showButtonDemo(); } catch (SQLException ex) { Logger.getLogger(Frame2new.class.getName()).log(Level.SEVERE, null, ex); } } }); controlPanel.add(ufButton); controlPanel.add(afButton); controlPanel.add(billButton); controlPanel.add(fkButton); controlPanel.add(dlButton); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } public void viewButtonDemo(){ headerLabel.setText("Blood Bank Management System"); this.headerLabel.setFont(new Font(null, Font.BOLD, 27)); headerLabel.setForeground(Color.white); JButton fkButton = new JButton("Donors"); JButton billButton = new JButton("Blood Donations"); JButton afButton = new JButton("Add Donor"); JButton ufButton = new JButton("Update Donor"); JButton dlButton = new JButton("Delete Donor"); fkButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { ItemInfo ii=new ItemInfo(); try { ii.showButtonDemo(); } catch (SQLException ex) { Logger.getLogger(Frame2new.class.getName()).log(Level.SEVERE, null, ex); } } }); }
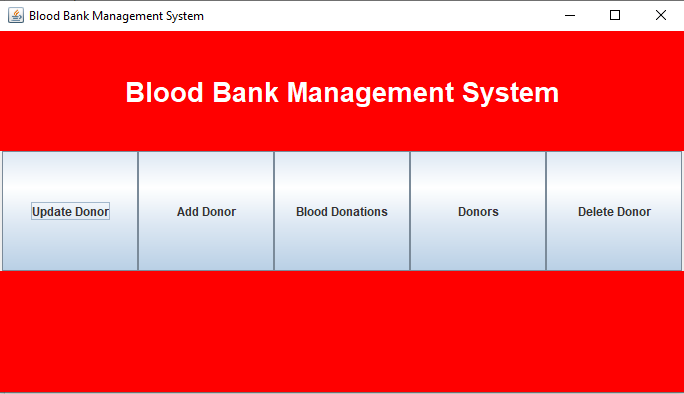
3. Add Donor module
This module helps to add a new donor to the Blood bank System. Name the file addDonor.java.
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class AddDonor { private JFrame mainFrame; private JLabel headerLabel; private JPanel controlPanel; private JLabel id,name,price,quantity; private static int count = 0; GridLayout experimentLayout = new GridLayout(0,2); ResultSet rs; public static void main(String[] args){ EnterDonor swingControlDemo = new EnterDonor(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Insert a new Donor!"); mainFrame.setSize(700,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.getContentPane().setBackground(Color.red); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ mainFrame.setVisible(false); } }); headerLabel = new JLabel("", JLabel.CENTER); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Blood Bank Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 27)); name = new JLabel("Enter Donor Name"); JTextField tf2=new JTextField(); tf2.setSize(100,40); price = new JLabel("Enter Blood Group"); JTextField tf3=new JTextField(); tf3.setSize(100,40); quantity = new JLabel("Enter Quantity"); JTextField tf4=new JTextField(); tf4.setSize(100,40); JButton okButton = new JButton("OK"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("insert into bloodbankmanagement(name,bloodgroup,quantity) values (?,?,?)"); pst.setString(1, tf2.getText()); pst.setDouble(2, Double.parseDouble(tf3.getText())); pst.setInt(3, Integer.parseInt(tf4.getText())); pst.execute(); JOptionPane.showMessageDialog(null, "Done Inserting " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); JOptionPane.showMessageDialog(null, "Inserting Error : " + tf2.getText()); }finally{ } okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); try{ pst.setDouble(2, Double.parseDouble(tf3.getText())); pst.setInt(3, Integer.parseInt(tf4.getText())); pst.execute(); JOptionPane.showMessageDialog(null, "Done Inserting " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); JOptionPane.showMessageDialog(null, "Inserting Error : " + tf2.getText()); } } }); JPanel jp = new JPanel(null); jp.add(name); jp.add(tf2); jp.add(price); jp.add(tf3); jp.add(quantity); jp.add(tf4); jp.setSize(500,500); jp.setLayout(experimentLayout); controlPanel.add(jp); jp.add(okButton); mainFrame.setLocationRelativeTo(null); mainFrame.setVisible(true); } }

4. Donor details Module
This module helps to view the donor details in the Blood Bank Management System Project in Java. Name the file DonorDetails.java.
import java.awt.*; import java.awt.event.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import javax.swing.*; public class DonorDetails { private JFrame mainFrame; private JLabel headerLabel; private JPanel controlPanel; public static void main(String[] args) throws SQLException{ ItemInfo swingControlDemo = new ItemInfo(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Donor Details"); mainFrame.setSize(700,400); mainFrame.getContentPane().setBackground(Color.red); mainFrame.setLayout(new FlowLayout()); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ } }); headerLabel = new JLabel("", JLabel.CENTER); controlPanel = new JPanel(); controlPanel.setSize(700,400); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); //mainFrame.add(statusLabel); mainFrame.setVisible(true); } public void showButtonDemo() throws SQLException{ headerLabel.setText("Blood Bank Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 25)); String[] columnNames = {"Donor ID","Donor Name", "Blood Group", "Quantity" }; Object[][] data = new Object[100][4]; PreparedStatement pst; ResultSet rs; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("select * from bloodbankmanagement"); rs = pst.executeQuery(); int i=0; while(rs.next()){ data[i][0] = rs.getInt("id"); data[i][1] = rs.getString("name"); data[i][2] = rs.getDouble("bloodgroup"); data[i][3] = rs.getInt("quantity"); i++; } mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("Error"); JOptionPane.showMessageDialog(null, "Error !"); } JTable table = new JTable(data, columnNames); table.setSize(400, 400); table.setVisible(true); // table.setEnabled(false); controlPanel.add(new JScrollPane(table, JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER)); //controlPanel.add(table); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); JTable table = new JTable(data, columnNames); table.setSize(400, 400); table.setVisible(true); // table.setEnabled(false); controlPanel.add(new JScrollPane(table, JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER)); //controlPanel.add(table); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } JLabel totalP = new JLabel(); Object data[][] = new Object[100][3]; int i = 0; ViewDonor(){ JPanel jp1 = new JPanel(); setBackground(Color.red); //jp1.getContentPane().setBackground(Color.orange); this.setLayout(new GridLayout(2,2)); JLabel a = new JLabel("Donor Name : "); jp1.add(a); JLabel b = new JLabel("Quantity : "); jp1.add(b); quantity = new JTextField(50); jp1.add(quantity); JButton ok = new JButton("OK"); JPanel jp2 = new JPanel(); jp2.setSize(700, 400); jp1.add(ok); ok.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); ResultSet rs; try{ pst = con.mkDataBase().prepareStatement("select f_prize from bloodbankmanagement where name = ?"); pst.setString(1, f.getText()); rs = pst.executeQuery(); } catch(Exception ex){ System.out.println(ex); } } }); // jp2.setLayout(new GridLayout(1,1)); jp2.setLayout(new FlowLayout()); jp2.add(totalP); jp2.add(new JScrollPane(cart, JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER)); addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { int count = 1; double vat = 15; } }); this.add(jp1); this.add(jp2); this.setSize(600,550); this.setLocationRelativeTo(null); this.setVisible(true); } } }

5. Update the Donor Module
This module helps to update the donor details in the Blood Bank Management System. Name the file updateDonor.java.
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class UpdatDonor { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; private JLabel id,name,price,quantity; private static int count = 0; GridLayout experimentLayout = new GridLayout(0,2); ResultSet rs; public static void main(String[] args){ UpdateDonor swingControlDemo = new UpdateDonor(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Update Donor Details"); mainFrame.setSize(700,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.getContentPane().setBackground(Color.red); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ mainFrame.setVisible(false); } }); headerLabel = new JLabel("", JLabel.CENTER); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,400); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Blood Bank Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 27)); headerLabel.setForeground(Color.white); name = new JLabel("Enter Donor Name"); JTextField tf2=new JTextField(); tf2.setSize(100,30); price = new JLabel("Enter Blood Group"); JTextField tf3=new JTextField(); tf3.setSize(100,30); quantity = new JLabel("Enter Quantity"); JTextField tf4=new JTextField(); tf4.setSize(100,30); JButton okButton = new JButton("UPDATE"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("UPDATE bloodbankmanagement SET quantity= ?, bloodgroup=? where name = ?"); pst.setString(3, tf2.getText()); pst.setDouble(2, Double.parseDouble(tf3.getText())); pst.setInt(1, Integer.parseInt(tf4.getText())); pst.execute(); JOptionPane.showMessageDialog(null, "Done Updating " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("Error"); JOptionPane.showMessageDialog(null, "Inserting Error : " + tf2.getText()); }finally{ } } }); JPanel jp = new JPanel(); jp.add(name); jp.add(tf2); jp.add(bloodgroup); jp.add(tf3); jp.add(quantity); jp.add(tf4); jp.setSize(200,200); jp.setLayout(experimentLayout); controlPanel.add(jp); jp.add(okButton); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } }

6. Delete the Donor Module
This module helps to delete the donor from the blood bank management system in Java. Name the file deleteDonor.java.
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.logging.Level; import java.util.logging.Logger; public class DeleteDonor { private JFrame mainFrame; private JLabel headerLabel; private JPanel controlPanel; private JLabel id,name,price,quantity; private static int count = 0; GridLayout experimentLayout = new GridLayout(1,1); ResultSet rs; public static void main(String[] args){ UpdateDonor swingControlDemo = new UpdateDonor(); swingControlDemo.showButtonDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Delete Donor Details"); mainFrame.setSize(700,400); mainFrame.getContentPane().setBackground(Color.red); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ mainFrame.setVisible(false); try{ pst = con.mkDataBase().prepareStatement("DELETE FROM bloodbankmanagement where name = ?"); pst.setString(1,tf2.getText()); pst.execute(); JOptionPane.showMessageDialog(null, "Item Deleted " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("EEEE"); JOptionPane.showMessageDialog(null, "Item not found : " + tf2.getText()); }finally{ } } }); headerLabel = new JLabel("", JLabel.CENTER); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.setVisible(true); } public void showButtonDemo(){ headerLabel.setText("Blood Bank Management System"); headerLabel.setFont(new Font(null, Font.BOLD, 27)); headerLabel.setForeground(Color.white); name = new JLabel("Enter Donor Name"); JTextField tf2=new JTextField(); tf2.setSize(100,30); JButton okButton = new JButton("DELETE"); okButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { PreparedStatement pst; DBConnection con = new DBConnection(); try{ pst = con.mkDataBase().prepareStatement("DELETE FROM bloodbankmanagement where name = ?"); pst.setString(1,tf2.getText()); pst.execute(); JOptionPane.showMessageDialog(null, "Item Deleted " + tf2.getText()); mainFrame.setVisible(false); }catch(Exception ex){ System.out.println(ex); System.out.println("EEEE"); JOptionPane.showMessageDialog(null, "Item not found : " + tf2.getText()); }finally{ } } }); JPanel jp = new JPanel(); jp.add(name); jp.add(tf2); jp.setSize(700,400); jp.setLayout(experimentLayout); controlPanel.add(jp); jp.add(okButton); mainFrame.setVisible(true); mainFrame.setLocationRelativeTo(null); } }
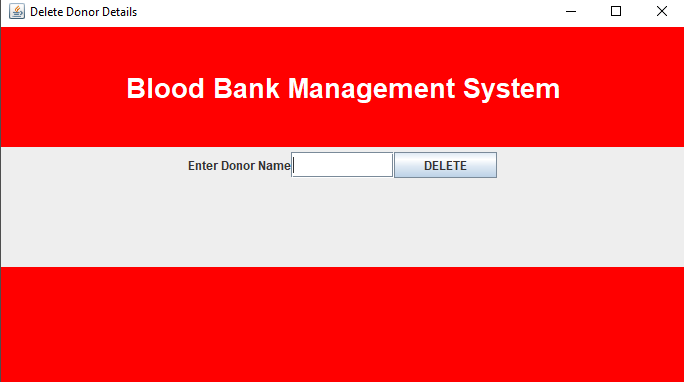
Video Output for Blood Bank Management System
Conclusion
In this project, we developed a GUI-based project, a Blood Bank Management System Project in Java and MySQL. The users are able to perform the operations such as Login, Adding donor details, updating donor details, viewing donor details, and deleting donor details. I hope you enjoyed doing this project!
Thank you for visiting our website.
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023
- Snake Game in Java