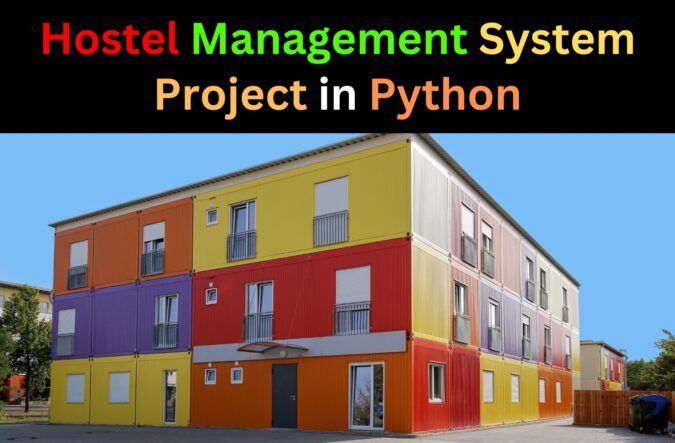
In this article, we will build a Hostel Management System Project in Python with Graphical User Interface and database integration. We will use the MongoDB database with pymongo module for managing data. This application can help hostel owners to make their work more convenient and Increase their Service Quality. This application can help users with adding rooms, booking rooms, adding or removing employees, etc.
Prerequisites
Following are the prerequisites for following along with this article:
- Python Programming Language
- tkinter module
- Pymongo Module
- MongoDB database
Features
The Hostel Manager can log in using credential admin as username and admin as password. He can change the username and password as well.
The manager has access to the following things:
- View all rooms, Add a room, Remove a room, and Book a room.
- View all employees, Add and Remove them.
- View all the feedback and add new feedback when required.
- Can change the username and password for login credentials.
- Can log out.
Set Up The Development Environment
- You should have MongoDB installed. MongoDB compass should also be installed to have more control over data using GUI provided by MongoDB.
- You should have pymongo module installed. Run “pip install pymongo” in the command prompt.
- Create three files main.py, gui.py, and database.py in the same folder.
Setting up the database
Copy the following code in the database.py file
from pymongo import MongoClient class DataBaseManager(): def __init__(self): """ Class to manage database """ self.client = MongoClient('localhost', 27017) self.db = self.client["Hostel_Management_System"] self.RoomsCollection = self.db["rooms"] self.AdminCollection = self.db["admins"] self.StudentCollection = self.db["students"] self.FeedBackCollection = self.db["feedBacks"] self.EmployeeCollection = self.db["employees"] def add_room(self,dict): dict["aviliability"] = "aviliable" dict["bookedfor"] = 0 self.RoomsCollection.insert_one(dict) def book_room(self,dict): doc = self.RoomsCollection.find_one({"Room Id":dict["Room Id"]}) doc["aviliability"] = "Occupied By " + dict["Name"] doc["bookedfor"] = dict["Booked For"] print(doc) self.RoomsCollection.find_one_and_replace({"Room Id":dict["Room Id"]},doc) self.StudentCollection.insert_one(dict) def check_authentication(self,username,password): dbusername = self.AdminCollection.find_one()["username"] dbpassword = self.AdminCollection.find_one()["password"] return username == dbusername and password == dbpassword def change_authentication_details(self,newusername,newpassword): doc = dict(self.AdminCollection.find_one()) doc["username"] = newusername doc["password"] = newpassword self.AdminCollection.find_one_and_replace({},replacement=doc) def insert_feedback(self,dict1): self.FeedBackCollection.insert_one(dict1) def get_feedbacks(self): feedbacks = [] for document in self.FeedBackCollection.find(): feedbacks.append(document) return feedbacks def get_employees(self): employees = [] for document in self.EmployeeCollection.find(): employees.append(document) return employees def get_students(self): students = [] for document in self.StudentCollection.find(): students.append(document) return students def get_rooms(self): rooms = [] for document in self.RoomsCollection.find(): rooms.append(document) return rooms def add_employee(self,dict1): self.EmployeeCollection.insert_one(dict1)
The above class manages getting, inserting, and modifying required data.
We have created Hostel_Management_System database and four collections inside the database which are
- RoomsCollection(For containing data regarding rooms)
- AdminCollection(For containing the username, and password for login)
- StudentCollection(For containing data regarding students)
- FeedbackCollection(For containing data regarding feedback and who gave them)
- EmployeeColleciton(For containing data regarding employees)
The methods in this class are pretty straightforward and self-explanatory as they do what they are called so there is no need to explain them.
Coding Hostel Management System Project in Python
gui.py
Copy the following code in gui.py
from tkinter import * from tkinter.scrolledtext import ScrolledText class Base(): """ Class to be inheritated by all the widgets in gui for uniformity """ background = "#191919" foreground = "#ECDBBA" labelframefont = "halvatica 10" normalfont = "halvatica 30" normalfont2 = "halvatica 10 " entryforeground = "#ECDBBA" entrybackground = "#C84B31" buttonbackground = "#2D4263" buttonforeground = "#ECDBBA" def Create_Welcome_Label(self,master): """ Shows welcome label """ Label(master,text="Hostel Management System",foreground=self.foreground,background=self.background,font="Halvatica 50").pack(pady = "30")
The above code starts the gui.py. We first import tkinter and scrolled text and then we create a Base class. This class will be inherited by all the classes in the GUI. This class contains background color, foreground color, and what fonts to use, etc for uniformity.
Creating Login Frame
Copy the following code in gui.py
class LoginFrame(LabelFrame,Base): def __init__(self,master): """ Class for handling login page in gui Inheritated from labelframe """ super().__init__(master=master,background=self.background,foreground = self.foreground, font=self.labelframefont,text="Login") self.Create_Welcome_Label(self.master) self.loginbutton,self.usernameentry,self.passwordentry = self.Create_Entrys_And_Buttons() def Create_Entrys_And_Buttons(self): """ creates entrys buttons and returnes necessary ones :return loginbutton,usernameentry,passwordentry) """ usernameentry = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont) passwordentry = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font = self. normalfont,show="*") Label(self,text="Username : ",foreground=self.foreground,background= self.background,font=self.normalfont).grid(row=0,column=0,padx=20,pady=5) Label(self,text="Password : ",foreground=self.foreground,background= self.background,font=self.normalfont).grid(row=1,column=0,padx=20,pady=5) usernameentry.grid(row=0,column=1,padx=20,pady=5,sticky=EW) passwordentry.grid(row=1,column=1,padx=20,pady=5,sticky=EW) self.rowconfigure((0,1),weight=1) self.columnconfigure((0,1),weight=1) loginbutton =Button(self,text="Login",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont,) loginbutton.grid(row = 2,column=0,columnspan=2,padx=20,pady=5,sticky='ew') return loginbutton,usernameentry,passwordentry def set_logincommand(self,command): self.loginbutton["command"] = lambda : command(self.usernameentry.get(),self.passwordentry.get()) def rebuild(self): """ Funtion to clear entrys after log out """ self.usernameentry.delete(0,END) self.passwordentry.delete(0,END)
This frame of Hostel Management System Project in Python handles the first login page. The rebuild function clears all the entries in the login page after logging in so that when the user logs out again the same text is not shown in the entry again.
Output:
Creating mainframe
Copy the following code in gui.py
class MainFrame(LabelFrame,Base): def __init__(self,master): """ Class to create MainPage of the app """ super().__init__(master=master,background=self.background,font=self.labelframefont,text="Functions",labelanchor="n",foreground=self.foreground) self.master = master self.create_buttons_and_welcome_label() def create_buttons_and_welcome_label(self): self.roommanagementbutton = Button(self,text="Room Management",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont,) self.feedbackmanagementbutton = Button(self,text="FeedBack Management",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont,) self.empolyeemanagementbutton = Button(self,text="Employee Management",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont,) self.viewstudentbutton = Button(self,text="View Students",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont,) self.changeUPbutton = Button(self,text="Change Username/Password",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont,) self.logoutbutton = Button(self,text="Log-out",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont,) self.buttons = (self.roommanagementbutton,self.feedbackmanagementbutton,self.empolyeemanagementbutton,self.viewstudentbutton,self.changeUPbutton,self.logoutbutton) for i in self.buttons: i.pack(expand=True,fill=X,pady=5,padx = 7) def pack1(self,*arr): self.Create_Welcome_Label(self.master) self.pack(*arr) def set_command_for_button(self,buttoncode,command): """ Class to set commands for button. :param buttoncode: (int) code to distinguish number and button :param command: function """ self.buttons[buttoncode]["command"] = command
This frame of Hostel Management System Project in Python handles the main page of the GUI. Method set command for button sets the command for the button according to the index passed.
Creating change Username Password Top Level window
This class creates a top level for changing usernames and passwords.
Copy the following code in gui.py
class ChangeUsernamePassword(Toplevel,Base): def __init__(self): """ Class to handle gui for changing usernames and passwords """ super().__init__(background=self.background) self.title = "ChangeUsernamePassword" self.changebutton,self.oldusernameentry,self.oldpasswordentry,self.newusernameentry,self.newpasswordentry = self.create_entrysandbuttons() def create_entrysandbuttons(self): """ Creates buttons and entries and returns some of them :return changebutton,oldusernameentry,oldpasswordentry,newusernameentry,newpasswordentry: """ oldpasswordentry = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font = self. normalfont2,show="*") oldusernameentry = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont2) newusernameentry = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont2) newpasswordentry = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font = self. normalfont2,show="*") Label(self,text="OldUsername : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=0,column=0,padx=20,pady=5) Label(self,text="NewUsername : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=1,column=0,padx=20,pady=5) Label(self,text="OldPassword : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=2,column=0,padx=20,pady=5) Label(self,text="NewPassword : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=3,column=0,padx=20,pady=5) oldusernameentry.grid(row=0,column=1,padx=20,pady=5,sticky=EW) newusernameentry.grid(row=1,column=1,padx=20,pady=5,sticky=EW) oldpasswordentry.grid(row=2,column=1,padx=20,pady=5,sticky=EW) newpasswordentry.grid(row=3,column=1,padx=20,pady=5,sticky=EW) changebutton =Button(self,text="Change",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont2,) changebutton.grid(row = 4,column=0,columnspan=2,padx=20,pady=5,sticky='ew') return changebutton,oldusernameentry,oldpasswordentry,newusernameentry,newpasswordentry def set_changebuttoncommand(self,command): self.changebutton["command"] = lambda : command(self.oldusernameentry.get(),self.oldpasswordentry.get(),self.newusernameentry.get(),self.newpasswordentry.get())
Output:
Creating Feedback Manager
Copy the following code in gui.py
class FeedbackManager(Frame,Base): def __init__(self): """ Class to display and add feedbacks """ super().__init__(background= self.background) self.create_widgets() def create_widgets(self): L1 = LabelFrame(self,font=self.labelframefont,background=self.background,foreground=self.foreground,text="FeedBacks") old_feedbacks = ScrolledText(L1,background = self.entrybackground,foreground = self.entryforeground,font = self.normalfont2) self.old_feedbacks = old_feedbacks self.old_feedbacks['state'] = DISABLED self.old_feedbacks.pack(fill = "both",expand=True) L1.grid(row=0,column=0, sticky= NSEW,rowspan=2,padx=5,pady=5) L2 = LabelFrame(self,font=self.labelframefont,background=self.background,foreground=self.foreground,text="AddFeedBacks") self.add_feedback_section = ScrolledText(L2,background = self.entrybackground,foreground = self.entryforeground,font = self.normalfont2) self.add_feedback_section.pack(fill=BOTH,expand=True) L2.grid(row = 0,column = 1,sticky= NSEW,columnspan=4,padx=4,pady=4) self.addfeedbackbutton = Button(self,text="AddFeedBack",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.addfeedbackbutton.grid(row = 1,column=3,sticky= NSEW,padx=2,pady=2) self.backbutton = Button(self,text="Back",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.backbutton.grid(row = 1,column=4,sticky="news",padx = 2,pady=2) Label(self,text="Given By : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=1,column=1,sticky="news") self.givenbyentry = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font = self. normalfont2) self.givenbyentry.grid(row = 1,column= 2,sticky="news") self.rowconfigure(0,weight=3) self.columnconfigure(0,weight=1) def display_feedbacks(self,feedbacks): """ displays the feedbacks :param feedbacks: list """ self.old_feedbacks["state"] = NORMAL for feedback in feedbacks: self.old_feedbacks.insert(END,"Given By : " + feedback["name"] + "\n") self.old_feedbacks.insert(END, feedback["feedbacktext"] + "\n") self.old_feedbacks["state"] = DISABLED def set_commandforaddfeedbackbuttons(self,command): """ :param command: function """ self.addfeedbackbutton["command"] = lambda : command({"name": self.givenbyentry.get(),"feedbacktext" : self.add_feedback_section.get(0.0,END)}) def set_commandforbackbutton(self,command): """ :param command: function """ self.backbutton["command"] = command
The feedback manager manages adding feedbacks and viewing previous feedbacks.
Output:
Creating Employee Manager
Copy the following code in gui.py
class EmployeeManager(LabelFrame,Base): def __init__(self,master): """ Class to view Employees and add or remove employees """ super().__init__(master=master,background=self.background,foreground = self.foreground, font=self.labelframefont,text="Employees : ") self.create_widgets() def create_widgets(self): self.viewemployees = ScrolledText(self,background = self.entrybackground,foreground = self.entryforeground,font = self.normalfont2) self.viewemployees.grid(row=0,column=0,columnspan = 3,sticky="news") self.viewemployees["state"] = DISABLED self.backbutton = Button(self,text="Back",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.backbutton.grid(row = 1,column=2,sticky="news",padx = 2,pady=2) self.addempolyeebutton = Button(self,text="AddEmployee",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.addempolyeebutton.grid(row = 1,column=0,sticky="news",padx = 2,pady=2) self.removeempolyeebutton = Button(self,text="RemoveEmployee",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.removeempolyeebutton.grid(row = 1,column=1,sticky="news",padx = 2,pady=2) self.rowconfigure(0,weight=1) self.columnconfigure((0,1,2),weight=1) def display_employees(self,employees): """ displays the feedbacks :param rooms: list """ self.viewemployees["state"] = NORMAL for employee in employees: for key,value in employee.items(): try: self.viewemployees.insert(END,key + " : " + value + "\n") except: pass self.viewemployees.insert(END,"\n") self.viewemployees["state"] = DISABLED def set_commandforbackbutton(self,command): """ :param command: function """ self.backbutton["command"] = command def set_commandforaddemployees(self,command): self.addempolyeebutton["command"] = command def set_commandforremoveemployees(self,command): self.removeempolyeebutton["command"] = command
Employee Manager lets you add employees, view employees, and remove employees.
Output:
It has Add employee and Remove employee Buttons they will create add_employee and remove_employee top levels.
Creating add employee top level
Copy the following code in gui.py
class addemployeetoplevel(Toplevel,Base): def __init__(self): """ Top Level To add employees """ super().__init__(background=self.background) self.required_values = ["Name","Email Address","Phone Number","Post","Qualification"] self.create_widgets() def create_widgets(self): entrys = [] for i in range(len(self.required_values)): Label(self,text=self.required_values[i] + " : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=i,column=0,sticky="news") e = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont) e.grid(row=i,column=1,sticky="news") entrys.append(e) self.entrys = entrys self.addemployeebutton =Button(self,text="Add",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont) self.addemployeebutton.grid(row=len(self.required_values) + 1,column=0,columnspan=2,sticky=NSEW) def set_addemployeebuttoncommand(self,command): dict = {} for i in range(len(self.required_values)): dict[self.required_values[i]] = self.entrys[i] self.addemployeebutton["command"] = lambda : command(dict)
It manages adding of employees.
Output:
Creating Remove Employee Toplevel
Copy the following code in gui.py
class removeempolyeetoplevel(Toplevel,Base): def __init__(self): """ Top Level To remove employees """ def __init__(self): """ Top Level To remove emoployee """ super().__init__(background=self.background) self.required_values = ["Name",] self.create_widgets() def create_widgets(self): entrys = [] for i in range(len(self.required_values)): Label(self,text=self.required_values[i] + " : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=i,column=0,sticky="news") e = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont) e.grid(row=i,column=1,sticky="news") entrys.append(e) self.entrys = entrys self.removeemployeebutton =Button(self,text="Remove",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont) self.removeemployeebutton.grid(row=len(self.required_values) + 1,column=0,columnspan=2,sticky=NSEW) def set_removeemployeebuttoncommand(self,command): dict = {} print("hell") for i in range(len(self.required_values)): dict[self.required_values[i]] = self.entrys[i] self.removeemployeebutton["command"] = lambda : command(dict[self.required_values[0]])
It handles removing of employees.
Output:
Creating room manager
The room manager module of Hostel Management System Project in Python handles adding rooms, viewing rooms, removing rooms, and booking rooms.
Copy the following code in gui.py
class RoomManager(LabelFrame,Base): def __init__(self,master): """ Class to view rooms , add remove or book rooms """ super().__init__(master=master,background=self.background,foreground = self.foreground, font=self.labelframefont,text="Rooms : ") self.create_widgets() def create_widgets(self): self.viewrooms = ScrolledText(self,background = self.entrybackground,foreground = self.entryforeground,font = self.normalfont2) self.viewrooms.grid(row=0,column=0,columnspan = 4,sticky="news") self.viewrooms["state"] = DISABLED self.backbutton = Button(self,text="Back",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.backbutton.grid(row = 1,column=3,sticky="news",padx = 2,pady=2) self.addroombutton = Button(self,text="AddRoom",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.addroombutton.grid(row = 1,column=0,sticky="news",padx = 2,pady=2) self.removeroombutton = Button(self,text="RemoveRoom",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.removeroombutton.grid(row = 1,column=1,sticky="news",padx = 2,pady=2) self.bookroombutton = Button(self,text="BookRoom",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.bookroombutton.grid(row = 1,column=2,sticky="news",padx = 2,pady=2) self.rowconfigure(0,weight=1) self.columnconfigure((0,1,2),weight=1) def display_rooms(self,rooms): """ displays the feedbacks :param rooms: list """ self.viewrooms["state"] = NORMAL for room in rooms: for key,value in room.items(): try: if key != "_id": self.viewrooms.insert(END,key + " : " + str(value) + "\n") except Exception as e: print(e) self.viewrooms.insert(END,"\n") self.viewrooms["state"] = DISABLED def set_commandforbackbutton(self,command): """ :param command: function """ self.backbutton["command"] = command def set_commandforaddroombutton(self,command): """ :param command: function """ self.addroombutton["command"] = command def set_commandforremoveroombutton(self,command): """ :param command: function """ self.removeroombutton["command"] = command def set_commandforbookroombutton(self,command): """ :param command: function """ self.bookroombutton["command"] = command
Output:
This page has add room button, remove room button, and book room button which
Will create add room toplevel, remove room toplevel, and book room toplevel respectively.
Creating Add Room Toplevel
Copy the following code in gui.py
class addroomtoplevel(Toplevel,Base): def __init__(self): """ Top Level To add employees """ super().__init__(background=self.background) self.required_values = ["Room Id","Room Description",] self.create_widgets() def create_widgets(self): entrys = [] for i in range(len(self.required_values)): Label(self,text=self.required_values[i] + " : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=i,column=0,sticky="news") e = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont) e.grid(row=i,column=1,sticky="news") entrys.append(e) self.entrys = entrys self.addroombutton =Button(self,text="Add",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont) self.addroombutton.grid(row=len(self.required_values) + 1,column=0,columnspan=2,sticky=NSEW) def set_addroombuttoncommand(self,command): dict = {} for i in range(len(self.required_values)): dict[self.required_values[i]] = self.entrys[i] self.addroombutton["command"] = lambda : command(dict)
It manages adding of rooms.
Output:
Creating remove room toplevel
Copy the following code in gui.py
class removeroomtoplevel(Toplevel,Base): def __init__(self): """ Top Level To add employees """ super().__init__(background=self.background) self.required_values = ["Room Id",] self.create_widgets() def create_widgets(self): entrys = [] for i in range(len(self.required_values)): Label(self,text=self.required_values[i] + " : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=i,column=0,sticky="news") e = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont) e.grid(row=i,column=1,sticky="news") entrys.append(e) self.entrys = entrys self.removeroombutton =Button(self,text="Remove",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont) self.removeroombutton.grid(row=len(self.required_values) + 1,column=0,columnspan=2,sticky=NSEW) def set_removeroombuttoncommand(self,command): self.removeroombutton["command"] = lambda : command(self.entrys[0])
It handles removing of rooms.
Output:
Creating book room top level
Copy the following code in gui.py
class bookroomtoplevel(Toplevel,Base): def __init__(self): """ Top Level To Book Room """ super().__init__(background=self.background) self.required_values = ["Room Id","Name","Email Address","Phone Number","Student Id","Booked For"] self.create_widgets() def create_widgets(self): entrys = [] for i in range(len(self.required_values)): Label(self,text=self.required_values[i] + " : ",foreground=self.foreground,background= self.background,font=self.normalfont2).grid(row=i ,column=0,sticky="news",padx=5,pady=5) e = Entry(self,foreground=self.entryforeground,background=self.entrybackground,font=self.normalfont) e.grid(row=i,column=1,sticky="news",padx=5,pady=5) entrys.append(e) self.entrys = entrys self.bookroombutton =Button(self,text="Book Room",background=self.buttonbackground,foreground=self.buttonforeground,font=self.normalfont) self.bookroombutton.grid(row=len(self.required_values),column=0,columnspan=2,sticky=NSEW) def set_bookroombuttoncommand(self,command): dict = {} for i in range(len(self.required_values)): dict[self.required_values[i]] = self.entrys[i] self.bookroombutton["command"] = lambda : command(dict)
This frame of Hostel Management System Project in Python lets you book the room for students.
Output:
Creating view student’s frame
This frame lets you view students and their details like phone numbers, Email Addresses, rooms in which they are staying and booked, etc.
Copy the following code in gui.py
class ViewStudentsFrame(LabelFrame,Base): def __init__(self,master): """ Class to view Students """ super().__init__(master=master,background=self.background,foreground = self.foreground, font=self.labelframefont,text="Students : ") self.create_widgets() def create_widgets(self): self.viewstudents = ScrolledText(self,background = self.entrybackground,foreground = self.entryforeground,font = self.normalfont2) self.viewstudents.grid(row=0,column=0,columnspan = 3,sticky="news") self.viewstudents["state"] = DISABLED self.backbutton = Button(self,text="Back",background=self.buttonbackground,foreground=self.buttonforeground,font = self.normalfont2) self.backbutton.grid(row = 1,column=2,sticky="news",padx = 2,pady=2) self.rowconfigure(0,weight=1) self.columnconfigure((0,1,2),weight=1) def display_students(self,students): """ displays the feedbacks :param rooms: list """ self.viewstudents["state"] = NORMAL for employee in students: for key,value in employee.items(): try: self.viewstudents.insert(END,key + " : " + value + "\n") except: pass self.viewstudents.insert(END,"\n") self.viewstudents["state"] = DISABLED def set_commandforbackbutton(self,command): """ :param command: function """ self.backbutton["command"] = command
Output:
Creating App Class
Finally, we create an app class that handles all the GUI activities like creating and packing room or employee manager, sending messages, etc
Copy the following code in gui.py
class App(Tk,Base): def __init__(self): """ Main class of gui which handles all UI activities """ super().__init__() self.title("Hostel Management System") self.geometry("1350x700") self.config(background=self.background) self.mainframe = MainFrame(self) self.loginframe = LoginFrame(self) self.feedbackmanager = FeedbackManager() self.employeemanager = EmployeeManager(self) self.roommanager = RoomManager(self) self.viewstudentsframe = ViewStudentsFrame(self) self.create_loginframe() def create_mainframe(self): self.mainframe.pack1() def create_loginframe(self): self.loginframe.pack(expand=True,fill=X,padx=300,ipadx=20,ipady=20) def create_feedbackmanager(self): self.feedbackmanager.pack(fill=BOTH,expand=True) def clear_window(self): """ clears all the widgets from the window """ for widget in self.pack_slaves(): widget.pack_forget() def create_changeusernamepassword(self): self.changeusernamepasswordtoplevel = ChangeUsernamePassword() def create_addemployeestoplevel(self): self.addemployeestoplevel = addemployeetoplevel() def create_removeemployeestoplevel(self): self.removeemployeestoplevel = removeempolyeetoplevel() def create_employeemanager(self): self.clear_window() self.employeemanager.pack(fill=BOTH,expand=True) def create_roommanager(self): self.clear_window() self.roommanager.pack(fill = BOTH,expand = True) def create_viewstudents(self): self.clear_window() self.viewstudentsframe.pack(fill = BOTH,expand = True) def create_addroomtoplevel(self): self.addroomtoplevel = addroomtoplevel() def create_removeroomtoplevel(self): self.removeroomtoplevel = removeroomtoplevel() def create_bookroomtoplevel(self): self.bookroomroplevel = bookroomtoplevel() def log_out(self): self.clear_window() self.loginframe.rebuild() self.create_loginframe() def log_in(self): self.clear_window() self.create_mainframe() def start_feedbackmanager(self): self.clear_window() self.create_feedbackmanager() def backtomainframe(self): self.clear_window() self.create_mainframe()
This class manages all the GUI activities. Some of the hard-to-understand methods of this class are:
- Back to the main frame: It clears other frames in the window and changes the frame to the main frame.
- Clear window.
It hides all the widgets of the window to change pages or frames.
Finally connecting the database and GUI through main.py
First, copy the following code in main.py
from gui import * from database import * from tkinter.messagebox import showinfo,askokcancel app = App() dbm = DataBaseManager() def login(username,password): if dbm.check_authentication(username=username,password=password): app.log_in() else: showinfo("Login Error","Invalid Username or password") def logout(): yes = askokcancel(title="Info",message="Are you sure you want to log out? ") if yes: app.log_out() def createusernamepassword(): app.create_changeusernamepassword() app.changeusernamepasswordtoplevel.set_changebuttoncommand(changeusernamepassword) def changeusernamepassword(oldusername,oldpassword,newusername,newpassword): if dbm.check_authentication(oldusername,oldpassword): dbm.change_authentication_details(newusername,newpassword) showinfo(title="Changed",message="Changed Username and Password") app.changeusernamepasswordtoplevel.destroy() def start_feedback(): app.start_feedbackmanager() app.feedbackmanager.display_feedbacks(dbm.get_feedbacks()) def add_feedback(dict): dbm.insert_feedback(dict) def add_employees(dict): dict_2 = {} for key,value in dict.items(): dict_2[key] = value.get() print(dict_2) dbm.add_employee(dict_2) app.addemployeestoplevel.destroy() def remove_employee(entry): dbm.EmployeeCollection.delete_one({"Name":entry.get()}) app.removeemployeestoplevel.destroy() def remove_room(entry): dbm.RoomsCollection.delete_one({"Room Id":entry.get()}) app.removeroomtoplevel.destroy() def add_room(dict): dict_2 = {} for key,value in dict.items(): dict_2[key] = value.get() dbm.add_room(dict_2) app.addroomtoplevel.destroy() def book_room(dict): dict_2 = {} for key,value in dict.items(): dict_2[key] = value.get() dbm.book_room(dict_2) app.bookroomroplevel.destroy() def create_addemployees_toplevel(): app.create_addemployeestoplevel() app.addemployeestoplevel.set_addemployeebuttoncommand(add_employees) def create_removeemployees_toplevel(): app.create_removeemployeestoplevel() app.removeemployeestoplevel.set_removeemployeebuttoncommand(remove_employee) def create_employeemanager(): app.create_employeemanager() app.employeemanager.display_employees(dbm.get_employees()) def create_addroomtoplevel(): app.create_addroomtoplevel() app.addroomtoplevel.set_addroombuttoncommand(add_room) def create_removeroomtoplevel(): app.create_removeroomtoplevel() app.removeroomtoplevel.set_removeroombuttoncommand(remove_room) def create_bookroomtoplevel(): app.create_bookroomtoplevel() app.bookroomroplevel.set_bookroombuttoncommand(book_room) def create_roommanager(): app.create_roommanager() app.roommanager.display_rooms(dbm.get_rooms()) def create_viewstudents(): app.create_viewstudents() app.viewstudentsframe.display_students(dbm.get_students()) app.loginframe.set_logincommand(login) app.mainframe.set_command_for_button(5,logout) app.mainframe.set_command_for_button(4,createusernamepassword) app.mainframe.set_command_for_button(1,start_feedback) app.mainframe.set_command_for_button(2,create_employeemanager) app.mainframe.set_command_for_button(0,create_roommanager) app.mainframe.set_command_for_button(3,create_viewstudents) app.feedbackmanager.set_commandforaddfeedbackbuttons(add_feedback) app.feedbackmanager.set_commandforbackbutton(lambda : app.backtomainframe()) app.employeemanager.set_commandforbackbutton(lambda : app.backtomainframe()) app.viewstudentsframe.set_commandforbackbutton(lambda: app.backtomainframe()) app.roommanager.set_commandforbackbutton(lambda : app.backtomainframe()) app.roommanager.set_commandforremoveroombutton(create_removeroomtoplevel) app.roommanager.set_commandforaddroombutton(create_addroomtoplevel) app.roommanager.set_commandforbookroombutton(create_bookroomtoplevel) app.employeemanager.set_commandforaddemployees(create_addemployees_toplevel) app.employeemanager.set_commandforremoveemployees(create_removeemployees_toplevel) app.mainloop()
In this file, we connect the database with the app. First, we create the objects of the database and app and we create many functions which are commands to be run by the buttons on the app. In the last, we set the commands to the appropriate function and run the mainloop. This completes the Hostel management app.
Output:
Download Source Code
Conclusion
We have completed the Hostel Management System Project in Python. It was a nice project for me to practice my GUI and database skills. Hope it was for you too. Don’t hesitate to modify the app as you need and always try to create something fun like this project.
Thank you for visiting our website.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter