
In this tutorial, we are going to learn about the Yield keyword in Python. It works like a return statement we used in functions. The yield returns a generator that works as an iterator.
Let us understand the syntax of Yield
def generator_y(x): for i in range(x): yield i
Syntax defines, we have function generator_y() where we pass the value x, The function for loop will generate or yield the value i for the range x.
Use of Yield Keyword
As the yield returns the generator that can be iterated to extract the values, it can be done by using the next() or for loop function. It’s a memory-efficient function.
Python continues to run the code for each iteration till it encounters the yield statement. It then sends the yield value to the function keeping it in the same state without exiting.
When the function is called the next time, it remembers the state of the execution and resumes the execution where it is stopped. It continues till the generator is not exhausted.
Difference between return and yield
Return | Yield |
Returns the result to the called function | It converts a function to a generator. Suspends the function and preserves its state |
It destroys the variables once execution is completed | The yield keyword does not destroy local variables of the function. |
There is one return statement for a function | There can be one or more yield statements in a function. |
The execution of the Function starts from the beginning if we are trying to execute it again. | In the case of the yield keyword, the execution of the function resumes where it was previously paused |
Lets us see the example of how the generator works along with the yield
Example 1
# Function returns a generator when it encounters 'yield'.
def example_generator():
x = 1
yield x
yield x + 1
yield x + 2
gen_obj = example_generator()
for i in gen_obj:
print(i)
Output:
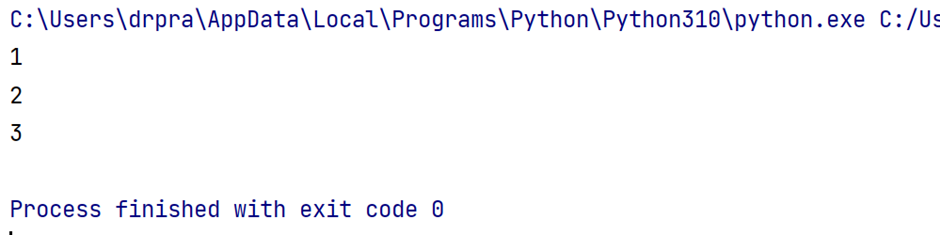
In this example, we have created the generator object gen_obj but it will iterate only once, if we try calling the generator a second time it will not give the output as the generator gets exhausted.
The solution for this problem is we have to convert this into the class that implements a ‘__iter()__’ method.
Example 2
class NumberIteration(object):
def __iter__(self):
x = 1
yield x
yield x + 1
yield x + 2
numberiteration = NumberIteration()
# created iterator twice
for i in numberiteration:
print(i)
for i in numberiteration:
print(i)
Output:
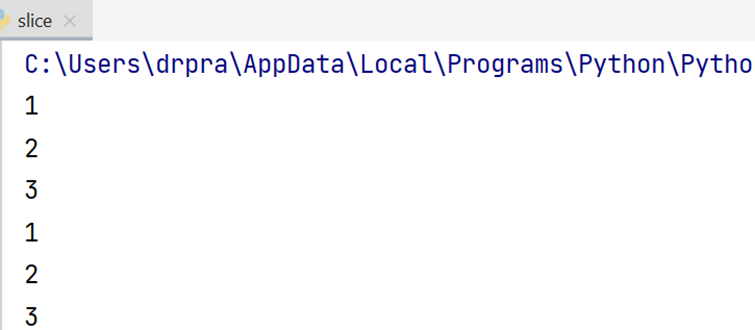
Generator function next() and yield keyword in Python
def newfun_generator():
yield "Welcome!"
yield "To Copyassignment.com"
funobj = newfun_generator()
print(type(funobj))
print(next(funobj))
print(next(funobj))
Output:

Working of Yield with list Function
#func to find the odd numbers from the list
sample_list = [1,2,3,4,5,6,7,8]
def print_oddnumbers(sample_list):
for i in sample_list:
if i % 2 != 0:
yield i
# printing odd numbers
print("The odd numbers in list are : ", end=" ")
for n in print_oddnumbers(sample_list):
print(n, end=" ")
Output:

Working of yield with split function
The split function is used in python to split the numbers and words or letters. Here we are using it with the yield function to get the count of the words.
# function to count the number of words
Sample_string= " Here we are to learn many things,\
Here we are to learn python"
def Yield_func(Sample_string):
for i in Sample_string:
if i == "Here":
yield i
num = 0
print("The count is : ", end="")
Sample_string = Sample_string.split()
for x in Yield_func(Sample_string):
num = num + 1
print(num)
Output:

Conclusion
In this article, we came to know the working of the yield keyword in Python step by step, it returns from the function without destroying the local variables state as well as protects the functions from exiting until the next() function is called and starts again the execution from the point it was halted.
Click here to discuss more on Yield Keyword.
Thank you for visiting our website
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter