
In this article, we are discussing the project Attendance Management System Project in JAVA and MySQL. This article is best for beginners looking to improve their skills. In the project, three levels of users are present, admins, teachers, and students. Admin can add and edit the teachers, students, and attendance. Teachers can add and edit the attendance only. Students can only view it.
Attendance Management System Project In Java: Project Overview
Project Name: | Attendance Management System Project in Java |
Abstract: | It’s a GUI-based project used with the Swing module to manage the attendance of an Institution. |
Language/s Used: | Java |
IDE: | Eclipse IDE for Java Developers |
Java version (Recommended): | Java SE 18.0. 2.1 |
Database: | MySQL |
Type: | Desktop Application |
Recommended for: | Final Year Students |
Setting Up the environment
Make sure JDK is present in your system. It can be installed using the IDE you are using.
The first step is to create a project in the name you wish. Create a package named “Attendance“. We are creating java classes in this package.
To connect the project to the database, follow the steps:
- Make sure MySQL is installed on your system.
- Download the MySQL connector from here.
- Open the properties of the project from the dropdown list which appears on right-click on the project. Navigate to the libraries tab and click on the classpath. Select “Add External JARs…”. Select the file you downloaded. This step will differ on IDE you are using.
MySQL Setup for Attendance Management System Project In Java
1. Creating the database
-- Creating database
create database attendance;
2. Selecting the database
-- Selecting the database
use attendance;
3. Creating the user table
-- User Table
CREATE TABLE user(id int primary key, username varchar(25), name varchar(25), password varchar(25), prio int);
4. Creating the class table
-- Class Table
CREATE TABLE class(id int primary key, name varchar(25));
5. Creating the student table
-- Student Table
CREATE TABLE students(id int primary key, name varchar(25), class varchar(10));
6. Creating the teacher’s table
-- Teachers Table
CREATE TABLE teachers(id int primary key, name varchar(25));
7. Creating the attendance table
-- Attend Table
CREATE TABLE attend(stid int, dt date, status varchar(15), class varchar(15));
8. Adding the First user to get logged in
-- Creating admin user
INSERT INTO user VALUES(1, 'admin', 'Admin', 'admin', 1);
Coding Attendance Management System Project In Java
1. Main Module
File Name: “Main.java“
This module contains the main function. ie, the execution of the program begins here. This calls the login Module.
package Attendance; import java.sql.SQLException; public class Main { public static void main(String[] args) throws SQLException { Login login = new Login(); login.loginView(); } }
2. Login Module
File Name: “Login.java“
This module creates a login page in which the user can enter the username and password to log in. All the admins, teachers, and students can use this to log in.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JPasswordField; import javax.swing.JTextField; import java.sql.*; public class Login{ int usr = 0; public void loginView() { JFrame frame = new JFrame(); Font text = new Font("Times New Roman", Font.PLAIN, 20); Home hm = new Home(); TeacherView tview = new TeacherView(); StudentView sview = new StudentView(); //-------------------------LOGO-------------------------- JLabel attendance = new JLabel("ATTENDANCE"); attendance.setForeground(Color.decode("#37474F")); attendance.setBounds(100, 275, 400, 50); attendance.setFont(new Font("Verdana", Font.BOLD, 50)); frame.add(attendance); JLabel management = new JLabel("MANAGEMENT SYSTEM"); management.setForeground(Color.decode("#37474F")); management.setBounds(280, 310, 400, 50); management.setFont(new Font("Verdana", Font.BOLD, 15)); frame.add(management); //------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 500, 600); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#DEE4E7")); x.setBounds(965, 20, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------MINIMIZE----------------------------- JLabel min = new JLabel("_"); min.setForeground(Color.decode("#DEE4E7")); min.setBounds(935, 10, 100, 20); min.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(min); min.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.setState(JFrame.ICONIFIED); } }); //------------------------------------------------------------- //--------------------------LOGINTEXT--------------------------- JLabel lgn = new JLabel("LOGIN"); lgn.setForeground(Color.decode("#DEE4E7")); lgn.setBounds(625, 100, 350, 75); lgn.setFont(new Font("Times New Roman", Font.BOLD, 75)); frame.add(lgn); //--------------------------------------------------------------- //-------------------------USER-------------------------- JLabel user = new JLabel("Username"); user.setForeground(Color.decode("#DEE4E7")); user.setBounds(570, 250, 100, 20); user.setFont(text); frame.add(user); //------------------------------------------------------- //-----------------------USERFIELD----------------------- JTextField username = new JTextField(); username.setBounds(570, 285, 360, 35); username.setBackground(Color.decode("#DEE4E7")); username.setForeground(Color.decode("#37474F")); username.setFont(new Font("Times New Roman", Font.BOLD, 15)); frame.add(username); //--------------------------------------------------------- //-------------------------Password-------------------------- JLabel pass = new JLabel("Password"); pass.setForeground(Color.decode("#DEE4E7")); pass.setBounds(570, 350, 100, 20); pass.setFont(text); frame.add(pass); //------------------------------------------------------- //-----------------------PASSWORDFIELD----------------------- JPasswordField password = new JPasswordField(); password.setBounds(570, 385, 360, 35); password.setBackground(Color.decode("#DEE4E7")); password.setForeground(Color.decode("#37474F")); frame.add(password); //--------------------------------------------------------- //-------------------------WARNING-------------------------- JLabel warning = new JLabel(); warning.setForeground(Color.RED); warning.setBounds(625, 450, 250, 20); warning.setHorizontalAlignment(warning.CENTER); frame.add(warning); //------------------------------------------------------- //----------------------LOGIN---------------------------- JButton login = new JButton("LOGIN"); login.setBounds(625, 500, 250, 50); login.setFont(new Font("Times New Roman", Font.BOLD, 20)); login.setBackground(Color.decode("#DEE4E7")); login.setForeground(Color.decode("#37474F")); frame.add(login); login.addActionListener(new ActionListener() { @SuppressWarnings("deprecation") @Override public void actionPerformed(ActionEvent e) { try { int res = dbCheck(username.getText(), password.getText()); if(res == 0) { warning.setText("NO USER FOUND!!!"); username.setText(""); password.setText(""); } else if(res == -1) { warning.setText("WRONG PASSWORD!!!"); username.setText(""); password.setText(""); } else { if(res == 1) hm.homeView(usr); else if(res == 2) tview.tcView(usr); else if (res == 3) sview.stView(usr); frame.dispose(); } } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public int dbCheck(String name, String password) throws SQLException { //ENTER PORT, USER, PASSWORD. String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; String str = "SELECT * FROM user WHERE username = '" + name + "'"; Connection con = DriverManager.getConnection(url, user, pass); Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); if(rst.next()) { if(rst.getString("password").equals(password)) { usr = rst.getInt("id"); return rst.getInt("prio"); } else return -1; } else { return 0; } } }
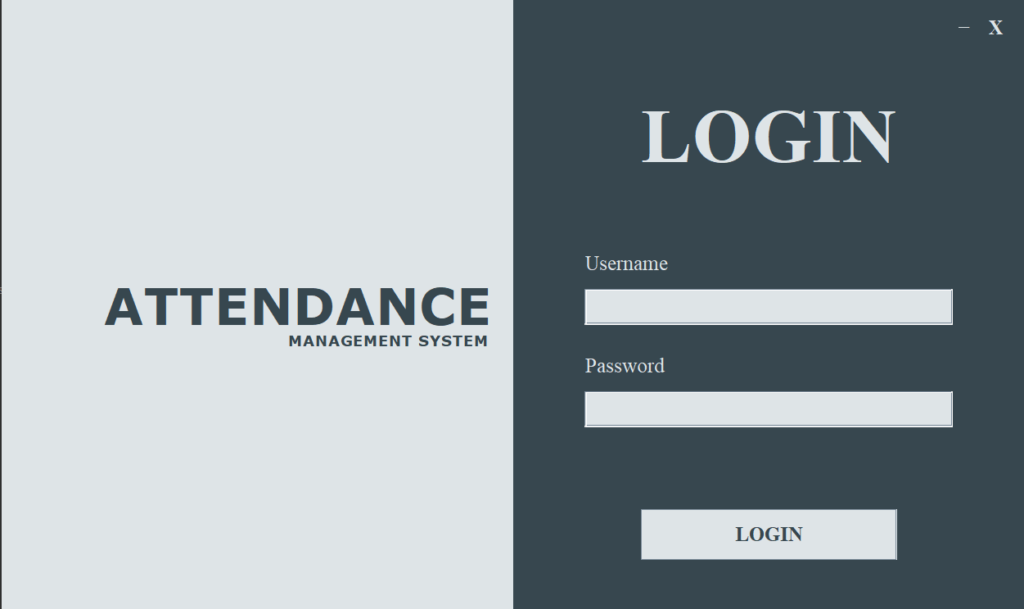
You can enter your username and password to log in. If Something went wrong, it will be displayed below.

Once the Login credentials get right, you will be taken to the homepage.
3. Home Page for admins
File Name: “Home.java“
On login, admins are taken to this page. We have options to add, edit and delete the admins, teachers, students, and attendance.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; public class Home{ public void homeView(int id) throws SQLException { JFrame frame = new JFrame(); Font btn = new Font("Times New Roman", Font.BOLD, 20); Admin adm = new Admin(); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------MINIMIZE----------------------------- JLabel min = new JLabel("_"); min.setForeground(Color.decode("#37474F")); min.setBounds(935, 0, 100, 20); frame.add(min); min.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.setState(JFrame.ICONIFIED); } }); //------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //-------------------Welcome--------------------------------- JLabel welcome = new JLabel("Welcome "+getUser(id)+","); welcome.setForeground(Color.decode("#DEE4E7")); welcome.setBounds(10, 50, 250, 20); welcome.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(welcome); //----------------------------------------------------------- //----------------------STUDENTS---------------------------- JButton students = new JButton("STUDENTS"); students.setBounds(150, 125, 700, 60); students.setFont(btn); students.setBackground(Color.decode("#DEE4E7")); students.setForeground(Color.decode("#37474F")); frame.add(students); students.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { Students std = new Students(); try { std.studentView(); } catch (SQLException e1) { // TODO Auto-generated catch block e1.printStackTrace(); } } }); //---------------------------------------------------------- //----------------------ADDATTENDANCE---------------------------- JButton addattendance = new JButton("ADD ATTENDANCE"); addattendance.setBounds(150, 250, 400, 60); addattendance.setFont(btn); addattendance.setBackground(Color.decode("#DEE4E7")); addattendance.setForeground(Color.decode("#37474F")); frame.add(addattendance); addattendance.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { AddAttendance addatt = new AddAttendance(); try { addatt.addView(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------------------------- //----------------------EDITATTENDANCE---------------------------- JButton editattendance = new JButton("EDIT ATTENDANCE"); editattendance.setBounds(600, 250, 250, 60); editattendance.setFont(btn); editattendance.setBackground(Color.decode("#DEE4E7")); editattendance.setForeground(Color.decode("#37474F")); frame.add(editattendance); editattendance.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { EditAttendance editatt = new EditAttendance(); try { editatt.editView(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------------------------- //----------------------TEACHERS---------------------------- JButton teacher = new JButton("TEACHERS"); teacher.setBounds(150, 375, 700, 60); teacher.setFont(new Font("Times New Roman", Font.BOLD, 20)); teacher.setBackground(Color.decode("#DEE4E7")); teacher.setForeground(Color.decode("#37474F")); frame.add(teacher); teacher.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { Teachers teacher = new Teachers(); try { teacher.teachersView(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------------------------- //----------------------USER---------------------------- JButton admin = new JButton("ADMIN"); admin.setBounds(150, 500, 250, 60); admin.setFont(new Font("Times New Roman", Font.BOLD, 20)); admin.setBackground(Color.decode("#DEE4E7")); admin.setForeground(Color.decode("#37474F")); frame.add(admin); admin.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { try { adm.adminView(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------------------------- //----------------------CLASS---------------------------- JButton classes = new JButton("CLASS"); classes.setBounds(450, 500, 400, 60); classes.setFont(new Font("Times New Roman", Font.BOLD, 20)); classes.setBackground(Color.decode("#DEE4E7")); classes.setForeground(Color.decode("#37474F")); frame.add(classes); classes.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { Class classroom = new Class(); classroom.classView(); } }); //---------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public String getUser(int id) throws SQLException { //ENTER PORT, USER, PASSWORD. String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; Connection con = DriverManager.getConnection(url, user, pass); String str = "SELECT name FROM user WHERE id = "+id; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); rst.next(); return rst.getString("name"); } }
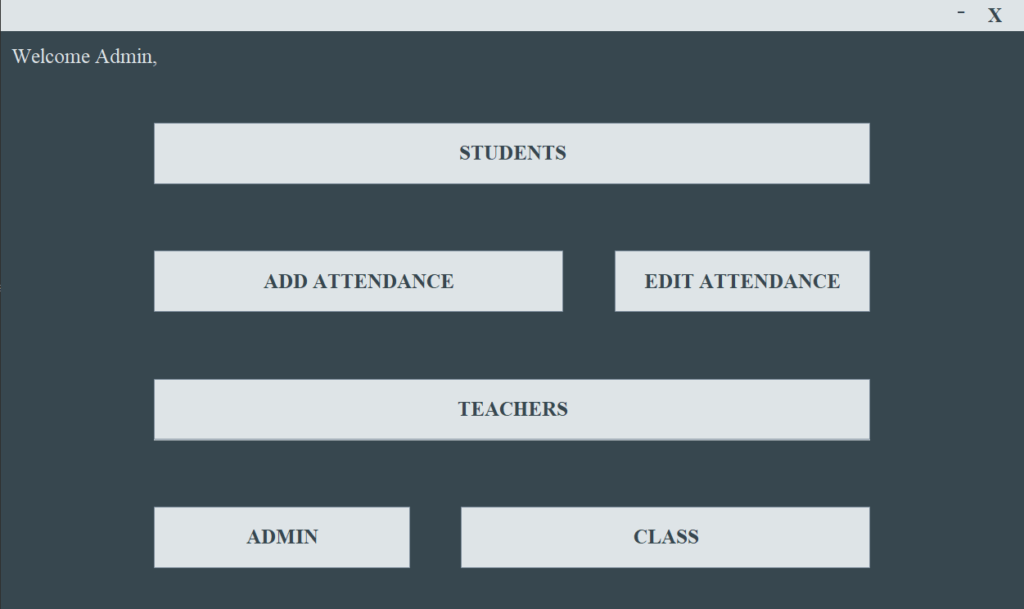
Each button shown on the page opens the respective window. You can add, edit and delete the items from it.
4. Home page for Teachers
File Name: “TeacherView.java“
The users with teacher credentials are directed to this page. We only have the option to add, edit and delete the attendance part. No other options are given.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; public class TeacherView { public void tcView(int id) throws SQLException { JFrame frame = new JFrame(); Font btn = new Font("Times New Roman", Font.BOLD, 20); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------MINIMIZE----------------------------- JLabel min = new JLabel("_"); min.setForeground(Color.decode("#37474F")); min.setBounds(935, 0, 100, 20); frame.add(min); min.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.setState(JFrame.ICONIFIED); } }); //------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //-------------------Welcome--------------------------------- JLabel welcome = new JLabel("Welcome "+getUser(id)+","); welcome.setForeground(Color.decode("#DEE4E7")); welcome.setBounds(10, 50, 250, 20); welcome.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(welcome); //----------------------------------------------------------- //----------------------ADDATTENDANCE---------------------------- JButton addattendance = new JButton("ADD ATTENDANCE"); addattendance.setBounds(150, 200, 650, 60); addattendance.setFont(btn); addattendance.setBackground(Color.decode("#DEE4E7")); addattendance.setForeground(Color.decode("#37474F")); frame.add(addattendance); addattendance.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { AddAttendance addatt = new AddAttendance(); try { addatt.addView(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------------------------- //----------------------EDITATTENDANCE---------------------------- JButton editattendance = new JButton("EDIT ATTENDANCE"); editattendance.setBounds(150, 350, 650, 60); editattendance.setFont(btn); editattendance.setBackground(Color.decode("#DEE4E7")); editattendance.setForeground(Color.decode("#37474F")); frame.add(editattendance); editattendance.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { EditAttendance editatt = new EditAttendance(); try { editatt.editView(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //---------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public String getUser(int id) throws SQLException { //ENTER PORT, USER, PASSWORD. String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; Connection con = DriverManager.getConnection(url, user, pass); String str = "SELECT name FROM user WHERE id = "+id; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); rst.next(); return rst.getString("name"); } }
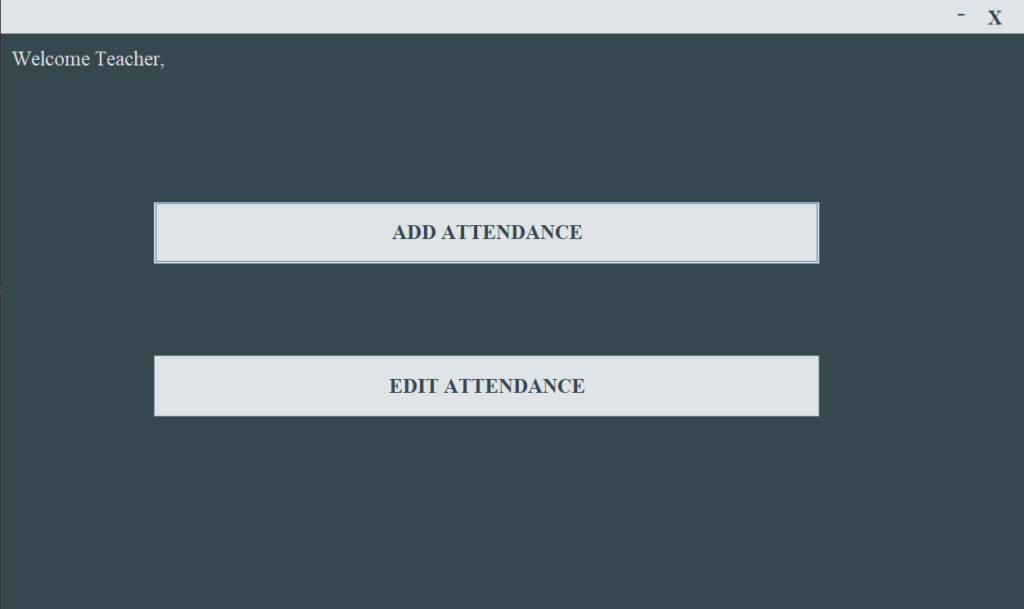
Here we can see two buttons for adding and editing the attendance of students. Deleting attendance can be done in the edit attendance part.
5. Homepage for students
File Name: “StudentView.java“
The students are directed to this page. They can view their total attendance, percentages, and their days of leaves.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollBar; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.table.DefaultTableModel; public class StudentView { Connection con; JFrame frame = new JFrame(); DefaultTableModel model = new DefaultTableModel(); public void stView(int id) throws SQLException { //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------MINIMIZE----------------------------- JLabel min = new JLabel("_"); min.setForeground(Color.decode("#37474F")); min.setBounds(935, 0, 100, 20); frame.add(min); min.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.setState(JFrame.ICONIFIED); } }); //------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //-------------------Welcome--------------------------------- JLabel welcome = new JLabel("Welcome "+getUser(id)+","); welcome.setForeground(Color.decode("#DEE4E7")); welcome.setBounds(10, 50, 250, 20); welcome.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(welcome); //----------------------------------------------------------- //----------------TABLE--------------------------------- JTable table=new JTable(); model = (DefaultTableModel)table.getModel(); model.addColumn("DATE"); model.addColumn("STATUS"); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(500, 50, 480, 525); table.setFont(new Font("Times New Roman", Font.BOLD, 20)); table.setRowHeight(50); frame.add(scPane); //------------------------------------------------------ //--------------------------INFO------------------------ JLabel totalclass = new JLabel("TOTAL CLASSES : "); totalclass.setBounds(25, 180, 250, 20); totalclass.setForeground(Color.decode("#DEE4E7")); totalclass.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(totalclass); JLabel ttbox = new JLabel(""); ttbox.setBounds(60, 230, 250, 20); ttbox.setForeground(Color.decode("#DEE4E7")); ttbox.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(ttbox); JLabel classAtt = new JLabel("CLASSES ATTENDED : "); classAtt.setBounds(25, 280, 250, 20); classAtt.setForeground(Color.decode("#DEE4E7")); classAtt.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(classAtt); JLabel atbox = new JLabel(""); atbox.setBounds(60, 330, 250, 20); atbox.setForeground(Color.decode("#DEE4E7")); atbox.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(atbox); JLabel classAbs = new JLabel("CLASSES MISSED : "); classAbs.setBounds(25, 380, 250, 20); classAbs.setForeground(Color.decode("#DEE4E7")); classAbs.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(classAbs); JLabel mtbox = new JLabel(""); mtbox.setBounds(60, 430, 250, 20); mtbox.setForeground(Color.decode("#DEE4E7")); mtbox.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(mtbox); JLabel AttPer = new JLabel("ATTENDANCE PERCENTAGE : "); AttPer.setBounds(25, 480, 300, 20); AttPer.setForeground(Color.decode("#DEE4E7")); AttPer.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(AttPer); JLabel prbox = new JLabel(""); prbox.setBounds(60, 530, 250, 20); prbox.setForeground(Color.decode("#DEE4E7")); prbox.setFont(new Font("Times New Roman", Font.PLAIN, 20)); frame.add(prbox); //------------------------------------------------------ //----------------------SETVALUES--------------------------- int[] arr = stat(4); ttbox.setText(String.valueOf(arr[0])); atbox.setText(String.valueOf(arr[1])); mtbox.setText(String.valueOf(arr[2])); prbox.setText(String.valueOf(arr[3])+"%"); //---------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public String getUser(int id) throws SQLException { //ENTER PORT, USER, PASSWORD. String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; con = DriverManager.getConnection(url, user, pass); String str = "SELECT name FROM user WHERE id = "+id; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); rst.next(); return rst.getString("name"); } public void tblupdt(int id) { try { ResultSet res = dbSearch(id); for(int i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getString("dt"), i, 0); model.setValueAt(res.getString("status"), i, 1); } } catch (SQLException e1) { e1.printStackTrace(); } } public int[] stat(int id) throws SQLException { String str = "SELECT COUNT(*) AS pre FROM attend WHERE stid = "+id+" AND status = 'Present'"; String str2 = "SELECT COUNT(*) AS abs FROM attend WHERE stid = "+id+" AND status = 'Absent'"; int[] x = new int[4]; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str); rst.next(); x[1] = rst.getInt("pre"); rst = stm.executeQuery(str2); rst.next(); x[2] = rst.getInt("abs"); x[0] = x[1] + x[2]; x[3] = (x[1]*100)/x[0]; tblupdt(id); return x; } public ResultSet dbSearch(int id) throws SQLException { String str1 = "SELECT * from attend where stid = "+id+" ORDER BY dt desc"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); return rst; } }

This is the interface for students. No buttons are included. The homepage is designed to show all the details.
6. Admin user control Module
File Name: “Admin.java“
This Module creates a page in which we can add, edit and delete the admin users.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextField; import javax.swing.table.DefaultTableModel; public class Admin{ DefaultTableModel model = new DefaultTableModel(); Font text = new Font("Times New Roman", Font.PLAIN, 18); Connection con; int check; JButton edit; JButton delete; JButton add; public void adminView() throws NumberFormatException, SQLException { JFrame frame = new JFrame(); Font btn = new Font("Times New Roman", Font.BOLD, 20); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------BACK--------------------------------- JLabel back = new JLabel("< BACK"); back.setForeground(Color.decode("#37474F")); back.setFont(new Font("Times New Roman", Font.BOLD, 17)); back.setBounds(18, 10, 100, 20); frame.add(back); back.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.dispose(); } }); //-------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //----------------TABLE--------------------------------- @SuppressWarnings("serial") JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ return false; } }; model = (DefaultTableModel)table.getModel(); model.addColumn("ID"); model.addColumn("USERNAME"); model.addColumn("NAME"); tblupdt(); table.getColumnModel().getColumn(0).setPreferredWidth(50); table.getColumnModel().getColumn(1).setPreferredWidth(200); table.getColumnModel().getColumn(2).setPreferredWidth(200); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(500, 50, 480, 525); frame.add(scPane); //------------------------------------------------------ //--------------------ID----------------------------------- JLabel id = new JLabel("ID : "); id.setFont(text); id.setBounds(25, 60, 40, 20); id.setForeground(Color.decode("#DEE4E7")); frame.add(id); JTextField idbox= new JTextField(); idbox.setBounds(60, 60, 50, 25); idbox.setBackground(Color.decode("#DEE4E7")); idbox.setFont(text); idbox.setForeground(Color.decode("#37474F")); idbox.setEditable(false); frame.add(idbox); //-------------------------------------------------------- //---------------------USERNAME------------------------- JLabel user = new JLabel("USERNAME : "); user.setFont(text); user.setBounds(25, 120, 150, 20); user.setForeground(Color.decode("#DEE4E7")); frame.add(user); JTextField username= new JTextField(); username.setBounds(25, 160, 400, 35); username.setBackground(Color.decode("#DEE4E7")); username.setFont(text); username.setForeground(Color.decode("#37474F")); username.setEditable(false); frame.add(username); //------------------------------------------------------ //-------------------NAME---------------------------------- JLabel nm = new JLabel("NAME : "); nm.setFont(text); nm.setBounds(25, 240, 150, 20); nm.setForeground(Color.decode("#DEE4E7")); frame.add(nm); JTextField name= new JTextField(); name.setBounds(25, 270, 400, 35); name.setBackground(Color.decode("#DEE4E7")); name.setFont(text); name.setForeground(Color.decode("#37474F")); name.setEditable(false); frame.add(name); //-------------------------------------------------------- //---------------------PASS-------------------------------- JLabel pass = new JLabel("PASSWORD : "); pass.setFont(text); pass.setBounds(25, 350, 150, 20); pass.setForeground(Color.decode("#DEE4E7")); frame.add(pass); JTextField password= new JTextField(); password.setBounds(25, 380, 400, 35); password.setBackground(Color.decode("#DEE4E7")); password.setFont(text); password.setForeground(Color.decode("#37474F")); password.setEditable(false); frame.add(password); //----------------------------------------------------------- //--------------------SAVEBUTTON--------------------------- JButton save = new JButton("SAVE"); save.setBounds(25, 500, 125, 50); save.setFont(btn); save.setBackground(Color.decode("#DEE4E7")); save.setForeground(Color.decode("#37474F")); save.setEnabled(false); frame.add(save); save.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(check == 1) { try { adder(Integer.parseInt(idbox.getText()), username.getText(), name.getText(), password.getText()); } catch (SQLException e1) { e1.printStackTrace(); } } else if(check == 2) { save.setEnabled(false); try { if(password.getText().equals("")) editor(Integer.parseInt(idbox.getText()), username.getText(), name.getText()); else editor(Integer.parseInt(idbox.getText()), username.getText(), name.getText(), password.getText()); } catch (SQLException e1) { e1.printStackTrace(); } } try { idbox.setText(String.valueOf(getid())); edit.setEnabled(false); delete.setEnabled(false); name.setText(""); username.setText(""); password.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------- //----------------------EDITBUTTON----------------------- edit = new JButton("EDIT"); edit.setBounds(175, 500, 125, 50); edit.setFont(btn); edit.setEnabled(false); edit.setBackground(Color.decode("#DEE4E7")); edit.setForeground(Color.decode("#37474F")); frame.add(edit); edit.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { edit.setEnabled(false); save.setEnabled(true); check = 2; username.setEditable(true); name.setEditable(true); password.setEditable(true); } }); //------------------------------------------------------- //--------------------ADDBUTTON------------------------- add = new JButton("ADD"); add.setBounds(325, 500, 125, 50); add.setFont(btn); add.setBackground(Color.decode("#DEE4E7")); add.setForeground(Color.decode("#37474F")); frame.add(add); add.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { add.setEnabled(false); save.setEnabled(true); delete.setEnabled(false); username.setEditable(true); name.setEditable(true); password.setEditable(true); check = 1; try { idbox.setText(String.valueOf(getid())); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------ //------------------------DELETEBUTTON----------------------- delete = new JButton("DELETE"); delete.setBounds(175, 432, 125, 50); delete.setFont(btn); delete.setBackground(Color.decode("#DEE4E7")); delete.setForeground(Color.decode("#37474F")); delete.setEnabled(false); frame.add(delete); delete.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { username.setEditable(false); name.setEditable(false); password.setEditable(false); edit.setEnabled(false); add.setEnabled(true); try { deleter(Integer.parseInt(idbox.getText())); idbox.setText(String.valueOf(getid())); name.setText(""); username.setText(""); password.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------------ //-----------------TABLE ACTION---------------------------- table.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { int row = table.getSelectedRow(); password.setText(""); idbox.setText(String.valueOf(table.getModel().getValueAt(row, 0))); username.setText(String.valueOf(table.getModel().getValueAt(row, 1))); name.setText(String.valueOf(table.getModel().getValueAt(row, 2))); edit.setEnabled(true); username.setEditable(false); password.setEditable(false); name.setEditable(false); save.setEnabled(false); delete.setEnabled(true); } }); //------------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public void tblupdt() { try { ResultSet res = dbSearch(); for(int i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getInt("id"), i, 0); model.setValueAt(res.getString("username"), i, 1); model.setValueAt(res.getString("name"), i, 2); } } catch (SQLException e1) { e1.printStackTrace(); } } public ResultSet dbSearch() throws SQLException { //ENTER PORT, USER, PASSWORD. String str1 = "SELECT * FROM user WHERE prio = 1"; String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; con = DriverManager.getConnection(url, user, pass); Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); return rst; } public int getid() throws SQLException { Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery("SELECT MAX(id) from user"); if(rst.next()) { return rst.getInt("MAX(id)")+1; } else { return 1; } } public void adder(int id, String user, String name, String password) throws SQLException { String adding = "insert into user values ("+id+", '"+user+"', '"+name+"', '"+password+"', 1)"; Statement stm = con.createStatement(); stm.executeUpdate(adding); } public void deleter(int id) throws SQLException { String del = "DELETE FROM user WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(del); } public void editor(int id, String username, String name, String password) throws SQLException { String update = "UPDATE user SET username = '"+username+"', name = '"+name+"', password = '"+password+"'WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(update); } public void editor(int id, String username, String name) throws SQLException { String update = "UPDATE user SET username = '"+username+"', name = '"+name+"' WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(update); } }

You can add the admin details here. Name is the name of the user while username is the credential required to login into the application. The Password field is used to enter the password for the login. The Edit button will be enabled on the selection of an item from the table.
7. Class control module
File Name: “Class.java“
This module helps us to create a page in which we can add, edit and delete the classes of the institution.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextField; import javax.swing.table.DefaultTableModel; public class Class{ DefaultTableModel model = new DefaultTableModel(); Connection con; int check; JButton edit; JButton delete; JButton add; public void classView() { JFrame frame = new JFrame(); Font text = new Font("Times New Roman", Font.PLAIN, 18); Font btn = new Font("Times New Roman", Font.BOLD, 20); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------BACK--------------------------------- JLabel back = new JLabel("< BACK"); back.setForeground(Color.decode("#37474F")); back.setFont(new Font("Times New Roman", Font.BOLD, 17)); back.setBounds(18, 10, 100, 20); frame.add(back); back.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.dispose(); } }); //-------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //--------------------ID----------------------------------- JLabel id = new JLabel("ID : "); id.setFont(text); id.setBounds(25, 150, 40, 20); id.setForeground(Color.decode("#DEE4E7")); frame.add(id); JTextField idbox= new JTextField(); idbox.setBounds(60, 150, 50, 25); idbox.setBackground(Color.decode("#DEE4E7")); idbox.setFont(text); idbox.setForeground(Color.decode("#37474F")); idbox.setEditable(false); frame.add(idbox); //-------------------------------------------------------- //-------------------NAME---------------------------------- JLabel nm = new JLabel("NAME : "); nm.setFont(text); nm.setBounds(25, 240, 150, 20); nm.setForeground(Color.decode("#DEE4E7")); frame.add(nm); JTextField name= new JTextField(); name.setBounds(25, 270, 400, 35); name.setBackground(Color.decode("#DEE4E7")); name.setFont(text); name.setForeground(Color.decode("#37474F")); name.setEditable(false); frame.add(name); //-------------------------------------------------------- //--------------------SAVEBUTTON--------------------------- JButton save = new JButton("SAVE"); save.setBounds(25, 500, 125, 50); save.setFont(btn); save.setBackground(Color.decode("#DEE4E7")); save.setForeground(Color.decode("#37474F")); save.setEnabled(false); frame.add(save); save.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(check == 1) { try { adder(Integer.parseInt(idbox.getText()), name.getText()); } catch (SQLException e1) { e1.printStackTrace(); } } else if(check == 2) { save.setEnabled(false); try { editor(Integer.parseInt(idbox.getText()), name.getText()); } catch (SQLException e1) { e1.printStackTrace(); } } try { idbox.setText(String.valueOf(getid())); edit.setEnabled(false); delete.setEnabled(false); name.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------- //----------------------EDITBUTTON----------------------- edit = new JButton("EDIT"); edit.setBounds(175, 500, 125, 50); edit.setFont(btn); edit.setEnabled(false); edit.setBackground(Color.decode("#DEE4E7")); edit.setForeground(Color.decode("#37474F")); frame.add(edit); edit.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { edit.setEnabled(false); save.setEnabled(true); check = 2; name.setEditable(true); } }); //------------------------------------------------------- //--------------------ADDBUTTON------------------------- add = new JButton("ADD"); add.setBounds(325, 500, 125, 50); add.setFont(btn); add.setBackground(Color.decode("#DEE4E7")); add.setForeground(Color.decode("#37474F")); frame.add(add); add.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { add.setEnabled(false); delete.setEnabled(false); save.setEnabled(true); name.setEditable(true); check = 1; try { idbox.setText(String.valueOf(getid())); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------ //------------------------DELETEBUTTON----------------------- delete = new JButton("DELETE"); delete.setBounds(175, 432, 125, 50); delete.setFont(btn); delete.setBackground(Color.decode("#DEE4E7")); delete.setForeground(Color.decode("#37474F")); delete.setEnabled(false); frame.add(delete); delete.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { name.setEditable(false); edit.setEnabled(false); add.setEnabled(true); try { deleter(Integer.parseInt(idbox.getText())); idbox.setText(String.valueOf(getid())); name.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------------ //----------------TABLE--------------------------------- @SuppressWarnings("serial") JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ return false; } }; model = (DefaultTableModel)table.getModel(); model.addColumn("ID"); model.addColumn("NAME"); tblupdt(); table.getColumnModel().getColumn(0).setPreferredWidth(100); table.getColumnModel().getColumn(1).setPreferredWidth(300); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(500, 50, 480, 525); frame.add(scPane); //------------------------------------------------------ //-----------------TABLE ACTION---------------------------- table.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { int row = table.getSelectedRow(); idbox.setText(String.valueOf(table.getModel().getValueAt(row, 0))); name.setText(String.valueOf(table.getModel().getValueAt(row, 1))); edit.setEnabled(true); save.setEnabled(false); delete.setEnabled(true); } }); //------------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public void tblupdt() { try { ResultSet res = dbSearch(); for(int i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getInt("id"), i, 0); model.setValueAt(res.getString("name"), i, 1); } } catch (SQLException e1) { e1.printStackTrace(); } } public int getid() throws SQLException { Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery("SELECT MAX(id) from class"); if(rst.next()) { return rst.getInt("MAX(id)")+1; } else { return 1; } } public ResultSet dbSearch() throws SQLException { //ENTER PORT, USER, PASSWORD. String str1 = "SELECT * FROM class"; String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; con = DriverManager.getConnection(url, user, pass); Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); return rst; } public void adder(int id, String name) throws SQLException { String adding = "insert into class values ("+id+", '"+name+"')"; Statement stm = con.createStatement(); stm.executeUpdate(adding); } public void deleter(int id) throws SQLException { String del = "DELETE FROM class WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(del); } public void editor(int id, String name) throws SQLException { String update = "UPDATE class SET name = '"+name+"'WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(update); } }
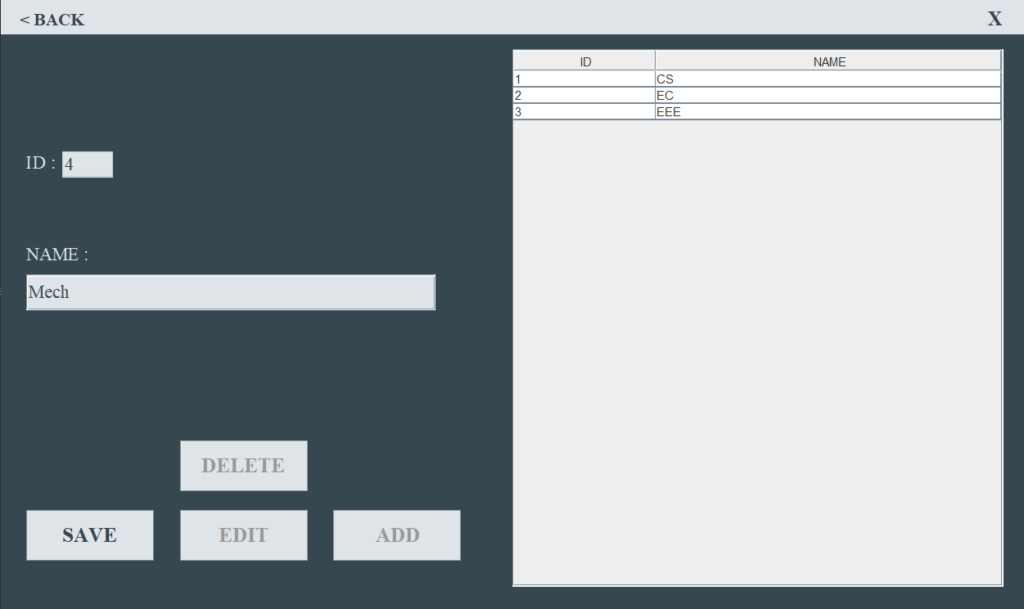
You can add, edit and delete the classes with this module.
8. Teacher Control Module
File Name: “Teachers.java“
This module here is used to manage the teachers in the institution.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextField; import javax.swing.table.DefaultTableModel; public class Teachers { DefaultTableModel model = new DefaultTableModel(); Connection con; int check; JButton edit; JButton delete; JButton add; public void teachersView() throws SQLException { Font text = new Font("Times New Roman", Font.PLAIN, 18); Font btn = new Font("Times New Roman", Font.BOLD, 20); JFrame frame = new JFrame(); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------BACK--------------------------------- JLabel back = new JLabel("< BACK"); back.setForeground(Color.decode("#37474F")); back.setFont(new Font("Times New Roman", Font.BOLD, 17)); back.setBounds(18, 10, 100, 20); frame.add(back); back.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.dispose(); } }); //-------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //----------------TABLE--------------------------------- @SuppressWarnings("serial") JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ return false; } }; model = (DefaultTableModel)table.getModel(); model.addColumn("ID"); model.addColumn("USERNAME"); model.addColumn("NAME"); tblupdt(); table.getColumnModel().getColumn(0).setPreferredWidth(50); table.getColumnModel().getColumn(1).setPreferredWidth(200); table.getColumnModel().getColumn(2).setPreferredWidth(200); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(500, 50, 480, 525); frame.add(scPane); //------------------------------------------------------ //--------------------ID----------------------------------- JLabel id = new JLabel("ID : "); id.setFont(text); id.setBounds(25, 60, 40, 20); id.setForeground(Color.decode("#DEE4E7")); frame.add(id); JTextField idbox= new JTextField(); idbox.setBounds(60, 60, 50, 25); idbox.setBackground(Color.decode("#DEE4E7")); idbox.setFont(text); idbox.setForeground(Color.decode("#37474F")); idbox.setEditable(false); idbox.setText(String.valueOf(getid())); frame.add(idbox); //-------------------------------------------------------- //---------------------USERNAME------------------------- JLabel user = new JLabel("USERNAME : "); user.setFont(text); user.setBounds(25, 120, 150, 20); user.setForeground(Color.decode("#DEE4E7")); frame.add(user); JTextField username= new JTextField(); username.setBounds(25, 160, 400, 35); username.setBackground(Color.decode("#DEE4E7")); username.setFont(text); username.setForeground(Color.decode("#37474F")); username.setEditable(false); frame.add(username); //------------------------------------------------------ //-------------------NAME---------------------------------- JLabel nm = new JLabel("NAME : "); nm.setFont(text); nm.setBounds(25, 240, 150, 20); nm.setForeground(Color.decode("#DEE4E7")); frame.add(nm); JTextField name= new JTextField(); name.setBounds(25, 270, 400, 35); name.setBackground(Color.decode("#DEE4E7")); name.setFont(text); name.setForeground(Color.decode("#37474F")); name.setEditable(false); frame.add(name); //-------------------------------------------------------- //---------------------PASS-------------------------------- JLabel pass = new JLabel("PASSWORD : "); pass.setFont(text); pass.setBounds(25, 350, 150, 20); pass.setForeground(Color.decode("#DEE4E7")); frame.add(pass); JTextField password= new JTextField(); password.setBounds(25, 380, 400, 35); password.setBackground(Color.decode("#DEE4E7")); password.setFont(text); password.setForeground(Color.decode("#37474F")); password.setEditable(false); frame.add(password); //----------------------------------------------------------- //--------------------SAVEBUTTON--------------------------- JButton save = new JButton("SAVE"); save.setBounds(25, 500, 125, 50); save.setFont(btn); save.setBackground(Color.decode("#DEE4E7")); save.setForeground(Color.decode("#37474F")); save.setEnabled(false); frame.add(save); save.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(check == 1) { try { adder(Integer.parseInt(idbox.getText()), username.getText(), name.getText(), password.getText()); } catch (SQLException e1) { e1.printStackTrace(); } } else if(check == 2) { save.setEnabled(false); try { if(password.getText().equals("")) editor(Integer.parseInt(idbox.getText()), username.getText(), name.getText()); else editor(Integer.parseInt(idbox.getText()), username.getText(), name.getText(), password.getText()); } catch (SQLException e1) { e1.printStackTrace(); } } try { idbox.setText(String.valueOf(getid())); edit.setEnabled(false); delete.setEnabled(false); name.setText(""); username.setText(""); password.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------- //----------------------EDITBUTTON----------------------- edit = new JButton("EDIT"); edit.setBounds(175, 500, 125, 50); edit.setFont(btn); edit.setEnabled(false); edit.setBackground(Color.decode("#DEE4E7")); edit.setForeground(Color.decode("#37474F")); frame.add(edit); edit.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { edit.setEnabled(false); save.setEnabled(true); check = 2; username.setEditable(true); name.setEditable(true); password.setEditable(true); } }); //------------------------------------------------------- //--------------------ADDBUTTON------------------------- add = new JButton("ADD"); add.setBounds(325, 500, 125, 50); add.setFont(btn); add.setBackground(Color.decode("#DEE4E7")); add.setForeground(Color.decode("#37474F")); frame.add(add); add.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { add.setEnabled(false); save.setEnabled(true); delete.setEnabled(false); username.setEditable(true); name.setEditable(true); password.setEditable(true); check = 1; try { idbox.setText(String.valueOf(getid())); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------ //------------------------DELETEBUTTON----------------------- delete = new JButton("DELETE"); delete.setBounds(175, 432, 125, 50); delete.setFont(btn); delete.setBackground(Color.decode("#DEE4E7")); delete.setForeground(Color.decode("#37474F")); delete.setEnabled(false); frame.add(delete); delete.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { username.setEditable(false); name.setEditable(false); password.setEditable(false); edit.setEnabled(false); add.setEnabled(true); try { deleter(Integer.parseInt(idbox.getText())); idbox.setText(String.valueOf(getid())); name.setText(""); username.setText(""); password.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------------ //-----------------TABLE ACTION---------------------------- table.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { int row = table.getSelectedRow(); password.setText(""); idbox.setText(String.valueOf(table.getModel().getValueAt(row, 0))); username.setText(String.valueOf(table.getModel().getValueAt(row, 1))); name.setText(String.valueOf(table.getModel().getValueAt(row, 2))); edit.setEnabled(true); username.setEditable(false); password.setEditable(false); name.setEditable(false); save.setEnabled(false); delete.setEnabled(true); } }); //------------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public void tblupdt() { try { ResultSet res = dbSearch(); for(int i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getInt("id"), i, 0); model.setValueAt(res.getString("username"), i, 1); model.setValueAt(res.getString("name"), i, 2); } } catch (SQLException e1) { e1.printStackTrace(); } } public int getid() throws SQLException { Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery("SELECT MAX(id) from user"); if(rst.next()) { return rst.getInt("MAX(id)")+1; } else { return 1; } } public ResultSet dbSearch() throws SQLException { //ENTER PORT, USER, PASSWORD. String str1 = "SELECT user.id, user.username, teachers.name FROM user, teachers where user.id = teachers.id"; String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; con = DriverManager.getConnection(url, user, pass); Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); return rst; } public void adder(int id, String user, String name, String password) throws SQLException { String adding = "insert into user values ("+id+", '"+user+"', '"+name+"', '"+password+"', 2)"; String adding2 = "insert into teachers values ("+id+", '"+name+"')"; Statement stm = con.createStatement(); stm.executeUpdate(adding); stm.executeUpdate(adding2); } public void deleter(int id) throws SQLException { String del = "DELETE FROM teachers WHERE id = "+id; String del2 = "DELETE FROM user WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(del); stm.executeUpdate(del2); } public void editor(int id, String username, String name, String password) throws SQLException { String update = "UPDATE user SET username = '"+username+"', name = '"+name+"', password = '"+password+"'WHERE id = "+id; String update2 = "UPDATE teachers SET name = '"+name+"' WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(update); stm.executeUpdate(update2); } public void editor(int id, String username, String name) throws SQLException { String update = "UPDATE user SET username = '"+username+"', name = '"+name+"' WHERE id = "+id; String update2 = "UPDATE teachers SET name = '"+name+"' WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(update); stm.executeUpdate(update2); } }
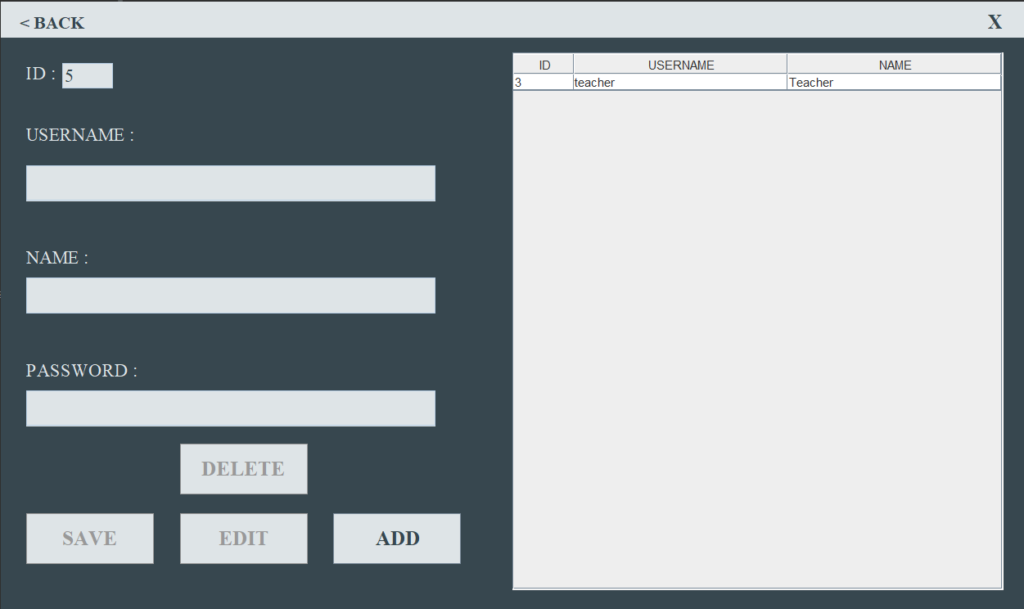
This window is the same as the admin. Here you can create changes in the teacher’s database.
9. Student control module
File Name: “Students.java“
The module controls the student database. Changes to the students are done using this module.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextField; import javax.swing.table.DefaultTableModel; public class Students { DefaultTableModel model = new DefaultTableModel(); Connection con; int check; JButton edit; JButton delete; JButton add; public void studentView() throws SQLException { Font text = new Font("Times New Roman", Font.PLAIN, 18); Font btn = new Font("Times New Roman", Font.BOLD, 20); JFrame frame = new JFrame(); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------BACK--------------------------------- JLabel back = new JLabel("< BACK"); back.setForeground(Color.decode("#37474F")); back.setFont(new Font("Times New Roman", Font.BOLD, 17)); back.setBounds(18, 10, 100, 20); frame.add(back); back.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.dispose(); } }); //-------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //----------------TABLE--------------------------------- @SuppressWarnings("serial") JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ return false; } }; model = (DefaultTableModel)table.getModel(); model.addColumn("ID"); model.addColumn("USERNAME"); model.addColumn("NAME"); tblupdt(); table.getColumnModel().getColumn(0).setPreferredWidth(50); table.getColumnModel().getColumn(1).setPreferredWidth(200); table.getColumnModel().getColumn(2).setPreferredWidth(200); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(500, 50, 480, 525); frame.add(scPane); //------------------------------------------------------ //--------------------ID----------------------------------- JLabel id = new JLabel("ID : "); id.setFont(text); id.setBounds(25, 60, 40, 20); id.setForeground(Color.decode("#DEE4E7")); frame.add(id); JTextField idbox= new JTextField(); idbox.setBounds(60, 60, 50, 25); idbox.setBackground(Color.decode("#DEE4E7")); idbox.setFont(text); idbox.setForeground(Color.decode("#37474F")); idbox.setEditable(false); idbox.setText(String.valueOf(getid())); frame.add(idbox); //-------------------------------------------------------- //--------------------CLASS--------------------------------- JLabel classes = new JLabel("CLASS : "); classes.setFont(text); classes.setBounds(250, 60, 100, 20); classes.setForeground(Color.decode("#DEE4E7")); frame.add(classes); @SuppressWarnings("unchecked") JComboBox clss= new JComboBox(classEt()); clss.setBounds(350, 60, 50, 25); clss.setEnabled(false); frame.add(clss); //------------------------------------------------------------ //---------------------USERNAME------------------------- JLabel user = new JLabel("USERNAME : "); user.setFont(text); user.setBounds(25, 120, 150, 20); user.setForeground(Color.decode("#DEE4E7")); frame.add(user); JTextField username= new JTextField(); username.setBounds(25, 160, 400, 35); username.setBackground(Color.decode("#DEE4E7")); username.setFont(text); username.setForeground(Color.decode("#37474F")); username.setEditable(false); frame.add(username); //------------------------------------------------------ //-------------------NAME---------------------------------- JLabel nm = new JLabel("NAME : "); nm.setFont(text); nm.setBounds(25, 240, 150, 20); nm.setForeground(Color.decode("#DEE4E7")); frame.add(nm); JTextField name= new JTextField(); name.setBounds(25, 270, 400, 35); name.setBackground(Color.decode("#DEE4E7")); name.setFont(text); name.setForeground(Color.decode("#37474F")); name.setEditable(false); frame.add(name); //-------------------------------------------------------- //---------------------PASS-------------------------------- JLabel pass = new JLabel("PASSWORD : "); pass.setFont(text); pass.setBounds(25, 350, 150, 20); pass.setForeground(Color.decode("#DEE4E7")); frame.add(pass); JTextField password= new JTextField(); password.setBounds(25, 380, 400, 35); password.setBackground(Color.decode("#DEE4E7")); password.setFont(text); password.setForeground(Color.decode("#37474F")); password.setEditable(false); frame.add(password); //----------------------------------------------------------- //--------------------SAVEBUTTON--------------------------- JButton save = new JButton("SAVE"); save.setBounds(25, 500, 125, 50); save.setFont(btn); save.setBackground(Color.decode("#DEE4E7")); save.setForeground(Color.decode("#37474F")); save.setEnabled(false); frame.add(save); save.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if(check == 1) { try { adder(Integer.parseInt(idbox.getText()), username.getText(), name.getText(), password.getText(), String.valueOf(clss.getSelectedItem())); } catch (SQLException e1) { e1.printStackTrace(); } } else if(check == 2) { save.setEnabled(false); try { if(password.getText().equals("")) editor(Integer.parseInt(idbox.getText()), username.getText(), name.getText(), String.valueOf(clss.getSelectedItem())); else editor(Integer.parseInt(idbox.getText()), username.getText(), name.getText(), password.getText(), String.valueOf(clss.getSelectedItem())); } catch (SQLException e1) { e1.printStackTrace(); } } try { idbox.setText(String.valueOf(getid())); edit.setEnabled(false); delete.setEnabled(false); name.setText(""); username.setText(""); password.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------- //----------------------EDITBUTTON----------------------- edit = new JButton("EDIT"); edit.setBounds(175, 500, 125, 50); edit.setFont(btn); edit.setEnabled(false); edit.setBackground(Color.decode("#DEE4E7")); edit.setForeground(Color.decode("#37474F")); frame.add(edit); edit.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { edit.setEnabled(false); save.setEnabled(true); check = 2; username.setEditable(true); name.setEditable(true); password.setEditable(true); clss.setEnabled(true); } }); //------------------------------------------------------- //--------------------ADDBUTTON------------------------- add = new JButton("ADD"); add.setBounds(325, 500, 125, 50); add.setFont(btn); add.setBackground(Color.decode("#DEE4E7")); add.setForeground(Color.decode("#37474F")); frame.add(add); add.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { add.setEnabled(false); save.setEnabled(true); delete.setEnabled(false); username.setEditable(true); name.setEditable(true); password.setEditable(true); clss.setEnabled(true); check = 1; try { idbox.setText(String.valueOf(getid())); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------ //------------------------DELETEBUTTON----------------------- delete = new JButton("DELETE"); delete.setBounds(175, 432, 125, 50); delete.setFont(btn); delete.setBackground(Color.decode("#DEE4E7")); delete.setForeground(Color.decode("#37474F")); delete.setEnabled(false); frame.add(delete); delete.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { username.setEditable(false); name.setEditable(false); password.setEditable(false); clss.setEnabled(false); edit.setEnabled(false); add.setEnabled(true); try { deleter(Integer.parseInt(idbox.getText())); idbox.setText(String.valueOf(getid())); name.setText(""); username.setText(""); password.setText(""); while(model.getRowCount() > 0) model.removeRow(0); tblupdt(); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------------ //-----------------TABLE ACTION---------------------------- table.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { int row = table.getSelectedRow(); password.setText(""); idbox.setText(String.valueOf(table.getModel().getValueAt(row, 0))); username.setText(String.valueOf(table.getModel().getValueAt(row, 1))); name.setText(String.valueOf(table.getModel().getValueAt(row, 2))); edit.setEnabled(true); username.setEditable(false); password.setEditable(false); clss.setEnabled(false); name.setEditable(false); save.setEnabled(false); delete.setEnabled(true); add.setEnabled(true); } }); //------------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public void tblupdt() { try { ResultSet res = dbSearch(); for(int i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getInt("id"), i, 0); model.setValueAt(res.getString("username"), i, 1); model.setValueAt(res.getString("name"), i, 2); } } catch (SQLException e1) { e1.printStackTrace(); } } public int getid() throws SQLException { Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery("SELECT MAX(id) from user"); if(rst.next()) { return rst.getInt("MAX(id)")+1; } else { return 1; } } public ResultSet dbSearch() throws SQLException { //ENTER PORT, USER, PASSWORD. String str1 = "SELECT user.id, user.username, students.name FROM user, students where user.id = students.id"; String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; con = DriverManager.getConnection(url, user, pass); Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); return rst; } public void adder(int id, String user, String name, String password, String classes) throws SQLException { String adding = "insert into user values ("+id+", '"+user+"', '"+name+"', '"+password+"', 3)"; String adding2 = "insert into students values ("+id+", '"+name+"', '"+classes+"')"; Statement stm = con.createStatement(); stm.executeUpdate(adding); stm.executeUpdate(adding2); } public void deleter(int id) throws SQLException { String del = "DELETE FROM students WHERE id = "+id; String del2 = "DELETE FROM user WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(del); stm.executeUpdate(del2); } public void editor(int id, String username, String name, String password, String classes) throws SQLException { String update = "UPDATE user SET username = '"+username+"', name = '"+name+"', password = '"+password+"'WHERE id = "+id; String update2 = "UPDATE students SET name = '"+name+"', class = '"+classes+"' WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(update); stm.executeUpdate(update2); } public void editor(int id, String username, String name, String classes) throws SQLException { String update = "UPDATE user SET username = '"+username+"', name = '"+name+"' WHERE id = "+id; String update2 = "UPDATE students SET name = '"+name+"', class = '"+classes+"' WHERE id = "+id; Statement stm = con.createStatement(); stm.executeUpdate(update); stm.executeUpdate(update2); } public String[] classEt() throws SQLException { String str1 = "SELECT name from class"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); String[] rt = new String[25]; int i=0; while(rst.next()) { rt[i] = rst.getString("name"); i++; } return rt; } }
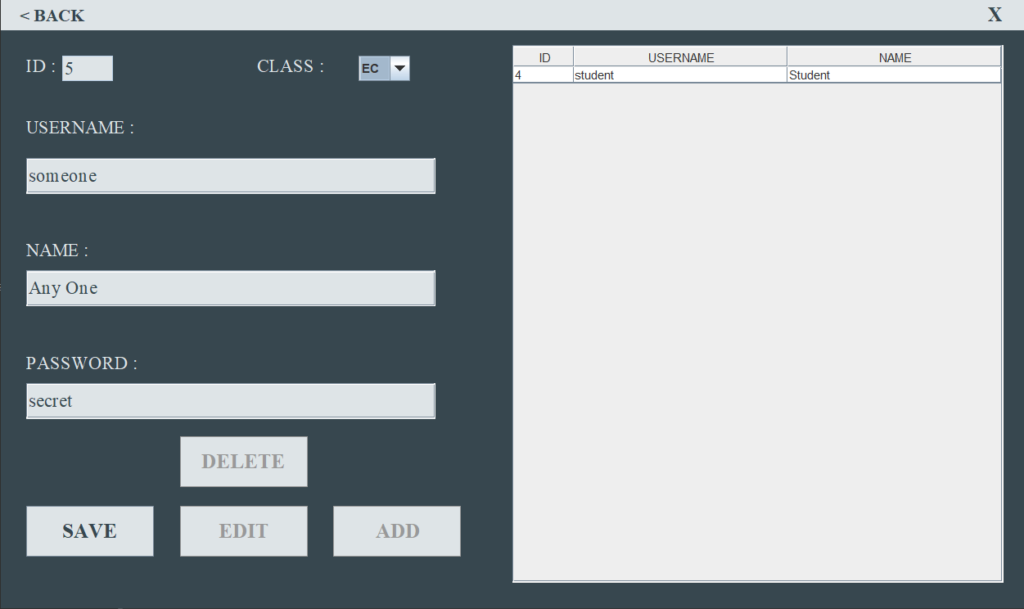
A window similar to that we have seen in the admin and teacher control module. A drop-down list of classes is added to select the class of the student.
10. Add Attendance Module
This module helps in adding the attendance of the student. This attendance can be edited later using another window.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.text.SimpleDateFormat; import java.util.Date; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextField; import javax.swing.table.DefaultTableModel; public class AddAttendance { Connection con; DefaultTableModel model = new DefaultTableModel(); public void addView() throws SQLException { connect(); JFrame frame = new JFrame(); Font text = new Font("Times New Roman", Font.PLAIN, 18); Font btn = new Font("Times New Roman", Font.BOLD, 20); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------BACK--------------------------------- JLabel back = new JLabel("< BACK"); back.setForeground(Color.decode("#37474F")); back.setFont(new Font("Times New Roman", Font.BOLD, 17)); back.setBounds(18, 10, 100, 20); frame.add(back); back.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.dispose(); } }); //-------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //----------------TABLE--------------------------------- @SuppressWarnings("serial") JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ return false; } }; model = (DefaultTableModel)table.getModel(); model.addColumn("ID"); model.addColumn("NAME"); model.addColumn("STATUS"); table.getColumnModel().getColumn(0).setPreferredWidth(50); table.getColumnModel().getColumn(1).setPreferredWidth(200); table.getColumnModel().getColumn(2).setPreferredWidth(200); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(500, 50, 480, 525); frame.add(scPane); //------------------------------------------------------ //-------------------------DATE------------------------- JLabel dt = new JLabel("DATE : "); dt.setFont(text); dt.setBounds(25, 60, 75, 20); dt.setForeground(Color.decode("#DEE4E7")); frame.add(dt); JTextField dtbox= new JTextField(); dtbox.setBounds(100, 60, 150, 25); dtbox.setBackground(Color.decode("#DEE4E7")); dtbox.setFont(text); dtbox.setForeground(Color.decode("#37474F")); String dateInString =new SimpleDateFormat("yyyy-MM-dd").format(new Date()); dtbox.setText(dateInString); frame.add(dtbox); //------------------------------------------------------- //--------------------CLASS--------------------------------- JLabel classes = new JLabel("CLASS : "); classes.setFont(text); classes.setBounds(25, 150, 100, 20); classes.setForeground(Color.decode("#DEE4E7")); frame.add(classes); @SuppressWarnings("unchecked") JComboBox clss= new JComboBox(classEt()); clss.setBounds(110, 150, 50, 25); frame.add(clss); //------------------------------------------------------------ //---------------------ALREADY ADDED------------------------ JLabel txt = new JLabel(""); txt.setFont(text); txt.setBounds(125, 525, 350, 20); txt.setForeground(Color.red); frame.add(txt); //------------------------------------------------------------- //----------------------VIEWBUTTON----------------------- JButton view = new JButton("VIEW"); view.setBounds(175, 275, 150, 50); view.setFont(btn); view.setBackground(Color.decode("#DEE4E7")); view.setForeground(Color.decode("#37474F")); frame.add(view); view.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { try { if(check(String.valueOf(clss.getSelectedItem()), dtbox.getText())) txt.setText("Attendance Already marked!!!"); else tblupdt(String.valueOf(clss.getSelectedItem())); } catch (SQLException e1) { e1.printStackTrace(); } } }); //------------------------------------------------------- //----------------------ABSENTBUTTON----------------------- JButton ab = new JButton("ABSENT"); ab.setBounds(75, 365, 150, 50); ab.setFont(btn); ab.setBackground(Color.decode("#DEE4E7")); ab.setForeground(Color.decode("#37474F")); frame.add(ab); ab.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { table.setValueAt("Absent", table.getSelectedRow(), 2); } }); //------------------------------------------------------- //----------------------PRESENTBUTTON----------------------- JButton pre = new JButton("PRESENT"); pre.setBounds(275, 365, 150, 50); pre.setFont(btn); pre.setBackground(Color.decode("#DEE4E7")); pre.setForeground(Color.decode("#37474F")); frame.add(pre); pre.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.out.print(table.getSelectedRow()); table.setValueAt("Present", table.getSelectedRow(), 2); } }); //------------------------------------------------------- //----------------------SUBMITBUTTON----------------------- JButton sbmt = new JButton("SUBMIT"); sbmt.setBounds(175, 450, 150, 50); sbmt.setFont(btn); sbmt.setBackground(Color.decode("#DEE4E7")); sbmt.setForeground(Color.decode("#37474F")); frame.add(sbmt); sbmt.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { for(int i= 0; i<table.getRowCount(); i++) { try { addItem(Integer.parseInt(String.valueOf(table.getValueAt(i, 0))), String.valueOf(table.getValueAt(i, 2)), dtbox.getText(), String.valueOf(clss.getSelectedItem())); } catch (NumberFormatException | SQLException e1) { e1.printStackTrace(); } } for (int i=0; i < model.getRowCount(); i++) { model.removeRow(i); model.setRowCount(0); } } }); //------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public void connect() throws SQLException { //ENTER PORT, USER, PASSWORD. String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; con = DriverManager.getConnection(url, user, pass); } public ResultSet dbSearch(String classes) throws SQLException { String str1 = "SELECT * from students where class = '"+classes+"'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); return rst; } public String[] classEt() throws SQLException { String str1 = "SELECT name from class"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); String[] rt = new String[25]; int i=0; while(rst.next()) { rt[i] = rst.getString("name"); i++; } return rt; } public void tblupdt(String classes) { for (int i=0; i < model.getRowCount(); i++) { model.removeRow(i); model.setRowCount(0); } try { ResultSet res = dbSearch(classes); for(int i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getInt("id"), i, 0); model.setValueAt(res.getString("name"), i, 1); model.setValueAt("Present", i, 2); } } catch (SQLException e1) { e1.printStackTrace(); } } public void addItem(int id, String status, String date, String classes) throws SQLException { String adding = "INSERT INTO attend values("+id+", '"+date+"', '"+status+"', '"+classes+"')"; Statement stm = con.createStatement(); stm.executeUpdate(adding); } public boolean check(String classes, String dt) throws SQLException { String str1 = "select * from attend where class = '"+classes+"' AND dt = '"+dt+"'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); if(rst.next()) return true; else return false; } }

We can choose the class and press the view button to show the students in the class. Select the student and we can change the status and save it. It is present for all the students by default. You cannot Add the attendance which is added before. We can edit it using the edit module.
11. Attendance Edit Module
Module to edit and delete the added attendance. Attendances once added are only shown in the edit window.
package Attendance; import java.awt.Color; import java.awt.Font; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.text.SimpleDateFormat; import java.util.Date; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextField; import javax.swing.table.DefaultTableModel; public class EditAttendance { Connection con; DefaultTableModel model = new DefaultTableModel(); public void editView() throws SQLException { connect(); JFrame frame = new JFrame(); Font text = new Font("Times New Roman", Font.PLAIN, 18); Font btn = new Font("Times New Roman", Font.BOLD, 20); //------------------------CLOSE--------------------------- JLabel x = new JLabel("X"); x.setForeground(Color.decode("#37474F")); x.setBounds(965, 10, 100, 20); x.setFont(new Font("Times New Roman", Font.BOLD, 20)); frame.add(x); x.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.exit(0); } }); //---------------------------------------------------------- //-----------------------BACK--------------------------------- JLabel back = new JLabel("< BACK"); back.setForeground(Color.decode("#37474F")); back.setFont(new Font("Times New Roman", Font.BOLD, 17)); back.setBounds(18, 10, 100, 20); frame.add(back); back.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { frame.dispose(); } }); //-------------------------------------------------------------- //------------------Panel---------------------------------- JPanel panel = new JPanel(); panel.setBounds(0, 0, 1000, 35); panel.setBackground(Color.decode("#DEE4E7")); frame.add(panel); //--------------------------------------------------------- //----------------TABLE--------------------------------- @SuppressWarnings("serial") JTable table=new JTable(){ public boolean isCellEditable(int row,int column){ if(column == 3) return true; else return false; } }; model = (DefaultTableModel)table.getModel(); model.addColumn("ID"); model.addColumn("NAME"); model.addColumn("STATUS"); table.getColumnModel().getColumn(0).setPreferredWidth(50); table.getColumnModel().getColumn(1).setPreferredWidth(200); table.getColumnModel().getColumn(2).setPreferredWidth(200); JScrollPane scPane=new JScrollPane(table); scPane.setBounds(500, 50, 480, 525); frame.add(scPane); //------------------------------------------------------ //-------------------------DATE------------------------- JLabel dt = new JLabel("DATE : "); dt.setFont(text); dt.setBounds(25, 60, 75, 20); dt.setForeground(Color.decode("#DEE4E7")); frame.add(dt); JTextField dtbox= new JTextField(); dtbox.setBounds(100, 60, 150, 25); dtbox.setBackground(Color.decode("#DEE4E7")); dtbox.setFont(text); dtbox.setForeground(Color.decode("#37474F")); String dateInString =new SimpleDateFormat("yyyy-MM-dd").format(new Date()); dtbox.setText(dateInString); frame.add(dtbox); //------------------------------------------------------- //--------------------CLASS--------------------------------- JLabel classes = new JLabel("CLASS : "); classes.setFont(text); classes.setBounds(25, 150, 100, 20); classes.setForeground(Color.decode("#DEE4E7")); frame.add(classes); @SuppressWarnings("unchecked") JComboBox clss= new JComboBox(classEt()); clss.setBounds(110, 150, 50, 25); frame.add(clss); //------------------------------------------------------------ //----------------------VIEWBUTTON----------------------- JButton view = new JButton("VIEW"); view.setBounds(175, 275, 150, 50); view.setFont(btn); view.setBackground(Color.decode("#DEE4E7")); view.setForeground(Color.decode("#37474F")); frame.add(view); view.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { tblupdt(String.valueOf(clss.getSelectedItem()), dtbox.getText()); } }); //------------------------------------------------------- //----------------------ABSENTBUTTON----------------------- JButton ab = new JButton("ABSENT"); ab.setBounds(75, 365, 150, 50); ab.setFont(btn); ab.setBackground(Color.decode("#DEE4E7")); ab.setForeground(Color.decode("#37474F")); frame.add(ab); ab.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { table.setValueAt("Absent", table.getSelectedRow(), 2); } }); //------------------------------------------------------- //----------------------PRESENTBUTTON----------------------- JButton pre = new JButton("PRESENT"); pre.setBounds(275, 365, 150, 50); pre.setFont(btn); pre.setBackground(Color.decode("#DEE4E7")); pre.setForeground(Color.decode("#37474F")); frame.add(pre); pre.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.out.print(table.getSelectedRow()); table.setValueAt("Present", table.getSelectedRow(), 2); } }); //------------------------------------------------------- //----------------------SUBMITBUTTON----------------------- JButton sbmt = new JButton("SUBMIT"); sbmt.setBounds(75, 450, 150, 50); sbmt.setFont(btn); sbmt.setBackground(Color.decode("#DEE4E7")); sbmt.setForeground(Color.decode("#37474F")); frame.add(sbmt); sbmt.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { for(int i= 0; i<table.getRowCount(); i++) { try { editItem(Integer.parseInt(String.valueOf(table.getValueAt(i, 0))), String.valueOf(table.getValueAt(i, 2)), dtbox.getText()); } catch (NumberFormatException | SQLException e1) { e1.printStackTrace(); } } for (int i=0; i < model.getRowCount(); i++) { model.removeRow(i); model.setRowCount(0); } } }); //------------------------------------------------------- //----------------------DELETEBUTTON----------------------- JButton del = new JButton("DELETE"); del.setBounds(275, 450, 150, 50); del.setFont(btn); del.setBackground(Color.decode("#DEE4E7")); del.setForeground(Color.decode("#37474F")); frame.add(del); del.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String adding = "DELETE FROM attend WHERE class = '"+String.valueOf(clss.getSelectedItem())+"' AND dt = '"+dtbox.getText()+"'"; try { Statement stm = con.createStatement(); stm.executeUpdate(adding); } catch (SQLException e1) { // TODO Auto-generated catch block e1.printStackTrace(); } for (int i=0; i < model.getRowCount(); i++) { model.removeRow(i); model.setRowCount(0); } } }); //------------------------------------------------------- //------------------------------------------------------- frame.setSize(1000,600); frame.setResizable(false); frame.setLayout(null); frame.setUndecorated(true); frame.setLocationRelativeTo(null); frame.setVisible(true); frame.setFocusable(true); frame.getContentPane().setBackground(Color.decode("#37474F")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //-------------------------------------------------------------- } public void connect() throws SQLException { //ENTER PORT, USER, PASSWORD. String url = "jdbc:mysql://localhost:3306/attendance"; String user = "root"; String pass = "password"; con = DriverManager.getConnection(url, user, pass); } public ResultSet dbSearch(String classes, String dt) throws SQLException { //ENTER PORT, USER, PASSWORD. String str1 = "SELECT * from attend, students where attend.stid=students.id AND attend.class = '"+classes+"' AND attend.dt = '"+dt+"'"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); return rst; } public String[] classEt() throws SQLException { String str1 = "SELECT name from class"; Statement stm = con.createStatement(); ResultSet rst = stm.executeQuery(str1); String[] rt = new String[25]; int i=0; while(rst.next()) { rt[i] = rst.getString("name"); i++; } return rt; } public void tblupdt(String classes, String dt) { try { for (int i=0; i < model.getRowCount(); i++) { model.removeRow(i); model.setRowCount(0); } ResultSet res = dbSearch(classes, dt); for(int i=0; res.next(); i++) { model.addRow(new Object[0]); model.setValueAt(res.getInt("stid"), i, 0); model.setValueAt(res.getString("name"), i, 1); model.setValueAt(res.getString("status"), i, 2); } } catch (SQLException e1) { e1.printStackTrace(); } } public void editItem(int id, String status, String date) throws SQLException { String adding = "UPDATE attend SET status = '"+status+"' WHERE stid = "+id+" AND dt = '"+date+"'"; Statement stm = con.createStatement(); stm.executeUpdate(adding); } }
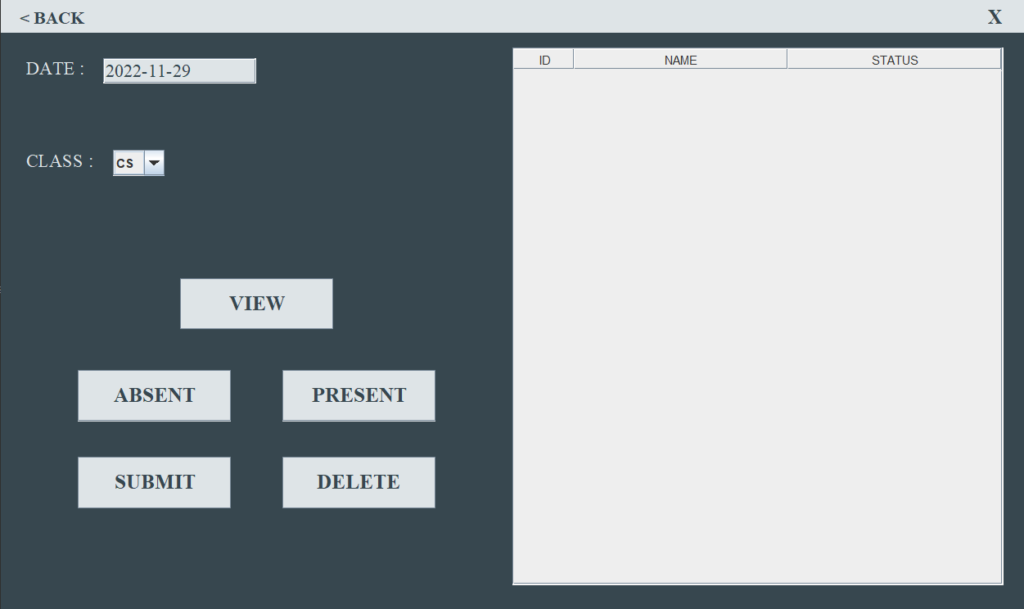
This window here is the output of “EditAttendance.java”. We can just set the date and class to get the records of previously added attendance to edit it. Just use the delete button to delete the whole record of that class of the respective date.
Output for Attendance Management System Project In Java:
The final output of the project will be as shown.
For Admin users:
For Teachers:
For Students:
Conclusion
In this article, we have created an Attendance Management System Project in Java and MySQL. It can create, edit and delete admins, teachers, and students. Also helps to register attendance based on the class of students.
Thank You for visiting our website.
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023
- Snake Game in Java