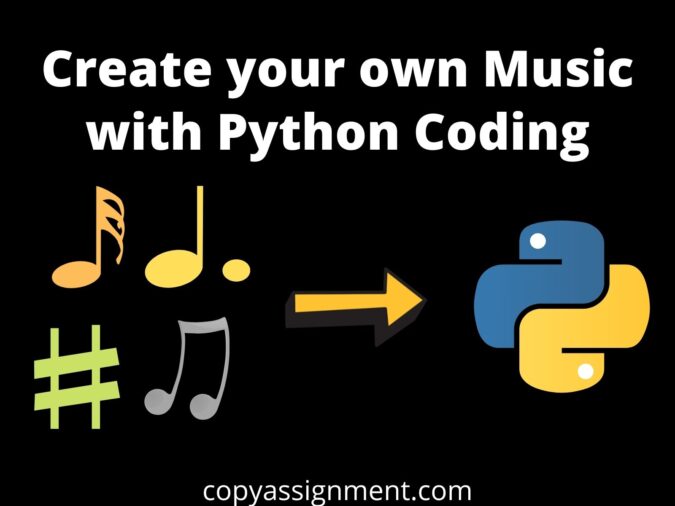
You are here because you want to learn to create your own Music with Python Coding or you are excited to know how someone or you can make music by coding? Right!
And you know what’s the fun part? You don’t need any physical instrument or any type of professional experience to compose your own music with Python.
What do I need?
To create your own Music with coding or to Create your own Music with Python, make sure that you are pretty familiar with Python Programming and you have an up-to-date browser and an Internet Connection. Nothing else is required. Now, let’s start to create your own Music with Python Coding Language. Just follow these simple steps below:–
Step 1: Opening Earsketch Programming Environment
EarSketch is a free educational programming environment. Its core purpose is to teach coding in two widely used languages, Python and Javascript, through music composing and remixing.
Click here to go to Earsketch.
From there, click on “Start Coding” to land on the Programming Interface.
When you click on Start Coding, You will land on a page that must be looking like this:
From this point, you can Either choose to start your tour to get familiar with the Earsketch Environment.
But, I am skipping the tour. So, when you click on Skip… You must be seeing something like this :
Now, You have to click on Create a New Script, It will pop up a dialog box like this, where it will ask you the name of the Script and Script Language, As we are using Python to code here so, choose the scripting language to be Python :
So, When you click on Create, you will see that there is some pre-written code in the Code Editor, that is :
# python code # script_name: # # author: # description: # from earsketch import * init() setTempo(120) finish()
Here, It imports everything from earsketch using, “from earsketch import *”
Then it initializes the whole library using “init()” And then, there is “setTempo(120)”- So, Tempo means the speed of the soundtrack and it is measured in beats per minute (BPM). And setTempo sets the speed of the soundtrack. So, by default, we get it as 120. So we will let it remain the same.
And then there is a “finish()” in the end, what it does is that it ends the
soundtrack.
STEP 2: Let’s create a list of the sound clips that we need in our music
As you can see, there is a Content Manager which has 3,600 sound clips that are produced from various instruments and that we can use in making our own music, without getting any copyright strike. You can browse and preview all the clips and then add them according to your own taste. The sound clips that we are gonna use in this project are added to a list named as sounds are below, but feel free to use any sound clip you like.
sounds=[YG_TRAP_BRASS_1,YG_TRAP_BRASS_2,YG_TRAP_BRASS_3,YG_TRAP_BRA SS_4,YG_TRAP_BRASS_5,HIPHOP_BASSSUB_001,RD_RNB_SFX_TONEBASS_ 1,YG_NEW_HIP_HOP_BASS_1,Y07_BASS]
You can copy this above sounds list inside your code editor.
Now, It’s time to play some music!
STEP 3: Playing Music
Now, we will be playing some music. And the whole sound playing code that we are gonna write is gonna be between setTempo(120) and finish(). But before that, I want to let you know the function that we are gonna use.
We are going to use the “fitMedia()” function to add your sound clip into the
Digital Audio Workstation (DAW) provided to us.
Syntax:
fitMedia(<Track_Name>, <Track_Number>, <Starting_Point>, <Ending_Point>
So we already have our track names stored in our sounds list, now we can simply access them with the help of their index number.
So, now here is an example for you,
fitMedia(sounds[0], 1, 1, 3)
Now what this will do, It’s gonna fit the first track in our sounds list in the Digital Audio Workstation, at Track number 1, from 1st point to the 3rd point.
Now, that’s how we are gonna add other clips to our DAW. But remember that two clips at the same track number, with overlapping Starting and Ending points, can cause a problem to your composed music.
In this project, we want that the whole clip should be of 15 points, i.e. around 28 seconds and we want the Brass tracks (From sounds list 0-4th index) to be played from the point where the previous track ended and all the tracks are gonna be of 3 points each, i.e. around 4 and 1/2 seconds each.
So, what our code is gonna look like :
fitMedia(sounds[0], 1, 1, 3) fitMedia(sounds[1], 2, 3, 6) fitMedia(sounds[2], 3, 6, 9) fitMedia(sounds[3], 4, 9, 12) fitMedia(sounds[4], 5, 12, 15)
As you can see, we have made the ending point of the previous track as the starting point of the next track. And also that we have put all the clips in consecutive track numbers.
Let’s run this:
You must be able to see something like this in your DAW.
Play it and listen to it.
Now, let’s add other clips also to our music.
I am gonna add the Hip-Hop Bass Beats to our music from the 8th point till the end, i.e. the 15th point. So, I am gonna add:
fitMedia(sounds[5], 6, 8,15)
Run and listen to it.
Now, we will add the code for the sound clip at the 6th index, i.e. Tone Bass (RD_RNB_SFX_TONEBASS_1). And we will play it from the 2nd point till the end of the clip.
fitMedia(sounds[6], 7, 2, 15)
Run and listen to it.
Now we will add soft bass to our whole track. This sound clip is at the 7th index of our sounds list. Add the below code:
fitMedia(sounds[7], 8, 1, 15)
Run and listen to it.
Now we will be adding the last clip to our music, i.e. some hard bass from the 1st point till the 8th point because after that we have already put an awesome bass drop sound clip and we are gonna give this clip a fade-in effect also by using a function called setEffect().
We can use many effects in this setEffect() function, like VOLUME, DELAY, DISTORTION, PITCH, etc.
But as we are going to make a Fade in effect, we will be using the VOLUME effect, and it’s GAIN effect parameter. Because we want the clip to gain volume from low volume to the normal volume.
setEffect() takes 7 arguments, that are:
setEffect(<Track_Number_Where_Effect_is_to_be_added>, <EFFECT>,<EFFECT PARAMETER>, START, START_POINT, END, END_POINT)
Now, let’s write the code for it!
fitMedia(sounds[8], 9, 1, 8) setEffect( 9, VOLUME, GAIN, -30, 1, 0, 8)
The above code, adds the bass soundtrack to your DAW and the setEffect()
acts on the 9th track by Gaining the Volume from -30 dB to 0dB, between 1st point to the 8th point.
So, add it and then run it.
The final code snippet must have been looking like this:
# python code # script_name: # # author: # description: # from earsketch import * init() setTempo(120) sounds = [YG_TRAP_BRASS_1,YG_TRAP_BRASS_2,YG_TRAP_BRASS_3,YG_TRAP_BRA SS_4,YG_TRAP_BRASS_5,HIPHOP_BASSSUB_001,RD_RNB_SFX_TONEBASS_ 1,YG_NEW_HIP_HOP_BASS_1,Y07_BASS] fitMedia(sounds[0], 1, 1, 3) fitMedia(sounds[1], 2, 3, 6) fitMedia(sounds[2], 3, 6, 9) fitMedia(sounds[3], 4, 9, 12) fitMedia(sounds[4], 5, 12, 15) fitMedia(sounds[5], 6, 8,15) fitMedia(sounds[6], 7, 2, 15) fitMedia(sounds[7], 8, 1, 15) fitMedia(sounds[8], 9, 1, 8) setEffect( 9, VOLUME, GAIN, -30, 1, 0, 8) finish()
And your final DAW of our “Create your own Music with Python” project must have been looking like this:
See, what I made, check this video:
Listen to the final Music… Good huh!
Well, this article was just to give you a basic understanding of how to use Earsketch and Create your own Music with Python.
You can do a lot more than this. There is a lot of function available to do many cool things, like making your own beat, that will help you to make a remix of songs. You can also add your local audio files in your DAW. There
are a lot of things that you can explore. Keep exploring new things.
Our team hopes for your Magnificent Journey in Coding.
Hope you liked this article.
Thank You!
Also Read:
Python Turtle Design of Spiral Square and Hexagon
Colored Hexagons Turtle Design
Shutdown Computer with Voice in Python
Snake Game in Python using Pygame
Crack Any Password Using Python
Get WiFi Passwords With Python