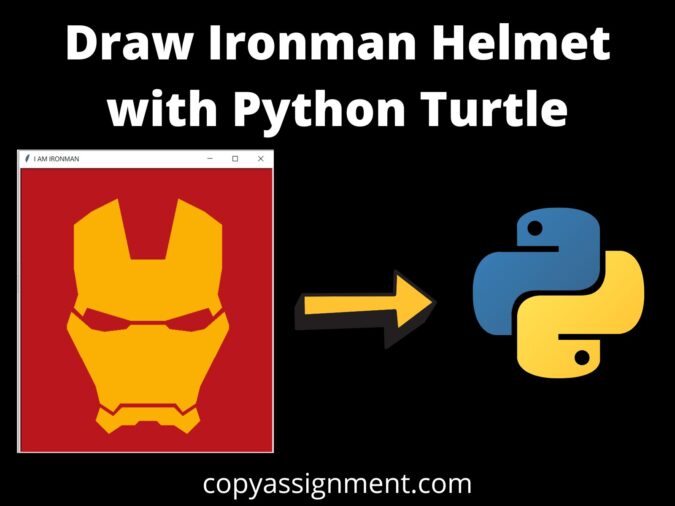
In this tutorial, we will be learning to Draw Ironman Helmet with Python Turtle. We will first read the code and then read the explanation where we will fully understand the code.
Code:
import turtle ankur1 = [[(-40, 120), (-70, 260), (-130, 230), (-170, 200), (-170, 100), (-160, 40), (-170, 10), (-150, -10), (-140, 10), (-40, -20), (0, -20)], [(0, -20), (40, -20), (140, 10), (150, -10), (170, 10), (160, 40), (170, 100), (170, 200), (130, 230), (70, 260), (40, 120), (0, 120)]] ankur2 = [[(-40, -30), (-50, -40), (-100, -46), (-130, -40), (-176, 0), (-186, -30), (-186, -40), (-120, -170), (-110, -210), (-80, -230), (-64, -210), (0, -210)], [(0, -210), (64, -210), (80, -230), (110, -210), (120, -170), (186, -40), (186, -30), (176, 0), (130, -40), (100, -46), (50, -40), (40, -30), (0, -30)]] ankur3 = [[(-60, -220), (-80, -240), (-110, -220), (-120, -250), (-90, -280), (-60, -260), (-30, -260), (-20, -250), (0, -250)], [(0, -250), (20, -250), (30, -260), (60, -260), (90, -280), (120, -250), (110, -220), (80, -240), (60, -220), (0, -220)]] turtle.hideturtle() turtle.bgcolor('#ba161e') # Dark Red turtle.setup(500, 600) turtle.title("I AM IRONMAN") ankur1Goto = (0, 120) ankur2Goto = (0, -30) ankur3Goto = (0, -220) turtle.speed(2) def logo(a, b): turtle.penup() turtle.goto(b) turtle.pendown() turtle.color('#fab104') # Light Yellow turtle.begin_fill() for i in range(len(a[0])): x, y = a[0][i] turtle.goto(x, y) for i in range(len(a[1])): x, y = a[1][i] turtle.goto(x, y) turtle.end_fill() logo(ankur1, ankur1Goto) logo(ankur2, ankur2Goto) logo(ankur3, ankur3Goto) turtle.hideturtle() turtle.done()
Output:

Explanation:
First Part:
- In the first part of the Draw Ironman Helmet, we will import the turtle module and set the variable ankur1,ankur2,ankur3, and its value as below code.
ankur1=[[(-40, 120), (-70, 260), (-130, 230), (-170, 200), (-170, 100), (-160, 40), (-170, 10), (-150, -10), (-140, 10), (-40, -20), (0, -20)],[(0, -20), (40, -20), (140, 10), (150, -10), (170, 10), (160, 40), (170, 100), (170, 200), (130, 230), (70, 260), (40, 120), (0, 120)]]
ankur2=[[(-40, -30), (-50, -40), (-100, -46), (-130, -40), (-176, 0), (-186, -30), (-186, -40), (-120, -170), (-110, -210), (-80, -230), (-64, -210), (0, -210)],[(0, -210), (64, -210), (80, -230), (110, -210), (120, -170), (186, -40), (186, -30), (176, 0), (130, -40), (100, -46), (50, -40), (40, -30), (0, -30)]]
ankur3=[[(-60, -220), (-80, -240), (-110, -220), (-120, -250),(-90, -280), (-60, -260), (-30, -260), (-20, -250), (0, -250)],[(0, -250), (20, -250), (30, -260), (60, -260), (90, -280), (120, -250),(110, -220), (80, -240), (60, -220), (0, -220)]]
- Then we will hide the turtle and set the background color to #ba161e and set the size to 500 / 600.
- Likewise, we will make the above turtle to move in the above codes. Set the speed to 2.
Second Part:
- Create a function name logo() with the parameters “a” and “b”. Inside this function, call the penup(), goto(b),pendown() method and set the color to #fab104 and begin the fill.
- After that inside the function itself, create a for loop with the range of { len(a[0]) }. Inside this loop, set x, y = a [0][i]. Then, goto (x, y).
- Coming out of the loop, again create another for loop with the range of { len(a[1]) }. Inside this loop, set x, y = a[1][i]. Then, goto x,y and coming out of the loop, end the fill.
Last Part:
- In this part just call the functions in the following order and hide the turtle.
logo(ankur1,ankur1Goto)
logo(ankur2,ankur2Goto)
logo(ankur3,ankur3Goto)
Thank You for reading till the end. If you found something wrong in the post, please let us know using comments.
Keep Learning
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle
- Draw Dell Logo using Python Turtle
- Draw Spider web using Python Turtle