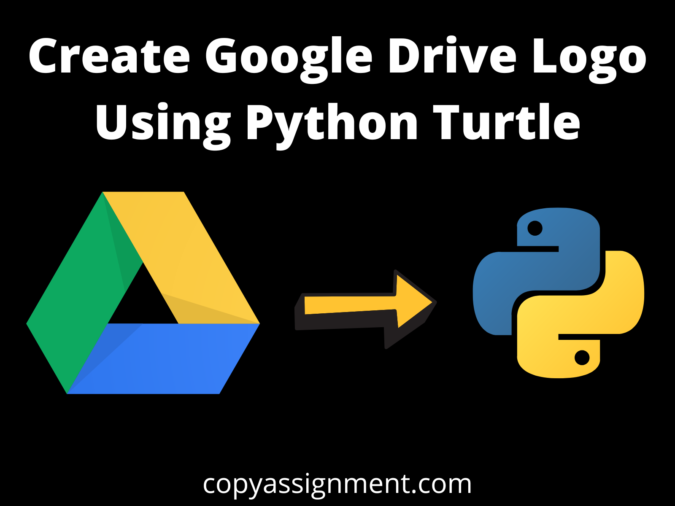
Introduction:
Hello and welcome to the copyassignment where we will learn how to Draw Google Drive Logo Using Python turtle. This could be very interesting for both beginners and experienced coders to learn. This could be simple and easy to understand because we have explained the code in simple terms.
Well, Google Drive is a platform where a free online storage service that allows users to store and access files. The service syncs documents, photos, and other data across all of the user’s devices, including mobile phones, tablets, and computers.
Step 1. Importing Libraries.
#import turtle library
import turtle as t
Step 2: Set the background color and pen color
t.Screen().bgcolor("Black") #set the background color as black
t.pencolor("White") #set pen color as white
Step 3: Make a blue-green and yellow box
#blue box
t.begin_fill() #fill the color
t.fillcolor('#4688F4') #take a blue color hash or t.fillcolor(“blue”)
t.forward(170) #move turtle forward at given pixel
t.left(60) #move turtle 60 degree left
t.forward(50) #move 50 pixel forward
t.left(120) #move turtle 120 degree left
t.forward(220) #move 50 pixel forward
t.left(120) #move turtle 120 degree left
t.forward(50) #move 50 pixel forward
t.end_fill() #end the fill color
#some changes to the steps in the blue box
#green box
t.begin_fill() #fill the color
t.fillcolor('#1FA463') #take a green color hash or t.fillcolor(“green”)
t.left(120) #move turtle 120 degree left
t.forward(200) #move 200 pixel forward
t.left(120) #move turtle 120 degree left
t.forward(50) #move 50 pixel forward
t.left(60) #move turtle 60 degree left
t.forward(150) #move 150 pixel forward
t.left(60) #move turtle 60 degree left
t.forward(50) #move 50 pixel forward
t.end_fill() #end the fill color
Step 4: set the pen positions
t.penup() #This method is used to stop the drawing pen
t.left(120) #move turtle 120 degree left
t.forward(200) #move 200 pixel forward
t.left(120) #move turtle 120 degree left
t.forward(50) #move 50 pixel forward
t.pendown() #Start drawing of the turtle pen
#After blue and green go for yellow box
#yellow box
t.begin_fill() #Start drawing of the turtle pen
t.fillcolor('#FFD048') #take a yellow color hash or t.fillcolor(“yellow”)
t.left(125) #move turtle 125 degree left
t.forward(160) #move 160 pixel forward
t.left(55) #move turtle 55 degree left
t.forward(53) #move 53 pixel forward
t.left(126) #move turtle 126 degree left
t.forward(163) #move 163 pixel forward
t.end_fill() #end the fill color
t.done() #now we complete the code with done function
Complete Code to Draw Google Drive Logo Using Python:
import turtle as t t.hideturtle() t.Screen().bgcolor("Black") t.pencolor("white") t.pensize(0) #blue t.begin_fill() t.fillcolor('#4688F4') t.forward(170) t.left(60) t.forward(50) t.left(120) t.forward(220) t.left(120) t.forward(50) t.end_fill() #green t.begin_fill() t.fillcolor('#1FA463') t.left(120) t.forward(200) t.left(120) t.forward(50) t.left(60) t.forward(150) t.left(60) t.forward(50) t.end_fill() t.penup() t.left(120) t.forward(200) t.left(120) t.forward(50) t.pendown() #yellow t.begin_fill() t.fillcolor('#FFD048') t.left(125) t.forward(160) t.left(55) t.forward(53) t.left(126) t.forward(163) t.end_fill() t.done()
Our code is complete, and we finally run it to see a Google Drive logo.
After running the code, it will open a new window and begin generating the Google Drive logo; after completed, the result should look like this.
Output

I hope you find it useful. You can make minor adjustments to the code to create an interesting logo.
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle
- Draw Dell Logo using Python Turtle
- Draw Spider web using Python Turtle