
In this article, we will build a project on how to extract audio from video using Python. Several libraries and techniques are available in Python for converting Video to Audio. We will use the moviepy library to convert video to audio in this project.
For building this project make sure that you have the latest version of Python installed in your system. Let’s get started!
Extract Audio from Video using Python: Project Overview
Project Name: | Extract Audio from Video using Python |
Abstract | It’s a GUI-based project, we will create a GUI to select any video to extract audio from it. We will use the Tkinter library for GUI. |
Language/s Used: | Python |
IDE | Thonny and PyCharm are recommended |
Python version (Recommended): | Python 3.x |
Database: | Not required |
Type: | Desktop Application |
Recommended for | Beginners and Intermediates |
Steps to Extract Audio from Video using Python with MoviePy library
Handling the video in its raw binary format will become complicated and hence for this purpose, we will make use of an external library called moviepy. MoviePy can read and write all the most common audio and video formats, including GIF, and runs on various operating systems such as Windows, Linux, and macOS, with Python version 2.7 and above.
1. Install moviepy library
This library does not come inbuilt and hence we need to install and we will use the package manager pip for this purpose. To install the moviepy Library, open the terminal and write the following command
pip install moviepy
2. Import moviepy library
Now, let’s get started by importing the libraries. We need to import the moviepy package or the editor class alone specifically.
# Import moviepy
import moviepy.editor
3. Load the video
Before we move to the next step, let’s learn about video formats. We should know our video’s format to do the conversion without any problem. There are many video formats, some of the common formats are WMV (wmv, wma, asf*), OGG (ogg, oga, ogv, ogx), 3GP (3gp, 3gp2, 3g2, 3gpp, 3gpp2) and MP4 (mp4, m4a, m4v, f4v, f4a, m4b, m4r, f4b, mov).
We need to create a VideoFileClip object by referencing the video file through the parameter.
#Load the Video Clip
video = moviepy.editor.VideoFileClip("Video.mp4")
Inside the method, we are adding the path of the video. The video we are going to convert is in mp4 format.
4. Extract the audio
Besides the video format, it’s also a good practice to know some audio formats. Here are some of the common formats MP3, AAC, WMA, and AC3. Now, we have some ideas about both formats. It’s time to do the conversion using the MoviePy library.
We will now extract the audio of the video that we defined. Extracting the audio is as simple as accessing the audio member of the VideoFileClip object that we created. Then we have to write the extracted audio into a different file whose filename has to be specified.
#Extract and export the Audio
video.audio.write_audiofile("Audio.mp3")
We will convert the video to MP3 format, it is the most common one. Depending on the requirement, we can change the format. For example, while using audio for speech recognition, the wav format works better.
# Import moviepy import moviepy.editor #Load the Video video = moviepy.editor.VideoFileClip("Video.mp4") #Extract the Audio audio = video.audio #Export the Audio audio.write_audiofile("Audio.mp3")
Complete Python GUI code for Extracting Audio from Video
We can easily learn and build Tkinter Video to Audio Converter in Python because it has a lot of classes and functions for this purpose. So now, let’s start with the implementation part. You can understand the code line-by-line with the help of comments.
# Import the moviepy library import moviepy.editor as me # Import required functions from the tkinter from tkinter import * from tkinter.filedialog import askopenfilename,asksaveasfilename # Create variable to store audio filename filename='' def convert(): global filename filetypes=(("Audio files","*.mp3 , *.waw , *.ogg"),("All files","*.*")) #Load the Video Clip video=me.VideoFileClip(filename) #Extract the Audio audio=video.audio #Export the Audio file=asksaveasfilename(filetypes=filetypes) audio.write_audiofile(f'{file}{format.get()}') #Create a label to display Converted label5=Label(root,text="Converted!!!",font=("Arial",18),fg="green") label5.config(bg="pink") label5.pack() label5.place(x=450,y=300) def select(): global filename filetypes = ( ('video files', '*.WEBM , *.MPG, *.MP2 , *.MPEG , *.MPE , *.MPV , *.MP4 , *.M4P , *.M4V , *.AVI , *.WMV , *.MOV , *.QT , *.FLV , *.SWF , *.AVCHD'), ('All files', '*.*') ) filename=askopenfilename(filetypes=filetypes) # Create a label to diaplay video selected label3.config(text="Video Selected",fg="green") label3.config(bg="pink") # Create a label label4=Label(root,text="Select Audio format",font=("Arial",18)) label4.config(bg="pink") label4.pack() label4.place(x=125,y=250) # Create options to choose audio format options=[".mp3",".ogg",".wav"] format.set(".mp3") menu=OptionMenu(root,format,*options) menu.pack() menu.place(x=375,y=250) # Create a button to convert video to audio button3=Button(root,text="Export",bg='light blue',font=("Harlow Solid",12),command=convert,width=10,height=1) button3.pack() button3.place(x=250,y=300) # Create the basic GUI window root=Tk() # Set the background color of GUI application root.configure(bg='pink') # Set the geometry of the GUI application root.geometry("700x450") root.minsize(600,350) root.maxsize(600,350) # Set the title of the GUI application root.title("Video to Audio Converter") # Create a label for heading label1=Label(root,text="Extract Audio from Video",font=("Arial",32)) label1.config(bg="pink") label1.pack() # Create a label to display Select any Video file label2=Label(root,text="Select any Video file",font=("Arial",18)) label2.config(bg="pink") label2.pack() label2.place(x=190,y=100) # Create a button for choosing video button1=Button(root,text="Choose file",bg='light blue',font=("Harlow Solid",12),command=select,width=10,height=1) button1.pack() button1.place(x=250,y=200) label3=Label(root,font=("Footlight MT",18,"bold")) label3.pack() label3.place(x=225,y=150) format=StringVar() # Start the GUI application root.mainloop()
Steps to use this GUI app to Extract Audio from Video using Python
Step 1:

Step 2:

Step 3:
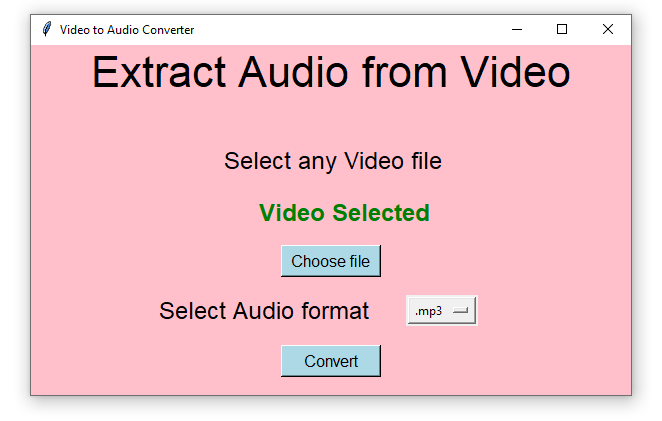
Step 4:
Once we click on the convert button, we need to specify the name of the audio file which needs to be saved.

We can see that our Audio file is saved in the corresponding folder.
Conclusion
In this article, we learn how to convert video to audio using two ways. We first saw how to use moviepy library to extract audio from video. Then, we built a GUI application to convert video to audio using Python. There may be many other ways using them you can do this task. Hope this article was helpful for you to learn how to extract audio from video using python. Happy Learning!
Also Read:
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- MoviePy: Python Video Editing Library
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter