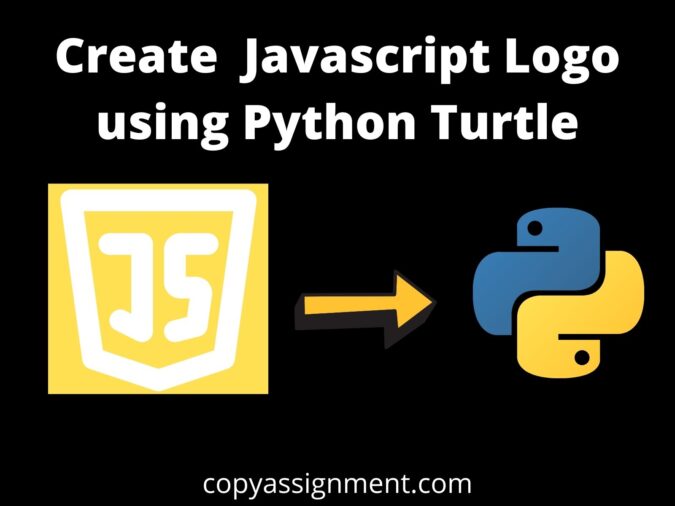
Introduction
Hello, and welcome friends to learn a new topic today. We are going to draw the Javascript Logo using a python turtle module. It’s super easy and exciting to learn as we have used simple lines of code. It is understandable to all the beginners interested in learning python. We provide all the explanations and comments for the lines of code as it helps in easy understanding of code.
We have many project topics on python turtle and articles written on that, so keep visiting our website www.violet-cat-415996.hostingersite.com
For the complete code, move to the bottom of the page and you will get the entire code along with the output.
So let’s begin drawing Javascript Logo
Import Turtle
import turtle t=turtle.Turtle()
These lines of code import the module turtle. We have created the object t of the turtle module to access its methods and functions.
Draw the outer box of Javascript Logo using Python Turtle
t.goto(-20,-70) #set the color t.color("#F0DB4F","#F0DB4F") t.begin_fill() t.pendown() t.left(165) t.forward(100) t.right(70) t.forward(220) t.setheading(0) t.forward(229) t.penup() t.goto(-20,-70) t.setheading(0) t.pendown() t.left(15) t.forward(100) t.left(70) t.forward(229) t.end_fill() t.penup()
In this block of code, we have initialized the position of the turtle to goto(-20,-70). The color is initialized to #F0DB4F. Here we have initialized the position to goto(-20,-70) twice. Drawn the outer line of the logo from both sides with the specified coordinates and the distance.
Draw the ‘J’ of the javascript logo
#set the position to draw 'J' t.goto(-35,-20) t.setheading(90) t.color("white","white") t.pendown() t.begin_fill() t.forward(150) t.left(90) t.forward(20) t.left(90) t.forward(130) t.setheading(90) t.left(75) t.forward(40) t.left(110) t.forward(20) t.penup() t.goto(-35,-20) t.setheading(90) t.left(75) t.pendown() t.forward(60) t.end_fill() t.penup()
In this piece of code, we have to initialise the position of the turtle to goto(-35,-20). The heading position is 90. The color is initialized to white, the forward and left functions are used to draw the ‘J’ letter of the Javascript Logo.
Draw the yellow box for ‘S’ Letter
t.color(“yellow”,”yellow”)
#Set the position
t.goto(-20,-55)
t.setheading(90)
t.pendown()
t.begin_fill()
t.forward(215)
t.right(90)
t.forward(100)
t.right(95)
t.forward(195)
t.left(90)
t.end_fill()
t.penup()
In this block of code, we have to initialize the color to yellow and initialize the position to goto(-20,-55). The heading position is 90 i.e North . Here we have drawn the yellow box by using Python turtle module functions.
Draw the ‘S ‘ letter of the javascript Logo
#Draw the S of the Dell Logo t.color("white","white") t.pensize(2) t.goto(-10,-20) t.begin_fill() t.setheading(0) t.left(15) t.pendown() t.forward(65) t.left(70) t.forward(70) t.left(90) t.left(15) t.forward(50) t.right(100) t.forward(50) t.right(90) t.forward(50) t.left(85) t.forward(20) t.left(95) t.forward(70) t.left(90) t.forward(90) t.left(100) t.forward(50) t.right(105) t.forward(37) t.right(75) t.forward(50) t.left(85) t.forward(20) t.end_fill()
We have to draw the ‘ S’ letter of the Javascript logo. Initialized the position to goto(-10,-20). Here we have made use of forward , left and right functions to generate the S letter where forward() is used to move forward a specified distance .left to move left angle and right to move right angle while drawing the S letter of javascript logo i.e JS.
Complete Code to Draw Javascript Logo using Python Turtle
#import Turtle import turtle t=turtle.Turtle() t.penup() #set the position of turtle to draw the outline of Logo t.goto(-20,-70) #set the color t.color("#F0DB4F","#F0DB4F") t.begin_fill() t.pendown() t.left(165) t.forward(100) t.right(70) t.forward(220) t.setheading(0) t.forward(229) t.penup() t.goto(-20,-70) t.setheading(0) t.pendown() t.left(15) t.forward(100) t.left(70) t.forward(229) t.end_fill() t.penup() #set the position to draw 'J' t.goto(-35,-20) t.setheading(90) t.color("white","white") t.pendown() t.begin_fill() t.forward(150) t.left(90) t.forward(20) t.left(90) t.forward(130) t.setheading(90) t.left(75) t.forward(40) t.left(110) t.forward(20) t.penup() t.goto(-35,-20) t.setheading(90) t.left(75) t.pendown() t.forward(60) t.end_fill() t.penup() #Draw the yellow box for 's' letter t.color("yellow","yellow") #Set the position t.goto(-20,-55) t.setheading(90) t.pendown() t.begin_fill() t.forward(215) t.right(90) t.forward(100) t.right(95) t.forward(195) t.left(90) t.end_fill() t.penup() #Draw the S of the Dell Logo t.color("white","white") t.pensize(2) t.goto(-10,-20) t.begin_fill() t.setheading(0) t.left(15) t.pendown() t.forward(65) t.left(70) t.forward(70) t.left(90) t.left(15) t.forward(50) t.right(100) t.forward(50) t.right(90) t.forward(50) t.left(85) t.forward(20) t.left(95) t.forward(70) t.left(90) t.forward(90) t.left(100) t.forward(50) t.right(105) t.forward(37) t.right(75) t.forward(50) t.left(85) t.forward(20) t.end_fill() t.hideturtle() turtle.done()
Output
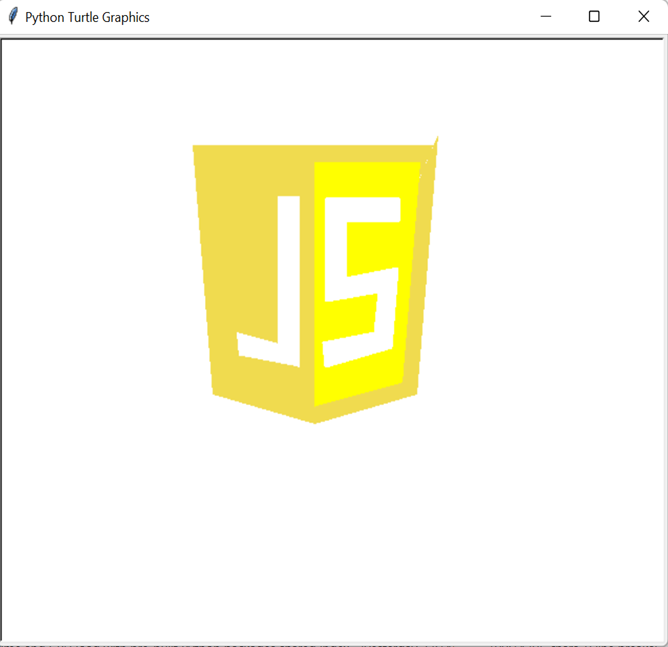
Here you will see, that we have successfully created the Javascript logo using the Python Turtle module
For more articles like this, keep visiting our website.
Thank you for reading this article.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector