
Introduction
Today’s topic will be how to Draw Instagram Reel Logo Using Python Turtle.
Hey guys, tell us something that we all use in our daily lives. Someone wants to know how the Instagram reel logo was created. Can we create this logo in the simplest and most basic programming language, Python? Definitely! We can do it.
Reels on Instagram are short, amusing videos in which you can express your creativity and bring your business to life. People come to Reels to be a part of cultural trends, collaborate with the community, and explore new ideas.
Step 1: Importing Turtle Library
#import turtle library
import turtle as t
Step 2: Set the Pen Size, color, speed, and Background Color
t.speed(100) #speed set as 100
t.bgcolor('#fb3958') #red instagram hash color
t.pencolor('white') #set pen color as white
t.pensize(10) #set pen size 10
Step 3: Draw a Squircles(the words “square” and “circle”)
t.penup() #This method is used to stop the drawing pen
t.goto(100,100) #This method is used to move the turtle to an absolute position. This method has Aliases: goto, stop
t.pendown() #Start drawing of the turtle pen.
t.left(180) #move turtle 180 degree left
def border(): #declare a function border
t.forward(150) #moves the pen in the forward direction by 150 pixel.
for i in range(90): #for loop till 90
t.forward(1) #move 1 pixel forward
t.left(1) #move turtle 1 degree left
border() # call the function border
border()
border()
border()
Step 4: Draw Creating A Horizontal Line
t.penup() #This method is used to put an end to the drawing pen.
for i in range(90): #for loop till 90
t.backward(1) #move backward to 1
t.right(1) #move turtle 1 degree right
t.left(90) #move turtle 90 degree left
t.pendown() #Start drawing of the turtle pen
t.forward(259) #move 259 pixel forward
Step 5: Draw First Diagonal Line.
t.penup() #stop the drawing pen
t.right(180) #move turtle 180 degree right
t.forward(85) #move 85 pixel forward
t.left(120) #move turtle 120 degree left
t.pendown() #Start drawing of the turtle pen
t.forward(60) #move 60 pixel forward
t.right(120) #move turtle 120 degree right
Step 6: Draw Second Diagonal Line.
t.penup() #stop the drawing pen
t.forward(100) #move 100 pixel forward
t.right(60) #move turtle 60 degree right
t.pendown() #Start drawing of the turtle pen
t.forward(60) #move 60 pixel forward
Step 7: Draw a Triangle.
t.penup() #stop the drawing pen
t.goto(5,-20) #add that to the coordinates x and y axis
t.left(60) #move turtle 60 degree left
t.right(90) #move turtle 90 degree right
t.pendown() #Start drawing of the turtle pen
for i in range(3): #for loop till 3
t.forward(70) #move 70 pixel forward
t.left(120) #move turtle 120 degree left
t.hideturtle() # this method is used to make the turtle invisible
t.done() #now we complete the code with done function
To see the results, simply copy and paste the code into your desired environment, or use an online Python compiler.
Source Code to Draw Instagram Reel Logo Using Python:
import turtle as t t.speed(100) t.bgcolor('#fb3958') t.pencolor('white') t.pensize(10) t.penup() t.goto(100,100) t.pendown() t.left(180) def border(): t.forward(150) for i in range(90): t.forward(1) t.left(1) border() border() border() border() t.penup() # Creating A Horizontal Line and One Diagonal Line for i in range(90): t.backward(1) t.right(1) t.left(90) t.pendown() t.forward(259) t.penup() t.right(180) t.forward(85) t.left(120) t.pendown() t.forward(60) t.right(120) # Draw another Diagonal Line t.penup() t.forward(100) t.right(60) t.pendown() t.forward(60) #Draw a Triangle t.penup() t.goto(5,-20) t.left(60) t.right(90) t.pendown() for i in range(3): t.forward(70) t.left(120) t.hideturtle() t.done()
Our code is finished, and we run it to see an Instagram reel logo.
After running the code, a new window will open and the Instagram reel logo will be generated; when finished, the result should look like this.
Output
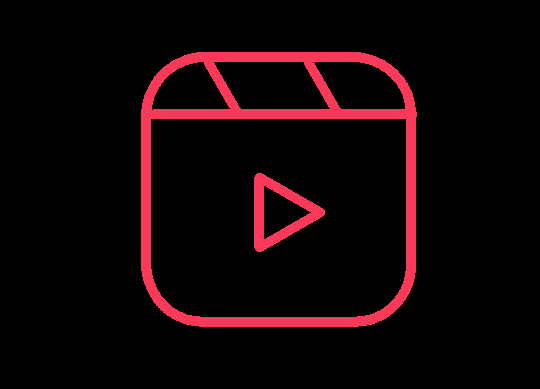
Thank you for reading this article.
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle
- Draw Dell Logo using Python Turtle
- Draw Spider web using Python Turtle