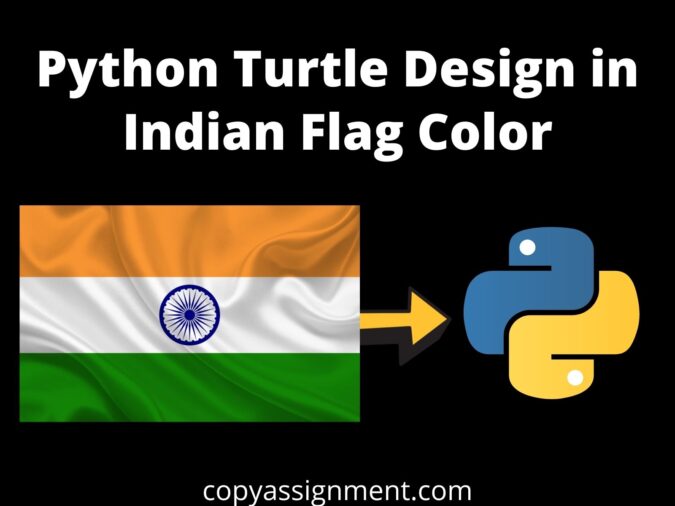
In this article, we will be learning about a very cool Design using Python Turtle which uses Indian flag color. The code for the turtle program is given below. Accordingly, this article will explain to you how you can code in turtle that will allow you to create the shapes. We will first see the code and then, we will understand it line-by-line. Now let’s see the code for design.
Code:
import turtle turtle.bgcolor('black') wn=turtle.Screen() tr=turtle.Turtle() move=1 tr.speed("fastest") for i in range (360): tr.write("Welcome ",'false','center',font=('Showcard gothic',50)) tr.penup() tr.goto(-200,100) tr.pendown() tr.color("orange") tr.right(move) tr.forward(100) tr.penup() tr.color("white") tr.pendown() tr.right(30) tr.forward(60) tr.pendown() tr.color("light green") tr.left(10) tr.forward(50) tr.right(70) tr.penup() tr.pendown() tr.color('light blue') tr.forward(50) tr.color('light green') tr.pu() tr.pd() tr.color("light blue") tr.forward(100) tr.color('brown') tr.forward(200) tr.pu() tr.pd() tr.color('light green') tr.circle(2) tr.color('light blue') tr.circle(4) tr.pu() tr.fd(20) tr.pd() tr.circle(6) tr.pu() tr.fd(40) tr.pd() tr.circle(8) tr.pu() tr.fd(80) tr.pd() tr.circle(10) tr.pu() tr.fd(120) tr.pd() tr.circle(20) tr.color('yellow') tr.circle(10) tr.pu() tr.pd() tr.color('white') tr.forward(150) tr.color('red') tr.fd(50) tr.color ('blue') tr.begin_fill() tr.penup() tr.home() move=move+1 tr.penup() tr.forward(50) turtle.done()
Output:
Explanation:
- First, import turtle and set the background color to black and let the variable “wn” be the turtle.Screen() and the variable “tr” to turtle.Turtle()
- Likewise, let the value of the “move” variable be 1, and speed is the fastest. Similarly, write a for loop with the range of 360. This will help draw the figure from every angle.
- Accordingly, write “Welcome” with the code in line 10. Then lift the pen and goto (-200, 100) and keep the pen down. Make the color of “tr” to orange and move right, forward at an angle of 100 degrees, and lift the pen.
- Now, make the color of the pen white and lift the pen. And move right at an angle of 30 degrees and forward at an angle of 60 degrees. And put the pen down.
- Subsequently, change the color to light green and move left, forward, and right at an angle of 10, 50, and 70 simultaneously. Then just put the pen down.
- Now, change the color of the pen to light blue, move forward at 50 degrees. And again change the color to light green. Then call the functions pu() and pd() that mean pen up and pen down turtle writing.
- Similarly, make the color light blue and move forward at 100. In addition, make the color brown and move forward 200. And call the pu() , fd() with the parameter 20, and pd() simultaneously.
- Additionally, call the circle() function with the parameter 6, the pu() function, the fd() function with the parameter 20 and the pd() function.
- Continuing, call the circle() function with the parameter 6, the pu() function, the fd() function with the parameter 40 and finally the pd() function.
- Call the circle, pu, fd, pd accordingly.
- Diverging, change the color to yellow and call the circle(10), pu(), and pd().
- Again, change the color to white and move forward at 150. Then change the color to red and call fd(50)
- Lastly, change the pen to blue and call the function begin_fill(). Lift the pen up and call home(). Add 1 to the “move” variable.
- Coming out of the loop, move forward 50 and call turtle.done()
Comment your queries or anything you want to know or tell us based on this post or website.
Thanks for reading
Keep Learning
Also read:
Python Turtle Beautiful Design
Snake Game in Python using Pygame
Rock Paper Scissor Game Using Python Tkinter
Password Generator Application In Python
Colored Hexagons Turtle Design