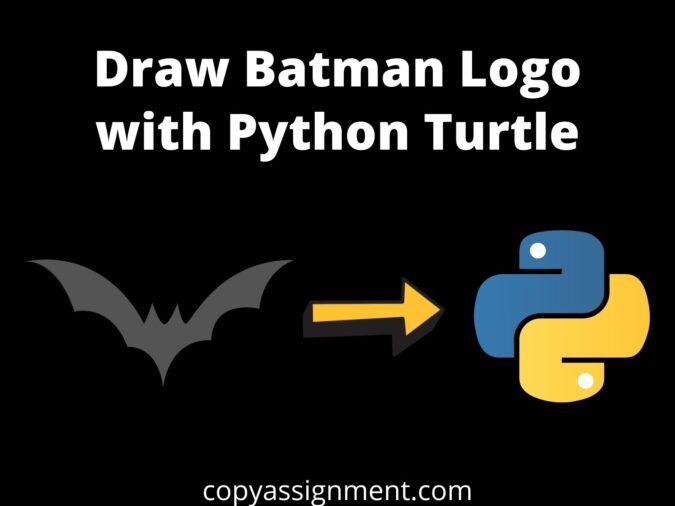
In this tutorial, we will be learning how we can draw batman logo with Python turtle. We will first read the code and read the explanation to fully understand the code.
Code:
import turtle import math kalam = turtle.Turtle() kalam.speed(500) window = turtle.Screen() window.bgcolor("#000000") kalam.color("yellow") ankur = 20 kalam.left(90) kalam.penup() kalam.goto(-7 * ankur, 0) kalam.pendown() for a in range(-7 * ankur, -3 * ankur, 1): x = a / ankur rel = math.fabs(x) y = 1.5 * math.sqrt((-math.fabs(rel - 1)) * math.fabs(3 - rel) / ((rel - 1) * (3 - rel))) * ( 1 + math.fabs(rel - 3) / (rel - 3)) * math.sqrt(1 - (x / 7) ** 2) + ( 4.5 + 0.75 * (math.fabs(x - 0.5) + math.fabs(x + 0.5)) - 2.75 * ( math.fabs(x - 0.75) + math.fabs(x + 0.75))) * (1 + math.fabs(1 - rel) / (1 - rel)) kalam.goto(a, y * ankur) for a in range(-3 * ankur, -1 * ankur - 1, 1): x = a / ankur rel = math.fabs(x) y = (2.71052 + 1.5 - 0.5 * rel - 1.35526 * math.sqrt(4 - (rel - 1) ** 2)) * math.sqrt( math.fabs(rel - 1) / (rel - 1)) kalam.goto(a, y * ankur) kalam.goto(-1 * ankur, 3 * ankur) kalam.goto(int(-0.5 * ankur), int(2.2 * ankur)) kalam.goto(int(0.5 * ankur), int(2.2 * ankur)) kalam.goto(1 * ankur, 3 * ankur) print("Batman Logo with Python Turtle") for a in range(1 * ankur + 1, 3 * ankur + 1, 1): x = a / ankur rel = math.fabs(x) y = (2.71052 + 1.5 - 0.5 * rel - 1.35526 * math.sqrt(4 - (rel - 1) ** 2)) * math.sqrt( math.fabs(rel - 1) / (rel - 1)) kalam.goto(a, y * ankur) for a in range(3 * ankur + 1, 7 * ankur + 1, 1): x = a / ankur rel = math.fabs(x) y = 1.5 * math.sqrt((-math.fabs(rel - 1)) * math.fabs(3 - rel) / ((rel - 1) * (3 - rel))) * ( 1 + math.fabs(rel - 3) / (rel - 3)) * math.sqrt(1 - (x / 7) ** 2) + ( 4.5 + 0.75 * (math.fabs(x - 0.5) + math.fabs(x + 0.5)) - 2.75 * ( math.fabs(x - 0.75) + math.fabs(x + 0.75))) * (1 + math.fabs(1 - rel) / (1 - rel)) kalam.goto(a, y * ankur) for a in range(7 * ankur, 4 * ankur, -1): x = a / ankur rel = math.fabs(x) y = (-3) * math.sqrt(1 - (x / 7) ** 2) * math.sqrt(math.fabs(rel - 4) / (rel - 4)) kalam.goto(a, y * ankur) for a in range(4 * ankur, -4 * ankur, -1): x = a / ankur rel = math.fabs(x) y = math.fabs(x / 2) - 0.0913722 * x ** 2 - 3 + math.sqrt(1 - (math.fabs(rel - 2) - 1) ** 2) kalam.goto(a, y * ankur) for a in range(-4 * ankur - 1, -7 * ankur - 1, -1): x = a / ankur rel = math.fabs(x) y = (-3) * math.sqrt(1 - (x / 7) ** 2) * math.sqrt(math.fabs(rel - 4) / (rel - 4)) kalam.goto(a, y * ankur) kalam.penup() kalam.goto(300, 300) turtle.done()
Output:

Explanation:
First Part:
- In the first part of Draw Batman Logo with Python Turtle, we will import the turtle and the math module. Then, we will set “kalam” as the turtle pen and speed as 500. Now, set the screen of the turtle to “window” and set the background color of the screen to black and the pen color to yellow.
- Likewise, set the value of the “ankur” variable as 20.
Second Part:
- Move the turtle left at an angle of 90 degrees and pick the pen up. Then, goto(-1*ankur,0) and put the pen down.
- We will now be using the math module. Similarly, create a for loop with the range of { -7*ankur,-3*ankur,1 }. Inside this loop, set the value of the “x” variable as (a / ankur). Then, set the value of “rel” to math.fabs(x). This will return the float absolute of the “x”. Likewise, we will set the value of y as { (-math.fabs(rel-1))*math.fabs(3-rel)/((rel-1)*(3-rel)))*(1+math.fabs(rel-3)/(rel-3))*math.sqrt(1-(x/7)**2)+(4.5+0.75*(math.fabs(x-0.5)+math.fabs(x+0.5))-2.75*(math.fabs(x-0.75)+math.fabs(x+0.75)))*(1+math.fabs(1-rel)/(1-rel) } in side of a sqrool of the power 1.5; which will determine the angles of the turtle. Similarly, take the “kalam” to (a, y*ankur)
- Coming out of the loop, make the kalam go to (-1*ankur, 3*ankur). Do the same as in the code below.
kalam.goto(-1*ankur,3*ankur)
kalam.goto(int(-0.5*ankur),int(2.2*ankur))
kalam.goto(int(0.5*ankur),int(2.2*ankur))
kalam.goto(1*ankur,3*ankur)
Third Part:
- In this part of the code, create a for loop with the range of { 1*ankur+1,3*ankur+1,1 }. Inside this loop, set the value of “x” variable as (a/ankur) and set rel as in the second part. All the other for loops are of the same type but with different ranges and arguments.
- At last, pick the pen up and goto(300, 300) and end the code with exitonclick() method.
Thank You for reading till the end. Comment your queries if you have any.
Keep Learning.
Also Read:
Draw Doraemon with Python Turtle
Draw Ironman Helmet with Python Turtle
Python Turtle Design of Spiral Square and Hexagon
Many Beautiful Designs using Python Turtle
Python Turtle Design of Circle Mania