The factorial of a number is defined as the product of all natural numbers up to that number. For example, factorial of 5 or 5! is 1 x 2 x 3 x 4 x 5 = 120.
Extra long factorials are factorials of numbers greater than 20. They can be very big with an order of magnitude greater than 2^64 which can not be stored even in long long data types in C or C++. Modern languages like Python and JavaScript can easily store them.
Python Code for Extra Long Factorials Hackerrank Solution
def extraLongFactorials(n):
# Write your code here
fact = 1
for num in range(2, n+1):
fact *= num
print(fact)
if __name__ == '__main__':
n = int(input().strip())
extraLongFactorials(n)
Input
25
Output
15511210043330985984000000
JS Code for Extra Long Factorials Hackerrank Solution
Javascript output large numbers in scientific notation. We will get the output of 25! as 1.5511210043330984e+25. To avoid this we have to use BigInt.
function extraLongFactorials(n) {
// Write your code here
var fact = BigInt(1)
for(var num=2n; num<=n; num++){
fact = fact * num
}
console.log(fact.toString())
}
function main() {
const n = parseInt(readLine().trim(), 10);
extraLongFactorials(n);
}
Output
15511210043330985984000000
HackerRank Snapshot
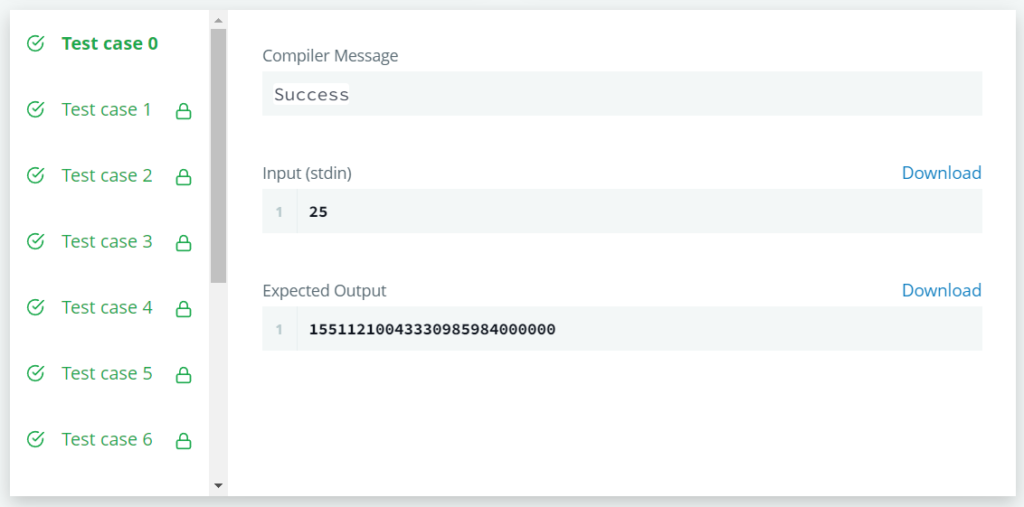
Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- HackerRank Day 8 Solution in Python: Dictionaries and Maps
- HackerRank Day 7 Solution in Python: Arrays
- HackerRank Day 6 Solution in Python: Let’s review
- HackerRank Day 5 Solution in Python: Loops
- HackerRank Day 4 Solution in Python: Class vs Instance
- HackerRank Day 3 Solution in Python: Intro to Conditional Statements
- HackerRank Day 2 Solution in Python: Operators
- HackerRank Day 1 Solution in Python: Data Types
- HackerRank Day 0 Solution in Python: Hello World
- HackerRank Day 29 Solution in Python: Bitwise AND
- HackerRank Day 28 Solution in Python: RegEx, Patterns, and Intro to databases
- HackerRank Day 27 Solution in Python: Testing
- HackerRank Day 26 Solution in Python: Nested Logic
- HackerRank Day 25 Solution in Python: Running Time and Complexity
- HackerRank Day 24 Solution in Python: More Linked Lists
- HackerRank Day 23 Solution in Python: BST Level Order Traversal
- HackerRank Day 22 Solution in Python: Binary Search Trees
- Find Peak Element LeetCode 162
- HackerRank Day 20 Solution in Python: Sorting
- HackerRank Day 19 Solution in Python: Interfaces
- HackerRank Day 18 Solution in Python: Queues and Stacks
- HackerRank Day 17 Solution in Python: More Exceptions
- HackerRank Day 16 Solution: Exceptions – String to Integer
- Explained: Allocate minimum number of pages
- HackerRank Day 15 Solution in Python: Linked List
- Search a 2D matrix: leetcode 74
- Maximum Subarray Sum: Kadane’s Algorithm
- HackerRank Day 13 Solution in Python: Abstract Classes
- HackerRank Day 14 Solution in Python: Scope