What is factorial?
The factorial of a number(non-negative integers) is the product of all positive numbers that are less than or equal to the number itself. The factorial for negative numbers is not defined and for 0 it is 1(exception). It is denoted by !
Formula:
n! = n x (n-1) x (n-2) x (n-3) … 1
Examples:
1! => 1 => 1
2! => 2 x 1 => 2
3! => 3 x 2 x 1 => 6
4! => 4 x 3 x 2 x 1 => 24
5! => 5 x 4 x 3 x 2 x 1 => 120
We will find the factorial of a number in Python using 6 different methods. Let’s start
Method 1: Using for loop
n = int(input('Enter number: ')) factorial = 1 for i in range(1, n+1): factorial = factorial * i print('Enter value greater than equal to 0' if n < 0 else f'Factorial of {n} is {factorial}')
Method 2: Using while loop
num = int(input('Enter number: ')) n = num factorial = 1 while n >= 1: factorial = factorial * n n = n - 1 print('Enter value greater than equal to 0' if n < 0 else f'Factorial of {num} is {factorial}')
Method 3: With function using for loop
def factorial(n): num = 1 for i in range(1, n+1): num = num * i return num n = int(input('Enter number: ')) print('Enter value greater than equal to 0' if n < 0 else f'Factorial of {n} is {factorial(n)}')
Method 4: With function using while loop
def factorial(n): num = 1 while n > 1: num = num * n n = n - 1 return num n = int(input('Enter number: ')) print('Enter value greater than equal to 0' if n < 0 else f'Factorial of {n} is {factorial(n)}')
Method 5: With recursive function
def factorial(n): if n > 1: return n * factorial(n-1) return 1 n = int(input('Enter number: ')) print('Enter value greater than equal to 0' if n < 0 else f'Factorial of {n} is {factorial(n)}')
Method 6: Using in-built factorial() method of math module
import math n = int(input('Enter number: ')) print('Enter value greater than equal to 0' if n < 0 else f'Factorial of {n} is {math.factorial(n)}')
Output:
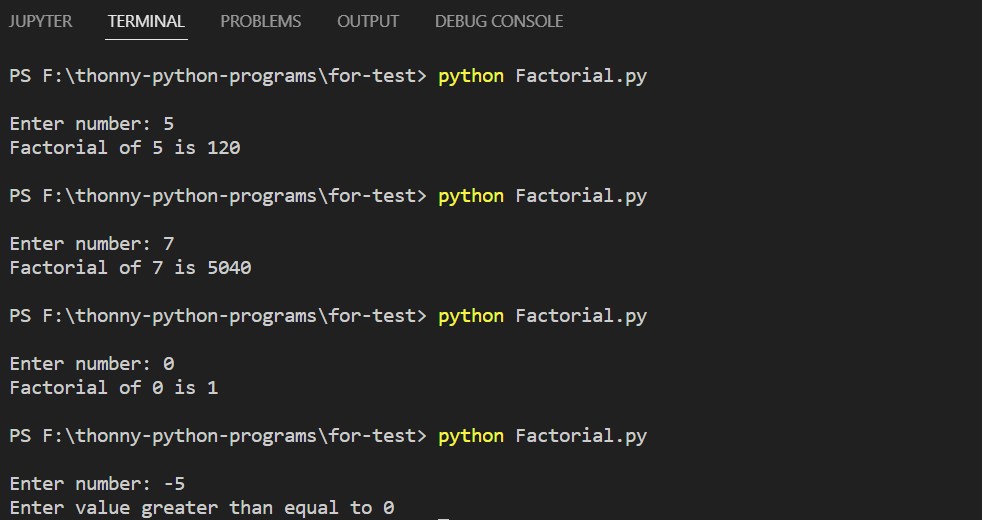
Also Read:
- Python | Check Armstrong Number using for loop
- Python | Factorial of a number using for loop
- Create your own ChatGPT with Python
- Filter List in Python | 10 methods
- Python | Check if a string is a palindrome or not without Recursion
- Python | Check if a number is an Armstrong Number or not using Recursion
- Python | Check if a number is an Armstrong Number or not without using Recursion
- Python | Shuffle a list using recursion
- Python | Shuffle a list without recursion
- Python | Implementing switch case using functions
- Python | Find LCM using function
- Python | Find HCF using function
- Python | Convert the binary number to decimal without using library function
- Python | Create a basic operations calculator(+, -, /, and x), create a separate function for each operation
- Python | Detecting the number of local variables declared in a function
- Python | Making a chain of function decorators (bold italic underline etc)
- Python | Access function inside a function
- Python | Create a function with a pass statement
- Python | Function to calculate the square root of a number
- Python | A function that calculates the power of a number
- Python | A function that accepts 2 integers and adds them and returns their sum
- Python | Function that takes a list of integers and returns the last integer
- Python | Return multiple values from a function
- Python function that takes a list and returns a new list with unique elements of the first list
- Python | Generate a random number in the range of 1 to 10 using the function
- Python | Check Whether a Given Number is Even or Odd using Recursion
- Python | Print Binary Equivalent of an Integer using Recursion
- Python | Print Binary Equivalent of a Number without Using Recursion
- Python | Reverse a string using recursion
- Python | Find Sum of Digit of a Number Without Recursion