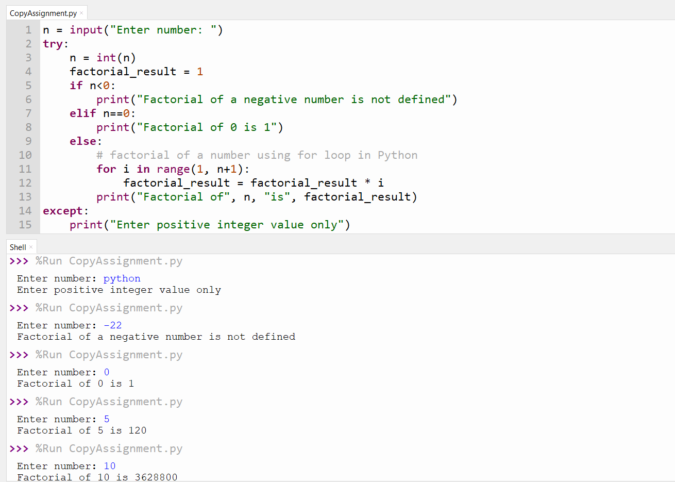
The factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n. The factorial of 0 is defined to be 1. The factorial formula can be expressed as:
n! = 1 * 2 * 3 * … * n
Code to calculate factorial of a number using for loop in Python
n = input("Enter number: ") try: n = int(n) factorial_result = 1 if n<0: print("Factorial of a negative number is not defined") elif n==0: print("Factorial of 0 is 1") else: # factorial of a number using for loop in Python for i in range(1, n+1): factorial_result = factorial_result * i print("Factorial of", n, "is", factorial_result) except: print("Enter positive integer value only")
Output:
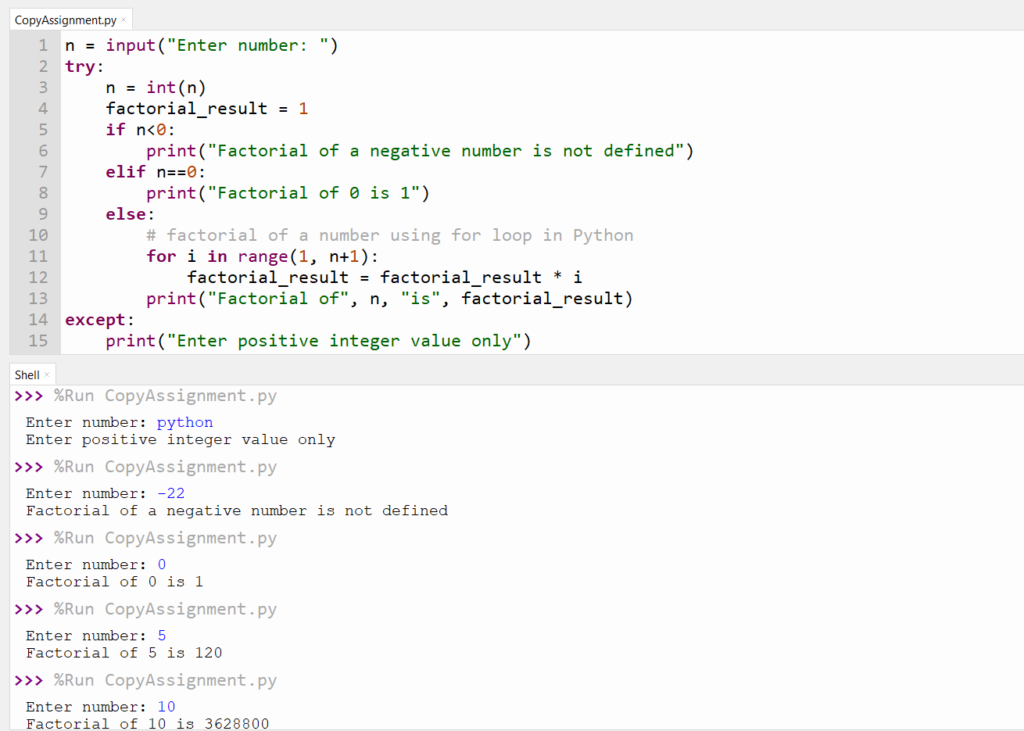
Also read:
- Python | Asking the user for input until they give a valid response
- Python | How to iterate through two lists in parallel?
- Python | How to sort a dictionary by value?
- Python | Remove items from a list while iterating
- Python | How to get dictionary keys as a list
- How to represent Enum in Python?
- 5 methods | Flatten a list of lists | Convert nested list into a single list in Python
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- Create your own ChatGPT with Python
- Filter List in Python | 10 methods
- Radha Krishna using Python Turtle
- Yield Keyword in Python
- Python Programming Examples | Fundamental Programs in Python
- Python | Delete object of a class
- Python | Modify properties of objects
- Python classmethod
- Python | Create a class that takes 2 parameters, print both parameters
- Python | Create a class, create an object of the class, access, and print property value
- Python | Calculator using lambda
- Python | Multiply numbers using lambda
- Python | Print Namaste using lambda
- Iterate over a string in Python
- Python | join() | Join list of strings
- Python | isalnum() method | check if a string consists only of alphabetical characters or numerical digits
- Python | isupper() and islower() methods to check if a string is in all uppercase or lowercase letters
- Python | count substring in a String | count() method
- Python | enumerate() on String | Get index and element
- Python | Convert a String to list using list() method
- Euler’s Number in Python