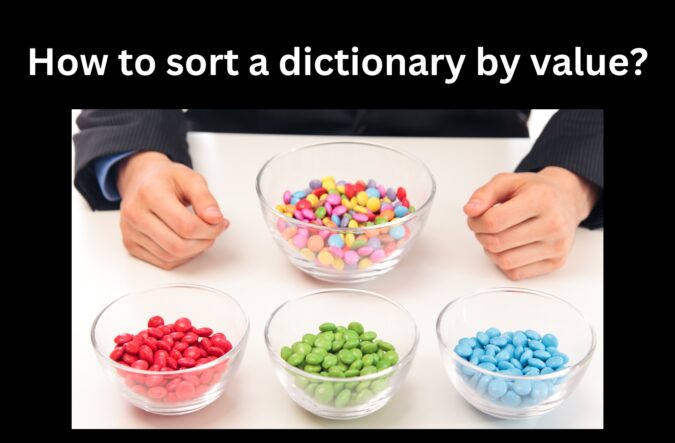
Sorting a dictionary by value is a common task in Python and we will see different ways of how can accomplish this task. Also, sorting a dictionary with respect to keys is simpler than sorting it by values.
Method 1: Sort a dictionary using for loop
This is the most straightforward method to sort a dictionary by its value. In this approach, we first sort the values of the dictionary in ascending order. We then loop through the sorted values and for each sorted value, we loop through the keys of the dictionary when we find a key with a value that matches the current sorted value, we add this key-value pair to the new dictionary that we have created to store the answer. In this way, we keep on adding all the key-value pairs in the newly created dictionary and finally print the sorted dictionary.
dict1 = {"Python":3, "Java": 2, "JavaScript": 1, "C++": 5, "Ruby":4} sorted_values = sorted(dict1.values()) # Sort the values of original dictionary in ascending order sorted_dict = {} # Create a new dictionary to store the final result for i in sorted_values: for k in dict1.keys(): if dict1[k] == i: # if the value is same as the sorted value sorted_dict[k] = dict1[k] # add it to the new dictionary break print(sorted_dict)
Output:
{'JavaScript': 1, 'Java': 2, 'Python': 3, 'Ruby': 4, 'C++': 5}
Method 2: Using bubble sort by converting the dictionary to list
In this approach, we convert the dictionary to a list and apply the bubble sort algorithm to the list to sort the element. But this is not that straightforward as the list contains tuples that store the key-value pairs in it.
Let’s try to print and see what the list looks like when we convert it from the dictionary.
langdict={"Python":3, "Java": 2, "JavaScript": 1, "C++": 5, "Ruby":4} langlist=list(langdict.items()) # convert dictionary to list print(langlist)
Output:
[('Python', 3), ('Java', 2), ('JavaScript', 1), ('C++', 5), ('Ruby', 4)]
As you can see the list contains all the key-value pairs in the form of a tuple. So if we want to perform bubble sort on this, we have to use the key index as 0 and the value index as 1 for each tuple in the list.
Now, Let’s understand the working of the below code to perform bubble sort-
First, we convert the dictionary to a list of tuples called langlist using the list() method. Then we use two nested for loops to iterate through the list and compare each value with every other value in the list. If the value at index “i” is greater than the value at index “j”, we swap the two values. After comparing all the values with each other and placing them in the correct position, we convert the sorted list back into a dictionary using the dict() method and store it in a variable called sortdict, and finally, print it.
langdict={"Python":3, "Java": 2, "JavaScript": 1, "C++": 5, "Ruby":4} langlist=list(langdict.items()) # convert dictionary to list print(langlist) l=len(langlist) for i in range(l-1): # for i in range(4) 0,1,2,3 for j in range(i+1,l): # for j in range(1,5) 1,2,3,4 if langlist[i][1]>langlist[j][1]: # langlist[0][1]>langlist[1][1] 3>2 t=langlist[i] print(t) langlist[i]=langlist[j] langlist[j]=t sortdict=dict(langlist) # convert list to dictionary print(sortdict)
Output:
{'JavaScript': 1, 'Java': 2, 'Python': 3, 'Ruby': 4, 'C++': 5}
Method 3: Using the sorted() function
We can also use the inbuilt sorted() function of Python to sort the dictionary by its value. The sorted() function in Python is used to sort iterable such as a list or dictionary and it returns a sorted list of all the items.
The sorted() function takes three arguments –
- iterable – like a list, tuple, or dictionary
- key – it is an optional value that can be a function that helps in applying custom comparison(we will use this to sort the dictionary by values)
- reverse – It is used to sort the iterable in ascending or descending order. it Is also an optional value and it can be either true or false.
Here, we will use different methods of passing the key argument to the sorted() function to sort a dictionary by value.
3.1 Using the lambda function
The lambda function is a small anonymous function which means it does not have any name. We can pass a lambda function which contains the logic for the comparison of values as the key argument in sorted() function like this
langdict={"Python":3, "Java": 2, "JavaScript": 1, "C++": 5, "Ruby":4} langlist = sorted(langdict.items(), key=lambda x:x[1]) sortdict = dict(langlist) print(sortdict)
Output:
{'JavaScript': 1, 'Java': 2, 'Python': 3, 'Ruby': 4, 'C++': 5}
3.2 Using operator.itemgetter() method
The operator module is a built-in module in Python that provides a set of functions like itemgetter() that can be used to perform different operations. For example, rather than using the “+” operator, we can use the add() function of the operator module to add two numbers.
In this approach, we will use the operator.itemgetter() method to write the comparison logic. Here, itemgetter(1) will get the value of each key-value pair in the dictionary.
import operator langdict={"Python":3, "Java": 2, "JavaScript": 1, "C++": 5, "Ruby":4} langlist= sorted(langdict.items(), key=operator.itemgetter(1)) sortdict=dict(langlist) print(sortdict)
Output:
{'JavaScript': 1, 'Java': 2, 'Python': 3, 'Ruby': 4, 'C++': 5}
3.3 Using list comprehension with sorted() function
We can also use dict.items() method with the sorted() function to sort a dictionary by value.
langdict={"Python":3, "Java": 2, "JavaScript": 1, "C++": 5, "Ruby":4} langlist=sorted((value, key) for (key,value) in langdict.items()) sortdict=dict([(k,v) for v,k in langlist]) print(sortdict)
Output:
{'JavaScript': 1, 'Java': 2, 'Python': 3, 'Ruby': 4, 'C++': 5}
3.4 Using dict.get() method with sorted()
In Python, dict.get() is a method that can be used to get the corresponding values of each key in a dictionary. This function returns the value associated with that key if the key is found in the dictionary otherwise it returns None. We can use dict.get() as the comparison argument in the sorted() function to sort a dictionary by value.
langdict={"Python":3, "Java": 2, "JavaScript": 1, "C++": 5, "Ruby":4} sorted_dict = {} sorted_keys = sorted(langdict, key=langdict.get) # sorted keys for w in sorted_keys: # iterate over the sorted keys sorted_dict[w] = langdict[w] # add the sorted key-value pair to the new dictionary print(sorted_dict)
Output:
{'JavaScript': 1, 'Java': 2, 'Python': 3, 'Ruby': 4, 'C++': 5}
Conclusion
In this article, we explored various approaches like using built-in functions as well as sorting algorithms like bubble sort to sort a dictionary by value. I hope you find this article helpful.
Thank you for visiting our website.
Also read:
- Python | Asking the user for input until they give a valid response
- Python | How to iterate through two lists in parallel?
- Python | How to sort a dictionary by value?
- Python | Remove items from a list while iterating
- Python | How to get dictionary keys as a list
- How to represent Enum in Python?
- 5 methods | Flatten a list of lists | Convert nested list into a single list in Python
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- Create your own ChatGPT with Python
- Filter List in Python | 10 methods
- Radha Krishna using Python Turtle
- Yield Keyword in Python
- Python Programming Examples | Fundamental Programs in Python
- Python | Delete object of a class
- Python | Modify properties of objects
- Python classmethod
- Python | Create a class that takes 2 parameters, print both parameters
- Python | Create a class, create an object of the class, access, and print property value
- Python | Calculator using lambda
- Python | Multiply numbers using lambda
- Python | Print Namaste using lambda
- Iterate over a string in Python
- Python | join() | Join list of strings
- Python | isalnum() method | check if a string consists only of alphabetical characters or numerical digits
- Python | isupper() and islower() methods to check if a string is in all uppercase or lowercase letters
- Python | count substring in a String | count() method
- Python | enumerate() on String | Get index and element
- Python | Convert a String to list using list() method
- Euler’s Number in Python