
Introduction
Most of us have come across the if __name__ == “__main__” in Python while doing a program or we specifically write this function while doing a program. So what does this function basically do?
Here, we are going to see why we are using this if __name__ == “__main__” statement in the programs and how it is used and makes the program meaningful.
What is the __name__ attribute?
The Python interpreter includes the __name__ attribute while we execute the python code as it comes by default. It is a special attribute and it is one of the important names in the given local scope.
Example
Give the command dir() on the python console
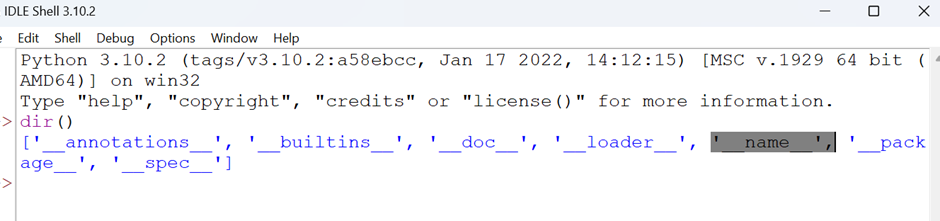
Here you can see the __name__ is being found in the listing of the special attributes in the dir() function that specifies the class name, current module, or the module from where it is invoked.
Understanding __name__ == “__main__”
Let us create two files of python file1module.py and file2module.py
In file1module.py write the following code
print("First file name is {}".format(__name__))
Output:

So what actually happened here is that before python executes the code it sets some special variable and __name__ is one of the special variables when it executes the python file directly it sets __name__ == “__main__” so we are getting the output as the main function.
Consider the next python file file2module.py
import file1module
print("Second file name is {}".format(__name__))
Output:

Now in the second file i.e file2module.py we have actually imported the module file1module hence we are importing the functionalities of that module in our file2module.py. Now you can see the difference between the outputs. The first file is not considered as the __main__ file as it no longer runs directly and it is being imported in the second file as a module here file2module.py is considered as the __main__ module.
Now, write the following code in file1module.py
print("This is statement will be executed always")
def main():
print("First file name is {}".format(__name__))
if __name__== "__main__":
main()
Output:
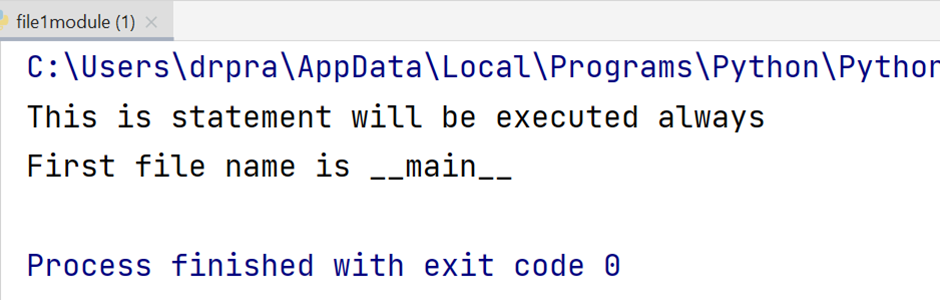
The output here is __main__ which is pretty common as we have applied the if condition allowing it to print the output defined in the main function().
In the file2module.py
import file1module
print("Second file name is {}".format(__name__))
Output:
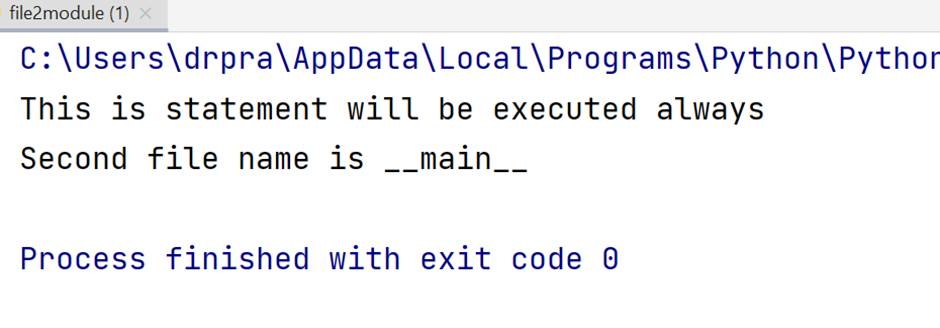
Here we can see the output in the second file is the __main__ as the “file1module” is imported in the file2module.py so it will not consider the file1module as the __main__. Hence it will consider the condition if __name__ == “__main__” for the file2module.py and execute accordingly.
Run a particular function from file1module.py
import file1module
file1module.main()
print("Second file name is {}".format(__name__))
Output:

In some cases, if we want to run a particular function defined in file1module.py we can run it by calling it by filename.name_of_the_function. Hence we can get the output with the defined function from the imported module in the current module.
Conclusion
Hence we have learned if __name__ == “__main__” do in Python that allows executing the code as the script if you run the program directly and not being imported as a module. Hence this is providing different behavior when we are importing it as the module.
Hope you like this piece of information. Please let us know if you want us to include any important information left out to share.
Click here to discuss more on if __name__ == “__main__”
Thank you for reading this article.
Also Read:
- Python | Asking the user for input until they give a valid response
- Python | How to iterate through two lists in parallel?
- Python | How to sort a dictionary by value?
- Python | Remove items from a list while iterating
- Python | How to get dictionary keys as a list
- How to represent Enum in Python?
- 5 methods | Flatten a list of lists | Convert nested list into a single list in Python
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- Create your own ChatGPT with Python
- Filter List in Python | 10 methods
- Radha Krishna using Python Turtle
- Yield Keyword in Python
- Python Programming Examples | Fundamental Programs in Python
- Python | Delete object of a class
- Python | Modify properties of objects
- Python classmethod
- Python | Create a class that takes 2 parameters, print both parameters
- Python | Create a class, create an object of the class, access, and print property value
- Python | Calculator using lambda
- Python | Multiply numbers using lambda
- Python | Print Namaste using lambda
- Iterate over a string in Python
- Python | join() | Join list of strings
- Python | isalnum() method | check if a string consists only of alphabetical characters or numerical digits
- Python | isupper() and islower() methods to check if a string is in all uppercase or lowercase letters
- Python | count substring in a String | count() method
- Python | enumerate() on String | Get index and element
- Python | Convert a String to list using list() method
- Euler’s Number in Python