Today in this article, we will talk about some operations that can be performed on the database using the queries of MongoDB. In this article on CRUD operations in MongoDB with Python, we will learn how to perform the operations like Create, Read, Update, and Delete to manipulate the documents in the database. This time we will perform these operations using Python. Before performing those 4 basic operations, let us look at how to use and connect MongoDB with Python.
Installing pymongo
To access the MongoDB database and perform CRUD operations in MongoDB, Python needs a MongoDB driver. We’ll be using the “PyMongo” MongoDB driver in this article. Tools for dealing with the MongoDB database from Python are included in the PyMongo package. Python.exe must be present in your system’s PATH for the installer to succeed. Define Python in your system environment accordingly. Using the pip tool, you can install MongoDB Connector.
Enter the following command on the command prompt to install PyMongo:
pip install pymongo
Now that we have installed pymongo, the next step is to start our MongoDB Database Server
Starting MongoDB Database Server
This can simply be done by opening the Terminal and by typing “mongo“

Importing pymongo and Establishing Connection
from pymongo import MongoClient
client = MongoClient("mongodb://localhost:27017")
Explanation:
Finally, we are starting with the CRUD operations in MongoDB. First, we need to import our installed library. This can be done by the first line written above. Now to establish the connection with MongoDB, we need to enter the Connection URI. This URI can be found when you open your MongoDB Compass. This URL needs to be passed to the MongoClient.

Connecting to Database and Retrieving Collection
database_name = "Customer"
db = client[database_name]
collection_name = "customer_info"
collection = db[collection_name]
Explanation:
Now that we have made our connection, it is time to make a database. To make a database we first declared a variable and entered the name of our database into it. Then, we passed that to the client variable that stores our connection. Once the database is connected, now we can access the collections in it or make collections. In the same way, we can make or access the collection by passing it to the variable that stores our database info.
NOTE: It must be kept in mind that we cannot see either the database or collection in MongoDB compass until and unless there is a document in it. So whenever we are creating a database or collection, it is necessary to at least insert one document to check whether our database and collection are created or not.
Finally, we will start with CRUD operations in MongoDB with Python.
C – Creating / Inserting documents
There are two methods for adding documents to a collection:
- Adding just one document to the collection.
- Adding a number of documents to the collection
Let us take a look at the below code:
collection.insert_many([{
"contact": 8877665544,
"item_purchased": "motorola",
"name": "sam johnson",
"country": "australia",
"state": "new south wales",
"store": "moto store - australia"
},
{"contact": 1234512345,
"item_purchased": "iphone",
"name": "borris john",
"state": "texas",
"store": "ivenus - texas",
"country": "america"
},
{
"contact": 3245678412,
"item_purchased": "micormax",
"name": "harry berklin",
"country": "australia",
"state": "Queensland",
"store": "micro store - australia"
},
{
"contact": 9898989898,
"item_purchased": "samsung galaxy ",
"name": "malella harison",
"state": "karnataka",
"store": "samsung - india",
"country": "India"
},
])
Explanation:
- insert_one() – This function is used to add a single document to a collection
- insert_many() – This method is used to add numerous documents. In this instance, we’ve added a number of papers to the “customer_info” collection. Here, in the insert_many() function we use commas to divide up numerous items before passing them into the customer_info collection. Multiple items are being handed in when brackets are used between parentheses. A nested method is a common name for such a method.
State of Database before inserting 4 documents
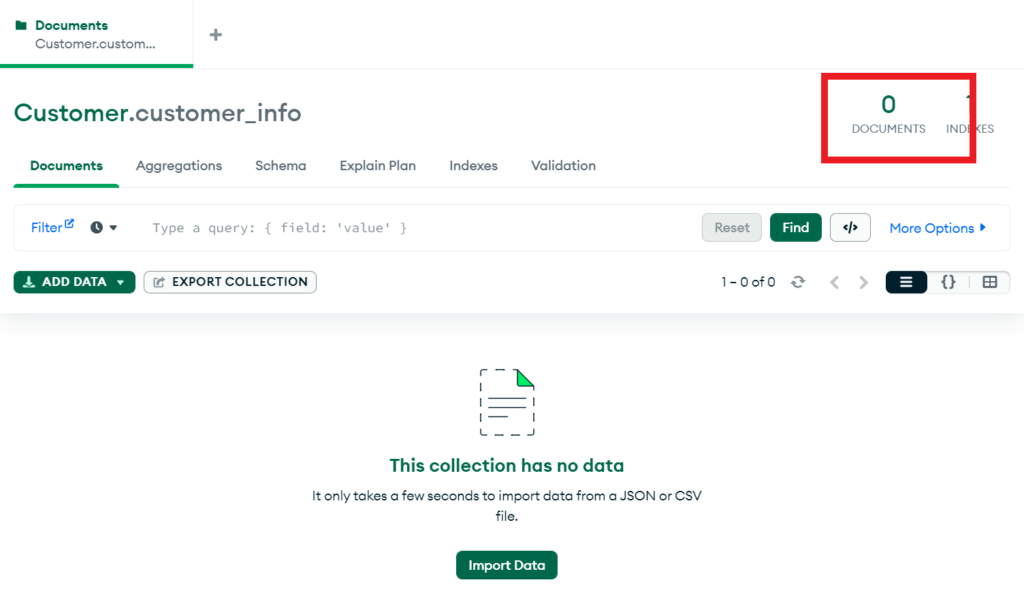
State of Database after inserting 4 documents

R – Reading documents
This mode of operation simply refers to the accessing/reading of the documents that are stored in the database. R stands for read in CRUD operations in MongoDB or any other database.
These two methods can be used to get data.
- Obtaining one document
- Obtaining several papers
Using find_one() to read one document
from pymongo import MongoClient client = MongoClient("mongodb://localhost:27017") database_name = "Customer" db = client[database_name] collection_name = "customer_info" collection = db[collection_name] single_doc = collection.find_one() print(single_doc)
Output:

Explanation:
find_one() method only provides the first document of a collection that meets the specified criteria.
Using find_many() to read one document
multiple_doc = collection.find() for data in multiple_doc: print(data)
Output:
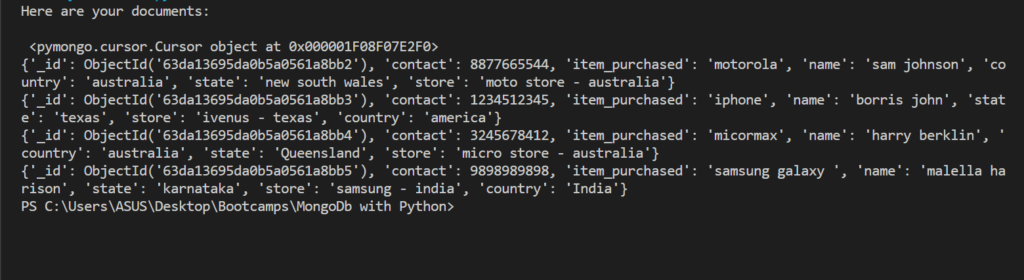
Explanation:
In MongoDB, if we want to access multiple documents then we use the find_many() function which is used to return the document or documents depending on the supplied criteria.
U – Updating documents
The following two methods can be used to update the documents.
- Changing just one document
- Changing several documents
Two arguments must be supplied to the function in order to update the documents. A filter parameter makes up the first parameter, while an update parameter makes up the second. The query that matches the revised document is the filter parameter. The update option specifies the changes that should be made to the document.
Using update_one() to read one document
from pymongo import MongoClient client = MongoClient("mongodb://localhost:27017") database_name = "Customer" db = client[database_name] collection_name = "customer_info" collection = db[collection_name] update_single = collection.update_one({"name": "sam johnson"}, {"$set":{ "contact": 1212}}) print(update_single)
Output before executing update_one() function:

Output after executing update_one() function:
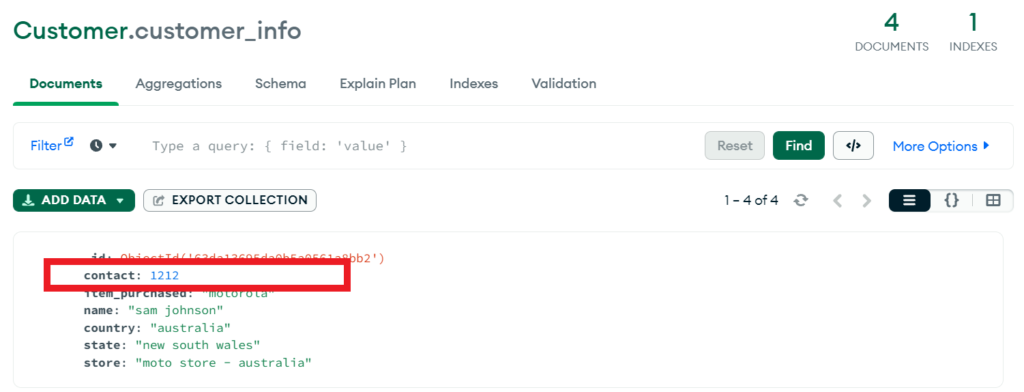
Explanation:
We utilize the update_one() function to update a single document. Two arguments must be supplied to the procedure in order to update the documents. A filter parameter makes up the first parameter, while an update parameter makes up the second. The query that matches the revised document is the filter parameter. The update option specifies the changes that should be made to the document. The value for the fields we want to change will be provided using the “$set” key in the following step. The update will be applied if the specified filter matches the already-existing record.
Using update_many() to read many documents
update_multiple = collection.update_many({"country": "australia"}, {"$set":{ "country": "AUS"}})
print(update_multiple)
Output:

Explanation:
Here in the above query, we used the update_many() function that helps us in updating multiple documents in one go. Here we selected all the documents that have the country Australia and then using the $set, we updated it with the value AUS.
D – Deleting documents
D stands for delete in CRUD operations in MongoDB or any other database. Moving on to the last operation in our article on CRUD operations in Python MongoDB. Deleting simply refers to removing the documents from the MongoDB database. The following two methods can be used to delete documents.
- Removing a single document
- Eliminating several documents
Removing a single document using the delete_one() function
from pymongo import MongoClient client = MongoClient("mongodb://localhost:27017") database_name = "Customer" db = client[database_name] collection_name = "customer_info" collection = db[collection_name] delete_single = collection.delete_one({ "contact": 1212}) print(delete_single)
Output before deleting a single document

Output before deleting multiple documents
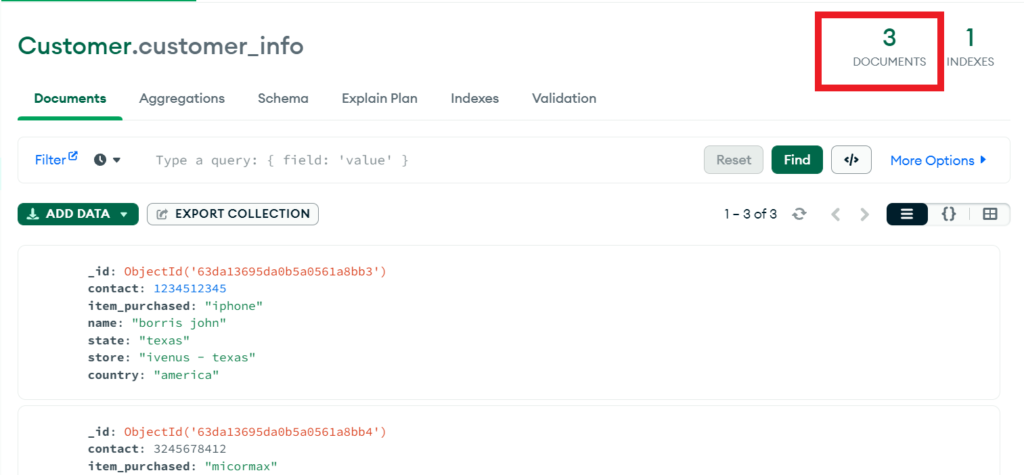
Explanation:
Like the update and create operations, the delete operation only affects a single collection. Additionally, deletions of a single document are atomic. The documents you wish to delete from a collection can be defined using filters and criteria when performing delete actions. The criteria and filters function similarly to the filter options in Python’s read-only CRUD operations in MongoDB. One document can be eliminated from a MongoDB collection using the delete_one() function. Filters identify the document that will be removed. Only records that match the chosen filter are eliminated.
Removing multiple documents using the delete_many() function
delete_multiple = collection.update_many({"country": "australia"}, {"$set":{ "country": "AUS"}})
print(delete_single)
Output:

Explanation:
This function is used to delete multiple documents, based on the condition passed in the function, the value is matched and corresponding documents are deleted. We must always be careful whenever using the delete_many() function, as multiple documents get deleted. Here 2 documents got deleted as after the update operation we had two docs that had “AUS” as the country name.
Dropping a collection
To drop an entire collection in one go, we can simply use the drop() function. Executing this will delete all the documents that are stored in that particular document and the user will not be able to retrieve those documents.
collection.drop()
Required Files
Conclusion
Here is the end of our article on CRUD operations in MongoDB with Python, we learned about some functions that we can use to perform CRUD operations. I tried my best to explain the connection process the easiest way possible. We hope this article turns out to be a great source of learning for you. We will soon be back with another article till then keep Learning, Execting, and Building.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter