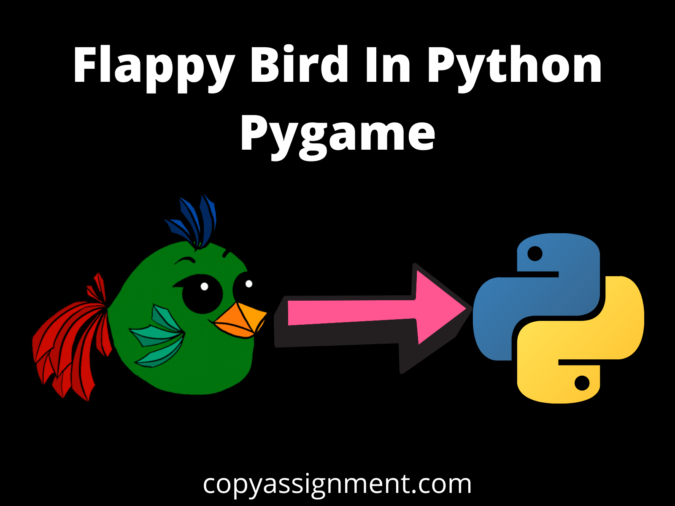
OVERVIEW OF THE PROJECT
Hi there, welcome to the copyassignment hope you all are doing wonderful. Today, we are here to make a very interesting game Flappy Bird In Python Pygame. Hope you are familiar with this entertaining and very fascinating game.
We would see how we can code the game Flappy bird from Scratch. My aim is to provide you with the knowledge of game making with lots of logic building which can help you in your future projects.
We are creating this game with the help of the most popular and easy-to-understand programming language ‘PYTHON’ also this is my personal favorite programming language.
We will use Pygame which is a set of modules for creating our game Flappy bird. Hope you all are excited so much because I can feel your vibe from here.
So, don’t worry about any hard technical terms we’ll guide you from scratch to make this game and I’ll try my best to clear all the concepts which we will be using to make this.
Before going further let’s have a look at the game we are going to make today just be with me and will make this happen. Just let go of all the thoughts from your mind and be patient and just be with us.
LINK FOR FLAPPY BIRD SOURCE CODE SPRITES AND MUSIC
PREREQUISITE /PREFACE OF THE GAME
Before starting to code let me tell you the requirements and also let me explain some terms that we are going to use in this project because we are here for beginners also.
These are just some basics you can also ignore this section if you are familiar with it already. In this project, you just need basic knowledge of python and some Logic and you are good to go.
So, without any further delay let’s start our journey. But before that make sure that you have:-
- Python is installed on your computer.
- A Code Editor because it makes your work easy. I use Visual Studio Code you can use Pycharm or any editor you want. It doesn’t matter.
- Pygame library is installed because if not then open the command prompt or terminal on your computer and then type one of the following commands in the terminal:
1.”pip install pygame”
2.”py -m pip install -U pygame –user”
3.”py -m pip install pygame”
Now, we have installed Pygame on our computer. Let’s quickly understand What the Pygame is and what does it do?
STARTING WITH THE Flappy Bird In Python Pygame
Pygame is a set of modules or we can say a library by Python which we use for making games.
There are 4 things important in the Pygame: –
- Game Loop
- Events
- Sprites
- Sounds
Game loop is an infinite loop in which we blit our Sprites and play Sounds by handling or accessing Events from the user.
Blitting of the Sprites frequently in a loop gives the illusion to the user that they are playing the game.
However it’s just the game of removing images and blitting new ones, but it happens so fast that’s why our eyes can’t detect it and because of that our sensory nerves make it a game.
After having all this now we can start to code but before that, I also said that I’ll introduce some basic terms that we are going to use in our Game. So, have a look at these terms:-
Function | Description |
init() | Initializes all of the imported Pygame modules (returns a tuple indicating success and failure of initializations) |
set_mode() | Takes a tuple or a list as its parameter to create a surface (tuple preferred) |
update() | Updates the screen |
quit() | Used to uninitialized everything |
set_caption() | Will set the caption text on the top of the display screen |
event.get() | Returns list of all events |
time.Clock() | Helps track time |
font.SysFont() | Will create a Pygame font from the System font resources |
mouse.get_pos() | Returns the coordinates of the Mouse cursor |
get_height() | Gives the height of the object |
get_width() | Gives the Width of the Object |
SOME BASIC FUNCTIONS AND THEIR USES
Now, we are ready. So let’s start this beautiful journey and I said earlier be with us and you will end up having a great project.
CREATING GAME WINDOW & ADDING SPRITES AND SOUNDS
We will make a folder for ex- ‘flappy bird’ in this case in which we will keep all our sprites and sounds to make it easy. Don’t worry I’ll provide you with that.
Now, we will create our game window for that first we’ll have to import Pygame and other modules too which we are going to use in this game.
So for importing Pygame we’ll just write ‘import pygame’ and it will import the pygame for us. Let’s look at the code below for a better understanding of Flappy Bird In Python Pygame.
We’ll create a file ‘main.py’ in which we are going to write all of our codes you can name anything you want but there shouldn’t be any module’s name otherwise it may throw an error.
Our files will look something like this.

import random #for generating random numbers import sys #To Exit the game import pygame from pygame.locals import * #Basic Pygame Imports
Now, run the code and check whether our modules are imported. If they are imported, there won’t be any error, but if it throws an error, you have to check the code again whether you have misspelled or pygame isn’t installed successfully.
CREATING GLOBAL VARIABLES FOR FLAPPY BIRD IN PYTHON PYGAME
After importing we’ll create some ‘Global Variables’ which we will use throughout the game. So let’s create some ‘Global Variables’. Our game window will also be a Global variable. So, look at the code below.
import random #for generating random numbers import sys #To Exit the game import pygame from pygame.locals import * #Basic Pygame Imports #Global Variables for The Game FPS = 32 SCREENWIDTH = 289 SCREENHEIGHT = 511 SCREEN = pygame.display.set_mode((SCREENWIDTH, SCREENHEIGHT))
First, we stated the ‘FPS’ which is nothing but the ‘Frame Per Second’ we gave it a value of 32 which means we’ll play our games in 32 ‘Frame Per Second’ in simple words 32 images will blit on the screen in just One Second.
Then, we declare the ‘SCREENWIDTH’ and ‘SCREENHEIGHT’ and give it values of 289 and 511 which is ‘LENGTH & BREADTH’ of our game window. And after that by using the code ‘pygame.display.set_mode()’ we made our game window.
if you are having problem in understanding ‘pygame.display.set_mode()’. Then you can check out our other posts ‘CAR RACE GAME IN PYGAME FROM SCRATCH‘. There we have discussed in detail the working of these codes.
Now, we’ll do our next task which is declaring some variables and will make some dictionaries and will load our sprites as well.
So look at the code below.
import random #for generating random numbers import sys #To Exit the game import pygame from pygame.locals import * #Basic Pygame Imports #GLOBAL VARIABLES FPS = 32 SCREENWIDTH = 289 SCREENHEIGHT = 511 SCREEN = pygame.display.set_mode((SCREENWIDTH, SCREENHEIGHT)) GROUNDY = SCREENHEIGHT*0.8 GAME_SPRITES = {} GAME_SOUNDS = {} PLAYER = 'resources\SPRITES\\bird.png' BACKGROUND = 'resources\SPRITES\\bg.jpeg' PIPE = 'resources\SPRITES\pipe.png '
First of all, we have declared the variable ‘GROUNDY’ which is for our ‘base image’.

After then we created the dictionary ‘GAME_SPRITES’ and ‘GAME_SOUNDS’ after then we gave paths to our bird, background, pipes images.
LOADING ALL THE SPRITES AND SOUNDS
Now, we will load all the SPRITES & SOUNDS for our game. So, let’s look that what we have in our SPRITES & SOUNDS.


So we have seen our all sprites we have numbers from 0 to 9 which we’ll use for blitting our Score. We have a base image which we can also say is the ground of our game, and we have a background image which is the background of our game.
After then we have a bird image which is our player and then a game over which we’ll display after game over and then home and then a message which will be our Welcome Screen after then we have pipes
because it would be obstacles through which our bird has to be flapped and then retry which we will use to play the game again.
So, now it’s time to load all the SPRITES & SOUNDS. Let’s have a look at the code.
import random #for generating random numbers import sys #To Exit the game import pygame from pygame.locals import * #Basic Pygame Imports FPS = 32 SCREENWIDTH = 289 SCREENHEIGHT = 511 SCREEN = pygame.display.set_mode((SCREENWIDTH, SCREENHEIGHT)) GROUNDY = SCREENHEIGHT*0.8 GAME_SPRITES = {} GAME_SOUNDS = {} PLAYER = 'resources\SPRITES\\bird.png' BACKGROUND = 'resources\SPRITES\\bg.jpeg' PIPE = 'resources\SPRITES\pipe.png ' ### This is the point from Where Our Game is going to be Started ### if __name__ == "__main__": pygame.init() #Initializing the Modules of Pygame FPSCLOCK = pygame.time.Clock() #for controlling the FPS pygame.display.set_caption('Flappy Bird With Sameer') #Setting the Caption of The Game #### LOADING THE SPRITES #### GAME_SPRITES['numbers'] = ( pygame.image.load('resources\SPRITES\\0.png').convert_alpha(), pygame.image.load('resources\SPRITES\\1.png').convert_alpha(), pygame.image.load('resources\SPRITES\\2.png').convert_alpha(), pygame.image.load('resources\SPRITES\\3.png').convert_alpha(), pygame.image.load('resources\SPRITES\\4.png').convert_alpha(), pygame.image.load('resources\SPRITES\\5.png').convert_alpha(), pygame.image.load('resources\SPRITES\\6.png').convert_alpha(), pygame.image.load('resources\SPRITES\\7.png').convert_alpha(), pygame.image.load('resources\SPRITES\\8.png').convert_alpha(), pygame.image.load('resources\SPRITES\\9.png').convert_alpha(), ) S GAME_SPRITES['background'] = pygame.image.load(BACKGROUND).convert_alpha() GAME_SPRITES['player'] = pygame.image.load(PLAYER).convert_alpha() GAME_SPRITES['message'] = pygame.image.load('resources\SPRITES\message.png').convert_alpha() GAME_SPRITES['base'] = pygame.image.load('resources\SPRITES\\base.png').convert_alpha() GAME_SPRITES['pipe'] = ( pygame.transform.rotate(pygame.image.load(PIPE).convert_alpha(), 180), #### UPPER PIPES, WE JUST ROTATED THE PIPE BY 180deg pygame.image.load(PIPE).convert_alpha() #### LOWER PIPES ) #Game Sounds GAME_SOUNDS['die'] = pygame.mixer.Sound('resources\AUDIO\die.wav') GAME_SOUNDS['hit'] = pygame.mixer.Sound('resources\AUDIO\hit.wav') GAME_SOUNDS['point'] = pygame.mixer.Sound('resources\AUDIO\point.wav') GAME_SOUNDS['swoosh'] = pygame.mixer.Sound('resources\AUDIO\swoosh.wav') GAME_SOUNDS['wing'] = pygame.mixer.Sound('resources\AUDIO\wing.wav')
I don’t think I need to explain anything in the above code because there is nothing to explain here however I have commented out in the code as well so you can understand it from there.
After then we’ll create a while loop inside this we’ll call our welcomeScreen function and mainGame function. Don’t worry we’ll create them we haven’t created them yet.
while True:
welcomeScreen() #Shows a welcomescreen to the user until they starts the game
mainGame() #This is our main game funtion