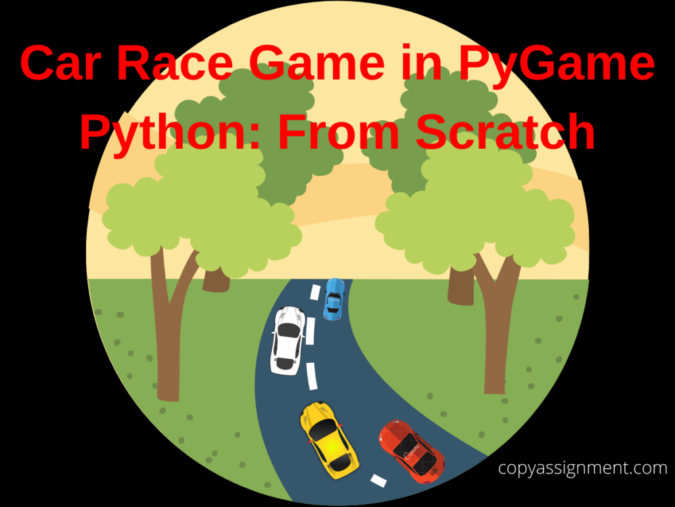
Check the video below to know about our Car Race Game in PyGame and Python we are going to make. If you don’t know Pygame, then you should not worry, we will guide you from scratch. You just need some basic Python skills, other than Python, we will guide you with everything.
Introduction to Car Race Game in PyGame
Welcome to the copyassignment, a place to learn to code easily and quickly.
In this tutorial, we are going to make a very interesting game with lots of logic that will help you to understand Python more easily.
We’ll be using one of the most popular Python libraries for making this Car Race Game.
I’ll try my best to clear all the concepts which we are going to use to make this game. Stick to the end and I’m sure you will end up with a great project.
The focus of this tutorial is to make you understand the working of Pygame and Python so that you may not only make this Car Race Game in PyGame but can also create your own with your own idea.
So, without any further delay let’s start our journey. But before that make sure that you have:-
- Python installed on your computer.
- A Code Editor because it makes your work easy. I use Visual Studio Code you can use Pycharm or any editor you want. It doesn’t matter.
- Pygame library is installed because if not then open command prompt or terminal on your computer and then type one of the following commands in terminal:
1.”pip install pygame”
2.”py -m pip install -U pygame –user”
3.”py -m pip install pygame”
After installing Pygame, you can start to develop the game.
For downloading the images and music click on the link given below.
RACING BEAST SPRITES AND MUSIC
Make a folder and put all the stuff like images, sounds, etc, which we’ll require in our game in that folder. Don’t worry I’ll provide you with all the stuff which I used in this game. Open the folder in VS Code or Pycharm or any code editor you are using. Look at the image below our all pieces of stuff are located in a single folder.

I am using VS Code so, in VS Code, you will get this type of interface and then make a file with .py extension for example – main.py where we’ll write our code.
But before starting coding I want to introduce some functions that we’ll be using in our game and these functions are also being used in almost all Pygame projects.
Function | Description |
init() | Initializes all of the imported Pygame modules (returns a tuple indicating success and failure of initializations) |
display.set_mode() | Takes a tuple or a list as its parameter to create a surface (tuple preferred) |
update() | Updates the screen |
quit() | Used to uninitialized everything |
set_caption() | Will set the caption text on the top of the display screen |
event.get() | Returns list of all events |
Surface.fill() | Will fill the surface with a solid color |
time.Clock() | Helps track time |
font.SysFont() | Will create a Pygame font from the System font resources |
Creating the first game window in PyGame
After having all the stuff, now we are ready to make our game window. Obviously, the first thing we need to do is to import the Pygame.
import pygame
This code will import the Pygame library which we’ll be using for the game. After importing the Pygame, now we need to initialize the Pygame so as to use all of the cool methods and all of the stuff that is inside the pygame.
This code will import the Pygame library which we’ll be using for the game. After importing the Pygame, now we need to initialize the Pygame so as to use all of the cool methods and all of the other stuff that is inside the Pygame. To initialize the Pygame type the following code.
pygame.init() #initializes the Pygame
from pygame.locals import* #import all modules from Pygame
This will initialize and import all modules from Pygame. This line is very important so whenever from now if you are going to create a game with Pygame. Make sure you have added this line otherwise Pygame code is not going to work. Thus, if you want that your Pygame should work effectively without giving any error so you should never forget these lines.
Now, after initializing the Pygame, let’s move out to make our Game window where we can play our game. Pygame makes it really, really very easy. It’s just one line of code. For creating the Game window just type the following line after the previous code.
screen = pygame.display.set_mode((798,600))
What we have done is we created a screen variable in which we accessed the pygame’s display.set_mode() function. That’s why we typed pygame.
Let me explain this.
Pygame is a set of modules and from that module, the display is also a module, and from the display module set_mode() is a function that takes a tuple as a parameter in this case, (798,600) is a tuple which is nothing but the length and breadth of the window respectively in pixels. In other words, Pygame is a parent of display and display is a child and display is a parent of set_mode() and set_mode() is a child of display (I just used an analogy to make you understand). So to access the child we used DOT(.).


Now, we have created the Game window but when we run our code we’ll see that the window comes and disappears within a second.
To solve this problem we have to make an infinite game loop that will display our game window until we close it. For this, type the following lines of code.
while True :
pass

Now, run the code you will see that now our game window isn’t disappearing within a second. But wait, there another problem arose that our system got hanged. You can’t even close the game after pressing the cross button which is on the right corner of the game window.
Don’t worry it’s just because of the infinite loop just open the task manager and kill the Pygame Window task. Now everything is fine, but even if you rerun the code without changing it, you have to face the same problem again.
If you are following this tutorial carefully you might have one question arising in your mind that why we couldn’t close our game window just by clicking on the cross button?
If this question arose in your mind then it means you are giving your full attention because without questioning you can’t learn anything. So, the answer to this question is very simple that we haven’t created any quit function yet in our code. So for that, we are going to be using something known as an event.
We’ll get into events more a little bit later but for right now just understand first What is an event in PyGame? and the answer is event is anything that is happening inside our game window like moving the cursor, pressing any key of the keyboard, etc.
Even pressing the close button is also an event in Pygame. So, this pressing of the close button is known as quit event in Pygame. So, what we are going to do now is that we are making sure that when we pressing the close button we exit out of the loop so that our game window can close.
For that, we are going to create a variable let’s say run and give it a boolean value True and inside the while loop instead of typing while True now we can type while Run, and whenever we want to break outside of this infinite loop we are just gonna turn the value of run into False and it will automatically break this while loop.
run = True
while run:
I think that you are familiar with the while loop if you don’t know what while true do basically is, it runs infinitely and when this value of true turns into False it comes out of the while loop. I won’t go much more into it because it’s just the basics of Python programming.
Now, after writing the while run what we need to do is to go through all the events that are happening inside our game window. So for that, we are going to create a for loop and create an event variable and then we are going to loop through all of the events that are happing inside the for loop. Look at the following code for this.
run = True
while run:
for event in pygame.event.get():
This makes sure that all of the events that are happening get into this pygame.event.get() then we can loop through all of the events and check one by one if the close button has been pressed so that we can change the run loop into the False to get out of the loop. Our code will look like this.
run = True
while run:
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
Inside our for loop we checked that if the close button has been pressed or not. If yes, we change the value of run to False to break the loop. If not then we are continuing with our infinite while run loop.
We’ll go to more events later but for now, just understand what we have done yet. Now run the following code to check if everything is working or not.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False
We have successfully created our game window for our Car Race Game in PyGame without any error and also solve some basic doubts and understood the uses of all code that we have used yet. Now, I am summarising what we have done till yet for your convenience.
We imported and initialized the Pygame and then created a screen variable in which we give the value for our game window. After then we made the game loop so that our game window couldn’t disappear quickly. After then we accessed all the events that are happening inside our game window in a while loop. After then we check all the events one by one and checked whether a cross button or close button has been pressed if yes we get out of the loop.
Changing the PyGame window Title
In the previous section, we have created our game window, but it was not personalized because you will notice that it says Pygame window and a peculiar logo. So, in this section, we are going to learn how to change the Title logo and background color of our game window.
Changing the title in Pygame is very easy, you just need to type the following lines of code.
#changing title of the game window
pygame.display.set_caption('Racing Beast')

What the above code will do is it will change the title of our game window from ‘Pygame window‘ to ‘Racing Beast‘ obviously you can use your desired name but I used Racing Beast. Since we are dealing with our display that’s why we used ‘pygame.display‘ and ‘set_caption‘ is a method to change the caption or the title of our window.
Changing the PyGame window Logo
Now we will change that weird logo to something related to the car. As I have said in the starting to collect all the stuff related to the game in one folder so it would be easy for you.
For changing the logo we will first create a variable logo in which we will load our image and then with the help of the ‘set_icon‘ method we’ll change the logo. So our code will look like this
#changing the logo
logo = pygame.image.load('car game\logo.jpeg')
pygame.display.set_icon(logo)
What we did is we created a variable logo in which we loaded the image that we are using for the icon for our game through the ‘pygame.image.load()’ method it is used to load image from file or file-like object. Then with the help of the ‘pygame.display.set_icon()’ function, we wrote a variable logo in which our image is loaded. Now run the code and check if everything is okay. See the image below.

NOTE – Sometimes, pygame can give you an error saying that could not open the image.
It is because the path that we have given for our image in this case (‘car game\logo.jpeg‘) is a path so try to change the path so the error would be fixed. But in VS Code it is easier, see the video below to learn how to give the accurate path. Simply go to the image and right-click on that image and select copy relative path from the drop-down and paste it into the parenthesis () with inverted commas this pygame.image.load(‘car game\logo.jpeg‘)
Changing the PyGame window Background
Now, we have changed the logo of our game now it’s time to change our background colour. The default colour of our game window is ‘black‘ we’ll also using the black colour because we have a background image but for your knowledge, I’ll tell you how to change the background colour so that if you want to use this in another game.
For changing the background color of our game window we use ‘surface.fill( () )’. It takes a tuple value as a parameter in the form of RGB values. Notice here surface means the variable which we have used for creating our game window in this we have used ‘screen’ (see in creating our first game window) variable for creating our game window. So, instead of the surface.fill use screen.fill. So look at the code below.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False if event.type == pygame.KEYDOWN: if event.key == K_RIGHT: maincarX += 5 if event.key == K_LEFT: maincarX -= 5 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((255,0,0))
Notice we have written the code inside our while loop because the while loop is our game loop and it will continue until we finish or quit our game, otherwise it will come once and then disappear. Now run the code and check.
If you have run the code you will notice that there is nothing happened to our game window.

It happened because we didn’t update our display. In Pygame, it is very essential to update the display; otherwise, our code wouldn’t show the appropriate result. So every time you display any image, sound, etc make sure to update the display. For this just type ‘pygame.display.update()‘. Now run the following code and check if everything is okay.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False if event.type == pygame.KEYDOWN: if event.key == K_RIGHT: maincarX += 5 if event.key == K_LEFT: maincarX -= 5 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((255,0,0)) pygame.display.update()
Now you can see below that our screen has been changed.

I can write it directly but I didn’t because I wanted to show the importance of this ‘pygame.display.update()‘ line. Because if you learn from your mistake then that mistake remains always remembered. To understand this is a very important line. So never miss it.
Till now we have learned how to create the game window and how to customize it like changing title, logo, and background color. I hope I was able to explain the terms clearly.
Now, we’ll be adding player images, enemy images and also give our background an image. We’ll change the background color to black from red it was only for showing how this works.
Adding images to PyGame
Till now we have customized our game window now it’s time to add images or sprites to our game. Pygame makes it very easy we’ll load our image as previously we have done it with ‘pygame.image.load()‘.
After loading the sprites or images we have to display them on our game window for this we’ll use the ‘blit function’ – this is used to display images on our window.
But you might have one question in your mind that where will my image will display on our game window. So it’s our choice where we want our images to be displayed. We’ll display images with their x and y coordinates.
If you have studied ‘coordinate geometry‘ in your school then you must be familiar with the x and y coordinates. If you don’t know I’ll give a short idea about it.
Every point has some X and Y coordinates which we represent with the number and the origin is (0,0). Here first 0 is the value of X coordinate and the second 0 is the value of Y coordinate. If you look at our code by which we created our game window which is
screen = pygame.display.set_mode((798,600))
In this, we have passed the screen variable a value of (798,600) which is nothing but X and Y coordinates from (0,0) to (798,600). It’s mean the starting point of our game window is (0,0) and the last point is (798,600). Look at the picture for a better understanding.

Notice there are four values:-
- At the top left corner, the value of (X, Y) is (0, 0) which is our window’s starting point.
- At the top right corner, the value of (X, Y) is (798, 0).
- At the bottom left corner, the value of (X, Y) is (0, 600).
- At the bottom right corner, the value of (X, Y) is (798, 600).
We are observing that in the 1st and 2nd cases, the value of X is increasing but the value of Y is not increasing while in the 3rd and 4th cases, both X and Y are increasing.
How these values are changing :-
You will notice that when we go from left to right our X increases and when we go from up to bottom our Y increases.
So, we conclude that our X increases when we go from left to right and decreases from when we go from right to left.
our Y increases when we go from up to bottom and decreases from when we go from bottom to up.
X and Y can also be negative. See the image below for better understanding.

So, let’s use this knowledge in our game. I hope you understood this clearly. Now let’s code to give our background an image.
#setting background image
bg = pygame.image.load('car game/bg.png')
run = True
while run:
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
#CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE
screen.fill((0,0,0))
#displaying the background image
screen.blit(bg,(0,0))
pygame.display.update()
What we did above is we created a variable ‘bg‘ and loaded our background image in that variable.
I have said earlier that whatever we want to use till the end of the game we have to do it in a while loop that’s why we used the ‘screen.blit‘ method. In the while loop, ‘blit‘ is used to display the image and ‘screen‘ our pygame surface or window.
‘screen.blit()‘ takes two things as a parameter which comes inside the parenthesis. First is the object we want to display and second (x, y) coordinates means where we want to display our image in the game window.
So here we have ‘screen.blit(bg,(0,0))‘ bg which is our background image, and (0, 0) is a place where we want to place our image. Why we used (0, 0) as coordinates because our background image is of (798,600) pixels which will cover our whole game window.
You can change the value and check what happens after that. I left it as a task for you to understand it better.
Now run the following code and check everything is working or not.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #setting background image bg = pygame.image.load('car game/bg.png') run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) pygame.display.update()
If nothing wrong with the code then our output will look something like this. Here is our output.

We have successfully changed the background image. Now we’ll display our main car which is player and enemies’ cars but before that, we’ll define a function ‘gameloop()‘ what we have coded yet from changing the background will put in it and call our gameloop function. I’ll tell you why we did that because it will be difficult to understand it now. So look at the change in our code after defining the gameloop function.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) pygame.display.update() gameloop()
Don’t worry there will not be change in the output of the game.
Now, it’s time to display our main car which is our player. So, let’s do it. First, we have to load our image and then blit it in while loop. Run and check it working or not.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) pygame.display.update() gameloop()
What we just did is we loaded our image and make two variables ‘maincarX‘ and ‘maincarY‘ passed them some values which will our player’s x and y and then displayed our player image in the while loop. These x and y values came from the trial and error method. Just apply some logic and you’ll get the desired result.
In this case, we want to display our main car at the bottom and half of the width of the screen. Thus our X should be lower than the width of the screen and Y should be lower than 600. Check by changing these values until you get the desired result.
Later, we used ‘screen.blit(maincar,(maincarX,maincarY))‘ in while loop to display our image in which the first parameter is maincar which is our image, and the second is its axes. If everything is correct you will get output something like this.

Using this logic now we’ll display other cars. I am not going to explain it because everything will be the same. Later, we’ll change something in it but for now, I’m just displaying it.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 #other cars car1 = pygame.image.load('car game\car1.jpeg') car2 = pygame.image.load('car game\car2.png') car3 = pygame.image.load('car game\car3.png') run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) #displaing other cars screen.blit(car1,(200,100)) screen.blit(car2,(300,100)) screen.blit(car3,(400,100)) pygame.display.update() gameloop()
Here is the output of the above code. Just run and check it yourself.

Now we have displayed our all cars successfully but it is only an image we can’t do anything with it now. So, in the next section, we will move our car by pressing the keyboard keys.
Moving an object in Python
Now, we are going to see how to move our main car left, right, up, and down. For making our car move we need to change the axis of the car. See the image below to understand the mechanics behind it.

Our image is at 350X if we add 5X to it our new maincarX will be 355X and if we subtract 5X to our maincarX then our maincarX will be 345X, and the same happens with Y-axis.
Now, we’ll try to do something in our code that when we press the right arrow key our car will move to the right when we press the left arrow key the car will move to the left when we press the up arrow key the car will move to up and when we press down arrow key the car will move to the down.
As I have said earlier that everything we do in our game is an event. Therefore pressing the keys is also and lifting up the keys is also an event.
So first, we have to access the keydown event and then we will check if arrow keys are pressed or not? If it has been pressed we would give them some statement. Thus look at the code then I’ll explain what we did.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 maincarX_change = 0 maincarY_change = 0 #other cars car1 = pygame.image.load('car game\car1.jpeg') car2 = pygame.image.load('car game\car2.png') car3 = pygame.image.load('car game\car3.png') run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False if event.type == pygame.KEYDOWN: if event.key == pygame.K_RIGHT: maincarX_change += 5 if event.key == pygame.K_LEFT: maincarX_change -= 5 if event.key == pygame.K_UP: maincarY_change -= 5 if event.key == pygame.K_DOWN: maincarY_change += 5 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) #displaing other cars screen.blit(car1,(200,100)) screen.blit(car2,(300,100)) screen.blit(car3,(400,100)) #updating the values maincarX += maincarX_change maincarY +=maincarY_change pygame.display.update() gameloop()
Change in the code-:
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
maincarX_change += 5
if event.key == pygame.K_LEFT:
maincarX_change -= 5
if event.key == pygame.K_UP:
maincarY_change -= 5
if event.key == pygame.K_DOWN:
maincarY_change += 5
#updating the values
maincarX += maincarX_change
maincarY +=maincarY_change
We first checked that if any key has been pressed by ‘if event.type == pygame.keydown’ and then we checked that if ‘right, left, up and down arrow keys’ has been pressed. If yes, we gave them some commands for left and right keys and some commands for up and down keys.
Left & Right keys will change X-axis and UP & Down keys will change Y-axis. After then we updated the value of the maincarX and maincarY in the while loop. Run the above code and check it. Below is the output of the above code.
But still, there is some problem with our car that first, it hasn’t any boundary means our car can go beyond our game window and second it is not in our control means when we press any of the arrow keys our car keep moving even after lifting up the key.
We’ll add boundaries to our game in the next section. So let’s solve another problem first. To solve that problem we have to ask one question that why is our car moving even after lifting up the key? If you remember that I have said anything which happens inside our game window is an event so lifting up the key is also an event.
Thus, we have passed the commands for pressing the key but we haven’t passed any commands for lifting up the key. So, lifting up the key isn’t doing anything. For this, we also have to check that if we have lifted up the key and give the command for the arrow keys also. Look at the code below.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 maincarX_change = 0 maincarY_change = 0 #other cars car1 = pygame.image.load('car game\car1.jpeg') car2 = pygame.image.load('car game\car2.png') car3 = pygame.image.load('car game\car3.png') run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #checking if any key has been pressed if event.type == pygame.KEYDOWN: if event.key == pygame.K_RIGHT: maincarX_change += 5 if event.key == pygame.K_LEFT: maincarX_change -= 5 if event.key == pygame.K_UP: maincarY_change -= 5 if event.key == pygame.K_DOWN: maincarY_change += 5 #checking if key has been lifted up if event.type == pygame.KEYUP: if event.key == pygame.K_RIGHT: maincarX_change = 0 if event.key == pygame.K_LEFT: maincarX_change = 0 if event.key == pygame.K_UP: maincarY_change = 0 if event.key == pygame.K_DOWN: maincarY_change = 0 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) #displaing other cars screen.blit(car1,(200,100)) screen.blit(car2,(300,100)) screen.blit(car3,(400,100)) #updating the values maincarX += maincarX_change maincarY +=maincarY_change pygame.display.update() gameloop()
Change in the code:-
#checking if key has been lifted up
if event.type == pygame.KEYUP:
if event.key == pygame.K_RIGHT:
maincarX_change = 0
if event.key == pygame.K_LEFT:
maincarX_change = 0
if event.key == pygame.K_UP:
maincarY_change = 0
if event.key == pygame.K_DOWN:
maincarY_change = 0
Run the code and check everything is working or not. Below is the output below of the above code.
What we did is we first checked if any key has been lifted up or not if yes then we checked if it is any of the arrow keys if yes we changed maincarX_change and maincarY_change to 0. So that our car stops when we lift up the key.
Till now we have learned lots of things along with the movement mechanics behind the game. Now, we’ll add a boundary to our game and will also display our enemies randomly in our game.
Adding boundaries PyGame:
In this section, we’ll give our car a boundary or a playing area so that it can move only in that area and can’t go beyond the game window. Also, we’ll be displaying our enemies’ cars randomly in the playing area. So that it can give you the feel of a real game. So, let’s do that.
First, we have to find the value of (X, Y) which we’ll be using as a border. In this case, the value of Y at the top and bottom of the game window is 0,600 respectively. We got Y, now we have to find the X. Find X by trial and error or you can try two things. First, place your car at the desired place and print the value of ‘maincarX’. Second, you can change the value of ‘maincarX’ and check where it is displaying now. Try changing the value till you get the desired result. So that value of X will be your X to use as a boundary. Now, look at the code.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 maincarX_change = 0 maincarY_change = 0 #other cars car1 = pygame.image.load('car game\car1.jpeg') car2 = pygame.image.load('car game\car2.png') car3 = pygame.image.load('car game\car3.png') run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #checking if any key has been pressed if event.type == pygame.KEYDOWN: if event.key == pygame.K_RIGHT: maincarX_change += 5 if event.key == pygame.K_LEFT: maincarX_change -= 5 if event.key == pygame.K_UP: maincarY_change -= 5 if event.key == pygame.K_DOWN: maincarY_change += 5 #checking if key has been lifted up if event.type == pygame.KEYUP: if event.key == pygame.K_RIGHT: maincarX_change = 0 if event.key == pygame.K_LEFT: maincarX_change = 0 if event.key == pygame.K_UP: maincarY_change = 0 if event.key == pygame.K_DOWN: maincarY_change = 0 #setting boundary for our main car if maincarX < 178: maincarX = 178 if maincarX > 490: maincarX = 490 if maincarY < 0: maincarY = 0 if maincarY > 495: maincarY = 495 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) #displaing other cars screen.blit(car1,(200,100)) screen.blit(car2,(300,100)) screen.blit(car3,(400,100)) #updating the values maincarX += maincarX_change maincarY +=maincarY_change pygame.display.update() gameloop()
Change in the Code :-
#setting boundary for our main car
if maincarX < 178:
maincarX = 178
if maincarX > 490:
maincarX = 490
if maincarY < 0:
maincarY = 0
if maincarY > 495:
maincarY = 495
What we did is we first find the appropriate values of (X, Y), and then we checked that if our ‘maincarX and maincarY‘ passes that value then we changed our maincarX and maincarY to the same value again. Run & check is it working or not? Below is the output of the above code.
Now, we will display enemy cars randomly. We have got the X-axis of the playing area from the above code, so we’ll use that X value to display enemy cars randomly in the playing area.
For displaying enemy cars randomly, we’ll need a random module. So we’ll first import the random module. This is very simple just write ‘import random‘ and it will import the random module.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame import random Now, we'll change all enemy cars X to some random value between 178 to 490 because it is the length of our playing area. Look at the code. import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame import random screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 maincarX_change = 0 maincarY_change = 0 #other cars car1 = pygame.image.load('car game\car1.jpeg') car1X = random.randint(178,490) car1Y = 100 car2 = pygame.image.load('car game\car2.png') car2X = random.randint(178,490) car2Y = 100 car3 = pygame.image.load('car game\car3.png') car3X = random.randint(178,490) car3Y = 100 run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #checking if any key has been pressed if event.type == pygame.KEYDOWN: if event.key == pygame.K_RIGHT: maincarX_change += 5 if event.key == pygame.K_LEFT: maincarX_change -= 5 if event.key == pygame.K_UP: maincarY_change -= 5 if event.key == pygame.K_DOWN: maincarY_change += 5 #checking if key has been lifted up if event.type == pygame.KEYUP: if event.key == pygame.K_RIGHT: maincarX_change = 0 if event.key == pygame.K_LEFT: maincarX_change = 0 if event.key == pygame.K_UP: maincarY_change = 0 if event.key == pygame.K_DOWN: maincarY_change = 0 #setting boundary for our main car if maincarX < 178: maincarX = 178 if maincarX > 490: maincarX = 490 if maincarY < 0: maincarY = 0 if maincarY > 495: maincarY = 495 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) #displaing other cars screen.blit(car1,(car1X,car1Y)) screen.blit(car2,(car2X,car2Y)) screen.blit(car3,(car3X,car3Y)) #updating the values maincarX += maincarX_change maincarY +=maincarY_change pygame.display.update() gameloop()
Change in the Code :-
import random
car1X = random.randint(178,490)
car1Y = 100
car2X = random.randint(178,490)
car2Y = 100
car3X = random.randint(178,490)
car3Y = 100
#displaing other cars
screen.blit(car1,(car1X,car1Y))
screen.blit(car2,(car2X,car2Y))
screen.blit(car3,(car3X,car3Y))
Here, random.randint(178,490) will generate a random number every time which will be our enemy car’s X. So in this way these cars’ X will change every time randomly. Hence, we have successfully displayed other cars randomly. Run the check it. In the next section, we will be focusing on the movement mechanics of the enemy.
Movement mechanics of the Enemy:
Now we will see the movement mechanics of the enemy. The logic behind this is the same as we have seen previously. The only difference here is we moved our car with our choice but in this case, the enemies will be coming infinitely and randomly. So let’s code.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame import random screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 maincarX_change = 0 maincarY_change = 0 #other cars car1 = pygame.image.load('car game\car1.jpeg') car1X = random.randint(178,490) car1Y = 100 car2 = pygame.image.load('car game\car2.png') car2X = random.randint(178,490) car2Y = 100 car3 = pygame.image.load('car game\car3.png') car3X = random.randint(178,490) car3Y = 100 run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #checking if any key has been pressed if event.type == pygame.KEYDOWN: if event.key == pygame.K_RIGHT: maincarX_change += 5 if event.key == pygame.K_LEFT: maincarX_change -= 5 if event.key == pygame.K_UP: maincarY_change -= 5 if event.key == pygame.K_DOWN: maincarY_change += 5 #checking if key has been lifted up if event.type == pygame.KEYUP: if event.key == pygame.K_RIGHT: maincarX_change = 0 if event.key == pygame.K_LEFT: maincarX_change = 0 if event.key == pygame.K_UP: maincarY_change = 0 if event.key == pygame.K_DOWN: maincarY_change = 0 #setting boundary for our main car if maincarX < 178: maincarX = 178 if maincarX > 490: maincarX = 490 if maincarY < 0: maincarY = 0 if maincarY > 495: maincarY = 495 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) #displaing other cars screen.blit(car1,(car1X,car1Y)) screen.blit(car2,(car2X,car2Y)) screen.blit(car3,(car3X,car3Y)) #updating the values maincarX += maincarX_change maincarY +=maincarY_change #movement of the enemies car1Y += 10 car2Y += 10 car3Y += 10 pygame.display.update() gameloop()
Change in the Code :-
#movement of the enemies
car1Y += 10
car2Y += 10
car3Y += 10
Notice, we are changing only the Y-axis of the enemies because we didn’t need to change its X-axis. There is also a bug in our code that is coming only one time. It is because we have only stated that to change the Y-axis and since it is in while loop, it is changing its axis even it crosses the game window.
Look at the output of the above code. I have slowed down the speed of enemies for you for a clear view.
To solve this problem we have to add a boundary for our enemies and display it again. I think you will understand better the code. So let’s code to move the enemies in a never-ending loop.
import pygame pygame.init() #initializes the Pygame from pygame.locals import* #import all modules from Pygame import random screen = pygame.display.set_mode((798,600)) #changing title of the game window pygame.display.set_caption('Racing Beast') #changing the logo logo = pygame.image.load('car game/logo.jpeg') pygame.display.set_icon(logo) #defining our gameloop function def gameloop(): #setting background image bg = pygame.image.load('car game/bg.png') # setting our player maincar = pygame.image.load('car game\car.png') maincarX = 350 maincarY = 495 maincarX_change = 0 maincarY_change = 0 #other cars car1 = pygame.image.load('car game\car1.jpeg') car1X = random.randint(178,490) car1Y = 100 car2 = pygame.image.load('car game\car2.png') car2X = random.randint(178,490) car2Y = 100 car3 = pygame.image.load('car game\car3.png') car3X = random.randint(178,490) car3Y = 100 run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False #checking if any key has been pressed if event.type == pygame.KEYDOWN: if event.key == pygame.K_RIGHT: maincarX_change += 5 if event.key == pygame.K_LEFT: maincarX_change -= 5 if event.key == pygame.K_UP: maincarY_change -= 5 if event.key == pygame.K_DOWN: maincarY_change += 5 #checking if key has been lifted up if event.type == pygame.KEYUP: if event.key == pygame.K_RIGHT: maincarX_change = 0 if event.key == pygame.K_LEFT: maincarX_change = 0 if event.key == pygame.K_UP: maincarY_change = 0 if event.key == pygame.K_DOWN: maincarY_change = 0 #setting boundary for our main car if maincarX < 178: maincarX = 178 if maincarX > 490: maincarX = 490 if maincarY < 0: maincarY = 0 if maincarY > 495: maincarY = 495 #CHANGING COLOR WITH RGB VALUE, RGB = RED, GREEN, BLUE screen.fill((0,0,0)) #displaying the background image screen.blit(bg,(0,0)) #displaying our main car screen.blit(maincar,(maincarX,maincarY)) #displaing other cars screen.blit(car1,(car1X,car1Y)) screen.blit(car2,(car2X,car2Y)) screen.blit(car3,(car3X,car3Y)) #updating the values maincarX += maincarX_change maincarY +=maincarY_change #movement of the enemies car1Y += 10 car2Y += 10 car3Y += 10 #moving enemies infinitely if car1Y > 670: car1Y = -100 if car2Y > 670: car2Y = -150 if car3Y > 670: car3Y = -200 pygame.display.update() gameloop()
Change in the code:-
#moving enemies infinitely
if car1Y > 670:
car1Y = -100
if car2Y > 670:
car2Y = -150
if car3Y > 670:
car3Y = -200
It was very simply what we did is we said that if enemies car which is our car1,car2, and car3. If their Y-axis becomes greater than 670 then change their Y-axis to -100, -150, and 200 respectively.
We gave 670 because our game window is 600 axes long so there should be greater value so that it seems that our car has been passed of the window and after that, we displayed these cars to -100, -150, and -200 to make us feel that another car is coming and we gave 50-pixel difference to use it as an offset. This is all about the trial and error method just try and give values that suit you.
Now everything should be fine. Look the output of above code.
This was a long journey I know this, but do you know? This was just the first part, we will come soon with 2nd part where we will complete this tutorial.
For downloading the images and music click on the link given below.
RACING BEAST SPRITES AND MUSIC
I tried my best with my all effort to explain the game development in python I tried to keep it at a very basic level so that even beginners can understand it. I hope you will all like my effort. However if you any problem or queries in any place to understanding it you can comment down or you can also send a message to me on Instagram. My Instagram id is @expert_in_python
Now, it’s time to show your creativity. Customize this game and add some new features to it and send it to me on Instagram @expert_in_python and @pythonhub. We would appreciate your effort. Till then keep learning, keep rocking and keep vising copyassignment.
Thank you.
Also Read:
- Stickman Game in PythonToday, we will make Stickman Game in Python. Stickman is a video game in which the player controls a stickman character and the stickman must be navigated to the exit. The character needs to jump to get on the platform and try not to fall down in order to get to the exit door and…
- Brick Breaker Game in PythonIntroduction In this article, we will develop a Brick Breaker game in Python. We will use Pygame to develop this Brick Breaker game. Pygame is a set of Python modules used for writing video games. It includes computer graphics and sound libraries designed to be used with Python to develop games. Let’s get started! Code…
- Tank game in PythonYou must be familiar with tank game, this is a video game where a player plays with the computer. We can design our own Tank game in Python. We will use the most famous gaming library in Python i.e. PyGame to develop our Tank game. Click here to copy the complete source code for the…
- GUI Piano in PythonHello folks! Today we are back with one another article on GUI Piano in Python. We all have played with piano once in our life. Now today is the time to build one on ourselves. This piano project will have all the functionalities that our physical piano used to have. For the development of Piano…
- Ludo Game in PythonToday we are back with one another fantastic article on game development articles in Python. In this article, we will talk about and learn about Ludo Game in Python. We will provide you with the complete source code along with a brief explanation of each function, method, or class that we use to develop this…
- Rock Paper Scissors Game in PythonHello folks! Today, we are back with a fantastic tutorial on the Rock Paper Scissors Game in Python. We all are familiar with the topic that we have chosen for today’s tutorial. We all played this game with our friends during our childhood time. Basically, we used to use our hand gestures in order to…
- Snake and Ladder Game in PythonIntroduction Are you looking for a fun way to learn Python coding? If so, why not create your own Snake and Ladder Game in Python? This step-by-step tutorial will show you how to craft a fully-functional game of Snake and Ladder in just a few simple steps. You’ll learn basic Python programming concepts, the fundamentals…
- Puzzle Game in PythonWho has not played a single puzzle game in their childhood? Probably everyone played once at least. Today, in this article, we will build a Puzzle Game in Python using the pygame library. Imagine a puzzle game at your fingertips that you can play anytime, anywhere. There are various puzzle games and today we will…
- Creating Dino Game in PythonWho has not played Google’s famous Dino Game whenever we are not connected to the internet? Probably everyone might have played this game once. Today, in this article, we will help you develop a Dino Game in Python. This tutorial will include an in-depth explanation of each line of code along with reference materials. We…
- Tic Tac Toe Game in PythonWho has not played this amazing game in our childhood days? Probably each and every person might have played this game once in their school days or later. Today, in this article on the Tic Tac Toe Game in Python, we will learn how to build this amazing game along with a detailed explanation of…
- Tetris game in Python CodeIn this article, we will build an amazing project Tetris game in Python code. In this project, we will use the pygame library to build the game. To create this project, ensure you have the latest version of Python installed in your system. Let’s get started! Pygame Pygame is a cross-platform set of Python modules…
- Drawing Application in Python TkinterIntroduction In this article, we will design and construct a basic Drawing Application in Python Tkinter GUI, where we can simply draw something on the canvas using a pencil and erase it with an eraser, as well as the ability to change the thickness of a pencil and eraser. We may also modify the canvas’s…
- Python Games Code | Copy and PasteHello friends, today, we will see all the Python games code which you can easily copy and paste into your system. We will see many Python games code and most of them are available on our website and others will be on other websites but don’t worry about that, we will provide links to all…
- Games in Python | Assignment Expert
- Space Invaders game using PythonWe have already developed multiple games using the turtle library and now continuing our series of projects development in Python using the turtle library, today, in this article, we are going to develop a Space Invaders game using Python.As we all know the number of various built-in functions that a turtle library offers is more…