
Hi everyone! In this post, we are going to discuss a very famous DSA problem known as the Maximum Product Subarray. It is a leetcode medium-level problem. We’ll discuss the problem statement, solution approach, and different methods to solve the problem. In the end, we will see the complete C++ code and Java code for the maximum product subarray.
Table of Contents
- Maximum product subarray problem statement
- Problem Explanation
- Maximum product subarray leetcode solution approach
- Brute Force Solution of Maximum Product Subarray
- Pseudo code of O ( N3 ) Approach
- Pseudo code of O (N2) Approach
- C++ Solution of Maximum product subarray
- Java Solution of Maximum product subarray
Maximum Product Subarray Problem Statement
Given an integer array arr
, find a non-empty subarray that has the largest product, and return that product. The test cases are generated so that the answer will fit in a 32-bit integer.
Note: A subarray is a contiguous subsequence of the array.
Input
The first line tells the number of test cases. Each test case consists of 2 lines. In the first line of the test case, we have N the number of elements present in the array. In the next line, we have N elements separated by spaces.
Output
Print an integer that tells the maximum product possible by multiplying two or more contiguous elements.
Example
Input: arr = [2,3,-2,4] Output: 6 Explanation: [2,3] has the largest product 6. Input: arr = [-2,0,-1] Output: 0 Explanation: The result cannot be 2, because [-2,-1] is not a subarray.
Maximum Product Subarray Problem Explanation
The problem is very simple and straightforward. You have to find the maximum product which is possible in the given array by multiplying one or more contiguous elements. The array can have positive integers, negative integers, and zero also. Note this thing that an array can have negative elements and any number of zeroes also. That basically means if you’re considering any sub-array that has one or more zero, its product would be 0 always.
Brute Force Solution of Maximum Product Subarray
The brute force solution is very simple. We can first find out all the sub-arrays of an array and then multiply all elements of the individual subarray and then take the maximum of those. The key idea is to traverse over every possible sub-array, find the product of each of these sub-arrays and return the maximum product from all these results.
If an array has 3 elements then the count of possible non-empty sub-arrays would be 6. An example is given below. Refer to the given image. To find out all the sub-arrays we will need 2 loops and then to multiply all elements we will again traverse all the elements of the subarray. That means O(N3) time complexity. It’s a very naive approach.
Pseudo code of O(N3) Approach
int solve(vector<int> &arr)
{
int ans = INT_MIN;
int n = arr.size();
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
{
int temp = 1;
for(int k = i; k <= j; k++)
temp = temp * arr[k];
ans = max(ans, temp);
}
}
return ans;
}
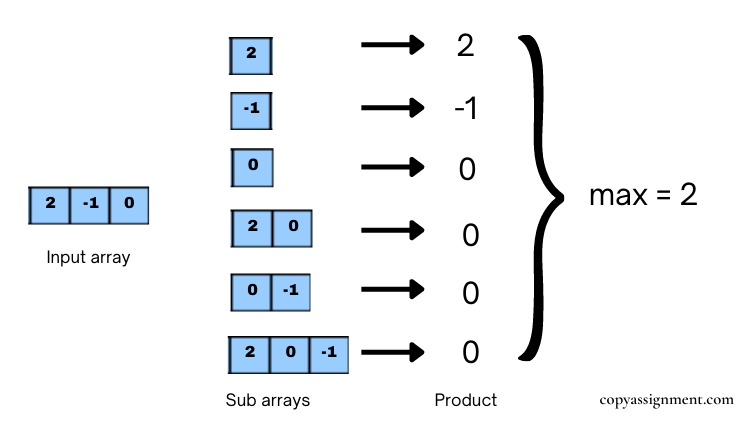
We can reduce this O(N3) time complexity to O(N2) easily. We just need to maintain the multiplication of elements while considering the sub-array elements.
int solve(vector<int> &arr)
{
int ans = INT_MIN;
int n = arr.size();
for(int i = 0; i < n; i++)
{
int temp = 1;
for(int j = 0; j < n; j++)
{
temp = temp * arr[j];
ans = max(ans, temp);
}
}
return ans;
}
Maximum Product Subarray O(N) Solution Approach
We can further optimize this to O(N). Now the question arises how? The optimized approach is not very intuitive but it’s really important to learn in order to tackle future problems. So, in the optimized approach, we traverse the array 2 times. One time from left to right (in forwarding direction) and the second time from right to left (in backward direction). Now while traversing we maintain maximum till now and current multiplication. Do not forget to handle the case when the current product becomes zero. You have to reset it to 1. Refer to the below pseudo code.
- Declare ans = INT_MIN and curr_prod = 1.
- Start loop from i = 0 to n-1 where n is the length of the array.
- Update curr_prod as curr_prod = curr_prod * arr[i].
- Update ans as ans = max(ans, curr_prod).
- Now check if curr_prod is 0, and reset it to 1.
- End loop.
- Update curr_prod as curr_prod = 1.
- Run loop from i = n -1 to 0.
- Update curr_prod as curr_prod = curr_prod * arr[i].
- Update ans as ans = max(ans, curr_prod).
- Now check if curr_prod is 0, and reset it to 1.
- End loop.
- Return ans.
Note: The claim is maximum product will lie from index 0 to k OR from index k to n-1.
The whole solution is based on the above claim. It’s an observation. You can verify this using some examples on your own. The time complexity of this solution would be O(N) since we’re just using 2 loops to traverse the given array in both directions. The space complexity would be O(1) since we are just using some variables and not using any auxiliary space.
C++ Solution of Maximum product subarray
int solve(vector<int>& arr)
{
int n = arr.size();
if(n == 1)
return arr[0];
long long ans = INT_MIN;
long long curr_prod = 1;
for(int i = 0; i < n; i++)
{
curr_prod = curr_prod * arr[i];
ans = max(curr_prod, ans);
if(curr_prod == 0)
curr_prod = 1;
}
curr_prod = 1;
for(int i = n-1; i >= 0; i--)
{
curr_prod = curr_prod * arr[i];
ans = max(curr_prod, ans);
if(curr_prod == 0)
curr_prod = 1;
}
return ans;
}
Time Complexity: O ( 2 * N ) => O ( N )
Space Complexity: O ( 1 )
Java Solution of Maximum product subarray
int solve(int[] nums)
{
int ans = Integer.MIN_VALUE, curr_prod = 1;
for(int i = 0; i < nums.length; i++) {
curr_prod = curr_prod * nums[i];
ans = Math.max(ans, curr_prod);
if(curr_prod == 0)
curr_prod = 1;
}
curr_prod = 1;
for(int i = nums.length-1; i>=0; i--)
{
curr_prod = curr_prod * nums[i];
ans = Math.max(ans, curr_prod);
if(curr_prod == 0)
curr_prod = 1;
}
return ans;
}
Time Complexity: O ( 2 * N ) => O ( N )
Space Complexity: O ( 1 )
If you’re preparing for product-based companies, you should definitely check out these Top 20 Array Coding Questions for Online Assessment.
If you want us to add more coding questions kindly tell us in the comments. Don’t forget to share it with your friends. Thanks 🙂
Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- HackerRank Day 8 Solution in Python: Dictionaries and Maps
- HackerRank Day 7 Solution in Python: Arrays
- HackerRank Day 6 Solution in Python: Let’s review
- HackerRank Day 5 Solution in Python: Loops
- HackerRank Day 4 Solution in Python: Class vs Instance
- HackerRank Day 3 Solution in Python: Intro to Conditional Statements
- HackerRank Day 2 Solution in Python: Operators
- HackerRank Day 1 Solution in Python: Data Types
- HackerRank Day 0 Solution in Python: Hello World
- HackerRank Day 29 Solution in Python: Bitwise AND
- HackerRank Day 28 Solution in Python: RegEx, Patterns, and Intro to databases
- HackerRank Day 27 Solution in Python: Testing
- HackerRank Day 26 Solution in Python: Nested Logic
- HackerRank Day 25 Solution in Python: Running Time and Complexity
- HackerRank Day 24 Solution in Python: More Linked Lists
- HackerRank Day 23 Solution in Python: BST Level Order Traversal
- HackerRank Day 22 Solution in Python: Binary Search Trees
- Find Peak Element LeetCode 162
- HackerRank Day 20 Solution in Python: Sorting
- HackerRank Day 19 Solution in Python: Interfaces
- HackerRank Day 18 Solution in Python: Queues and Stacks
- HackerRank Day 17 Solution in Python: More Exceptions
- HackerRank Day 16 Solution: Exceptions – String to Integer
- Explained: Allocate minimum number of pages
- HackerRank Day 15 Solution in Python: Linked List
- Search a 2D matrix: leetcode 74
- Maximum Subarray Sum: Kadane’s Algorithm
- HackerRank Day 13 Solution in Python: Abstract Classes
- HackerRank Day 14 Solution in Python: Scope