Problem Statement:
House Stark of Winterfell is a Great House of Westeros and the royal house of the Kingdom of the North. Every once a year, the king offers 5 people from his kingdom a visit to this house. But there are certain conditions,
1. these 5 people should not be below or equal to 50. The group will be rejected if this condition is not satisfied for even a single person.
2. Each of the people is allowed to visit only one floor. The floor is decided on the basis of their age.
The remainder left after dividing the age by 5 will be the floor a person can visit.
3. If two or more person allotted the same floor, then only one person can get that floor, the rest will be allocated vacant floors.
Remember the indexing starts from 0.
Given the ages of 5 people, determine which floor each individual can visit.
Example:
Input1:
Enter the ages of 5 people:
54 57 53 59 59
Output1:
Age: 59, Floor: 0
Age: 59, Floor: 1
Age: 57, Floor: 2
Age: 53, Floor: 3
Age: 54, Floor: 4
Input2:
Enter the ages of 5 people:
51 52 53 50 54
Output2:
This group is rejected.
Code for Starks Adventure in Python:
'''determine floors''' def floor(ages): n = len(ages) left = list() floors = [-1]*n for i in range(n): num = ages[i]%5 if(floors[num]==-1): floors[num] = ages[i] else: left.append(ages[i]) k=0 for i in range(n): if(floors[i]==-1): floors[i]=left[k] k+=1 return floors '''check if the group is valid''' def isValid(ages): flag = 0 for each in ages: if(each<=50): flag=1 break if(flag==1): return 0 else: return 1 '''input''' print("Enter the ages of 5 people: ") ages = list(map(int,input().split())) '''output''' if(isValid(ages)): floors = floor(ages) for i in range(5): print(f"Age: {floors[i]}, Floor: {i}") else: print("This group is rejected.")
Input and Output 1:
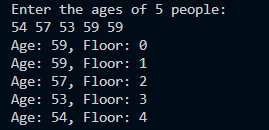
Input and Output 2:

Also Read:
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Shift Numbers in Python | Assignment Expert
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python