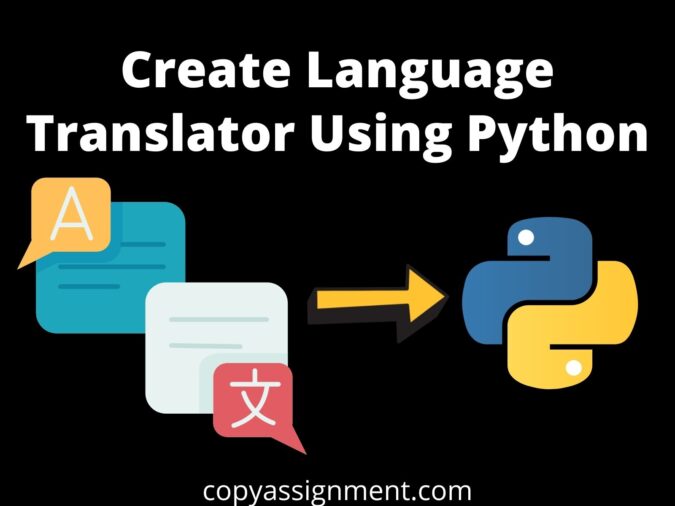
Today, we will see how we can Create Language Translator Using Python.
First, let’s have a look at the video to understand what we are going to do to Create Language Translator Using Python.
Install googletrans
To create a text translator using python, we need one external module i.e googletrans, to install the module, type the following command in your terminal–
pip install googletrans
About googletrans
Googletrans is a free and unlimited python library that implemented Google Translate API. This uses the Google Translate Ajax API to make calls to such methods as detecting and translate.
Code
Now, let’s understand the code using comments.
from googletrans import Translator # taking some text from our website in french language text = '''Python est un langage de programmation interprété et de haut niveau qui a été créé à la fin des années 1980, mais il a été implémenté en décembre 1989 par Guido Van Rossum . Le mot Python vient du serpent. Selon la récente enquête de StackOverflow , Python a dépassé la popularité de Java''' # Creating an instance of Translator to use # Google Translate ajax API translator = Translator() # detect- auto detects language of the input text dt = translator.detect(text) print(dt) # translate()-translates the text from source language to destination language # translate(self, text, dest='en', src='auto', **kwargs) # if we don't specify the dest(destination language) then # it translates to english translated = translator.translate(text) print(translated.text)
Output
Detected(lang=fr, confidence=0.95565623) Python is an interpreted and high level that was created in the late 1980s, but it was implemented in December 1989 by Guido Van Rossum. The word Python comes from the serpent. According to recent StackOverflow survey, Python has overtaken the popularity of Java
To get the available languages, run the following code:
Example
import googletrans print(googletrans.LANGUAGES)
Output
{'af': 'afrikaans', 'sq': 'albanian', 'am': 'amharic', 'ar': 'arabic', 'hy': 'armenian', 'az': 'azerbaijani', 'eu': 'basque', 'be': 'belarusian', 'bn': 'bengali', 'bs': 'bosnian', 'bg': 'bulgarian', 'ca': 'catalan', 'ceb': 'cebuano', 'ny': 'chichewa', 'zh-cn': 'chinese (simplified)', 'zh-tw': 'chinese (traditional)', 'co': 'corsican', 'hr': 'croatian', 'cs': 'czech', 'da': 'danish', 'nl': 'dutch', 'en': 'english', 'eo': 'esperanto', 'et': 'estonian', 'tl': 'filipino', 'fi': 'finnish', 'fr': 'french', 'fy': 'frisian', 'gl': 'galician', 'ka': 'georgian', 'de': 'german', 'el': 'greek', 'gu': 'gujarati', 'ht': 'haitian creole', 'ha': 'hausa', 'haw': 'hawaiian', 'iw': 'hebrew', 'he': 'hebrew', 'hi': 'hindi', 'hmn': 'hmong', 'hu': 'hungarian', 'is': 'icelandic', 'ig': 'igbo', 'id': 'indonesian', 'ga': 'irish', 'it': 'italian', 'ja': 'japanese', 'jw': 'javanese', 'kn': 'kannada', 'kk': 'kazakh', 'km': 'khmer', 'ko': 'korean', 'ku': 'kurdish (kurmanji)', 'ky': 'kyrgyz', 'lo': 'lao', 'la': 'latin', 'lv': 'latvian', 'lt': 'lithuanian', 'lb': 'luxembourgish', 'mk': 'macedonian', 'mg': 'malagasy', 'ms': 'malay', 'ml': 'malayalam', 'mt': 'maltese', 'mi': 'maori', 'mr': 'marathi', 'mn': 'mongolian', 'my': 'myanmar (burmese)', 'ne': 'nepali', 'no': 'norwegian', 'or': 'odia', 'ps': 'pashto', 'fa': 'persian', 'pl': 'polish', 'pt': 'portuguese', 'pa': 'punjabi', 'ro': 'romanian', 'ru': 'russian', 'sm': 'samoan', 'gd': 'scots gaelic', 'sr': 'serbian', 'st': 'sesotho', 'sn': 'shona', 'sd': 'sindhi', 'si': 'sinhala', 'sk': 'slovak', 'sl': 'slovenian', 'so': 'somali', 'es': 'spanish', 'su': 'sundanese', 'sw': 'swahili', 'sv': 'swedish', 'tg': 'tajik', 'ta': 'tamil', 'te': 'telugu', 'th': 'thai', 'tr': 'turkish', 'uk': 'ukrainian', 'ur': 'urdu', 'ug': 'uyghur', 'uz': 'uzbek', 'vi': 'vietnamese', 'cy': 'welsh', 'xh': 'xhosa', 'yi': 'yiddish', 'yo': 'yoruba', 'zu': 'zulu'}
The maximum character limit on a single text is 15k.
Available languages are:
‘af’: ‘afrikaans’,
‘sq’: ‘albanian’,
‘am’: ‘amharic’,
‘ar’: ‘arabic’,
‘hy’: ‘armenian’,
‘az’: ‘azerbaijani’,
‘eu’: ‘basque’,
‘be’: ‘belarusian’,
‘bn’: ‘bengali’,
‘bs’: ‘bosnian’,
‘bg’: ‘bulgarian’,
‘ca’: ‘catalan’,
‘ceb’: ‘cebuano’,
‘ny’: ‘chichewa’,
‘zh-cn’: ‘chinese (simplified)’,
‘zh-tw’: ‘chinese (traditional)’,
‘co’: ‘corsican’,
‘hr’: ‘croatian’,
‘cs’: ‘czech’,
‘da’: ‘danish’,
‘nl’: ‘dutch’,
‘en’: ‘english’,
‘eo’: ‘esperanto’,
‘et’: ‘estonian’,
‘tl’: ‘filipino’,
‘fi’: ‘finnish’,
‘fr’: ‘french’,
‘fy’: ‘frisian’,
‘gl’: ‘galician’,
‘ka’: ‘georgian’,
‘de’: ‘german’,
‘el’: ‘greek’,
‘gu’: ‘gujarati’,
‘ht’: ‘haitian creole’,
‘ha’: ‘hausa’,
‘haw’: ‘hawaiian’,
‘iw’: ‘hebrew’,
‘he’: ‘hebrew’,
‘hi’: ‘hindi’,
‘hmn’: ‘hmong’,
‘hu’: ‘hungarian’,
‘is’: ‘icelandic’,
‘ig’: ‘igbo’,
‘id’: ‘indonesian’,
‘ga’: ‘irish’,
‘it’: ‘italian’,
‘ja’: ‘japanese’,
‘jw’: ‘javanese’,
‘kn’: ‘kannada’,
‘kk’: ‘kazakh’,
‘km’: ‘khmer’,
‘ko’: ‘korean’,
‘ku’: ‘kurdish (kurmanji)’,
‘ky’: ‘kyrgyz’,
‘lo’: ‘lao’,
‘la’: ‘latin’,
‘lv’: ‘latvian’,
‘lt’: ‘lithuanian’,
‘lb’: ‘luxembourgish’,
‘mk’: ‘macedonian’,
‘mg’: ‘malagasy’,
‘ms’: ‘malay’,
‘ml’: ‘malayalam’,
‘mt’: ‘maltese’,
‘mi’: ‘maori’,
‘mr’: ‘marathi’,
‘mn’: ‘mongolian’,
‘my’: ‘myanmar (burmese)’,
‘ne’: ‘nepali’,
‘no’: ‘norwegian’,
‘or’: ‘odia’,
‘ps’: ‘pashto’,
‘fa’: ‘persian’,
‘pl’: ‘polish’,
‘pt’: ‘portuguese’,
‘pa’: ‘punjabi’,
‘ro’: ‘romanian’,
‘ru’: ‘russian’,
‘sm’: ‘samoan’,
‘gd’: ‘scots gaelic’,
‘sr’: ‘serbian’,
‘st’: ‘sesotho’,
‘sn’: ‘shona’,
‘sd’: ‘sindhi’,
‘si’: ‘sinhala’,
‘sk’: ‘slovak’,
‘sl’: ‘slovenian’,
‘so’: ‘somali’,
‘es’: ‘spanish’,
‘su’: ‘sundanese’,
‘sw’: ‘swahili’,
‘sv’: ‘swedish’,
‘tg’: ‘tajik’,
‘ta’: ‘tamil’,
‘te’: ‘telugu’,
‘th’: ‘thai’,
‘tr’: ‘turkish’,
‘uk’: ‘ukrainian’,
‘ur’: ‘urdu’,
‘ug’: ‘uyghur’,
‘uz’: ‘uzbek’,
‘vi’: ‘vietnamese’,
‘cy’: ‘welsh’,
‘xh’: ‘xhosa’,
‘yi’: ‘yiddish’,
‘yo’: ‘yoruba’,
‘zu’: ‘zulu’,
Also Read:
YouTube Video Downloader Application Using Python
GUI Application To See wifi password in Python
Crack Any Password Using Python