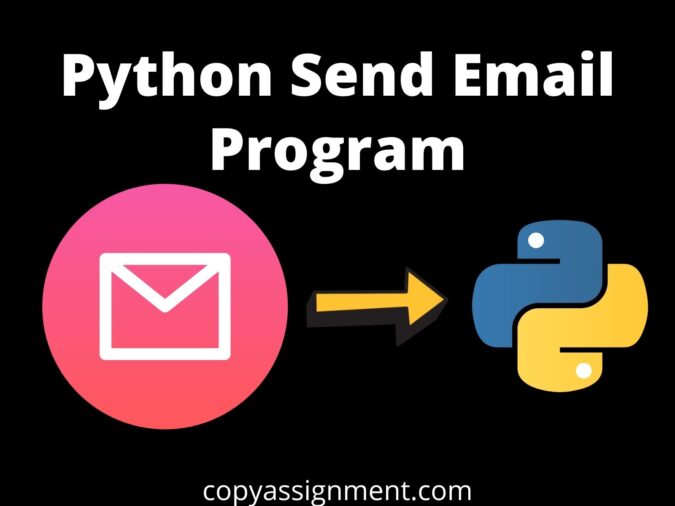
Did you know we can send emails using Python? Emails have become an essential part of any online profile. They are required to create accounts and managing those accounts across various platforms, applications, and websites. We mostly use emails for receiving and sending important information. So, today we will create a Python Send Email Program to send mail using python instead of email websites or software.
Check this video for more help:–
Credits– Edureka
How emails are sent generally?
When sending emails, the email is first taken through some protocols to determine whether it is spam mail or not. It usually has to be parsed by the Simple Mail Transfer Protocol(SMTP) and for various emails such as Gmail, it goes through more protocols. The SMTP also acts like a server whereby it receives the incoming email and locates the email address to send the email to. The SMTP cannot understand the domain name, so it contacts the DNS to decipher the IP address to send the email to.
Once the associated IP has been found, it can determine if that domain has any mail exchange servers (MX) which detail any additional information about where the message should be routed to.
By now, the SMTP has all the necessary information about the recipient to send the message from its server on to the email recipients MTA server. The MTA decides where exactly to put the mail, whether the recipient is using a client that works via POP or via the IMAP protocol. The recipient will then receive a new email notification and the mail will wait in the mailbox until it is fetched.
Sending emails using python
In this post, we’re going to create a simple Python Send Email Program without actually going to your email. There aren’t any prerequisites to this project if you already have python3.
Imports
You need to import two in-built modules in python; these are ‘smtp‘ and ‘ssl‘.
import ssl, smtplib #smtplib is the module used by python to send emails through SMTP. #The ssl module is used to access the Operating System Socket.
Definition
You need to define the server port that the smtp server will be run from the localhost.
import ssl, smtplib #smtplib is the module used by python to send emails through SMTP. #The ssl module is used to access the Operating System Socket. port = 465 #This port will be required later.
Login Essentials
We now need to get the sender’s email together with his or her password. Store them in variables that will be used in making an SMTP request.
import ssl, smtplib #smtplib is the module used by python to send emails through SMTP. #The ssl module is used to access the Operating System Socket. port = 465 #This port will be required later. email = input("Enter your email: ") #You key in the email address you want to send an email with. password = input("Enter your password: ") #This is the password to the email inputed before
Please note that most emails that you will send emails from needing authorization from the email. For example, if you’re using Gmail, you have to turn the less secure app on so that it can allow other apps to access your account. If your account still has this security feature on, it will throw back an error if you try sending your emails.
Email parameters
Next we need to get some required fields to send an email. An email is composed of the receiver’s address, a subject line and the body. We will input all this.
import ssl, smtplib #smtplib is the module used by python to send emails through SMTP. #The ssl module is used to access the Operating System Socket. port = 456 #This port will be required later. email = input("Enter your email: ") #You key in the email address you want to send an email with. password = input("Enter your password: ") #This is the password to the email inputed before recipient = input("Enter the email address to send the email to: ") #This is the email address which the emai is being sent to. subject = input("What is the subject of the email: ") #This is the subject head of the email text = input("Type your email here: ") #This is the body of the email. #We combine the subject and the text to form the subject head and the body. message = "Subject: {}\n\n{}".format(subject, text) context = ssl.create_default_context() #This method just creates a new class instance for implemenmtation of a secure protocol.
Initiating email server
And finally, we need to use the smtplib to send the email. We will first have to log in to send the email. If the login is successful, the email will be sent. If not, an error for authorization will show up in the terminal. After logging in we send the email.
We will have to close the SMTP and hence we place that block of code within a block that will automatically close when the email has been sent or will be closed.
import ssl, smtplib #smtplib is the module used by python to send emails through SMTP. #The ssl module is used to access the Operating System Socket. port = 465 #This port will be required later. email = input("Enter your email: ") #You key in the email address you want to send an email with. password = input("Enter your password: ") #This is the password to the email inputed before recipient = input("Enter the email address to send the email to: ") #This is the email address which the emai is being sent to. subject = input("What is the subject of the email: ") #This is the subject head of the email text = input("Type your email here: ") #This is the body of the email. #We combine the subject and the text to form the subject head and the body. message = "Subject: {}\n\n{}".format(subject, text) context = ssl.create_default_context() #This method just creates a new class instance for implemenmtation of a secure protocol. with smtplib.SMTP_SSL("smtp.gmail.com",port,context=context) as servers: #This syncs the local smtp server in the localhost with Gmail's server server.login(email,password) #This is the login credentials being checked. server.sendmail(email, recipient,message) #The server is initialized to send an email with the three parameters
Thanks for reading through my post and leave a comment below for any queries you might have.
You can check other posts below:
Create language translator using python
Download Youtube videos using python
Shut down computer with voice using python