Let’s see Company Logo Hackerrank Solution. A company wants a new logo. They want to set the letters of the logo based on the letters in their company name. They want to select 3 letters with the highest frequency in the company name. If the frequency of two letters is the same, then it should be sorted in alphabetical order.
Example
Input
aabbbccde
Output
b 3
a 2
c 2
Explanation
b is present 3 times. a, c are present 2 times, and d, e are present 1 time. So we include b first. a comes before c in alphabetical order so we include a and then we include c. 3 letters are done, so we discard the others.
Approach
First, we will need to calculate the frequency of each letter. This can be done using a dictionary in python. After that, we need to sort the values as per their frequency in descending order and their character in ascending order.
We will make use of the sorted function of python to do this. This would have been quite complicated in other languages, but Python makes it easy for us.
Let’s calculate the frequency
We check if the letter is present in the count if it is then we increment it by 1 and if it is not present we initialize it to 1.
count = {}
for val in s:
if count.get(val):
count[val] += 1
else:
count[val] = 1
Now the sorting part.
items = count.items()
This will give us a list of tuples containing a letter and their corresponding frequency
Now the most important part
items = sorted(items, key=lambda x: (x[1], -ord(x[0])), reverse=True)
We can pass sorted a function via the key argument to determine the sorting order. We are using the anonymous(lambda) function for this purpose.
lambda x: x[1] would have sorted the array by the frequency of each character. Setting reverse = True allows us to get the sorted array in descending order. But we also need to sort by the character in ascending order. Just lambda x: (x[1], x[0]) would sort characters also in descending order as the reverse is set to True. To overcome this we find the Unicode value of each character using the ord function and add a minus sign to it. The minus sign makes the value for the greatest and for z the smallest, thus it will sort in descending order which will again be reversed because of the reverse keyword and we will get the answer in ascending order.
Company Logo Hackerrank Solution in Python
s = input()
count = {}
for val in s:
if count.get(val):
count[val] += 1
else:
count[val] = 1
items = count.items()
items = sorted(items, key=lambda x: (x[1], -ord(x[0])), reverse=True)
for i in range(3):
print(items[i][0], items[i][1])
Output:
b 3
a 2
c 2
HackerRank screenshot

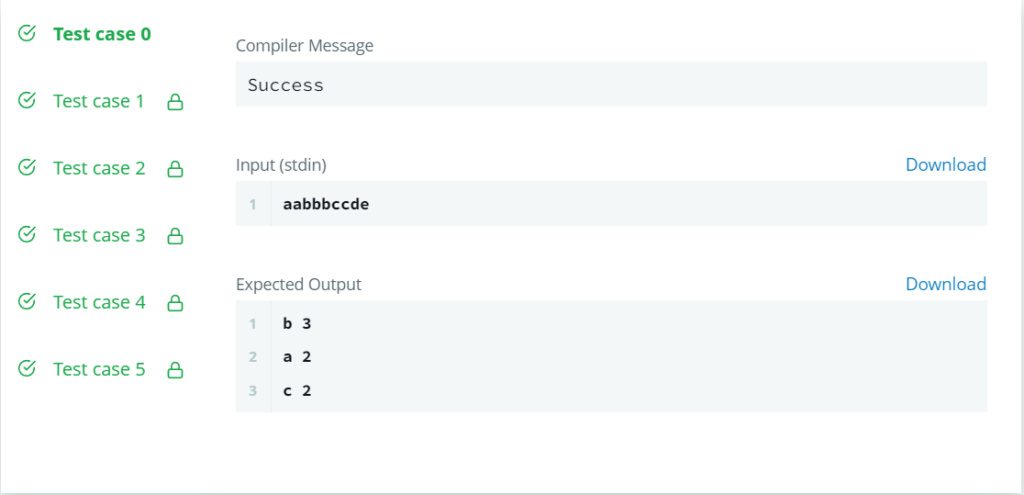
Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- HackerRank Day 8 Solution in Python: Dictionaries and Maps
- HackerRank Day 7 Solution in Python: Arrays
- HackerRank Day 6 Solution in Python: Let’s review
- HackerRank Day 5 Solution in Python: Loops
- HackerRank Day 4 Solution in Python: Class vs Instance
- HackerRank Day 3 Solution in Python: Intro to Conditional Statements
- HackerRank Day 2 Solution in Python: Operators
- HackerRank Day 1 Solution in Python: Data Types
- HackerRank Day 0 Solution in Python: Hello World
- HackerRank Day 29 Solution in Python: Bitwise AND
- HackerRank Day 28 Solution in Python: RegEx, Patterns, and Intro to databases
- HackerRank Day 27 Solution in Python: Testing
- HackerRank Day 26 Solution in Python: Nested Logic
- HackerRank Day 25 Solution in Python: Running Time and Complexity
- HackerRank Day 24 Solution in Python: More Linked Lists
- HackerRank Day 23 Solution in Python: BST Level Order Traversal
- HackerRank Day 22 Solution in Python: Binary Search Trees
- Find Peak Element LeetCode 162
- HackerRank Day 20 Solution in Python: Sorting
- HackerRank Day 19 Solution in Python: Interfaces
- HackerRank Day 18 Solution in Python: Queues and Stacks
- HackerRank Day 17 Solution in Python: More Exceptions
- HackerRank Day 16 Solution: Exceptions – String to Integer
- Explained: Allocate minimum number of pages
- HackerRank Day 15 Solution in Python: Linked List
- Search a 2D matrix: leetcode 74
- Maximum Subarray Sum: Kadane’s Algorithm
- HackerRank Day 13 Solution in Python: Abstract Classes
- HackerRank Day 14 Solution in Python: Scope