In this article, we will build an application, Process Monitor in python using psutil. Python has a wide range of libraries and packages, which makes it the best choice for many developers. In the same way, we are going to make use of the psutil package to build our application, Process Monitor in Python.
What is Process Monitor in Python?
A process monitor is a tool that displays system information like processes, memory, network, and other required information. There are plenty of tools available to accomplish this task, but we can easily make our own process monitor using Python. In Python, there is a module called psutil that we can use to retrieve various information about our system. psutil also provides lots of features to monitor the system.
psutil package in Python
With the psutil package installed in the Python environment, we can obtain information about CPU and RAM usage. This makes it easy to add system utilization monitoring functionality to your own Python program. With psutil, we can quickly build our process monitor in Python. We will see how to install the psutil package and how we can use it, to monitor the CPU and RAM usage with the Python program.
Prerequisites for the project
Let’s start our project by installing the dependencies required for the project. Use the following command to download the psutil module in python.
pip install psutil
Use the following command to download the pandas module in python.
pip install pandas
Steps to develop Process monitor in Python
After installing the psutil and pandas library, create a Python file and start importing the required libraries.
#Importing the required libraries import psutil import datetime import pandas as pd
Next, we have to create variables to store the respective information we are going to display on the screen.
#Creating lists to store the corresponding information cpu_usage= [] memory_usage = [] memory_usage_percentage =[] pids = [] name = [] status =[] create_time =[] threads =[]
The psutil module provides a Python generator function, process_iter() that can iterate over all the processes running on the local system.
#Getting application process information using psutil for process in psutil.process_iter(): pids.append(process.pid) name.append(process.name()) cpu_usage.append(process.cpu_percent(interval=1)/psutil.cpu_count()) memory_usage.append(round(process.memory_info().rss/(1024*1024),2)) memory_usage_percentage.append(round(process.memory_percent(),2)) create_time.append(datetime.datetime.fromtimestamp( process.create_time()).strftime("%Y%m%d - %H:%M:%S")) status.append(process.status()) threads.append(process.num_threads())
The functions we used in our program are used for the corresponding process in python.
pid() is used to get the process id number
name() is used to get the name of the process
cpu_percent() is used for the percentage of CPU utilization of the process
memory_info() function calculates memory usage by the process
memory_percent() is for finding the process memory percentage by comparing the process memory
create_time() finds the process creation time in seconds.
status() gives the running status of the process.
num_threads() prints the number of threads used by the process.
fromtimestamp() converts the creation time seconds in readable time format
strftime() function will convert the date-time object to a readable string
Next, let’s create a data dictionary that will contain all the process details.
#Saving the process information in a python dictionary data = {"PIds":pids, "Name": name, "CPU":cpu_usage, "Memory Usages(MB)":memory_usage, "Memory Percentage(%)": memory_usage_percentage, "Status": status, "Created Time": create_time, "Threads": threads, }
Convert the dictionary we stored, into Pandas DataFrame. Then set the index and sort the values. Finally print the details on the screen.
#Converting the dictionary into Pandas DataFrame process_df = pd.DataFrame(data) #Setting the index to pids process_df =process_df.set_index("PIds") #sorting the process process_df =process_df.sort_values(by='Memory Usages(MB)', ascending=False) #Adding MB at the end of memory process_df["Memory Usages(MB)"] = process_df["Memory Usages(MB)"].astype(str) + " MB" print(process_df)
And that’s it we have built our Process monitor program in python successfully!
Complete Program to Make a Process Monitor in Python
The full code to make a Process Monitor in Python is given below.
#Importing the required libraries import psutil import datetime import pandas as pd #Creating lists to store the corresponding information cpu_usage= [] memory_usage = [] memory_usage_percentage = [] pids = [] name = [] status =[] create_time =[] threads =[] #Getting application process information using psutil for process in psutil.process_iter(): pids.append(process.pid) name.append(process.name()) cpu_usage.append(process.cpu_percent(interval=1)/psutil.cpu_count()) memory_usage.append(round(process.memory_info().rss/(1024*1024),2)) memory_usage_percentage.append(round(process.memory_percent(),2)) create_time.append(datetime.datetime.fromtimestamp( process.create_time()).strftime("%Y%m%d - %H:%M:%S")) status.append(process.status()) threads.append(process.num_threads()) #Saving the process information in a python dictionary data = {"PIds":pids, "Name": name, "CPU":cpu_usage, "Memory Usages(MB)":memory_usage, "Memory Percentage(%)": memory_usage_percentage, "Status": status, "Created Time": create_time, "Threads": threads, } #Converting the dictionary into Pandas DataFrame process_df = pd.DataFrame(data) #Setting the index to pids process_df =process_df.set_index("PIds") #sorting the process process_df =process_df.sort_values(by='Memory Usages(MB)', ascending=False) #Adding MB at the end of memory process_df["Memory Usages(MB)"] = process_df["Memory Usages(MB)"].astype(str) + " MB" print(process_df)
When you execute the above program, it might take a few minutes to execute. After the complete execution, you will see a similar output:
Output:
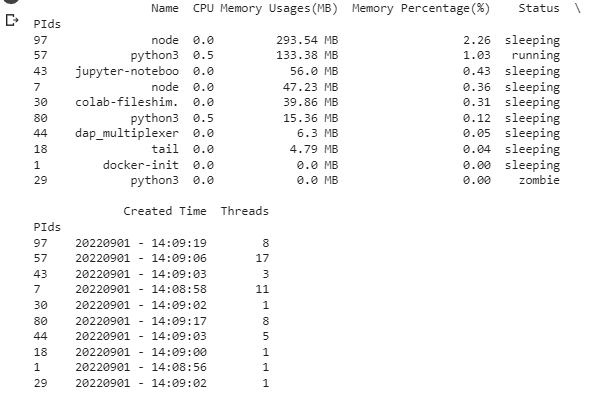
Conclusion
In this article, we developed an application, process monitor in python using psutil. We have also learned about a number of Python functions to obtain CPU and RAM usage, based on the psutil package. Hope you enjoyed building this project!
Also Read:
- What is web development for beginners?
- Python | Check Armstrong Number using for loop
- Python | Factorial of a number using for loop
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- Create your own ChatGPT with Python
- Radha Krishna using Python Turtle
- Python Programming Examples | Fundamental Programs in Python
- Python | Delete object of a class
- Python | Modify properties of objects
- Python classmethod
- Python | Create a class that takes 2 parameters, print both parameters
- Python | Create a class, create an object of the class, access, and print property value
- Python | Find the maximum element in a list using lambda and reduce()
- Python | filter numbers(that are not divisible by 3 and 4) from a list using lambda and filter()
- Python | lambda function that returns the maximum of 2 numbers
- Python | Convert all strings of a list to uppercase using lambda
- Python | Square numbers of a list using lambda
- Python | Reverse and convert a string to uppercase using lambda
- Python | Convert String to uppercase using lambda
- Python | Reverse a list using lambda
- Python | Calculator using lambda
- Python | Square of a number using lambda
- Python | Multiply numbers using lambda
- Python | lambda with None
- Python | lambda with pass statement
- Python | Add numbers using lambda
- Python | Print Namaste using lambda
- Iterate over a string in Python
- Python | join() | Join list of strings