
Today, we will be making a simple BMI Calculator in HTML CSS JavaScript. We will use JavaScript to calculate the BMI and display the result. We will also use JavaScript to validate the input fields. We will use HTML and CSS to create the form and display the result.
BMI stands for Body Mass Index. It is a simple calculation using a person’s height and weight. The formula is the weight (kg) / [height (m)]2. A BMI of 25.0 or more is overweight, while the healthy range is 18.5 to 24.9.
We will use JavaScript to calculate the BMI and display the result.
Requirements
- Code Editor (VS Code Preferred)
- Chromium Browser (Chrome Preferred)
- Basic Knowledge of HTML, CSS, Javascript, and Bootstrap
Features
- Calculate BMI
- Display BMI
- Display BMI Category
- Let the user select length units (cm, m, ft, in)
- Let the user select weight units (kg, lbs, g, oz)
- Scroll the controller to select the length and weight units
Folder Structure
We will be using HTML, CSS, BootStrap, and JavaScript for developing a BMI Calculator. Check out this image for the folder structure of our project.
The project will include two files, index.html, and index.js, we will be adding the small CSS within the HTML file itself to style the page further.
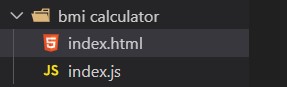
index.html contains our Html code.
some CSS and bootstrap have been used to style our web page.
logic.js contains all the logic.
Coding BMI Calculator in HTML CSS JavaScript
The main function for calculating the BMI is here
function calculateBMI(height, weight) {
const heightUnit = document.getElementById("height-unit").value;
const weightUnit = document.getElementById("weight-unit").value;
// Convert height to meter
if (heightUnit === "cm") {
height /= 100;
} else if (heightUnit === "in") {
height /= 39.37;
} else if (heightUnit === "ft") {
height /= 3.281;
}
// Convert weight to kg
if (weightUnit === "g") {
weight /= 1000;
} else if (weightUnit === "lbs") {
weight /= 2.205;
} else if (weightUnit === "oz") {
weight /= 35.274;
}
// calculate bmi from height in meter and weight in kg
bmi = weight / (height * height);
if (bmi < 18.5) {
remark = "Underweight";
textColor = "red";
} else if (bmi >= 18.5 && bmi <= 24.9) {
remark = "Normal";
textColor = "green";
} else if (bmi >= 25 && bmi <= 29.9) {
remark = "Overweight";
textColor = "orange";
} else if (bmi >= 30) {
remark = "Obese";
textColor = "red";
}
updateValue(bmi, remark, textColor);
}
HTML Code
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <title>BMI Calculator</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-Zenh87qX5JnK2Jl0vWa8Ck2rdkQ2Bzep5IDxbcnCeuOxjzrPF/et3URy9Bv1WTRi" crossorigin="anonymous" /> </head> <style> .calculator { display: flex; flex-direction: column; width: fit-content; height: min-content; background-color: #f1f1f1; padding: 50px; border-radius: 10px; box-shadow: 0 0 10px 0 rgba(0, 0, 0, 0.2); margin: 0 auto; margin-top: 50px; flex-wrap: wrap; } .form { margin: 40px auto 0 auto; } .input-value { display: flex; flex-direction: row; justify-content: center; } #height, #weight { width: 400px; } #weight-unit, #height-unit { width: fit-content; } .input-value * { margin: 0 5px; } </style> <body> <div class="calculator"> <h1 class="text-center">BMI Calculator</h1> <div class="form"> <div class="mb-3"> <label class="form-label"> Height: <div class="input-value"> <input id="height" class="form-range" type="range" value="1" min="1" max="300" /> <span id="height-value">0</span> <!-- Select Height unit --> <select id="height-unit" class="form-select"> <option value="cm">cm</option> <option value="m">m</option> <option value="in">in</option> <option value="ft">ft</option> </select> </div> </label class="form-label"> </div> <div class="mb-3"> <label class="form-label"> Weight: <div class="input-value"> <input id="weight" class="form-range" type="range" value="1" min="1" max="300" /> <span id="weight-value">0</span> <!-- Select Weight unit --> <select id="weight-unit" class="form-select"> <option value="kg">kg</option> <option value="g">g</option> <option value="lbs">lbs</option> <option value="oz">oz</option> </select> </div> </label> </div> </div> <div class="text-center"> <h1 class="bmi"></h1> <h2 class="remark"></h2> </div> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-OERcA2EqjJCMA+/3y+gxIOqMEjwtxJY7qPCqsdltbNJuaOe923+mo//f6V8Qbsw3" crossorigin="anonymous"></script> <!-- link js from index.js --> <script src="index.js"></script> </body> </html>
JavaScript Code
const height = document.getElementById("height"); const weight = document.getElementById("weight"); const bmiElem = document.querySelector(".bmi"); const remarkElem = document.querySelector(".remark"); const heightUnit = document.getElementById("height-unit"); const weightUnit = document.getElementById("weight-unit"); const heightValue = document.getElementById("height-value"); const weightValue = document.getElementById("weight-value"); let textColor = "green"; let remark = "Normal"; let bmi = 0; bmiElem.innerHTML = bmi; function resetDefault(height, weight) { if (height !== null) { height.value = height; } if (weight !== null) { weight.value = weight; } } function updateValue(bmi, remark, textColor) { bmiElem.innerHTML = `${bmi.toPrecision(4)}`; remarkElem.innerHTML = remark; remarkElem.style.color = textColor; } function calculateBMI(height, weight) { const heightUnit = document.getElementById("height-unit").value; const weightUnit = document.getElementById("weight-unit").value; // Convert height to meter if (heightUnit === "cm") { height /= 100; } else if (heightUnit === "in") { height /= 39.37; } else if (heightUnit === "ft") { height /= 3.281; } // Convert weight to kg if (weightUnit === "g") { weight /= 1000; } else if (weightUnit === "lbs") { weight /= 2.205; } else if (weightUnit === "oz") { weight /= 35.274; } // calculate bmi from height in meter and weight in kg bmi = weight / (height * height); if (bmi < 18.5) { remark = "Underweight"; textColor = "red"; } else if (bmi >= 18.5 && bmi <= 24.9) { remark = "Normal"; textColor = "green"; } else if (bmi >= 25 && bmi <= 29.9) { remark = "Overweight"; textColor = "orange"; } else if (bmi >= 30) { remark = "Obese"; textColor = "red"; } updateValue(bmi, remark, textColor); } // Adjust the min and max attribute of the height and weight input range heightUnit.addEventListener("change", () => { const heightUnitValue = heightUnit.value; if (heightUnitValue === "cm") { height.min = 1; height.max = 300; resetDefault(1, null); } else if (heightUnitValue === "m") { height.min = 1; height.max = 5; resetDefault(1, null); } else if (heightUnitValue === "in") { height.min = 1; height.max = 118; resetDefault(1, null); } else if (heightUnitValue === "ft") { height.min = 1; height.max = 9; resetDefault(1, null); } calculateBMI(height.value, weight.value); }); weightUnit.addEventListener("change", () => { const weightUnitValue = weightUnit.value; if (weightUnitValue === "kg") { weight.min = 1; weight.max = 300; resetDefault(null, 1); } else if (weightUnitValue === "g") { weight.min = 1000; weight.max = 300000; resetDefault(null, 1); } else if (weightUnitValue === "lbs") { weight.min = 2; weight.max = 661; resetDefault(null, 1); } else if (weightUnitValue === "oz") { weight.min = 35; weight.max = 10582; resetDefault(null, 1); } calculateBMI(height.value, weight.value); }); height.addEventListener("input", () => { heightValue.innerHTML = height.value; calculateBMI(height.value, weight.value); }); weight.addEventListener("input", () => { weightValue.innerHTML = weight.value; calculateBMI(height.value, weight.value); });
Output:
Image Output
Video output
Conclusion
I hope this was easy to understand BMI Calculator in HTML CSS JavaScript and that you enjoyed building and learning this at the same time, we learned to use sliders to select input. Along the way, we did some basic dom-manipulation to change the text elements on the page. We also learned extensive use of javascript conditional statements. We did end up making a project which we can use to calculate the BMI of any person even if the height and weight are in different units.
Also Read:
- What is web development for beginners?
- Chat App with Node.js and Socket.io
- Draw Doraemon using HTML and CSS
- Draw House using HTML and CSS
- Draw Dog using CSS
- Rock Paper Scissor in HTML CSS JavaScript
- Pong Game in HTML and JavaScript
- Tip Calculator in HTML and JavaScript
- Calculator in HTML CSS JavaScript
- BMI Calculator in HTML CSS JavaScript
- Color picker in HTML and JavaScript
- Number Guessing Game in JavaScript
- ATM in JavaScript
- Inventory Management System in JavaScript
- Courier Tracking System in HTML CSS and JS
- Word Count App in JavaScript
- To-Do List in HTML CSS JavaScript
- Tic-Tac-Toe game in JavaScript
- Music player using HTML CSS and JavaScript
- Happy Diwali in JavaSCript