
Introduction
Hello everyone!
Today we will make a simple Inventory Management System in JavaScript as a web development project. In this tutorial we will try to learn more about Dom manipulation also we will be using bootstrap to further make the task of styling our site much easier.
Requirements:
- Code Editor (VS Code Preferred)
- Chromium Browser (Chrome Preferred)
- Basic Knowledge of HTML, CSS, Javascript, and Bootstrap
Features:
- Good UI for all the pages
- Adding items to inventory
- Deleting all items from the inventory
- Clearing all items from all the fields
Folder structure of project:

index.html
- It will be the barebones structure file that will shape how our contents are on the website
style.css
- The styles file includes the alignment, aesthetics, and the basic overall look of how our website looks
main.js
- The javascript files include all the logic, including the login and logout features, the dom-manipulation tricks, and essential functionalities to show the status of the shipment.
Coding Inventory Management System in JavaScript
HTML Code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Inventory Management</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-Zenh87qX5JnK2Jl0vWa8Ck2rdkQ2Bzep5IDxbcnCeuOxjzrPF/et3URy9Bv1WTRi" crossorigin="anonymous" /> <link rel="stylesheet" href="main.css" /> </head> <body> <header> <nav class="navbar navbar-expand-lg bg-light"> <div class="container-fluid"> <a class="navbar-brand" href="#">Inventory Management</a> <button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation" > <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarNav"> <ul class="navbar-nav"> <li class="nav-item"> <a class="nav-link active" aria-current="page" href="#">Home</a> </li> <li class="nav-item"> <a class="nav-link" href="#">More Info</a> </li> </ul> <ul class="navbar-nav"> <li class="nav-item"> <a class="nav-link" href="#">Login</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Register</a> </li> </ul> </div> </div> </nav> </header> <main> <div class="container"> <div class="heading"> <h1> Inventory Manager <button type="button" onclick="clearAll()" class="btn btn-danger"> Clear All </button> </h1> </div> <div class="tabs"> <ul class="nav nav-tabs" id="myTab" role="tablist"> <li class="nav-item" role="presentation"> <button class="nav-link active" id="home-tab" data-bs-toggle="tab" data-bs-target="#home-tab-pane" type="button" role="tab" aria-controls="home-tab-pane" aria-selected="true" > Current Inventory </button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="profile-tab" data-bs-toggle="tab" data-bs-target="#profile-tab-pane" type="button" role="tab" aria-controls="profile-tab-pane" aria-selected="false" > Incoming Purchase </button> </li> <li class="nav-item" role="presentation"> <button class="nav-link" id="contact-tab" data-bs-toggle="tab" data-bs-target="#contact-tab-pane" type="button" role="tab" aria-controls="contact-tab-pane" aria-selected="false" > Outgoing Orders </button> </li> </ul> <div class="tab-content" id="myTabContent"> <div class="tab-pane fade show active" id="home-tab-pane" role="tabpanel" aria-labelledby="home-tab" tabindex="0" > <div class="heading"> <h4>Current Inventory</h4> <button type="button" onclick="clearCurrentInventory()" class="btn btn-danger" > Clear Inventory </button> </div> <div class="add-item"> <form class="form"> <div class="mb-3"> <label class="form-label"> Product Name: <input id="current_order_product_name" class="form-control" type="text" required /> </label> <label class="form-label"> Product Brand: <input id="current_order_product_brand" class="form-control" type="text" required /> </label> </div> <div class="mb-3"> <label class="form-label"> Quantity: <input id="current_order_product_quantity" class="form-control" type="number" required /> </label> <label class="form-label"> Product Price: <input id="current_order_product_price" class="form-control" type="number" required /> </label> </div> <div class="mb-3"> <button class="btn btn-success" onclick="addCurrentInventory()" type="button" > Add </button> <button class="btn btn-danger" type="reset">Reset</button> </div> </form> </div> <table class="table"> <thead> <tr> <th scope="col">#</th> <th scope="col">Name</th> <th scope="col">Brand</th> <th scope="col">Quantity</th> <th scope="col">Price</th> </tr> </thead> <tbody id="current_inventory_list"> <tr> <th scope="row">1</th> <td>Acer Nitro 7</td> <td>Acer</td> <td>10</td> <td>Rs 30000</td> </tr> <tr> <th scope="row">2</th> <td>Asus Rog 17</td> <td>Asus</td> <td>15</td> <td>Rs 50000</td> </tr> <tr> <th scope="row">3</th> <td>HP Rog 17</td> <td>HP</td> <td>20</td> <td>Rs 45000</td> </tr> </tbody> </table> </div> <div class="tab-pane fade" id="profile-tab-pane" role="tabpanel" aria-labelledby="profile-tab" tabindex="0" > <div class="heading"> <h4>Incoming Product</h4> <button onclick="clearIncomingOrder()" type="button" class="btn btn-danger" > Clear Incoming </button> </div> <div class="add-item"> <form class="form"> <div class="mb-3"> <label class="form-label"> Product Name: <input id="incoming_order_product_name" class="form-control" type="text" required /> </label> <label class="form-label"> Product Brand: <input id="incoming_order_product_brand" class="form-control" type="text" required /> </label> </div> <div class="mb-3"> <label class="form-label"> Quantity: <input id="incoming_order_product_quantity" class="form-control" type="number" required /> </label> <label class="form-label"> Product Price: <input id="incoming_order_product_price" class="form-control" type="number" required /> </label> </div> <div class="mb-3"> <button class="btn btn-success" onclick="addIncomingOrder()" type="button" > Add </button> <button class="btn btn-danger" type="reset">Reset</button> </div> </form> </div> <table class="table"> <thead> <tr> <th scope="col">#</th> <th scope="col">Name</th> <th scope="col">Brand</th> <th scope="col">Quantity</th> <th scope="col">Price</th> </tr> </thead> <tbody id="incoming_inventory_list"> <tr> <th scope="row">1</th> <td>Acer Nitro 7</td> <td>Acer</td> <td>30</td> <td>Rs 60000</td> </tr> <tr> <th scope="row">2</th> <td>Asus Rog 17</td> <td>Asus</td> <td>25</td> <td>Rs 90000</td> </tr> <tr> <th scope="row">3</th> <td>HP Rog 17</td> <td>HP</td> <td>40</td> <td>Rs 105000</td> </tr> </tbody> </table> </div> <div class="tab-pane fade" id="contact-tab-pane" role="tabpanel" aria-labelledby="contact-tab" tabindex="0" > <div class="heading"> <h4>Outgoing Orders</h4> <button type="button" onclick="clearOutgoingOrder()" class="btn btn-danger" > Clear Outgoing </button> </div> <div class="add-item"> <form class="form"> <div class="mb-3"> <label class="form-label"> Product Name: <input id="outgoing_order_product_name" class="form-control" type="text" required /> </label> <label class="form-label"> Product Brand: <input id="outgoing_order_product_brand" class="form-control" type="text" required /> </label> </div> <div class="mb-3"> <label class="form-label"> Quantity: <input id="outgoing_order_product_quantity" class="form-control" type="number" required /> </label> <label class="form-label"> Product Price: <input id="outgoing_order_product_price" class="form-control" type="number" required /> </label> </div> <div class="mb-3"> <button class="btn btn-success" onclick="addOutgoingOrder()" type="button" > Add </button> <button class="btn btn-danger" type="reset">Reset</button> </div> </form> </div> <table class="table"> <thead> <tr> <th scope="col">#</th> <th scope="col">Name</th> <th scope="col">Brand</th> <th scope="col">Quantity</th> <th scope="col">Price</th> </tr> </thead> <tbody id="outgoing_inventory_list"> <tr> <th scope="row">1</th> <td>Acer Nitro 7</td> <td>Acer</td> <td>5</td> <td>Rs 15000</td> </tr> <tr> <th scope="row">2</th> <td>Asus Rog 17</td> <td>Asus</td> <td>10</td> <td>Rs 25000</td> </tr> <tr> <th scope="row">3</th> <td>HP Rog 17</td> <td>HP</td> <td>15</td> <td>Rs 22500</td> </tr> </tbody> </table> </div> </div> </div> </div> </main> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-OERcA2EqjJCMA+/3y+gxIOqMEjwtxJY7qPCqsdltbNJuaOe923+mo//f6V8Qbsw3" crossorigin="anonymous" ></script> <script src="main.js"></script> </body> </html>
JavaScript Code:
let current_inventory = { 1: { name: "Acer Nitro 7", brand: "Acer", price: 1000, quantity: 10, }, 2: { name: "Asus ROG Strix", brand: "Asus", price: 1200, quantity: 5, }, 3: { name: "MSI GL65 Leopard", brand: "MSI", price: 1500, quantity: 3, }, 4: { name: "Lenovo Legion Y540", brand: "Lenovo", price: 1300, quantity: 7, }, 5: { name: "HP Omen 15", brand: "HP", price: 1100, quantity: 8, }, }; let incoming_purchase = { 1: { name: "Acer Nitro 7", brand: "Acer", price: 1000, quantity: 5, }, 2: { name: "Asus ROG Strix", brand: "Asus", price: 1200, quantity: 5, }, 3: { name: "MSI GL65 Leopard", brand: "MSI", price: 1500, quantity: 3, }, 4: { name: "Lenovo Legion Y540", brand: "Lenovo", price: 1300, quantity: 7, }, 5: { name: "HP Omen 15", brand: "HP", price: 1100, quantity: 8, }, 6: { name: "Dell G3", brand: "Dell", price: 900, quantity: 10, }, }; let orders = { 1: { name: "Acer Nitro 7", brand: "Acer", price: 1000, quantity: 5, }, }; function is_empty(string) { if (string.length == 0) { return true; } return false; } function getIndex(tbody) { let index = tbody.children.length || 0; return index; } function clearAll() { clearCurrentInventory(); clearIncomingOrder(); clearOutgoingOrder(); } function clearCurrentInventory() { document.querySelector("#current_inventory_list").innerHTML = ""; } function clearIncomingOrder() { document.querySelector("#incoming_inventory_list").innerHTML = ""; } function clearOutgoingOrder() { document.querySelector("#outgoing_inventory_list").innerHTML = ""; } function addCurrentInventory() { let productName = document.querySelector("#current_order_product_name").value; let productBrand = document.querySelector( "#current_order_product_brand" ).value; let productPrice = document.querySelector( "#current_order_product_price" ).value; let productQuantity = document.querySelector( "#current_order_product_quantity" ).value; if ( is_empty(productName) || is_empty(productBrand) || is_empty(productPrice) || is_empty(productQuantity) ) { alert("Please fill out all fields"); return; } let tbody = document.querySelector("#current_inventory_list"); let tr = `<tr> <th scope='row'>${getIndex(tbody) + 1}</th> <td>${productName}</td> <td>${productBrand}</td> <td>${productQuantity}</td> <td>$${productPrice}</td>`; tbody.innerHTML += tr; } function addIncomingOrder() { let productName = document.querySelector( "#incoming_order_product_name" ).value; let productBrand = document.querySelector( "#incoming_order_product_brand" ).value; let productPrice = document.querySelector( "#incoming_order_product_price" ).value; let productQuantity = document.querySelector( "#incoming_order_product_quantity" ).value; if ( is_empty(productName) || is_empty(productBrand) || is_empty(productPrice) || is_empty(productQuantity) ) { alert("Please fill out all fields"); return; } let tbody = document.querySelector("#incoming_inventory_list"); let tr = `<tr> <th scope='row'>${getIndex(tbody) + 1}</th> <td>${productName}</td> <td>${productBrand}</td> <td>${productQuantity}</td> <td>$${productPrice}</td>`; tbody.innerHTML += tr; } function addOutgoingOrder() { let productName = document.querySelector( "#outgoing_order_product_name" ).value; let productBrand = document.querySelector( "#outgoing_order_product_brand" ).value; let productPrice = document.querySelector( "#outgoing_order_product_price" ).value; let productQuantity = document.querySelector( "#outgoing_order_product_quantity" ).value; if ( is_empty(productName) || is_empty(productBrand) || is_empty(productPrice) || is_empty(productQuantity) ) { alert("Please fill out all fields"); return; } let tbody = document.querySelector("#outgoing_inventory_list"); let tr = `<tr> <th scope='row'>${getIndex(tbody) + 1}</th> <td>${productName}</td> <td>${productBrand}</td> <td>${productQuantity}</td> <td>$${productPrice}</td>`; tbody.innerHTML += tr; }
CSS Code:
.collapse { display: flex; justify-content: space-between; flex-direction: row; } .form-label { margin-right: 100px; } .heading { display: flex; flex-direction: row; justify-content: space-between; } @media screen and (max-width: 991px) { .collapse { display: inline; } }
Output:
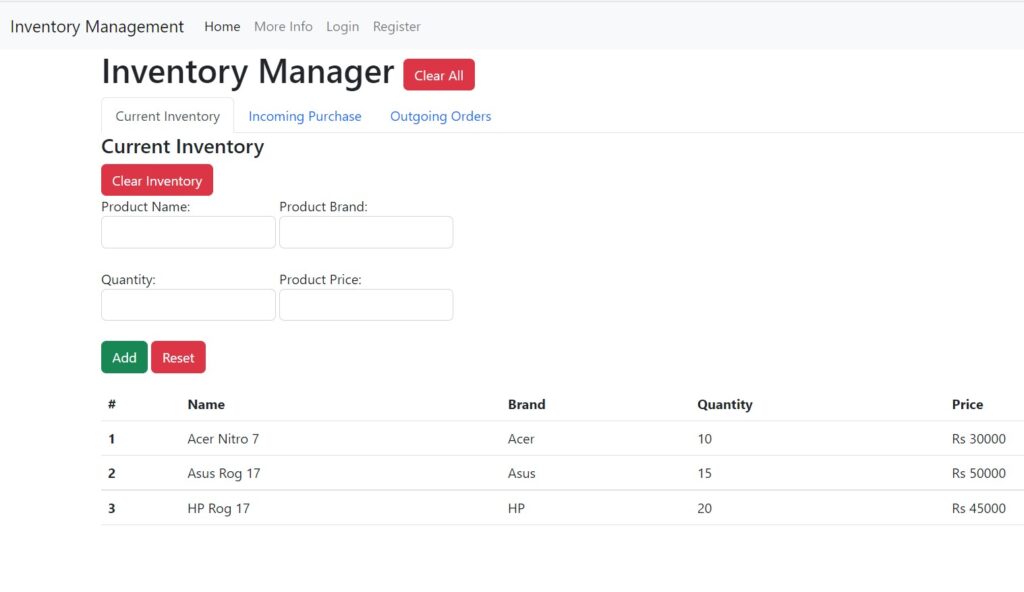
Also Read:
- What is web development for beginners?
- Chat App with Node.js and Socket.io
- Draw Doraemon using HTML and CSS
- Draw House using HTML and CSS
- Draw Dog using CSS
- Rock Paper Scissor in HTML CSS JavaScript
- Pong Game in HTML and JavaScript
- Tip Calculator in HTML and JavaScript
- Calculator in HTML CSS JavaScript
- BMI Calculator in HTML CSS JavaScript
- Color picker in HTML and JavaScript
- Number Guessing Game in JavaScript
- ATM in JavaScript
- Inventory Management System in JavaScript
- Courier Tracking System in HTML CSS and JS
- Word Count App in JavaScript
- To-Do List in HTML CSS JavaScript
- Tic-Tac-Toe game in JavaScript
- Music player using HTML CSS and JavaScript
- Happy Diwali in JavaSCript