
Today, we will be making a simple Calculator in HTML CSS JavaScript, where we can use the app to do basic arithmetic calculations. We will be using simple HTML and some basic javascript to get the job done.
We will use JavaScript to calculate and display the result.
Requirements
- Code Editor (VS Code Preferred)
- Chromium Browser (Chrome Preferred)
- Basic Knowledge of HTML, CSS, Javascript, and Bootstrap
Features
- Performing simple mathematical calculations
- Error handling in case of wrong expressions
Folder Structure
We will be using HTML, CSS, BootStrap, and JavaScript for developing a simple Calculator. Check out this image for the folder structure of our project.
The project will include three files, index.html, index.js, and style.css.
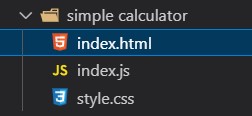
index.html contains our Html code.
style.css has been used to style our web page.
logic.js contains all the logic.
Coding Calculator in HTML CSS JavaScript
HTML Code
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <link rel="stylesheet" href="style.css"> <title>Calculator</title> </head> <body> <div class="container"> <h1>Simple Calculator</h1> <div class="calculator"> <input type="text" name="screen" id="display"> <table> <tr> <td><button>(</button></td> <td><button>)</button></td> <td><button>C</button></td> <td><button>=</button></td> </tr> <tr> <td><button>9</button></td> <td><button>8</button></td> <td><button>7</button></td> <td><button>+</button></td> </tr> <tr> <td><button>6</button></td> <td><button>5</button></td> <td><button>4</button></td> <td><button>-</button></td> </tr> <tr> <td><button>3</button></td> <td><button>2</button></td> <td><button>1</button></td> <td><button>X</button></td> </tr> <tr> <td><button>0</button></td> <td><button>.</button></td> <td><button>%</button></td> <td><button>/</button></td> </tr> </table> </div> </div> </body> <script src="index.js"></script> </html>
JavaScript Code
To do most of the calculations and detect user input we will take help from this loop, also we will try to catch errors when using the eval function in js, so we used a try-catch block.
let screen = document.getElementById("display"); buttons = document.querySelectorAll("button"); let screenValue = ""; for (item of buttons) { item.addEventListener("click", (e) => { buttonText = e.target.innerText; if (buttonText == "X") { buttonText = "*"; screenValue += buttonText; screen.value = screenValue; } else if (buttonText == "C") { screenValue = ""; screen.value = screenValue; } else if (buttonText == "=") { var result; try { result = eval(screenValue); } catch (error) { result = "Expression error"; } screen.value = result; } else { screenValue += buttonText; screen.value = screenValue; } }); }
CSS code
.container{ text-align: center; margin-top:23px } table{ margin: auto; } input{ border-radius: 21px; font-size:34px; height: 65px; width: 260px; scroll-behavior: smooth; } button{ border-radius: 20px; font-size: 20px; background: #ffffff; width: 60px; height: 50px; margin: 6px; } .calculator{ background-color: #161616; padding: 23px; border-radius: 53px; display: inline-block; }
Output:

Conclusion
I hope this was easy to understand Calculator in HTML CSS JavaScript and that you enjoyed building and learning this at the same time. Along the way, we did some basic dom-manipulation to change the text of the UI, and we also learned to handle exceptions in javascript.
Also Read:
- What is web development for beginners?
- Chat App with Node.js and Socket.io
- Draw Doraemon using HTML and CSS
- Draw House using HTML and CSS
- Draw Dog using CSS
- Rock Paper Scissor in HTML CSS JavaScript
- Pong Game in HTML and JavaScript
- Tip Calculator in HTML and JavaScript
- Calculator in HTML CSS JavaScript
- BMI Calculator in HTML CSS JavaScript
- Color picker in HTML and JavaScript
- Number Guessing Game in JavaScript
- ATM in JavaScript
- Inventory Management System in JavaScript
- Courier Tracking System in HTML CSS and JS
- Word Count App in JavaScript
- To-Do List in HTML CSS JavaScript
- Tic-Tac-Toe game in JavaScript
- Music player using HTML CSS and JavaScript
- Happy Diwali in JavaSCript