
Welcome friends, in this article we will learn how to draw windows logo using python turtle. This is going to be a very short but interesting article, especially for beginners because we have explained every line of code using comments so that even a beginner can understand the logic very easily.
You can check our website for more articles related to python turtle and its projects. You can use our website’s search bar or you can also visit our home page.
Let’s Draw Windows logo using Python Turtle
1. importing turtle
from turtle import *
2. setting turtle speed and width
speed(1)
width(4)
3. setting background color and turtle color
bgcolor('black')
color('blue')
Here, in the code, begin_fill() means to start filling the shape we are drawing with the color ‘blue’.
In the complete code, penup() means the turtle will not draw anything while it is moving from one coordinate to another, and pendown() means to turtle will draw if it is moving.
4. Drawing the blue color shape
# moving turtle to starting point
goto(-50,60)
pendown()
# going to right top
goto (100,100)
# going to right bottom
goto (100,-100)
# going to left bottom
goto(-50,-60)
# going to starting point
goto(-50,60)
5. Cutting the shape into two halves horizontally
# starting from left middle
goto(-50,0)
pendown()
# ending at right middle
goto(100, 0)
6. Cutting the shape into two halves vertically
# cutting the shape into two halves vertically
# starting from upper middle
goto (25,80)
pendown()
# ending at bottom middle
goto(25,-80)
7. Stopping the turtle with done()
done()
Complete code
# Windows Logo # importing turtle from turtle import * # setting turtle speed speed(1) width(4) # setting background color bgcolor('black') # setting color to blue color('blue') begin_fill() penup() # moving turtle to starting point goto(-50,60) pendown() # going to right top goto (100,100) # going to right bottom goto (100,-100) # going to left bottom goto(-50,-60) # going to starting point goto(-50,60) end_fill() penup() # setting turtle color to black color('black') # changing width of turtle width(10) # cutting the shape into two equal halves horizontally # starting from left middle goto(-50,0) pendown() # ending at right middle goto(100, 0) penup() # cutting the shape into two halves vertically # starting from upper middle goto (25,80) pendown() # ending at bottom middle goto(25,-80) done()
Output
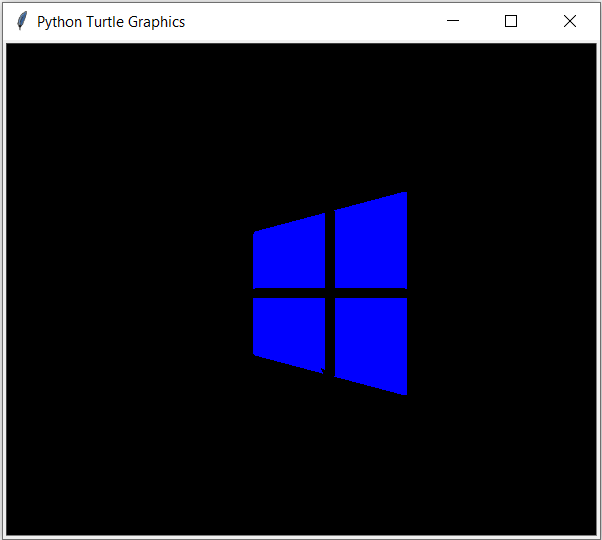
Thank you for reading this article.
Also read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle
- Draw Dell Logo using Python Turtle
- Draw Spider web using Python Turtle