While working with lists in python, you may have encountered a problem with splitting the list into equally-sized chunks. So, this article will help you learn different ways to split a list into equally-sized chunks.
Although I will provide a brief explanation of each method, it’s better for you to have a basic understanding of these concepts –
Let’s get into it!!
Method 1: Using loop with list slicing
Slicing is a method to take a small portion of the complete list. We can define the range of the elements that we want from the entire list using this syntax-
list[ start : end ]
Here, the start defines the starting index, and the end is the ending index+1 since the end index is exclusive.
For loop approach
We can use a for loop to iterate over the list and print a small part of size n in each iteration using list slicing. Here the for loop takes three parameters – start, end, and the last parameter n, which is the number of elements to skip at each iteration.
lst = [2, 4, 6, 8, 10, 12, 14, 16, 18] start = 0 #index of last element plus one because end index is exclusive end = len(lst) n = 3 #n is the number of steps to skip at each iteration for i in range(start, end, n): #set x to the current index i x = i #print a small part at each iteration print(lst[x:x+n])
Output:
[2, 4, 6] [8, 10, 12] [14, 16, 18]
While loop approach
The same logic can be implemented using a while loop like this –
lst = [2, 4, 6, 8, 10, 12, 14, 16, 18] start = 0 end = len(lst) n = 3 #set the loop index i to the start index i = start #loop through the list while i is less than the end index while i < end: #set x to the current index i x = i #print a small portion at each iteration print(lst[x:x+n]) #increment i by the step size i += n
Output:
[2, 4, 6] [8, 10, 12] [14, 16, 18]
Method 2: Using the yield keyword
The yield keyword is used to remember the current state of a function even after the function has returned back to the caller. This can be useful when you want to work with lots of data, but don’t want to slow down your computer by trying to store everything in memory at once.
In the below code, we define a function that takes two parameters, a list, and a size. Inside the function, a for loop is used to iterate over the list and list slicing is used to extract a portion of the list of specified size, starting from the current index, which is returned to the caller by using the yield keyword. The yield keyword pauses the execution of the function and returns the chunk to the caller. The caller’s for loop then prints this chunk and makes the next call to the function. The function then resumes from the point where it was paused and continues with the next index.
def break_list(lst, size): for i in range(0, len(lst), size): # Yield the sublist of the specified size yield lst[i:i+size] lst = [2, 4, 6, 8, 10, 12, 14, 16, 18] # Define the size of the sublists size = 3 # Loop through the sublists and print them for i in break_list(lst, size): print(i)
Output:
[2, 4, 6] [8, 10, 12] [14, 16, 18]
This way we can use the yield keyword to split a list into equally-sized chunks.
Method 3: Using List comprehension
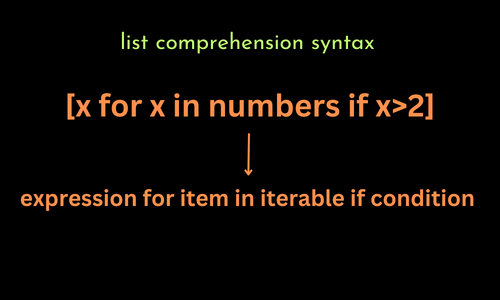
List comprehension can also be used to split a list into equally-sized chunks. List comprehension is a way to create a new list from an existing list. It allows you to write a single line of code instead of using a loop with append statement to create a new list.
lst = [1, 2, 3, 4, 5, 6, 7, 8, 9] size = 3 # Create the output list using a list comprehension final_list = [lst[i:i + size] for i in range(0, len(lst), size)] print(final_list)
Output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Method 4: Using the Numpy library
Numpy also known as Numerical Python, is a Python library to work with multidimensional arrays. Numpy is very famous for scientific computing in Python and it provides various useful methods to work with arrays efficiently. We can use Numpy’s array_split() method to split a list into equally-sized chunks of the given length.
The array_split() function takes two parameters, the list as the first parameter and the size of the chunks as the second parameter. It returns a list of Numpy array objects of the specified size, which is then stored in the answer list and printed.
import numpy as np lst = [1, 2, 3, 4, 5, 6, 7, 8, 9] # Split the list into chunks of size 3 chunks = np.array_split(lst, 3) print(chunks)
Output:
[array([1, 2, 3]), array([4, 5, 6]), array([7, 8, 9])]
Conclusion
In this article, you have learned a few ways of splitting a list into equal-sized chunks in Python. From using a simple approach like for/while loop to an advanced approach such as the Numpy method. I hope this helps you in working with lists in Python.
Thank you for visiting our website.
Also read:
- Python | Asking the user for input until they give a valid response
- Python | How to iterate through two lists in parallel?
- Python | How to sort a dictionary by value?
- Python | Remove items from a list while iterating
- Python | How to get dictionary keys as a list
- How to represent Enum in Python?
- 5 methods | Flatten a list of lists | Convert nested list into a single list in Python
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- Create your own ChatGPT with Python
- Filter List in Python | 10 methods
- Radha Krishna using Python Turtle
- Yield Keyword in Python
- Python Programming Examples | Fundamental Programs in Python
- Python | Delete object of a class
- Python | Modify properties of objects
- Python classmethod
- Python | Create a class that takes 2 parameters, print both parameters
- Python | Create a class, create an object of the class, access, and print property value
- Python | Calculator using lambda
- Python | Multiply numbers using lambda
- Python | Print Namaste using lambda
- Iterate over a string in Python
- Python | join() | Join list of strings
- Python | isalnum() method | check if a string consists only of alphabetical characters or numerical digits
- Python | isupper() and islower() methods to check if a string is in all uppercase or lowercase letters
- Python | count substring in a String | count() method
- Python | enumerate() on String | Get index and element
- Python | Convert a String to list using list() method
- Euler’s Number in Python