
Sometimes, we want to remove leading zeros (0s) from a string like “00098” becomes “98” only. In Python, there are several ways to do this. We will be discussing some of them in this article.
"00921" --> "921"
1. The int() method to remove leading zeros in Python
You can use the Python int() method to remove leading zeros from a string like this-
my_string = "000213"
my_string = int(my_string)
print(my_string)
Output:
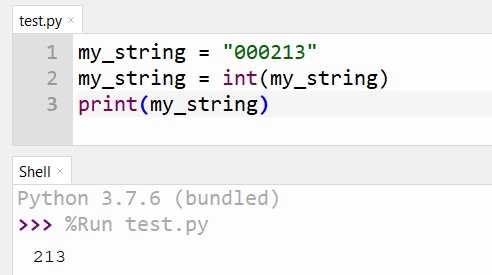
The first method is the int() method, which converts a string into an integer. While converting, it will automatically remove leading zeros in the string. Note that the string should only contain numbers and no letters, alphabets, or other symbols.
2. The lstrip() method to remove leading zeros
The strip() method takes a string argument. This method will remove the string given as the argument from the string in operation. When used, it automatically removes leading zeros ( only ) from the string. Note that this works for numbers and all characters accepted as a string. However, another method strip() will remove the leading and ending characters from the string. Python lstrip() docs.
You can use the Python lstrip() method to remove leading zeros from a string like this-
my_string = "00913"
my_string = my_string.lstrip('0')
print(my_string)
Output:
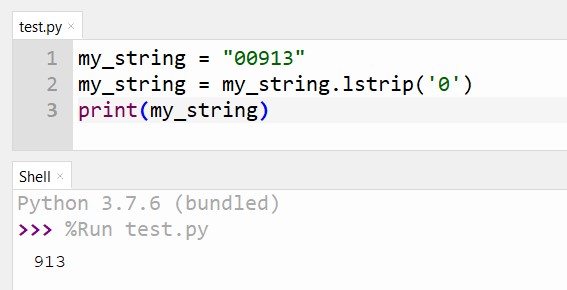
3. Reassigning until the character in the 0th index is “0”:
In this method, we will loop through a string and check whether the string’s first character is “0”. If the first character is “0,” then we will reassign the string from the first index. This is fairly simple and can be understood by looking at an example.
You can use the reassigning method to remove leading zeros from a string like this-
my_string = '0002134'
for i in my_string:
if i == '0':
my_string = my_string[1:]
print(my_string)
Output:
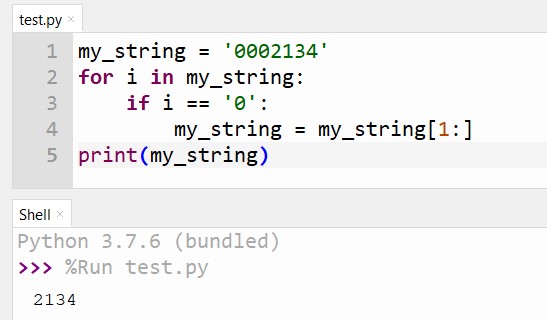
Output for the method of reassigning until the character in the 0th index is “0”
4. The startswith() method:
In this method, we will loop through a string and check whether the string’s first character is “0” with the startswith() method. This will return a boolean value, and we will use the same concept from 3rd way.
You can use the Python startswith() method to remove leading zeros from a string like this-
my_string = "00023"
for i in my_string:
if i == "0":
my_string = my_string[1:]
print(my_string)
>> 23
my_string = "00023"
for i in my_string:
if i == "0":
my_string = my_string[1:]
print(my_string)
>> 23
5. Using regex (Regular Expression) to remove leading zeros in Python string:
Python has a built-in Regular Expression module, namely, “re.” We can also remove the leading zeros from a string of numbers using this module. This is a simple task and can be understood once the code looks at.
The code:
import re
regex = "^0+(?!$)"
str = "000782"
str = re.sub(regex, "", str)
print(str)
Output:
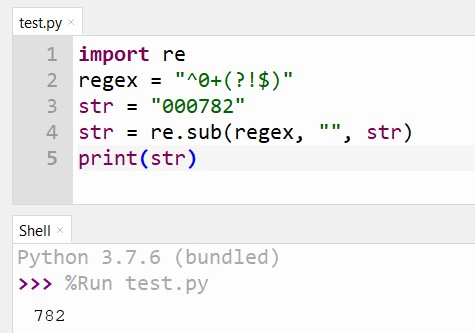
Possible Reasons to remove leading zeros or trailing zeros from a number or a string?
- For formatting time – 01:24 to 1:24
- For stripping IP Address
- For stripping units of data: 4000 to 4K
Checkout Short Video:
Keep Learning, Keep Coding
Also Read:
- Simple Code to compare Speed of Python, Java, and C++?
- Falling Stars Animation on Python.Hub October 2024
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value