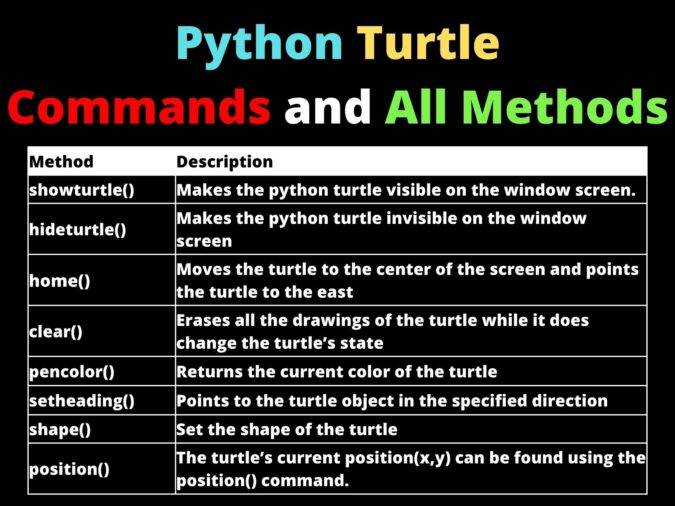
Introduction
In this tutorial, we are going to learn about the python turtle commands and methods which we are using in the python turtle programming where Turtle is a python library that is used in creating games, pictures, and graphic images.
If you have used Python Turtle a little bit, you may have observed that there are many commands i.e. methods. Those commands start from basic to advanced and we should learn at least basic Python turtle commands and methods to draw something using Python Turtle.
Here is the list of Python Turtle Commands and methods we are going to see in detail-
Method | Description |
---|---|
showturtle() | Makes the python turtle visible on the window screen. |
hideturtle() | Makes the python turtle invisible on the window screen. |
home() | Moves the turtle to the center of the screen and points the turtle to the east. |
clear() | Erases all the drawings of the turtle while it does change the turtle’s state. |
pencolor() | Returns the current color of the turtle |
setheading() | Points to the turtle object in the specified direction |
shape() | Set the shape of the turtle |
position() | The turtle’s current position(x,y) can be found using the position() command. |
goto() | Move the turtle from the current position to the given location i.e x,y |
begin_fill() | It just starts the filing operation in the turtle |
end_fill() | Fill the shape that is drawn after the last call to begin_fill() |
fillcolor() | Set the fillcolor or return the fillcolor |
dot() | Takes the size and the color as the parameters, draws the dot accordingly |
window_width() | Returns the width of the current window in pixel format |
up() | Makes Pen up and draw nothing on the screen until the down() command is called. |
down() | Puts the pen down on the screen and starts to draw while moving. |
forward() | Moves the turtle forward by the distance provided |
backward() | Moves the turtle backward by the distance provided |
color() | Changes the color of the turtle, default is black |
left() | Changes the direction of the turtle counterclockwise by the specified angle provided |
right() | Changes the direction of the turtle clockwise by the specified angle provided |
pensize() | Increases or decreases the size of the pen while drawing |
Python Turtle Commands and Methods
Now, we will see all Python Turtle commands i.e. all methods one by one.
Python Turtle commands showturtle()
Syntax
turtle.showturtle()
The showturtle() command makes the python turtle visible on the window screen.
Example
import turtle
turtle.showturtle()
turtle.done()
Output
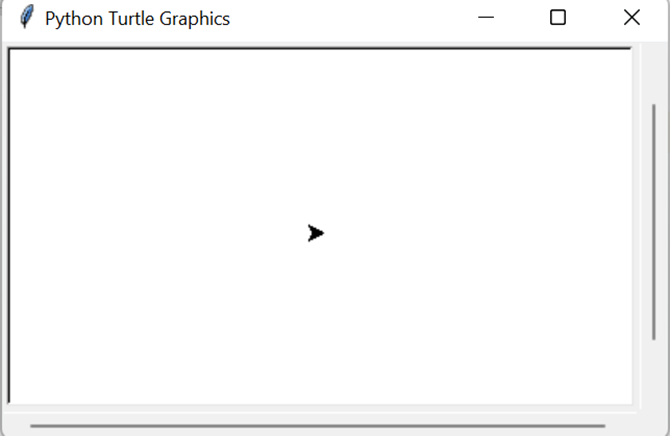
Python Turtle commands hideturtle()
Syntax
turtle.hideturtle()
The hideturtle() command makes the python turtle invisible on the window screen.
Example
import turtle
turtle.pencolor("red")
turtle.forward(50)
turtle.hideturtle()
turtle.done()
Output
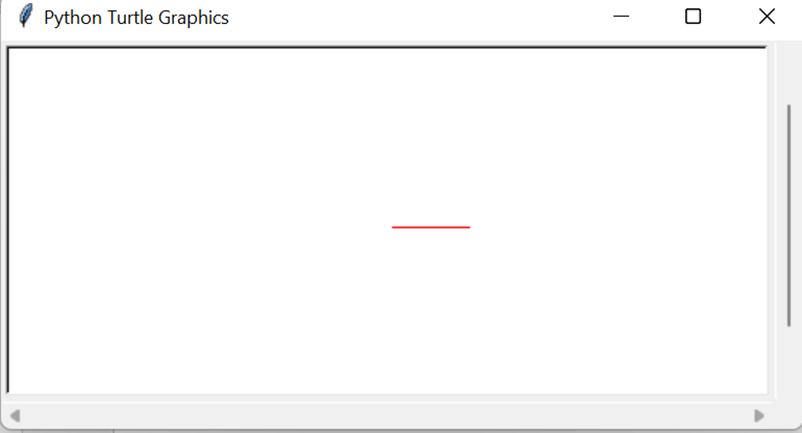
Python Turtle commands home()
Syntax
turtle.home()
The home() command moves the turtle to the center of the screen and points the turtle to the east.
Example
import turtle
turtle.pencolor("red")
turtle.forward(50)
turtle.home()
turtle.done()
Output

Python Turtle clear() Method
Syntax
turtle.clear()
The clear() method erases all the drawings of the turtle while it does change the turtle’s state.
Example
import turtle
turtle.pencolor("red")
turtle.forward(50)
turtle.clear()
turtle.done()
Output
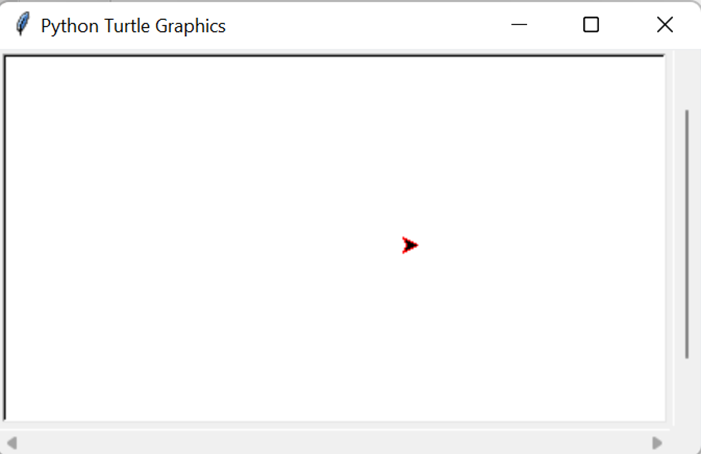
Python Turtle pencolor() method
Syntax
turtle.pencolor(string)
The pencolor() method returns the current color of the turtle when the arguments are not provided. The command changes the pencolor of the turtle to the specified RGB value of the specified string.
Example
import turtle
turtle.pencolor("red")
turtle.forward(30)
turtle.done()
Output

Python Turtle setheading() method
Syntax
turtle.setheading(degrees)
It points the turtle object in the specified direction. It is specified in degrees where 0 degrees is East, 90 degrees is north, 180 degrees is north and 270 degrees is south.
Example
import turtle
turtle.pencolor("red")
turtle.forward(50)
turtle.setheading(90)
turtle.done()
Output

Python Turtle shape() method
Syntax
turtle.shape("name of shape")
The shape() method in python programming language is used to set the shape of the turtle. In case the name of shape is not provided then it returns a default shape of the turtle. The name of shape accepts parameters such as circle, square, triangle, classic, and arrow.
Example
import turtle
turtle.shape("square")
turtle.pencolor("red")
turtle.forward(50)
turtle.done()
Output

Python Turtle position() method
Syntax
turtle.position()
The turtle’s current position(x,y) can be found using the position() method.
Example
import turtle
print(turtle.position())
turtle.done()
Output

Python Turtle goto() method
Syntax
turtle.goto(x,y)
The goto() command of the turtle is used to move the turtle from the current position to the given location i.e x,y along with the shortest linear path between the two locations where x and y are the coordinates of the screen.
Example
import turtle
turtle.goto(180,90)
turtle.done()
Output
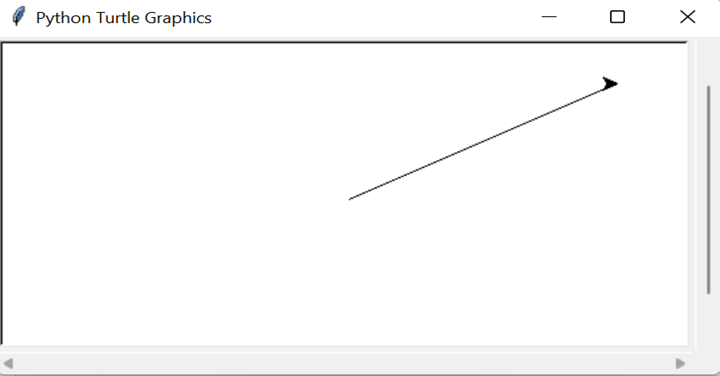
Python Turtle begin_fill() method
Syntax
turtle.begin_fill()
The begin_fill() command does not take arguments. It just starts the filing operation in the turtle. It needs to be called just before the drawing of the shape or the pattern.
Example
import turtle
turtle.color("red")
turtle.begin_fill()
turtle.circle(50)
turtle.end_fill()
turtle.done()
Output
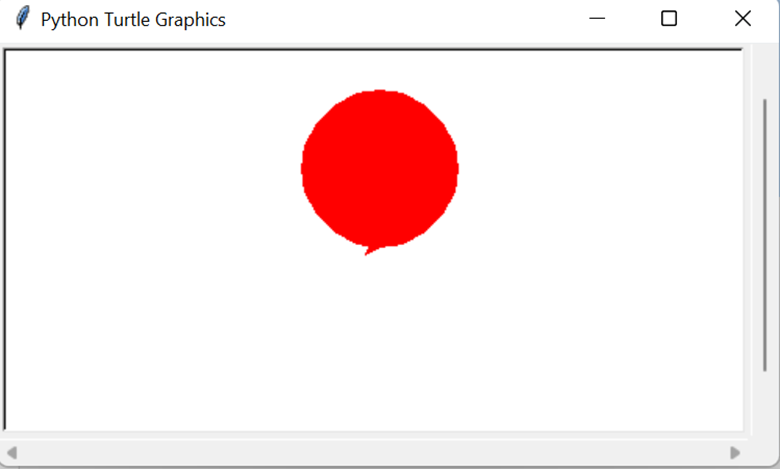
Python Turtle Commands end_fill()
Syntax
turtle.end_fill()
The end_fill() command does not take any arguments. This command is used to fill the shape that is drawn after the last call to begin_fill().
Example
import turtle
turtle.color("red")
turtle.begin_fill()
turtle.circle(50)
turtle.end_fill()
turtle.done()
Output

Python Turtle Commands fillcolor()
Syntax
turtle.fillcolor(*args)
The fillcolor() command is used to set the fillcolor or return the fillcolor. Suppose the turtle shape is square or polygon we can set the shape’s interior color with any valid color given in a color mode.
Example
import turtle
turtle.fillcolor("red")
turtle.begin_fill()
turtle.circle(50)
turtle.end_fill()
turtle.done()
Output
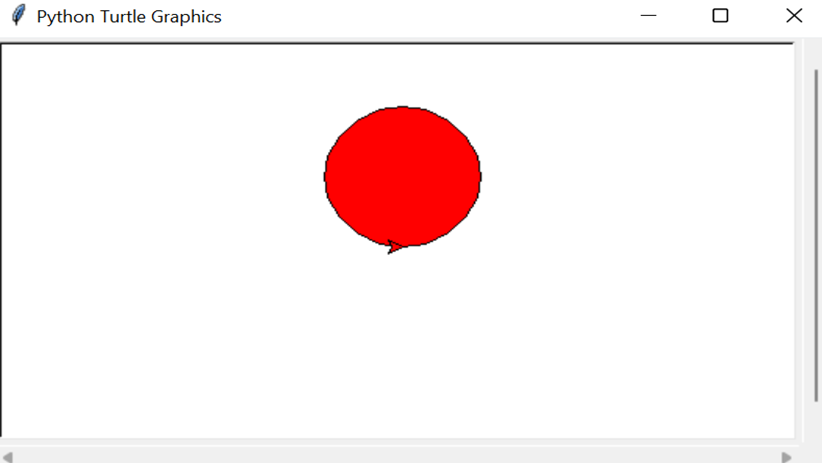
Python Turtle Commands dot()
Syntax
turtle.dot(size, *color)
This dot() command takes the size and the color as the parameters to draw a circular dot of the size provided and with the specified color at the current position.
Example
import turtle
turtle.dot(40,"green")
turtle.hideturtle()
turtle.done()
Output
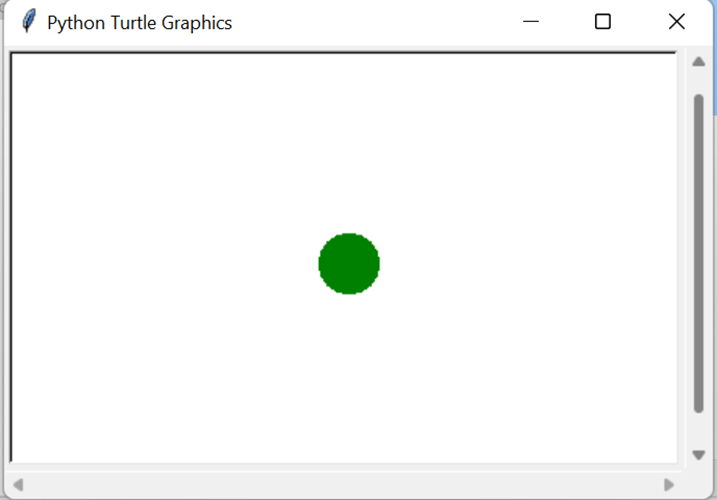
Python Turtle window_width() method
Syntax
turtle.window_width()
The window_width() is the method that does not require the argument. This method is used to return the width of the current window in pixel format.
Example
import turtle
print(turtle.window_width())
turtle.done()
Output

Python Turtle up() method
Syntax
turtle.up()
The up() method functions to put the pen up and draw nothing on the screen until the down() command is called. Hence this command stops all the drawings.
Example
import turtle
turtle.begin_fill()
turtle.circle(50)
turtle.end_fill()
turtle.up()
turtle.forward(60)
turtle.done()
Output

Python turtle down() method
Syntax
turtle.down()
The down() method is used to put the pen down on the screen and starts to draw while moving.
Example
import turtle
turtle.begin_fill()
turtle.circle(50)
turtle.end_fill()
turtle.down()
turtle.forward(60)
turtle.done()
Output
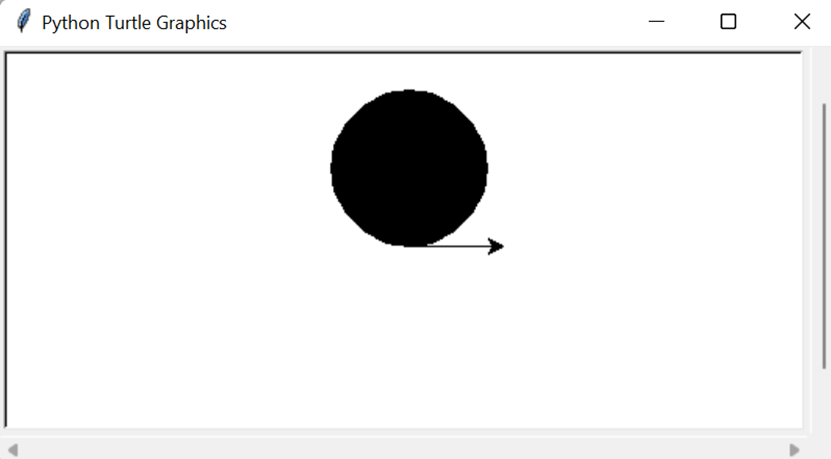
Python Turtle forward() method
Syntax
turtle.forward(distance)
This forward() method is used to move the turtle forward by the distance provided, where the direction of the turtle is headed. The distance parameter takes by how much distance the turtle will move ahead.
Example
import turtle
turtle.forward(80)
turtle.left(90)
turtle.forward(50)
turtle.done()
Output

Python Turtle backward() method
Syntax
turtle.backward(distance)
This backward() method is used to move the turtle backward by the distance provided i.e in the opposite direction of the turtle headed. The distance parameter takes by how much distance the turtle will move backward.
Example
import turtle
turtle.forward(80)
turtle.backward(50)
turtle.done()
Output

Python Turtle color() method
Syntax
turtle.color(*args)
The color() method of the python takes the default color as “black”. The args parameter is used to change the color of the turtle.
Example
import turtle
turtle.color("red")
turtle.circle(50)
turtle.done()
Output
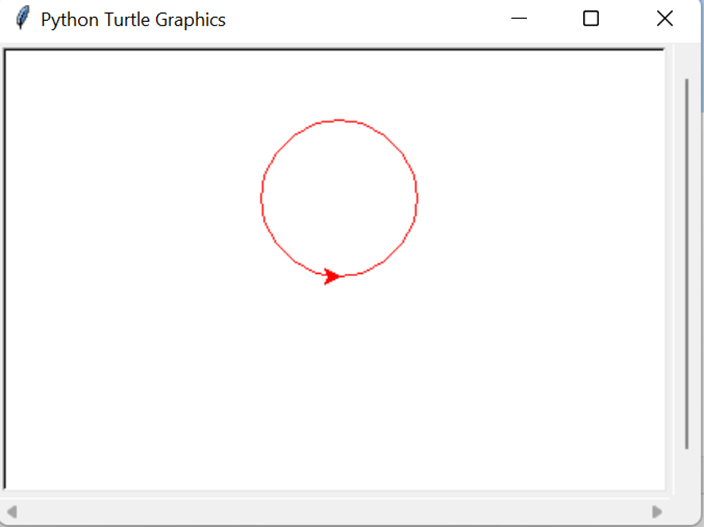
Python Turtle left() method
Syntax
turtle.left(angle)
The left() method is used to change the direction of the turtle counterclockwise by the specified angle provided. The angle parameter is required which mentions by what angle the turtle is moved left.
Example
import turtle
turtle.forward(80)
turtle.left(90)
turtle.forward(90)
turtle.done()
Output

Python Turtle right() method
Syntax
turtle.right(angle)
The right() method is used to change the direction of the turtle clockwise by the specified angle provided. The angle parameter is required which mentions by what angle the turtle is moved right.
Example
import turtle
turtle.forward(80)
turtle.right(90)
turtle.forward(90)
turtle.done()
Output

Python Turtle pensize() method
Syntax
turtle.pensize(size)
The pensize() method of the python turtle helps to increase or decrease the size of the pen while drawing. The size parameter gives the size of the pen we want to draw.
Example
import turtle
turtle.color("blue")
turtle.pensize(4)
turtle.forward(90)
turtle.left(30)
turtle.forward(50)
turtle.done()
Output
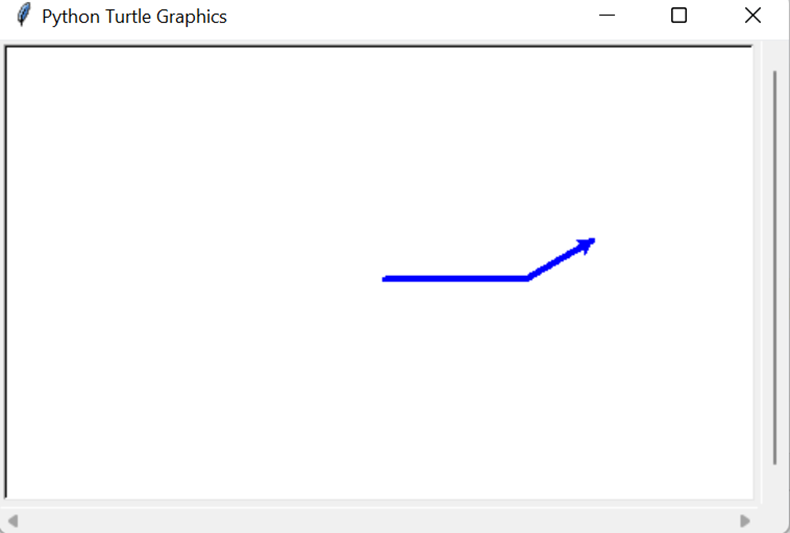
Conclusion
Here, we have covered a most of the important python turtle commands and methods that are required while doing python turtle programming.
Thank you for reading this article.
We hope this article on Python Turtle Commands and All Methods will Helps you.
Thank you for reading this article, click here to start learning Python in 2022.
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle
- Draw Dell Logo using Python Turtle
- Draw Spider web using Python Turtle