
This python turtle tutorial will help you understand every basic topic. There are many things you can do with Python and Design and Graphics is one of them. The Turtle module in Python uses another built-in module called “Tkinter” for its GUI. The turtle module in Python is generally taught to students who are a beginner in Python. By the end of this tutorial, you will be able to make most of the designs in your head with Python.
Requirements:
Turtle is a built-in module in Python like Tkinter, Random, etc. This is why we should not install it using pip. However, even if you get an error “NoSuchModuleError“, you can install it using the following command inside the terminal.
pip install turtle
Getting Started:
We will learn the Python Turtle module by designing some shapes like the Circle, Rectangle, Triangle, etc. Here are a few simple uses of the turtle module with the code and the output.
Python Turtle: Circle Shape
# first of all, import the turtle module
import turtle
# initalize the turtle
t = turtle.Turtle()
radius = 50 #declaring the unit of radius
t.circle(radius) #calling the circle(built-in) method

Python Turtle: Square Shape
# first of all, import the turtle module
import turtle
# initalize the turtle
t = turtle.Turtle()
length = 100 #declaring the length of the square(all sides are equal)
for x in range(4): #loop for 4 times
t.forward(length) #moving the pen forward at length unit
t.left(90) #moving the pen left at an angle of 90 degrees

Python Turtle: Rectangle Shape
# first of all, import the turtle module
import turtle
# initalize the turtle
t = turtle.Turtle()
length = 100
breadth = 150
#drawing 1st line
t.forward(length) # Forward turtle by length units
t.left(90) # Turn turtle by 90 degree
#drawing 2nd line
t.forward(breadth) # Forward turtle by breadth units
t.left(90) # Turn turtle by 90 degree
#drawing 3rd line
t.forward(length) # Forward turtle by length units
t.left(90) # Turn turtle by 90 degree
#drawing 4th line
t.forward(breadth) # Forward turtle by breadth units
t.left(90) # Turn turtle by 90 degree
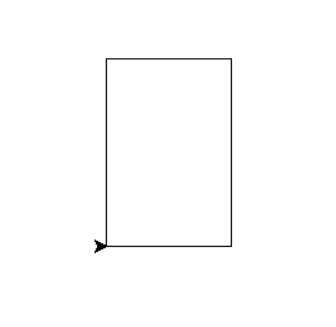
Python Turtle: Triangle Shape
# first of all, import the turtle module
import turtle
# initalize the turtle
t = turtle.Turtle()
unit = 300
angle = 120
#drawing the first line
t.forward(unit) #going forward with the "unit" unit
t.left(angle) #turning left at an angle of "angle"
#drawing the second line
t.forward(unit) #going forward with the "unit" unit
t.left(angle) #turning left at an angle of "angle"
#drawing the third line
t.forward(unit) #going forward with the "unit" unit
t.left(angle) #turning left at an angle of "angle"

Move the Turtle
- forward (the parameter is unit)
- backward (the parameter is unit)
- left (the parameter is an angle)
- right (the parameter is an angle)
Example:
# first of all, import the turtle module
import turtle
# initalize the turtle
t = turtle.Turtle()
t.forward(100)
#move the turtle right at 100 units
t.left(60)
#set the turtle left at an angle of 60 degrees
t.backward(100)
#move the turtle backwards at 100 units
t.right(60)
#set the turtle right at an angle of 60
t.left(120)
#set the turtle left at 120
t.forward(100)
#move the turtle 100 units and a triangle will be formed
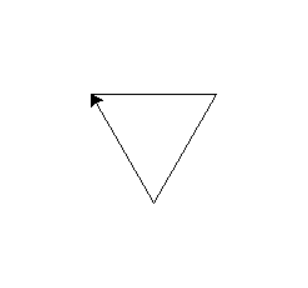
Replacements for above commands:
t.rt()
{replaced for right()}t.fd()
{replaced for forward()}t.lt()
{replaced for left()}t.bk()
{replaced for backward()}
Understanding the Quadrants:
Quadrants in Python Turtle are the same as in mathematics but here, we will be understanding it in the context.
The turtle method used in this context is “goto()” and the parameters of “goto()” are the coordinates.
Example:
import turtle
turtle.goto(100, 100)
#Taking to the given co-ordinates
turtle.goto(100, 0)
#Taking to the x-line of the co-ordinate
turtle.goto(0, 0)
#Taking the origin which will complete the triangle
turtle.goto(90, 0)
#making the perpendicular sign before 10 units
turtle.goto(90, 10)
#goint to 10 units above
turtle.goto(100, 10)
#going 10 units right
turtle.hideturtle()
#hiding the turtle
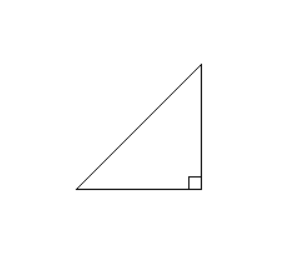
Here, we created a right-angled triangle without using the right, back, forward, or left methods.
Basic methods in Turtle:
Change the background color
You can change the background color of the screen to any color with the method bgcolor(). While changing the background, if you don’t specify the color to fill inside the shape, the shape will be covered in the same color too. You can reference it to as in the following example.
import turtle
turtle.bgcolor("red") #changing the background
turtle.circle(200) #drawing a circle with the radius 200
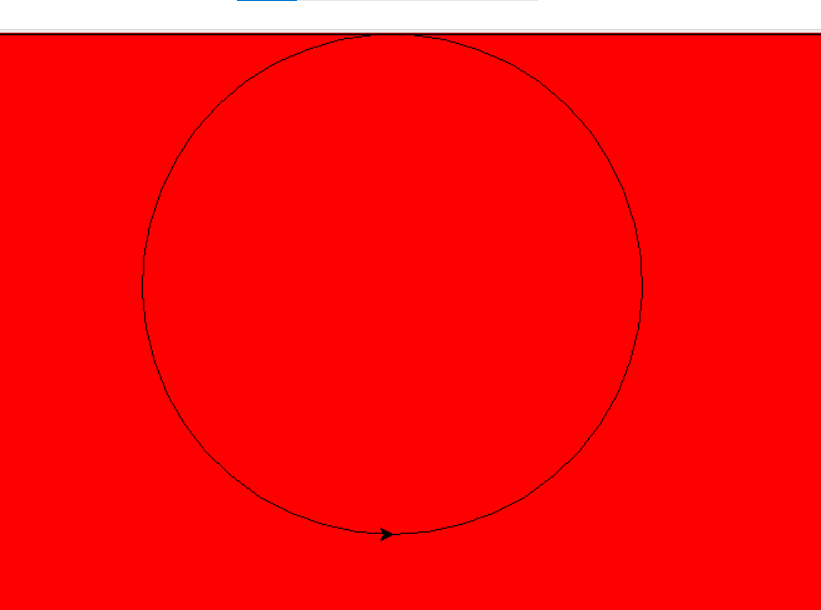
Change the color of the pen
You can change the color of the pen in python turtle with the method pencolor(). This method takes a parameter that refers to the desired color. You can set the color either by just giving the name of the color as a string. Or you can give the RGB code. When giving the RGB code, you give three parameters as numbers which are then separated by a comma. You can understand it more clear from the following example.
import turtle
turtle.colormode(255) #setting the colormode to 255 which is black
turtle.backward(100) #moving the turtle backward at 100 units
turtle.pencolor("red") #setting the pencolor to red
turtle.left(90) #setting the pen left at an angle of 90 degrees
turtle.backward(100) #moving the pen backward at a unit of 100
turtle.pencolor((41,41,253)) #setting the pencolor to blue with RGB code
turtle.left(90) #setting the pen left at an angle of 90 degrees
turtle.backward(100) #moving the code backward at 100 units
turtle.pencolor(41,253,41) #setting the pen color to green in RGB code.
turtle.left(90) #setting the pen left at an angle of 90 degrees
turtle.backward(100) #moving the turtle backward at 100 units
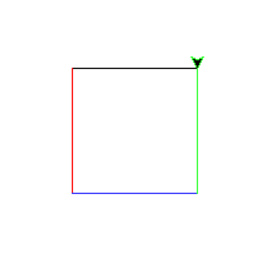
Fill the shape with a different color
You can fill color to a drawn shape with the method fill_color(). Give the parameter as the desired color. But, you should also use the methods begin_fill() and end_fill(). You can see it in the example below.
import turtle
turtle.begin_fill()
#begin the fill for the shape
turtle.fillcolor("green")
#set the color to fill as green
turtle.circle(50)
#create a circle with the radius of 50 units
turtle.end_fill()
#end the fill which will create a green circle

Change the speed of the turtle
You can change the turtle’s speed too with the method speed() where you will send the parameter as the desired speed. If the speed is set too high, then the drawing will not be seen properly, and the output will be shown directly.
import turtle
turtle.speed(30)
#setting the speed to 30
for i in range(6):
#loop 5 times as it is a pentagon
turtle.forward(100)
#moving the turtle forward at 100 units
turtle.left(60) #setting the turtle left at an angleof 60 degrees
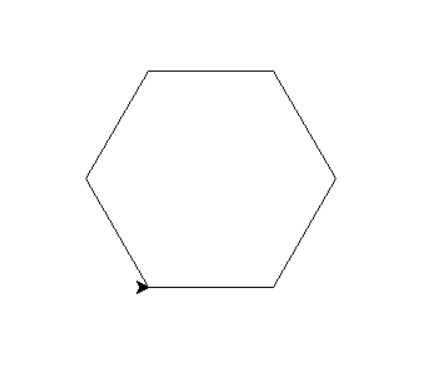
Change the title of the GUI
You can change the GUI title you have created with the method title() where you should give the parameter as the desired title name like in the example below.
import turtle
turtle.title("My first turtle GUI")
#Setting the title to "My first turtle GUI"

Change the size of the pen
You can even change the pen’s size to your desire, as in the code below, where you will give the parameter as a number.
import turtle
turtle.pensize(5)
#Setting the pen size to 5
turtle.forward(99)
#Moving the pen forward at the unit of 99
turtle.left(90)
turtle.forward(99)

Clear the GUI
If you have practiced a lot and your screen looks messy, then you can clear the GUI with the method clear() as in the example below
import turtle
turtle.circle(50) #creating a circle with the radius of 50 units
turtle.clear() #clearing the screen after creating the circle
Creating a dot
With the dot() method, you can create a dot. You can use the dot method with the parameter, which should be a number that defines the radius of the “dot” like in the following sample code.
import turtle
radius = 100 #Setting the value of radius variable to 100
turtle.dot(radius)
#Creating a turtle with the radius of "radius"

Stamping in the middle
You can create a stamp wherever the pen goes with the method stamp(), and there is no parameter to give. You can reference it as in the example below.
import turtle
turtle.stamp() #leaving a stamp of the turtle
turtle.fd(100) #moving forward at 100 units
turtle.stamp() #leaving a stamp of the turtle
turtle.fd(100) #moving forward at 100 units
turtle.stamp() #moving forward at 100 units

Change Shape of Pen
Likewise, if you change the turtle’s shape, then the stamp will also be changed like in the following example.
import turtle
turtle.shape("turtle") #setting the shape of the turtle to "turtle"
turtle.stamp() #leaving a stamp of the turtle
turtle.fd(100) #moving forward at 100 units
turtle.stamp() #leaving a stamp of the turtle
turtle.fd(100) #moving forward at 100 units
turtle.stamp() #moving forward at 100 units

Moreover, if you want to clear all the stamps, then use the clearstamp() method, and the parameter should be the id of the stamp.
import turtle
turtle.shape("turtle")
turtle.stamp() #leaving a stamp of the turtle
turtle.fd(100) #moving forward at 100 units
turtle.stamp() #leaving a stamp of the turtle
turtle.fd(100) #moving forward at 100 units
turtle.stamp() #moving forward at 100 units
turtle.clearstamp(5)
#clearing the stamp with id of 5
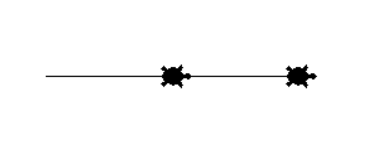
Positioning the turtle
There are some methods in this module that will help you position your turtle as desired. Some of the methods are discussed below.
- setpos(): This method takes two arguments “x” which is the number of vectors, and “y” which means the number of None. As the “y” will represent None, the x will represent the coordinates extracted from the pos() method.
- setx(): This method is used when we need to change the coordinate of “x” but the co-ordinate of “y” should remain unchanged. The argument is either an integer or a float number.
- sety(): This method is very similar to the setx() method. We use this method when we have to change the “y” co-ordinate and leave the x coordinate the same. The argument is either an integer or a float number.
- setheading()/seth(): The new version accepts the seth(). This method is used to set the angle of the turtle. Here, the argument is a number that will define the angle desired.
- home(): This method is used when the turtle is required to come to the origin or the (0, 0) coordinates and will set the heading as default.
- pos()/position(): This method is used when we need the turtle’s position. The pos() is used in the newer version.
Change the shape of the turtle
1. Turtle
import turtle
turtle.shape("turtle") #declaring the shape of the turtle as "turtle"

2. Arrow
import turtle
turtle.shape("arrow") #Declaring the shape of the turtle as arrow

3. Square
import turtle
turtle.shape("square")
#Declaring the shape of the turtle as square

Note: There are many other values for the shape of the turtle like triangle, classic, circle.
Methods to control the turtle vision
There are some methods that are used to control the turtle visually. Some of them are discussed below.
- hideturtle()/ht(): This method is used when the drawing is to be done, but the turtle should be invisible. There are no arguments to be given. The newer version supports the method ht().
- showturtle()/st(): This method is used when we need the make the turtle visible if the turtle was hidden before. There are no arguments used. The newer version supports the st() method.
- isvisible(): This method is used when we need to confirm if the turtle is hidden or not. This method will give an output as a boolean. If the turtle is hidden, the value will be False, else True.
Python Turtle: Spiral Triangle Project
import turtle as t
#importing the module as "t"
def spiral_shape(p,c):
#creating a function with parameters "p" and "c"
if p > 0:
#if the p is less than 0,
t.forward(p)
#moving the turtle forward at p units
t.right(c)
#setting the turtle right at an angle of c
spiral_shape(p-5,c)
#calling the function with the arguments as given
t.shape('classic')
#setting the shape of the turtle as "classic"
t.reset()
#resetting the turtle
t.pen(speed=25)
#setting the speed of the pen to 25
length = 400
#declaring a variable "length" to 400
turn_by = 121
#declaring a varibale "turn_by" to 121
t.penup()
#the drawing is not ready
t.setpos(-length/2, length/2)
#setting the position as given
t.pendown()
#starting to draw
spiral_shape(length, turn_by)
#calling the function with the given arguments

Explanation
- First, import the turtle library and create a function name spiral_shape and give the parameters “p” and “c.” Inside this function, check if the “p” is greater than 0. If so, then move the turtle forward at p units and set the turtle right at an angle of c. At last, call the spiral_shape function with the arguments “p-5” and “c.”
- Coming out of the function, set the turtle’s shape to “classic” and reset it. Now, set the speed of the pen to 25.
- Likewise, declare a variable “length” setting the value to 400 and declare another variable named “turn_by” and set the value to 121.
- Similarly, call the penup() method, which means it is not the time to start drawing. Then, set the position to (-length/2, length/2)
- Accordingly, call the pendown() method, which will start the drawing.
- Lastly, call the spiral_shape() function with the arguments “length” and “turn_by”
Thank You for reading till the end. We have many other small turtle projects on this website which you can visit yourself.
For more help, please watch this video
Keep Learning, Keep Coding
Tell us if you found something wrong in this article.
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle