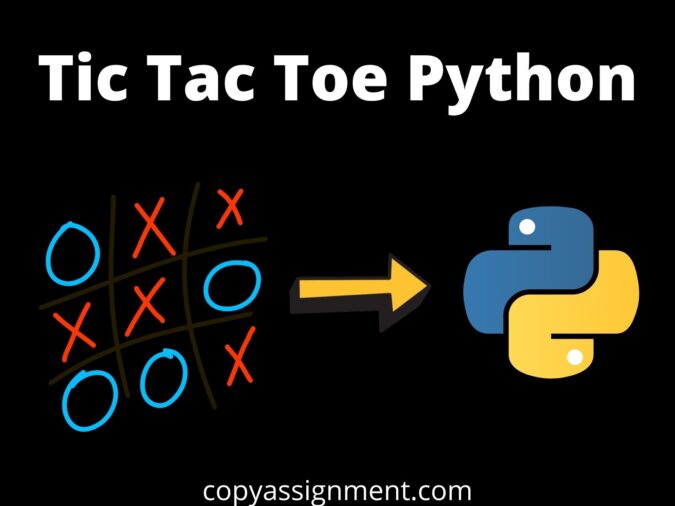
Tic Tac Toe Python, is what we are going to do in this post. Tic tac toe is a simple game that most if not everyone knows it. You can call it X’s and O’s game too.
How it is played
Two people play the game. They place either an x or an o in a grid of three by three. You win by placing your letter to fill a complete row or column or diagonally across the sudoku field.
Playing against a computer
Playing such a game against a computer involves implementing a sort of AI within the program. The AI makes moves in a manner similar to how a human will and should be simple and reasonable enough. So to sum it up, you shall be playing against a computer AI.
Definitions
Functions are defined in python using the ‘def’ word. We shall use a number of functions in our Tic Tac Toe Python program since a lot of code are reusable and will actually be reused several times to ensure the game runs. The functions are as follows:
1. The blank board
This is a variable declaration more than a function. We create a blank board with the spaces for placing the “x’s” and “o’s”. It is mostly a three-by-three board with blank slots.
board = [' ' for x in range(10)]
2. The board printing function
This function will print out to the board each time an ‘x’ or an ‘o’ is placed on the board. The board will look presentable on any shell or idle you run it on.
def printBoard(board):
print(" " + board[1] + "| " + board[2] + "| " + board[3])
print("---------")
print(" " + board[4] + "| " + board[5] + "| " + board[6])
print("---------")
print(" " + board[7] + "| " + board[8] + "| " + board[9])
The board variables are actual values saved in the board array.
3. A function to check if the board is full
We need to constantly need to check if the board is entire to end the game. If the board is full then it’s a tie. The board can lack to be full with no winner declared and hence a move can be made. The function is simple and returns a true or a false. This function will be called every time before a move is made.
def isBoardFull(board):
if board.count(" ") > 1:
return False
else:
return True
4. A function to check if there is a winner
If the board isn’t full, we then check if there is a winner. we declare a winner if three “x’s” or three “o’s” occur in one row or in a column or diagonally.
def isWinner(bo, le):
return (bo[7] == le and bo[8] == le and bo[9] == le) or
(bo[4] == le and bo[5] == le and bo[6] == le) or
(bo[1] == le and bo[2] == le and bo[3] == le) or
(bo[1] == le and bo[4] == le and bo[7] == le) or
(bo[2] == le and bo[5] == le and bo[8] == le) or
(bo[3] == le and bo[6] == le and bo[9] == le) or
(bo[1] == le and bo[5] == le and bo[9] == le) or
(bo[3] == le and bo[5] == le and bo[7] == le)
#Note that all this code falls on line.
#Do not go to the next line.
#"bo" stands for the board.
#"le" stands for the letter or symbol we're checking.
5. The insert letter function
We set a bias that the human user will always be the first to start and he will be using ‘x’. The user should then insert a number position on the board in which the ‘x’ will be placed. The number position is similar to the “T9” format and hence you insert a number between one and nine inclusive.
def insertLetter(letter, pos):
board[pos] = letter
This a simple function that inserts letters “x” and “o” to a position chosen either by the user or the computer AI
6. A function to check if the position is free
We define a function that checks if the position chosen by the user or the computer AI is free. It is a boolean function and will return true or false depending on the status of the position.
def spaceIsFree(pos):
return board[pos] == " "
7. A function for the player to move
This function prompts the user to enter a position on the board in which an x is to be placed. It checks if the user’s input is valid. If the input is valid, the position itself is checked for its status, either occupied or empty. It saves the letter in that position if it checks out with the two cases. If it doesn’t pass one case, then an error is thrown back respectively This means that if it is occupied, the user is alerted and told to pick a different number. If the user inputs a larger number, he/she is prompted to enter a valid number.
def playMove():
run = True
while run:
move = input("Please select position for 'X'(1-9): ")
try:
move = int(move)
if move > 0 and move < 10 :
if spaceIsFree(move):
run = False
insertLetter("x", move)
else:
print("Sorry this space is occupied")
else:
print("Please type the number within the range")
except:
print("Please type a number. ")
8. The AI function for the computer
This is the function that picks out the option the computer will make. Simply, it will first take a check for the available spaces and store the positions in a variable.
It will then check if it has any possible move that will allow it to win. If so then it will place its letter there.
If not, it will then check if the opponent, which is you, is about to win and if so, it then will counter and place a letter to block you from winning.
When neither is true, then it is free to place a letter anywhere in the available slots. However, to make it have some logic, it will set a letter randomly at the corners, if the corners themselves are empty. If not then it will proceed to pick the middle slot if opens.
When none of the cases above are true then it will randomly pick an empty edge slot and place its letter there.
def compMove():
possibleMoves = [x for x, letter in enumerate(board) if letter == ' ' and x != 0]
#The above line justs checks for empty slots in the board
move = 0
for let in ['o','x']:
for i in possibleMoves:
boardCopy = board[:]
boardCopy[i] = let
if isWinner(boardCopy, let):
move = i
return move
#This checks if one of the players has a wining move
cornersOpen = []
for i in possibleMoves:
if i in [1,3,7,9]:
cornersOpen.append(i)
#This checks for open corners
if len(cornersOpen) > 0:
move = selectRandom(cornersOpen)
return move
if 5 in possibleMoves:
move = 5
return move
This checks for open middle slot.
edgesOpen = []
for i in possibleMoves:
if i in [2,4,6,8]:
edgesOpen.append(i)
if len(edgesOpen) > 0:
move = selectRandom(edgesOpen)
return move
9. Select a random function
This function just simply selects a random position on the board for the computer to place its letter. This makes the AI unpredictable in some sense.
It is implemented in the AI function defined earlier. To produce a random number, we will use the random module.
def selectRandom(li):
#li is the list of open spots.
import random
ln = len(li)
r = random.randrange(0, ln)
return li[r]
10. The main function
The main function calls all the other functions in order. It is the backbone of the game and forms a chronological syntax of functions and other selected cases.
def main():
print("welcome tic tac toe")
printBoard(board)
#prints out the board
while not(isBoardFull(board)):
#This runs when the board isn't full
if not(isWinner(board, "o")):
playMove()
printBoard(board)
else:
print("Sorry, O's won this time! ")
break
#This checks if the computer has won
if not(isWinner(board, "x")):
move = compMove()
#The computer checks if it can make a move
if move == 0:
print("Tie game")
else:
insertLetter("o", move)
print("Comp placed an 'o' in position", move, ":")
printBoard(board)
else:
print("X's won this time! Good job")
break
#This checks if the user has won
#if the board is the board is full then it is tie
if isBoardFull(board):
print("Tie game")
The main function is a little complicated and requires you to go through it thoroughly line by line block by block. All we need to do now is to call our main function to run the game.
main()
Complete code for Tic Tac Toe Python
Let us now see the full code of the game combined into one .py file.
Remember that you can store the functions in different files but you will have to import them in the main function. However, to make the game simple and readable, we store all the code in one file
board = [' ' for x in range(10)] def insertLetter(letter, pos): board[pos] = letter def spaceIsFree(pos): return board[pos] == " " def printBoard(board): print(" " + board[1] + "| " + board[2] + "| " + board[3]) print("---------") print(" " + board[4] + "| " + board[5] + "| " + board[6]) print("---------") print(" " + board[7] + "| " + board[8] + "| " + board[9]) def isWinner(bo, le): return ((bo[7] == le and bo[8] == le and bo[9] == le) or (bo[4] == le and bo[5] == le and bo[6] == le) or (bo[1] == le and bo[2] == le and bo[3] == le) or (bo[1] == le and bo[4] == le and bo[7] == le) or (bo[2] == le and bo[5] == le and bo[8] == le) or (bo[3] == le and bo[6] == le and bo[9] == le) or (bo[1] == le and bo[5] == le and bo[9] == le) or (bo[3] == le and bo[5] == le and bo[7] == le)) def playMove(): run = True while run: move = input("Please select a position to place an 'X' (1-9): ") try: move = int(move) if move > 0 and move < 10 : if spaceIsFree(move): run = False insertLetter("x", move) else: print("Sorry this space is occupied") else: print("Please type the number within the range") except: print("Please type a number. ") def compMove(): possibleMoves = [x for x, letter in enumerate(board) if letter == ' ' and x != 0] move = 0 for let in ['o','x']: for i in possibleMoves: boardCopy = board[:] boardCopy[i] = let if isWinner(boardCopy, let): move = i return move cornersOpen = [] for i in possibleMoves: if i in [1,3,7,9]: cornersOpen.append(i) if len(cornersOpen) > 0: move = selectRandom(cornersOpen) return move if 5 in possibleMoves: move = 5 return move edgesOpen = [] for i in possibleMoves: if i in [2,4,6,8]: edgesOpen.append(i) if len(edgesOpen) > 0: move = selectRandom(edgesOpen) return move def selectRandom(li): import random ln = len(li) r = random.randrange(0, ln) return li[r] def isBoardFull(board): if board.count(" ") > 1: return False else: return True def main(): print("welcome tic tac toe") printBoard(board) while not(isBoardFull(board)): if not(isWinner(board, "o")): playMove() printBoard(board) else: print("Sorry, O's won this time! ") break if not(isWinner(board, "x")): move = compMove() if move == 0: print("Tie game") else: insertLetter("o", move) print("Computer placed an 'o' in position", move, ":") printBoard(board) else: print("X's won this time! Good job") break if isBoardFull(board): print("Tie game") main()
To get an in-depth understanding of how to create a tic-tac-toe game in python, watch the videos below.
Part 1
Part 2
Thanks for reading through my article.
Please comment below if you liked the article or found something wrong with it. You can also comment on your queries.
You can check other posts below:
Sudoku solver Python |Backtracking|Recursion
Create Language Translator Using Python